Challenges of Blue-Green Deployment Using a Single Database
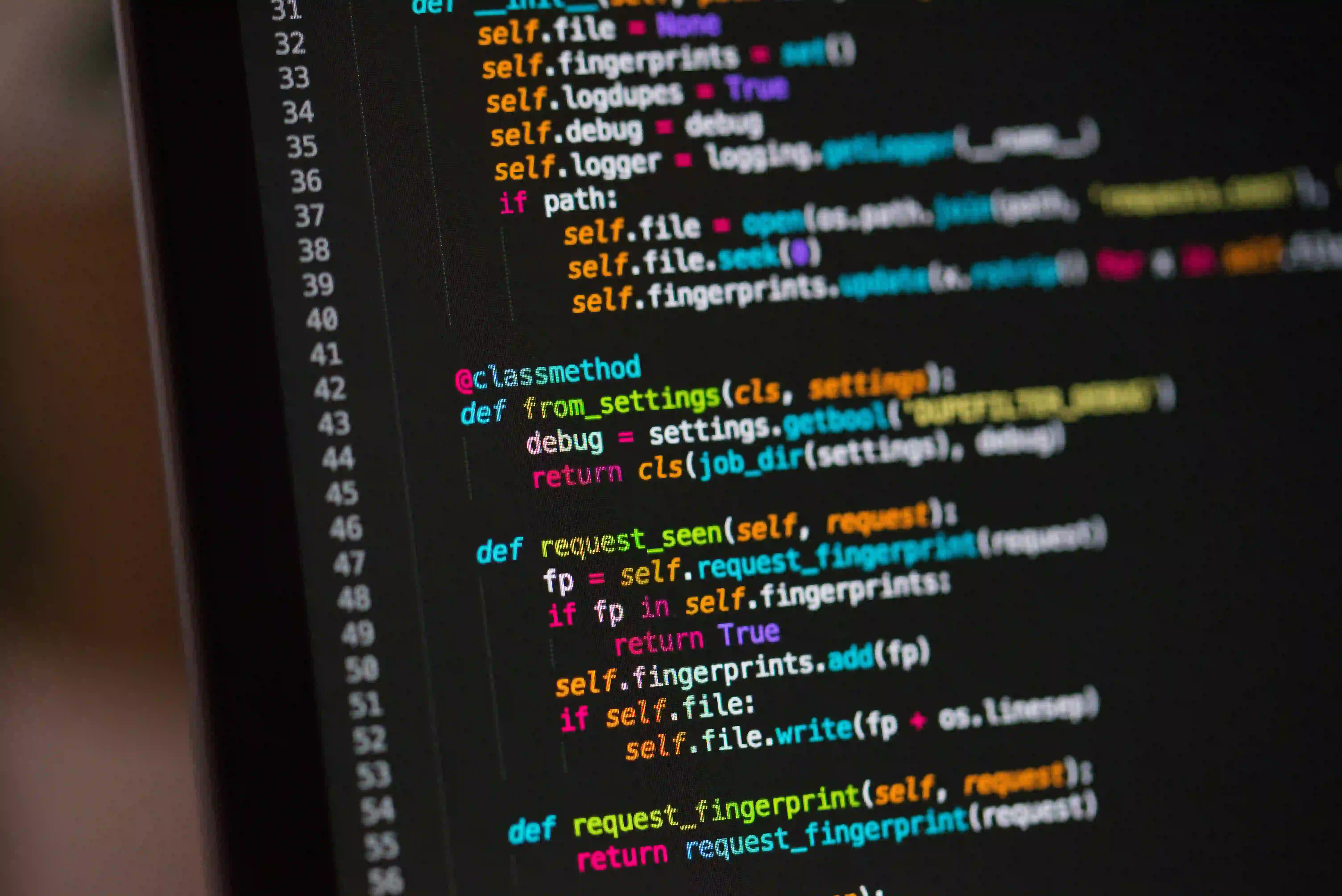
Challenges of Blue-Green Deployment Using a Single Database
In the world of continuous deployment and microservices architecture, blue-green deployment has emerged as a popular strategy for minimizing downtime and risks. This technique involves maintaining two identical environments: one termed "blue" (the live environment) and the other "green" (the staging environment). While the advantages of this approach are well documented, itβs essential to examine the challenges that arise specifically when both environments share a single database.
In this blog post, we will explore the implications of using a blue-green deployment strategy with a single database, addressing potential issues and offering solutions. By the end, you should have a deeper understanding of how to implement this strategy effectively, alongside practical code snippets to illustrate key concepts.
