Overcoming Latency Issues in Debezium with PostgreSQL and Redis
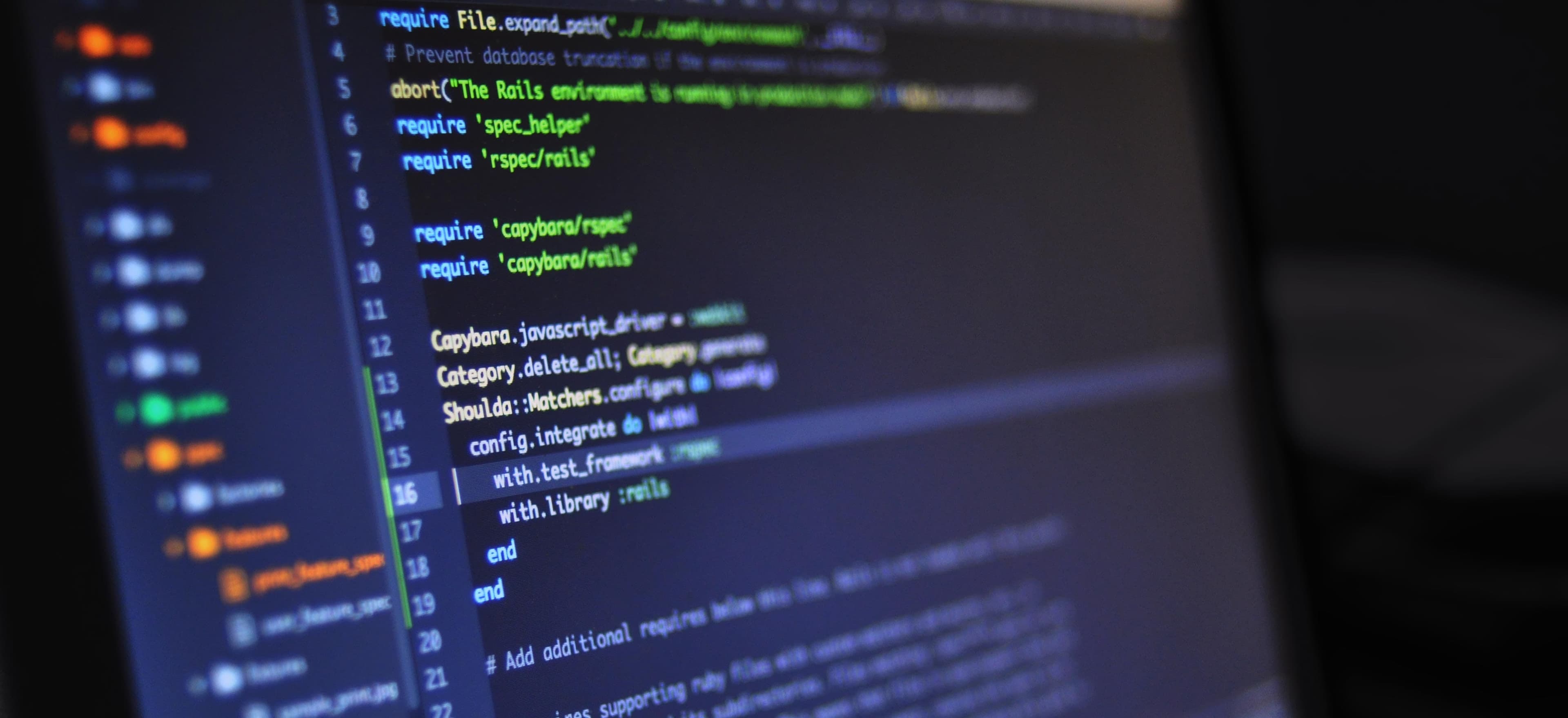
- Published on
Overcoming Latency Issues in Debezium with PostgreSQL and Redis
Latency is an inevitable challenge in distributed systems, especially when working with change data capture (CDC) tools such as Debezium. Coupled with robust databases like PostgreSQL and caching solutions like Redis, it's crucial to understand how to mitigate these latency issues effectively. This blog post will discuss strategies for optimizing performance and maintaining swift data synchronization across your architectures.
Understanding Debezium, PostgreSQL, and Redis
What is Debezium?
Debezium is an open-source project that provides a platform for CDC. It captures row-level changes in your databases and streams them in real-time. By doing so, it essentially allows you to sync data between the database and various applications.
Why PostgreSQL?
PostgreSQL is a powerful, open-source relational database known for its robustness, extensibility, and SQL compliance. It has built-in support for logical replication, making it a favorable choice for CDC tools like Debezium.
