Migrating Spring Applications: Common Pitfalls to Avoid
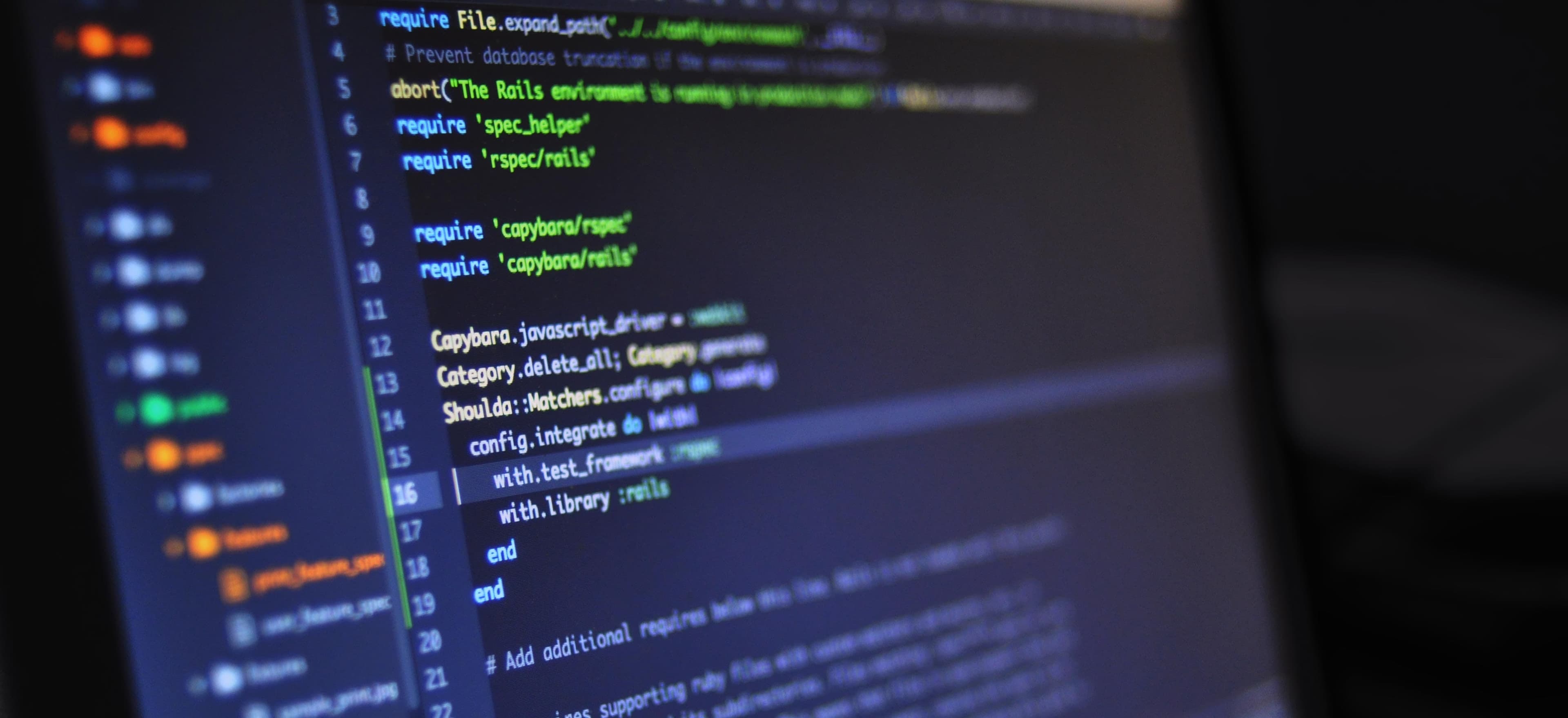
- Published on
Migrating Spring Applications: Common Pitfalls to Avoid
Migrating a Spring application is a daunting but sometimes necessary task. Whether you are upgrading to a new version, moving to a different server, or transitioning to a cloud environment, there are several potential pitfalls that can hinder your progress. This post will explore some of these obstacles, providing guidance on best practices to ensure a smooth migration.
Understanding the Migration Process
Before diving into the common pitfalls, it is essential to outline the migration process itself. The migration of a Spring application generally follows these steps:
- Assessment of Current Application: Analyzing existing code, dependencies, and configurations.
- Planning the Migration: Determining goals, timelines, and resource allocation.
- Migration Execution: Performing the actual migration and testing.
- Post-Migration Audit: Ensuring that the application runs smoothly in its new environment.
By understanding this process, you can be better prepared to avoid the common pitfalls that arise.