What is Blue-Green Deployment?
Blue-green deployment refers to the practice of running two identical production environments. At any time, one of these environments (the blue) serves all user traffic while the other (the green) is utilized to stage and test new releases. When the new version is ready, traffic is switched over to the green environment, allowing for seamless updates without downtime.
Benefits of Blue-Green Deployment
- Zero Downtime: Instantaneous switch between environments minimizes downtime.
- Easy Rollbacks: If issues are detected after switchover, reverting to the previous version is straightforward.
- Parallel Testing: The green environment can be used for testing new features without affecting production.
Core Challenges of a Single Database
While blue-green deployments can significantly enhance the deployment process, there are notable challenges when working with a shared database. Here are several key issues developers might encounter:
1. Schema Migrations
One of the primary challenges is database schema migrations. When deploying changes that modify database schemas, both environments need to be in sync.
Example Code Snippet: Schema Migration with Liquibase
<databaseChangeLog
xmlns="http://www.liquibase.org/xml/ns/dbchangelog"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.liquibase.org/xml/ns/dbchangelog
http://www.liquibase.org/xml/ns/dbchangelog/dbchangelog-3.8.xsd">
<changeSet id="1" author="user">
<createTable tableName="new_table">
<column name="id" type="int">
<constraints primaryKey="true" />
</column>
<column name="name" type="varchar(255)" />
</createTable>
</changeSet>
</databaseChangeLog>
Why? This code uses Liquibase to create a new table schema. However, if the blue environment is currently live, deploying this migration can break the app if not accounted for correctly, causing issues for users.
Solution
To mitigate this, adopt a backward-compatible migration strategy. Introduce changes in two phases: first, add the required columns or tables; next, deploy the application code that utilizes those changes.
2. Data Consistency
Maintaining data integrity becomes complex with simultaneous deployments, especially when the two environments might need to read/write to shared data.
Example Code Snippet: Using Versioning
Imagine two versions of a service that reads user data.
public User getUser(Long userId) {
return jdbcTemplate.queryForObject("SELECT * FROM users WHERE id = ?", new Object[]{userId}, new UserRowMapper());
}
Why? A change in the user table structure can lead to discrepancies. If the blue service reads new fields from the shared database that are not yet available for green, inconsistency arises.
Solution
Adopt read-safe coding practices. Ensure both environments can handle different schemas gracefully by implementing versioning in your API. This ensures that when the blue environment makes requests, it can handle both old and new data structures.
3. Feature Flags & Toggle Management
Feature flags are an essential part of agile development. However, handling them in a blue-green deployment scenario with a shared database could lead to confusion.
Example Code Snippet: Configuring Feature Flags
public class FeatureToggle {
private final Set<String> enabledFeatures = new HashSet<>();
public void enable(String feature) {
enabledFeatures.add(feature);
}
public boolean isEnabled(String feature) {
return enabledFeatures.contains(feature);
}
}
Why? The above class offers a simple way to manage feature toggles. However, if the flag states differ between the blue and green environments, you could deploy features that don't yet exist in the database.
Solution
Maintain global feature toggle management in a centralized location, ensuring that both environments read from the same configuration. This method ensures consistency and reduces potential errors.
4. Data Migration and Cleanup
Sometimes, during an upgrade, you may need to migrate certain data from older formats to newer ones. If not managed well, it can lead to runtime errors.
Example Code Snippet: Data Migration Process
public void updateUserData() {
// Suppose we need to migrate user status
jdbcTemplate.update("UPDATE users SET status = 'ACTIVE' WHERE last_login > NOW() - INTERVAL '30 days'");
}
Why? While migrating, if both environments try to write to the same data concurrently, it may lead to inconsistent application behavior.
Solution
Perform database migrations and data cleanup in a controlled manner. A best practice is to run such scripts only in the green environment and then switch traffic once validation is complete.
Final Considerations
The blue-green deployment pattern, while powerful, presents unique challenges when managed on a single database. By addressing schema migrations, data consistency, feature flags, and data migrations, you can enhance the reliability and effectiveness of your deployments.
Key Takeaways
- Implement backward-compatible migrations to prevent breaking changes.
- Use versioning and read-safe practices to maintain data integrity.
- Centralize feature flag management for uniformity across environments.
- Safeguard migrations and data processes in a controlled manner.
For further reading, consider checking out Martin Fowler's article on Feature Toggles and Red Hat's guide on Blue-Green Deployments.
By applying best practices and remaining conscious of these challenges, you can confidently leverage blue-green deployment within your development strategy. Happy coding!