Overcoming Common Challenges When Writing NetBeans Plugins
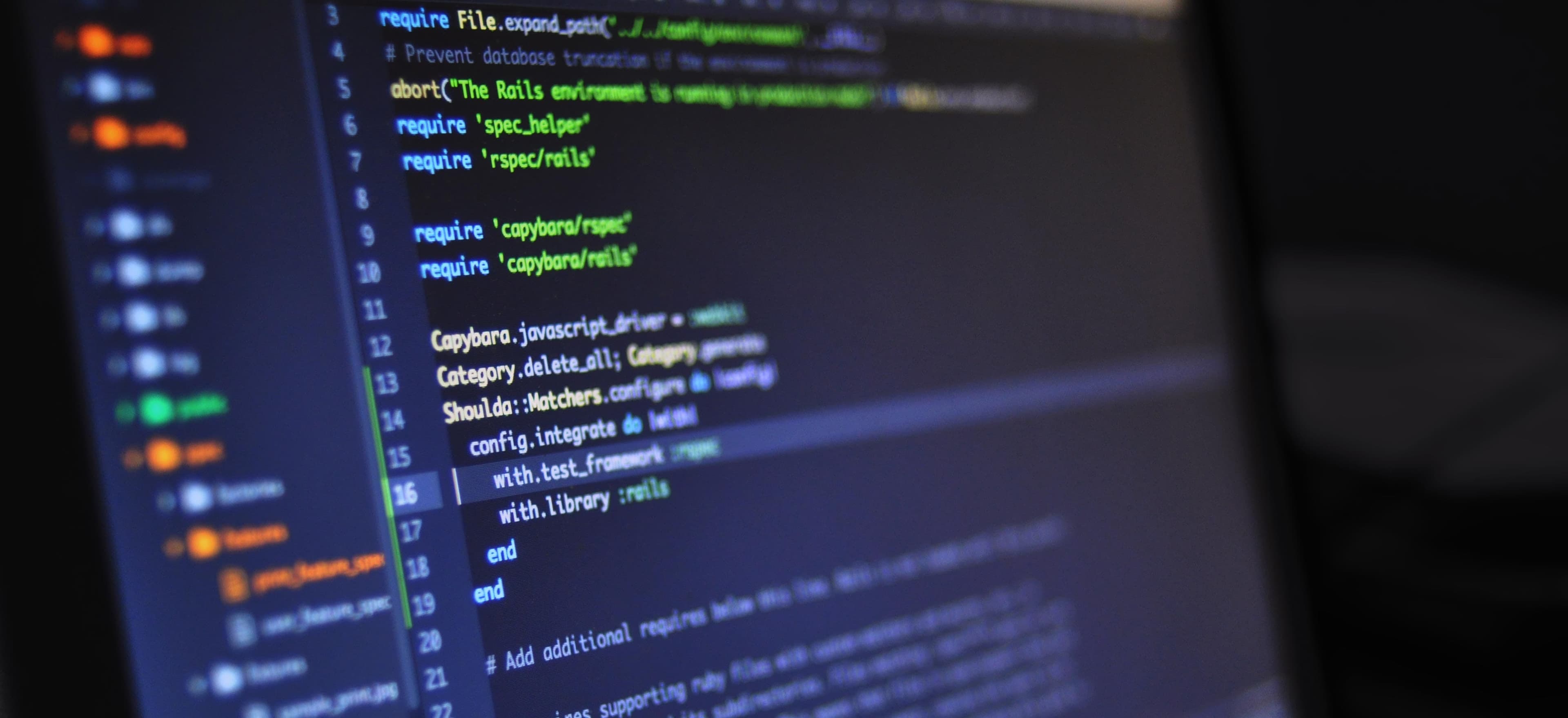
- Published on
Overcoming Common Challenges When Writing NetBeans Plugins
NetBeans is a powerful Integrated Development Environment (IDE) primarily used for Java development. One of its strong suits is its extensibility. Developers can create plugins to enhance NetBeans functionality tailored to specific needs. Yet, crafting a plugin isn't always smooth sailing. In this blog post, we will delve into common challenges faced while writing NetBeans plugins and provide useful strategies to overcome them.
Understanding the NetBeans Platform
Before we dive deep into challenges, let’s briefly examine the NetBeans architecture. It is built on the NetBeans Platform, which follows a modular design. This means that various features can be developed and deployed as独立 modules or plugins. If you’re new to the NetBeans Platform, you might want to explore the NetBeans Plugin Development Documentation.
