Overcoming Common Spring Boot Database Integration Issues
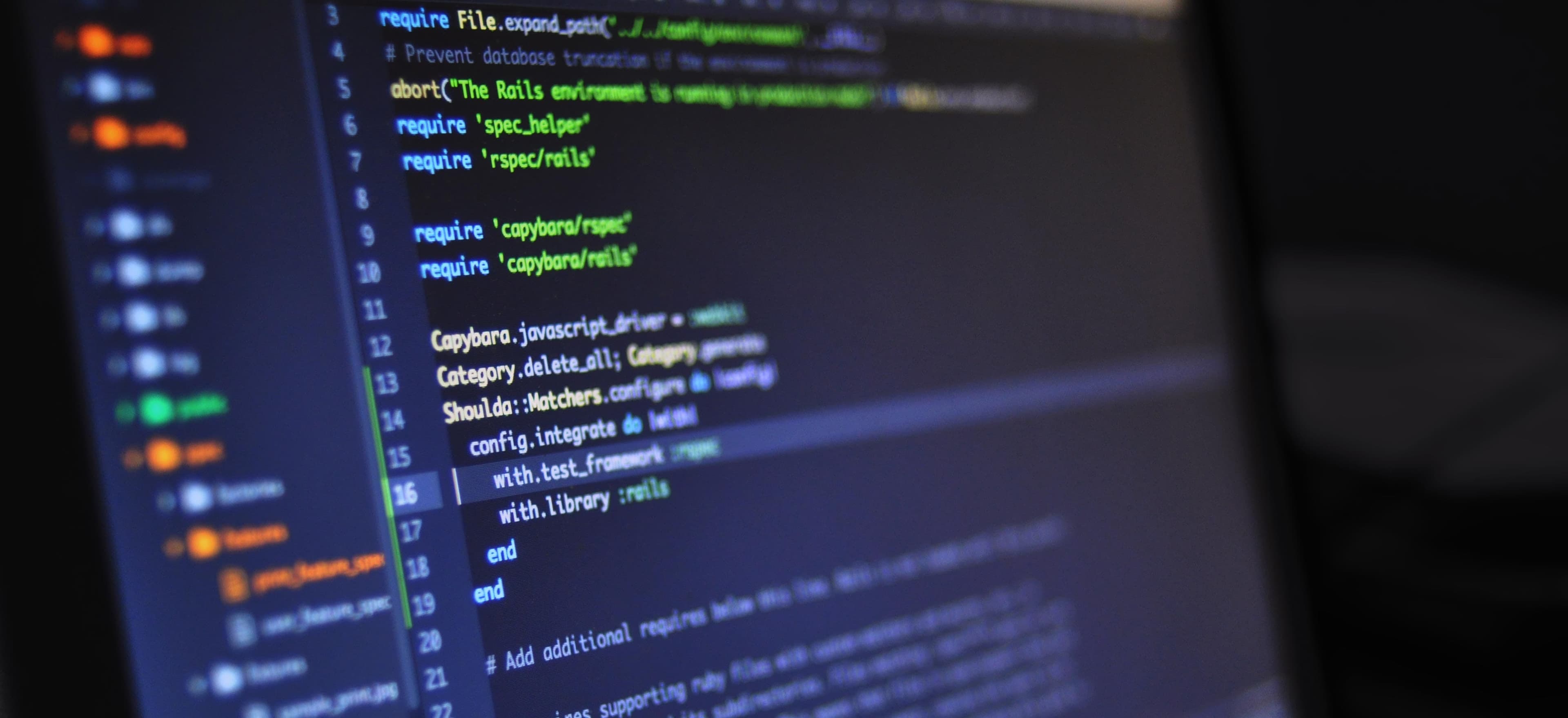
- Published on
Overcoming Common Spring Boot Database Integration Issues
Spring Boot has become the go-to framework for building enterprise-level applications. Its seamless integration with various databases simplifies development. However, as with any software solution, developers often face database integration issues. In this blog post, we'll discuss common Spring Boot database integration problems and how to resolve them effectively.
Understanding Spring Boot Database Integration
Before we dive into the issues, let's briefly understand how Spring Boot integrates with databases. Spring Boot uses Spring Data JPA, which provides a simplified way to interact with databases using Java Persistence API (JPA). By leveraging JPA and Hibernate, developers can perform operations on a database without writing complex SQL queries.
Here’s how a typical database configuration looks in Spring Boot. This example assumes you are using MySQL:
application.properties
spring.datasource.url=jdbc:mysql://localhost:3306/your_database
spring.datasource.username=your_username
spring.datasource.password=your_password
spring.jpa.hibernate.ddl-auto=update
spring.jpa.show-sql=true
These configurations establish a connection to your MySQL database. The ddl-auto
set to update
allows Hibernate to auto-generate the schema which is great for development but should be configured differently in production.
