Common Pitfalls When Implementing the Template Method Pattern
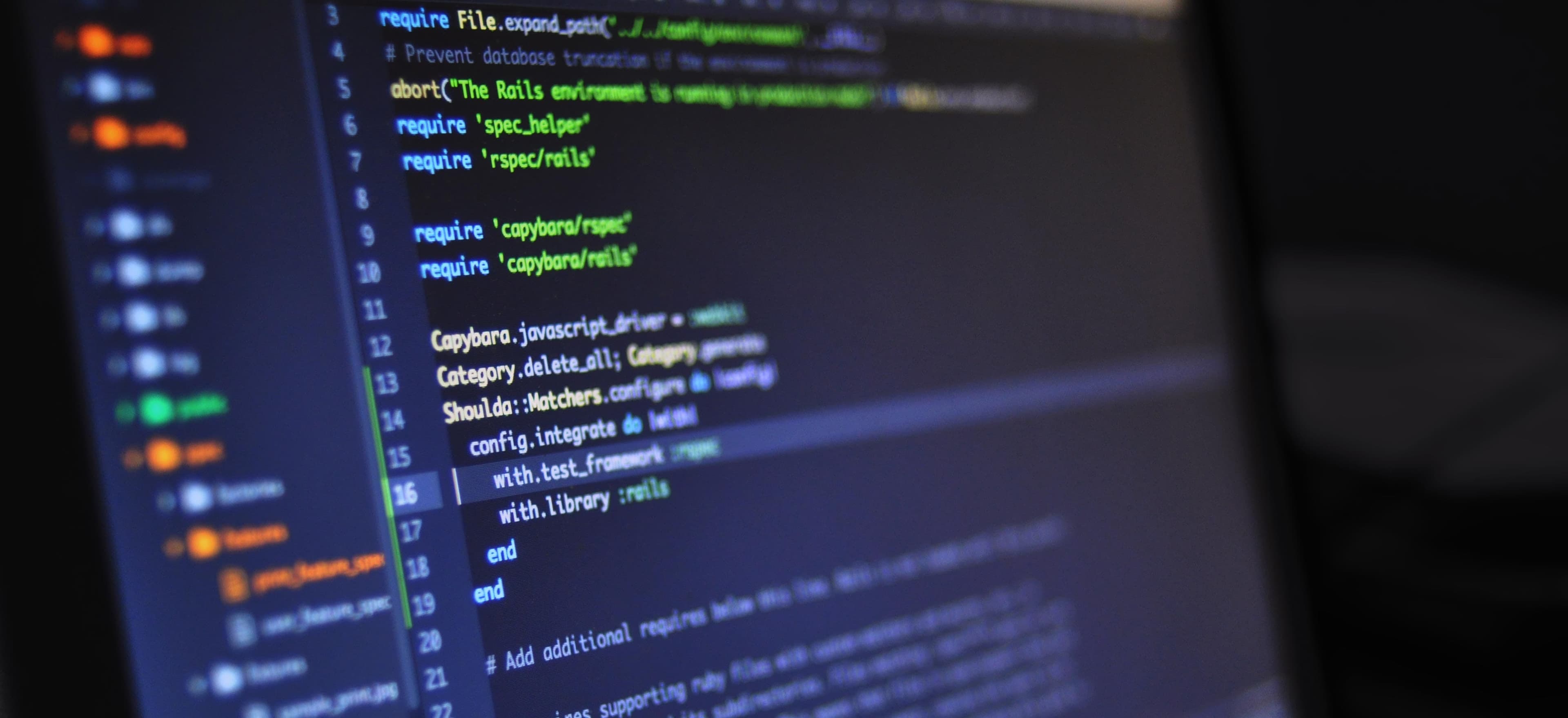
- Published on
Common Pitfalls When Implementing the Template Method Pattern
The Template Method Pattern is a behavioral design pattern that defines the skeleton of an algorithm in a method, deferring some steps to subclasses. This allows subclasses to redefine specific steps without changing the algorithm's structure. While it's a powerful pattern, developers often encounter pitfalls during its implementation. This blog post outlines the common mistakes made when using the Template Method Pattern and effective strategies to avoid them.
Understanding the Template Method Pattern
Before diving into the pitfalls, it’s essential to understand what the Template Method Pattern is and how it can be beneficial.
Basic Structure
The Template Method Pattern typically consists of:
- An Abstract Class: This class defines the template method, which lays out the steps of the algorithm.