1. Getting Started with Your Plugin
Challenge: Initial Setup and Configuration
Setting up the NetBeans development environment for plugin creation can be a daunting task for newcomers.
Solution: Pre-built Development Setup
To quickly get started, consider downloading the NetBeans Platform SDK. This SDK is a comprehensive tool that provides templates, sample code, and documentation to aid your development process. You can download the SDK via the Apache NetBeans Releases page.
Code Snippet:
Here's a simple code snippet to create a new NetBeans module:
@ModuleManifest
module my.sample.module {
requires org.openide.modules;
}
Explanation: This code snippet creates a new module called my.sample.module
. The requires
keyword indicates that our module depends on the org.openide.modules
package, which is essential for most NetBeans modules.
2. Understanding Module Dependencies
Challenge: Dependency Management
For a plugin to function correctly, managing dependencies can be quite challenging. It can lead to issues during installation if a required module is not available.
Solution: Use the NetBeans Module Dependency System
NetBeans facilitates dependency management through its @ModuleManifest
. Make sure to specify all your required modules in this file.
Code Snippet:
@ModuleManifest
module my.sample.plugin {
requires org.openide.awt;
requires org.openide.util;
provides org.openide.awt.ActionProcessor with MyActionProcessor;
}
Explanation: This shows how to specify that your plugin (my.sample.plugin
) requires org.openide.awt
and org.openide.util
. The provides
directive indicates a service that your module will implement.
For detailed guidance on module dependencies, refer to NetBeans Module Dependencies Documentation.
3. User Interface Design
Challenge: Designing the UI
Creating an intuitive user interface can be more complicated than it seems. It must blend well with the existing NetBeans UI while keeping the user experience in mind.
Solution: Utilize NetBeans UI Components
Instead of designing your UI from scratch, leverage existing NetBeans UI components. This ensures consistency with the IDE’s look and feel.
Code Snippet:
JPanel panel = new JPanel();
JButton button = new JButton("Click Me");
button.addActionListener(e -> System.out.println("Button Clicked!"));
panel.add(button);
Explanation: This snippet creates a simple GUI containing a button. When clicked, it prints a message to the console. The use of standard Swing components ensures it integrates well within the NetBeans environment.
Learn more about building UIs in NetBeans at NetBeans UI Development.
4. Handling Persistence
Challenge: Data Persistence and Management
Plugins often require data storage and retrieval, which involves managing persistent storage effectively.
Solution: Use the NetBeans Utilities
NetBeans provides various mechanisms for storing data, including files and databases. Use the Preferences
API for simple key-value storage.
Code Snippet:
Preferences prefs = Preferences.userNodeForPackage(MyClass.class);
prefs.put("key", "value");
Explanation: In this snippet, we store a key-value pair in user preferences corresponding to a certain user or application setting. This is a simple yet effective way to maintain user-specific configurations.
For more on data persistence, consult the NetBeans Persistence Documentation.
5. Debugging and Testing
Challenge: Debugging Plugin Issues
Debugging can be laborious, especially with the intricacies of plugin modules and their interactions.
Solution: Use the Built-in Debugger
NetBeans comes with a powerful built-in debugger that you can utilize for plugin development. Set breakpoints, step through the code, and inspect variables.
Code Snippet for Setting a Breakpoint:
System.out.println("Debugging...");
Explanation: By including simple logging statements, you can better trace the execution flow. Utilize breakpoints through the IDE interface for a more advanced debugging experience.
Check out NetBeans Debugging Documentation for more information on debugging techniques.
6. Documentation and Community Support
Challenge: Lack of Resources and Examples
Even with the available documentation, it's possible to find yourself stuck without a clear solution or example code.
Solution: Engage with the Community
Leverage forums and community support. The NetBeans community is vibrant, and places like Stack Overflow or NetBeans mailing lists can be invaluable resources.
In Conclusion, Here is What Matters
Writing NetBeans plugins can be an exquisite journey rich with learning experiences. By being aware of common challenges such as module dependencies, UI design, persistence management, and debugging, you can save time and enhance your productivity.
Remember, practice makes perfect. Experiment with existing plugins, consult the wealth of resources provided by the NetBeans community, and, most importantly, enjoy the process of creating!
For further reading, explore the official NetBeans Plugin Portal to discover existing plugins and gather insights for your own development endeavors.
Happy coding!
Checkout our other articles