Overcoming Legacy Code Challenges with Effective Stubs
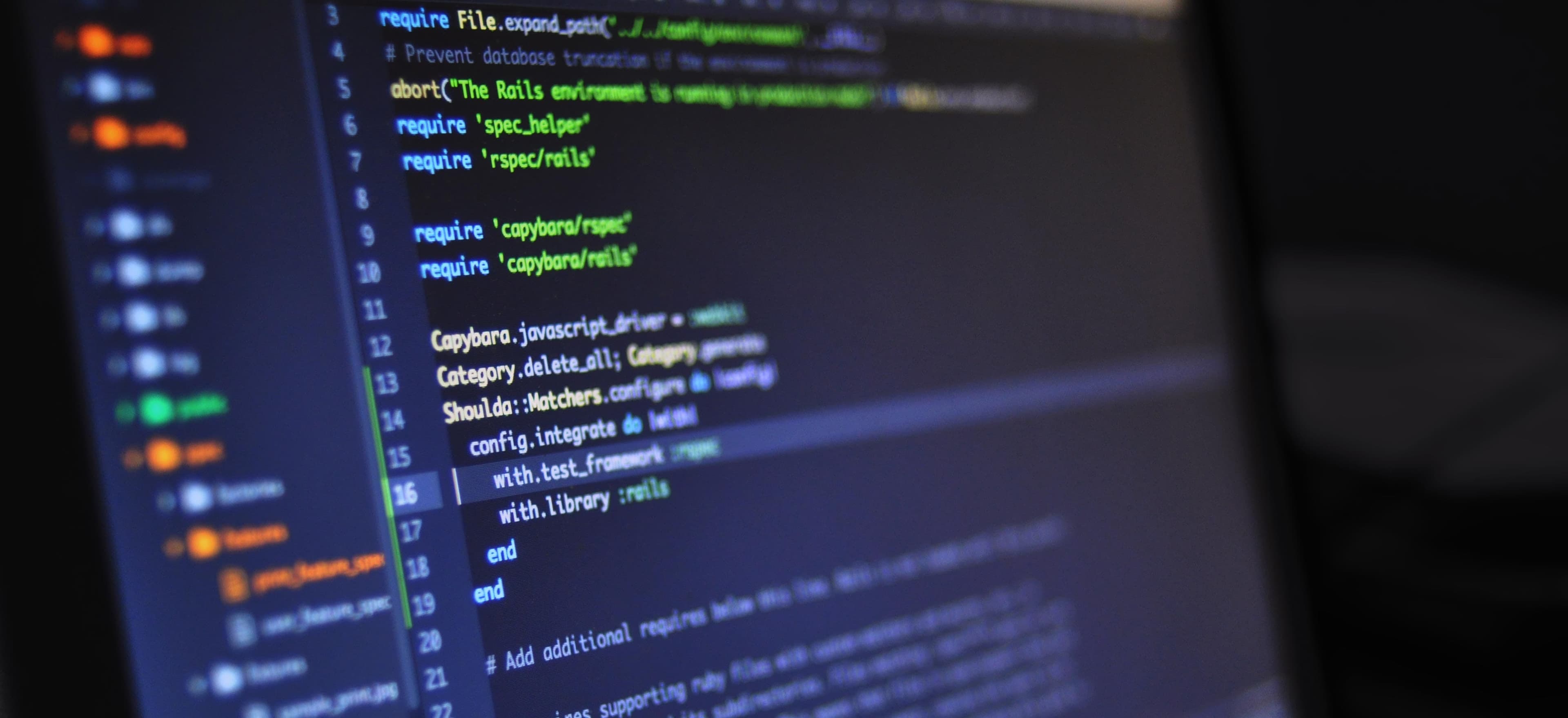
- Published on
Overcoming Legacy Code Challenges with Effective Stubs
In the world of software development, legacy code often has a bad reputation. The term "legacy code" typically refers to code that is difficult to maintain, understand, or modify. Legacy code can cause bottlenecks in development cycles, hinder innovation, and lead to an increase in bugs. However, there is hope. This blog post will discuss how effective stubs can help developers overcome the challenges posed by legacy code, making it more manageable and enabling smoother transitions during updates.
What are Stubs?
Stubs are a form of test doubles used in software testing to simulate the behavior of components that a system interacts with but aren’t available or are too complex to use during testing. This allows developers to isolate a portion of their code and test it without relying on external systems or parts of the application.
Why Use Stubs?
Using stubs serves several purposes:
- Isolation: Stubs allow you to isolate the unit of testing from its dependencies.
- Simplicity: They provide a simplified interaction model for components that otherwise might be too complex to deal with directly.
- Efficient: With stubs, you can write tests faster as you aren't reliant on external services that could introduce latency or downtime.
- Controlled Environment: Stubs provide a controlled environment to test specific functionalities and edge cases.
Understanding these benefits is key to leveraging stubs effectively, especially when grappling with legacy code.