Redis as a Caching Layer
Redis is an in-memory data structure store, often used as a database, cache, and message broker. With its high-performance characteristics, it is ideal for applications requiring low-latency data access.
Common Latency Issues with Debezium
- Network Latency: The time it takes for data to travel across networks can introduce delays.
- Data Transformation Overhead: Transforming captured data before sending it to downstream consumers can incur additional latency.
- Database Load: High transaction volume or complex queries in PostgreSQL can slow down change data capture.
- Processing Throughput: The rate at which Debezium can process changes can impact the overall performance.
Given these challenges, let's explore strategic approaches to mitigate latency when using Debezium with PostgreSQL and Redis.
Strategies for Reducing Latency
1. Optimize PostgreSQL Performance
To ensure PostgreSQL is running at peak performance, follow these practices:
- Indexing: Use indexing on commonly queried columns. This can significantly speed up read operations, allowing Debezium to capture changes quickly.
CREATE INDEX idx_example ON your_table (column_name);
Commentary: Indexes allow the database to locate data quickly. In CDC scenarios, this can reduce the load and capture time.
- Connection Pooling: Use a connection pool (such as PgBouncer) to manage database connections efficiently. This reduces the overhead of establishing new connections.
2. Reduce Change Data Capture Frequency
Debezium captures every change on the table. However, this can lead to excessive load. Consider these adjustments:
- Aggregate Changes: Instead of sending every individual change, aggregate changes and send them in batches.
// Pseudo-code Example for aggregating changes
List<ChangeRecord> changeBatch = new ArrayList<>();
while (hasMoreChanges()) {
ChangeRecord record = getNextChange();
changeBatch.add(record);
if (changeBatch.size() >= BATCH_SIZE) {
sendBatch(changeBatch);
changeBatch.clear();
}
}
Commentary: Batching reduces the number of messages sent to Redis, thus minimizing network overhead.
3. Utilize Redis for Caching
Use Redis effectively to cache frequently used data. This can significantly lower latency for read operations by reducing the number of times the application needs to interact with PostgreSQL.
Implementing Redis with Debezium
- Data Publishing: Whenever a change is detected by Debezium, publish it to Redis.
import redis.clients.jedis.Jedis;
public void publishChange(ChangeRecord record) {
try (Jedis jedis = new Jedis("localhost")) {
jedis.publish("changes", record.toJson());
}
}
Commentary: The Redis publish
command allows you to stream changes to connected subscribers, minimizing the need to hit the database directly for frequent reads.
- Subscriber Pattern: Connect your application to Redis to listen for changes.
import redis.clients.jedis.Jedis;
import redis.clients.jedis.JedisPubSub;
public class ChangeSubscriber extends JedisPubSub {
@Override
public void onMessage(String channel, String message) {
// Update local cache or perform necessary operations
}
}
// Usage
Jedis jedis = new Jedis("localhost");
jedis.subscribe(new ChangeSubscriber(), "changes");
Commentary: By subscribing to updates, your application can seamlessly remain in sync without constantly querying the database.
4. Asynchronous Processing
Implement asynchronous processing to handle changes from Debezium more efficiently.
// Using CompletableFuture for async processing
public CompletableFuture<Void> processChange(ChangeRecord record) {
return CompletableFuture.runAsync(() -> {
// Process record here
});
}
Commentary: Asynchronous processing allows your system to handle multiple records at once, significantly reducing overall latency.
5. Configure Advanced Debezium Settings
Adjust Debezium's settings for optimal performance:
- Max Batch Size: Increase the maximum batch size to allow Debezium to send changes in larger chunks.
- Snapshot Mode: Depending on your system requirements, adjusting the snapshot mode can reduce initial load time.
{
"snapshot.mode": "schema_only",
"max.batch.size": 2048
}
Commentary: This configuration allows for tailored performance based on your application's needs.
Monitoring and Troubleshooting
Monitoring is essential when optimizing performance. Tools such as PostgreSQL Enterprise Manager or Redis Monitoring can help identify bottlenecks.
Additionally, you can enable Debezium's logging to get more insight into processing times.
# In your Debezium connector properties
log.level=DEBUG
The Bottom Line
By combining Debezium, PostgreSQL, and Redis effectively, you can significantly overcome latency issues that arise in CDC operations. Implementing strategies like database optimization, change aggregation, caching, asynchronous processing, and advanced Debezium configurations will lead to improved performance and a more responsive application.
For further reading on similar topics, consider exploring:
- Debezium Documentation
- PostgreSQL Performance Tuning
- Redis as a Caching Layer
By proactively addressing these latency issues, your systems can become more efficient, resulting in a better user experience. Start implementing some of these strategies today and watch your performance improve!
Checkout our other articles