- Concrete Classes: These subclasses implement the specific steps of the algorithm.
Here's a simple example to illustrate:
abstract class AbstractCalculator {
// Template method
public final void calculate() {
readInput();
processInput();
displayResult();
}
abstract void readInput(); // Steps to be overridden
abstract void processInput(); // Steps to be overridden
void displayResult() { // Common behavior
System.out.println("Displaying results...");
}
}
class AdditionCalculator extends AbstractCalculator {
@Override
void readInput() {
System.out.println("Reading input for addition.");
}
@Override
void processInput() {
System.out.println("Processing addition of inputs.");
}
}
In this example, AbstractCalculator
defines the calculate()
method, which uses the readInput()
, processInput()
, and displayResult()
methods. The AdditionCalculator
class provides specific implementations for reading and processing input.
Common Pitfalls in the Template Method Pattern
1. Over-complicating the Template Method
One of the most significant pitfalls is making the template method too complex. It's essential to ensure that the template method retains clarity and purpose. Overly complicated methods can lead to difficulties in maintenance and understanding.
Solution
Keep the template method as simple as possible. For instance, if certain steps can be abstracted into their methods or classes, do so. This ensures that each step is clear and easy to manage.
2. Tight Coupling of Classes
Another common issue arises when subclasses become tightly coupled with the abstract class. This situation makes it difficult to reuse the subclasses in different contexts.
Solution
Use interfaces to reduce coupling. For example, instead of relying solely on the abstract class, introduce interfaces to define the operations, allowing various implementations without the need for changes in the template method.
3. Violation of the Open/Closed Principle
The Open/Closed Principle states that classes should be open for extension but closed for modification. If you often find yourself modifying the abstract class to add new behavior, you are likely violating this principle.
Solution
Design the structure in such a way that new algorithms or behavior can be added through new subclasses rather than modifying existing code. This encourages scalability and organization.
4. Lack of Clarity in Template Methods
If the template method lacks documentation or clear definitions, it can lead to ambiguities about how to implement child classes, culminating in incorrect implementations.
Solution
Always document your template methods. Comments in the code can clarify what each step is expected to do and how it fits within the overall algorithm.
abstract class DataProcessor {
// Template method
public final void process() {
readData(); // Read data from different sources
validateData(); // Validate that the data is correct
transformData(); // Transform data into the desired format
outputData(); // Output data to desired destination
}
abstract void readData();
abstract void validateData();
abstract void transformData();
void outputData() {
System.out.println("Outputting processed data...");
}
}
In this example, a well-documented template method provides clarity on what tasks each step should handle.
5. Overriding the Template Method
While subclasses can override specific steps in the algorithm, overriding the entire template method can be problematic. Developers might inadvertently introduce bugs or change critical behaviors.
Solution
Avoid overriding the template method in subclasses. Instead, focus on overriding only the necessary steps. This ensures consistent behavior while allowing for flexibility in implementation.
6. Failing to Provide Default Implementations
When steps in the template method are left completely abstract, it can lead to code duplication across subclasses. This scenario especially occurs when the steps have common behaviors.
Solution
Provide default implementations for common steps in the abstract class. This minimizes boilerplate code and enhances maintainability.
abstract class ReportGenerator {
public final void generateReport() {
fetchData();
formatData();
saveReport();
}
void formatData() { // Common implementation
System.out.println("Formatting the report data.");
}
abstract void fetchData(); // To be implemented by subclasses
abstract void saveReport(); // To be implemented by subclasses
}
7. Overusing the Pattern
Not every situation requires the Template Method Pattern. Overusing design patterns can lead to unnecessary complexity.
Solution
Evaluate whether the Template Method Pattern truly suits your specific scenario. If the behavior you want to define is simpler or doesn’t require the use of the pattern, consider other approaches.
The Bottom Line
The Template Method Pattern is a powerful tool in a software developer's arsenal, granting flexibility and scalability to your code. However, it comes with its pitfalls. By staying aware of these challenges—overcomplication, tight coupling, violation of principles, lack of clarity, unnecessary overriding, failing to provide defaults, and overuse—you can effectively leverage this design pattern for a cleaner, more maintainable codebase.
For a deeper dive into design patterns, I recommend reading Head First Design Patterns or reviewing the Java Design Patterns documentation. These resources provide valuable insights and practical examples to help you become proficient in your design endeavors.
Make sure to share your thoughts or experiences regarding the Template Method Pattern in the comments below, and happy coding!
Checkout our other articles