Now, let's explore the common issues and solutions.
1. Dependency Issues
Problem
One of the first hurdles developers encounter is dependency issues. If the necessary dependencies are not included in the pom.xml
or build.gradle
files, you may face runtime errors when trying to run database-related functionality.
Solution
Ensure you have included the following dependencies in your pom.xml
for Maven:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<scope>runtime</scope>
</dependency>
For Gradle, your build.gradle
should include:
implementation 'org.springframework.boot:spring-boot-starter-data-jpa'
runtimeOnly 'mysql:mysql-connector-java'
This step is critical to ensure Spring Boot recognizes your database and JPA capabilities.
2. Database Connection Issues
Problem
Upon starting your Spring Boot application, you may encounter the dreaded "Cannot connect to database" error. This can occur due to incorrect configuration or a misbehaving database server.
Solution
To troubleshoot database connectivity, follow these steps:
- Check the database server to make sure it is running.
- Validate the connection string in your configuration file.
- Ensure the username and password are correct.
Here is a snippet for double-checking the connection:
spring.datasource.url=jdbc:mysql://localhost:3306/your_database
spring.datasource.username=your_username
spring.datasource.password=your_password
If everything seems correct and the issue persists, try connecting to the database using another client (like MySQL Workbench) with the same credentials to rule out connection issues.
3. Wrong Entity Mapping
Problem
Another common issue is when the entity is not correctly mapped to the database table. This often leads to runtime errors like Unable to locate appropriate constructor
.
Solution
Verify that your entity class is annotated correctly. Here’s an example:
@Entity
@Table(name = "users")
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(name = "username", nullable = false, unique = true)
private String username;
@Column(name = "password", nullable = false)
private String password;
// Getters and Setters
}
Make sure that:
- You have the
@Entity
annotation to define the class as a JPA entity. - The table name matches your database.
- The fields are correctly annotated with
@Column
.
If the mappings are incorrect, Hibernate won't know where to store or retrieve data properly.
4. Transaction Management Issues
Problem
You might also encounter issues related to transactions, particularly when working with multiple repositories or data operations. Failures can occur if the transactions are not managed correctly, resulting in data inconsistency.
Solution
Use Spring's @Transactional
annotation to demarcate methods or classes in which transactions should be managed. Here is an example:
@Service
public class UserService {
@Autowired
private UserRepository userRepository;
@Transactional
public void registerUser(User user) {
// perform any necessary validations
userRepository.save(user);
}
}
This ensures that if an exception occurs during the execution of the registerUser
method, all database modifications will be rolled back, maintaining data integrity.
5. Data Not Found
Problem
When you attempt to fetch data, a common issue is that the expected records cannot be found. This might raise NoSuchElementException
or return null values.
Solution
Check your repository methods to ensure they are implemented correctly. Using Spring Data JPA's standard query methods can reduce risks. For example:
public interface UserRepository extends JpaRepository<User, Long> {
Optional<User> findByUsername(String username);
}
Using Optional
helps in avoiding null pointer exceptions. Further, consider logging the SQL queries if you suspect malformed queries.
6. Performance Issues
Problem
As the application scales, developers may notice performance degradation during database interactions. This can stem from a lack of proper indexing or inefficient queries.
Solution
Regularly analyze and optimize your SQL queries. Use JPA's criteria queries or Hibernate's criteria builder to create dynamic queries. Furthermore, ensure that your database schema is optimized:
- Indexing: Add indexes to frequently queried columns.
- Batch Processing: For bulk operations, switch to batch processing.
Here is how you can use EntityGraph
to optimize fetch joins:
@Entity
@NamedEntityGraph(
name = "userWithRoles",
attributePaths = {"roles"}
)
public class User {
//...
}
This allows you to fetch users along with their roles in a single query, significantly reducing database roundtrips.
7. Inconsistent Application State
Problem
In sophisticated applications, you might find that the application state becomes inconsistent due to various factors, including improper session handling with long-running transactions.
Solution
Implement proper session management by configuring the following in your application properties:
spring.jpa.properties.hibernate.current_session_context_class=thread
This ensures that Hibernate uses the same session within the same thread, preventing state inconsistency due to simultaneous access.
Final Thoughts
Spring Boot eases the database integration process significantly, enabling developers to focus on creating robust applications. However, it is vital to be aware of and tackle potential issues that may arise. By understanding the configurations, ensuring proper dependency management, and following best practices, you will be well-equipped to overcome common database integration issues.
For further reading on specific areas, consider checking out Spring Data JPA documentation or Hibernate documentation.
By tackling these challenges diligently, you enhance the resilience and performance of your Spring Boot applications. Happy coding!