Common Pitfalls to Avoid
1. Skipping the Assessment Phase
One of the biggest mistakes developers make is skipping the assessment phase. This leads to underestimating the complexity of the migration.
Why it Matters: If you are unaware of your application's dependencies, configurations, or even its architecture, you may face unexpected issues during migration.
Tip: Conduct a thorough assessment by creating an inventory of the libraries and dependencies used in your current application. Tools like Spring Initializr can assist in setting up new dependencies more efficiently.
Code Snippet: Dependency Analysis
Here is a sample code snippet that can help identify dependencies from a pom.xml
file:
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<!-- Add more dependencies as needed -->
</dependencies>
Commentary: Always list your dependencies clearly. Recognizing whether you are using outdated or deprecated libraries is crucial before initiating the migration.
2. Ignoring Compatibility Issues
Compatibility between different versions of Spring or plugins can lead to breaking changes. New features may roll in, while some prior functionalities could be deprecated.
Why it Matters: Ignoring these issues may cause runtime errors, which can derail your project timeline.
Tip: Review the Spring Framework Release Notes to understand what changes have been made between versions.
Code Snippet: Spring Boot Starter
If you are upgrading to a newer version, ensure your spring-boot-starter
is appropriate. An example of the change would be:
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-dependencies</artifactId>
<version>2.5.4</version> <!-- Check for the latest stable version -->
<relativePath/> <!-- lookup parent from repository -->
</parent>
Commentary: This allows you to maintain common versioning rather than upgrading dependencies individually, helping minimize compatibility issues.
3. Failing to Update Configuration Files
Configuration files play a critical role in the Spring ecosystem. Often, configuration properties may change or require updates based on new features or migrations.
Why it Matters: Outdated configurations can lead to unexpected behaviors that are troublesome to debug.
Tip: Review your application.properties
or application.yml
files carefully.
Code Snippet: Application Properties
Here’s how you might update a database connection string for a new environment:
# Previous
spring.datasource.url=jdbc:mysql://localhost:3306/mydb
# New
spring.datasource.url=jdbc:mysql://production-db-server:3306/mydb?useSSL=true
Commentary: The connection strings, user credentials, and other properties should reflect the new environment norms ensuring that your application interacts correctly with external systems.
4. Not Considering Database Schema Changes
Often, during the migration process, databases may get updated or changed along with the application. Not adapting your application to these changes can cause major headaches.
Why it Matters: If your code references old schema tables or field names, your application will face runtime exceptions and data integrity issues.
Tip: Use database migration tools like Flyway or Liquibase. They help keep your database migrations versioned and seamless.
Code Snippet: Flyway Example
A simple Flyway migration script might look like this:
-- V1__Create_person_table.sql
CREATE TABLE person (
id INT PRIMARY KEY,
name VARCHAR(100),
email VARCHAR(100)
);
Commentary: This structured approach ensures that you can make incremental changes to your schemas while keeping migrations tracked over time.
5. Overlooking Testing
Testing is the bedrock of any successful migration. By neglecting adequate testing, you are laying a foundation for problems down the road.
Why it Matters: It is the best way to ensure that all functionalities of the application perform as expected in the new environment.
Tip: Write both unit tests and integration tests. Ensure testing covers typical scenarios, as well as edge cases.
Code Snippet: JUnit Test Sample
Using JUnit to test your application logic could be structured like so:
@SpringBootTest
class PersonControllerTests {
@Autowired
private MockMvc mockMvc;
@Test
void testGetPerson() throws Exception {
this.mockMvc.perform(get("/api/person/1"))
.andExpect(status().isOk())
.andExpect(jsonPath("$.name").value("John Doe"));
}
}
Commentary: Regular unit tests should be automated and run against your application after deployment to ensure there are no regressions.
6. Not Communicating with the Team
Communication is key, yet often overlooked. Failing to involve all stakeholders during the migration plan can lead to discrepancies in expectations.
Why it Matters: Miscommunication can result in assumptions that affect the entire project scope.
Tip: Establish regular meetings, shared documentation, and a clear migration checklist accessible to all team members.
My Closing Thoughts on the Matter
Migrating a Spring application brings along a wealth of opportunities as well as challenges. By understanding and anticipating common pitfalls like skipping the assessment phase, ignoring compatibility issues, failing to update configuration files, overlooking schema changes, neglecting testing, and lacking communication, you can significantly improve your chances for a successful migration.
Being thorough and taking each of the steps seriously will lay down a robust and efficient application suited for the demands of modern deployment scenarios.
Always remember: a smooth migration lays the groundwork for a more maintainable, scalable, and efficient application.
For more detailed guidance on building with Spring applications, consider checking out the official Spring Documentation and Migrating Spring Boot Applications documentation.