The Legacy Code Landscape
Before diving deeper into stubs, it is essential to grasp the landscape of legacy code. According to Michael Feathers, author of Working Effectively with Legacy Code, "Legacy code is simply code without tests." This definition emphasizes that without proper testing, the risks of modifying legacy code increase significantly.
Common Challenges with Legacy Code
- Spaghetti Code: Legacy systems often contain tangled code that becomes challenging to navigate.
- Lack of Documentation: Inadequate documentation leads to misunderstandings and incorrect implementations.
- Dependencies on Deprecated Libraries: Many legacy systems are built on outdated libraries that have not been maintained.
- Difficulty in Testing: Testing legacy code can become complicated due to tightly coupled components and the absence of a proper testing framework.
Using Stubs to Tackle Legacy Code Challenges
Here, we focus on how we can use stubs to tackle the challenges of legacy code.
1. Isolating Dependencies
When you have legacy code that's tightly coupled with other systems, it can become impossible to test. By using stubs, you can replace those dependencies with simplified implementations that mimic their behavior. This allows you to focus on the unit you want to test.
Example: Isolating Database Calls
Consider a scenario where you have a method that retrieves user information from a database:
public class UserService {
private Database database;
public UserService(Database database) {
this.database = database;
}
public User getUserById(int userId) {
return database.query("SELECT * FROM users WHERE id = ?", userId);
}
}
In this example, the Database
class is an external dependency. Not only does it complicate unit testing, but it also ties the code to a specific database implementation.
Now, let's create a stub for the Database
class:
public class DatabaseStub extends Database {
@Override
public User query(String sql, int userId) {
// Return a canned response, mimicking a database query
return new User(userId, "John Doe", "john.doe@example.com");
}
}
Now, during testing, you can use the DatabaseStub
:
public class UserServiceTest {
@Test
public void testGetUserById() {
DatabaseStub databaseStub = new DatabaseStub();
UserService userService = new UserService(databaseStub);
User user = userService.getUserById(1);
assertNotNull(user);
assertEquals("John Doe", user.getName());
}
}
Commentary
- Why Use a Stub Here? The stub allows you to sidestep the complexities associated with a real database connection. It simplifies the testing setup while still allowing you to validate the behavior of your
UserService
class. - Test Results: The focus is squarely on the
UserService
functionality, leading to faster and more reliable tests.
2. Handling External Systems
In many cases, legacy code interacts with external services (like REST APIs or message queues). Testing these integrations can be tricky due to their unpredictability.
Example: Stubbing an External API Call
Assuming you have a method that calls an external weather API:
public class WeatherService {
private ExternalApi api;
public WeatherService(ExternalApi api) {
this.api = api;
}
public String getWeather(String location) {
return api.fetchWeather(location);
}
}
You can create a stub for ExternalApi
:
public class ApiStub extends ExternalApi {
@Override
public String fetchWeather(String location) {
// Return a fixed weather report
return "Sunny, 25°C";
}
}
When writing your tests:
public class WeatherServiceTest {
@Test
public void testGetWeather() {
ApiStub apiStub = new ApiStub();
WeatherService weatherService = new WeatherService(apiStub);
String weather = weatherService.getWeather("New York");
assertEquals("Sunny, 25°C", weather);
}
}
Commentary
- Why Use a Stub Here? This approach avoids the need to rely on an unstable external service, thus maintaining the predictability of your tests.
- Resilience: By using stubs, you enhance the resilience of your testing suite.
3. Gradual Refactoring
Legacy code can be refactored incrementally using stubs. Start by identifying key areas of legacy code that are ripe for change, and introduce stubs to help facilitate the refactoring process.
Example: Refactoring an Old Reporting Class
Imagine a legacy class that combines reporting and data-fetching:
public class ReportGenerator {
public String generateReport() {
// Directly interacts with various services
ExternalApi api = new ExternalApi();
DataBase db = new DataBase();
// Complex logic lines here...
return "Report Data";
}
}
To refactor, you could extract the data-fetching logic into separate methods or classes and use stubs in the report tests. By refactoring method by method, you can progressively build a stable base of tests around your legacy code until it's finally modernized.
My Closing Thoughts on the Matter
Legacy code isn't inherently a barrier to progress. By incorporating effective stubs into your testing strategy, you can systematically address many issues associated with legacy systems. Isolating dependencies, controlling external systems, and enabling gradual refactoring with stubs are all practical techniques that lead to a more maintainable codebase.
Finally, as the development landscape evolves, consider reading further on best practices in test-driven development via resources like Robert C. Martin's Clean Code. You might also explore more strategies, such as mocking and faking, for a deeper understanding of how you can navigate your legacy code challenges successfully.
By embracing these techniques, you can not only tame the mess that is legacy code but also invigorate your development process, allowing innovation to flourish.
Happy coding!
For further reading on stubs, mock objects, and effective unit testing, consider checking out resources like Mockito Documentation and The Art of Unit Testing by Roy Osherove.