Overcoming Common Pitfalls in Wizard Design Patterns
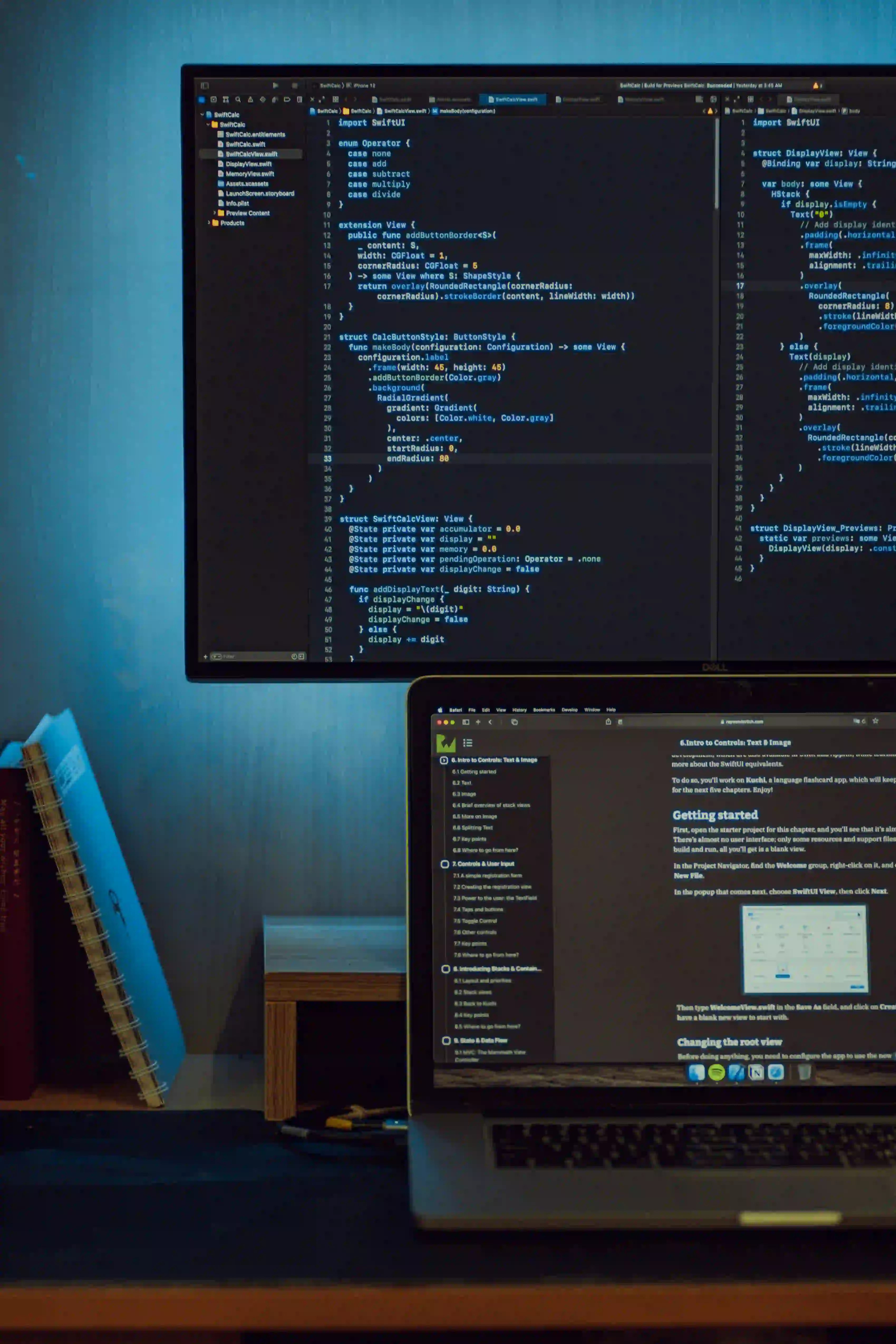
Overcoming Common Pitfalls in Wizard Design Patterns
Design patterns are established best practices that provide generalized solutions to common problems in software design. One of these patterns is the Wizard Design Pattern, which helps organize complex user interactions in a step-by-step manner. In this blog post, we will explore the Wizard Design Pattern, its common pitfalls, and ways to overcome them with practical Java examples.
What is a Wizard Design Pattern?
The Wizard Design Pattern is a user-centered design approach that breaks down complex tasks into simple, manageable steps. By guiding users through a series of logical steps, this pattern improves usability, especially in applications involving complicated processes such as forms, configurations, or setups.
Benefits of Using the Wizard Design Pattern
- Enhanced User Experience: Users can focus on one task at a time.
- Reduced Cognitive Load: Breaking down tasks minimizes the overwhelming feeling users may experience.
- Better Data Integrity: By validating inputs at each step, we can ensure high-quality data collection.
Key Pitfalls in Wizard Design Patterns
Despite the advantages of Wizard Design Patterns, developers often encounter pitfalls that can negatively affect the user experience. Below are common pitfalls and strategies to overcome them.
1. Poor Step Design
The Issue:
One of the most common pitfalls is poorly defined steps. If steps are ambiguous or not sequentially logical, users can quickly become frustrated.
Solution:
Ensure that each step has a clear purpose and logically leads to the next. This means making buttons, labels, and actions intuitive. Define clear objectives for each step.
public class RegistrationWizard {
private Step currentStep;
public void nextStep() {
if(currentStep == Step.USERINFO) {
currentStep = Step.EMAILVERIFICATION;
} else if(currentStep == Step.EMAILVERIFICATION) {
currentStep = Step.PAYMENT;
}
// Update UI accordingly
}
}
In this example, methodically defining the sequence of steps ensures that users easily understand what to expect next.
2. Lack of Feedback
The Issue:
Users often need feedback about their actions. When feedback is absent, users may feel lost.
Solution:
Provide immediate feedback on user actions. Visual cues, confirmation messages, or progress bars can significantly enhance the experience.
public class WizardStep {
public void validateData(UserInput input) {
if (input.isValid()) {
System.out.println("Input is valid.");
// Proceed to next step
} else {
System.out.println("Input is invalid. Please check and try again.");
// Highlight the error
}
}
}
Utilizing console messages as feedback ensures users are aware of their current validation state, allowing them to adjust inputs accordingly.
3. Missing Navigation Controls
The Issue:
Some wizards completely lock users in a linear path. This can create frustration, especially if users want to go back and review previous steps.
Solution:
Always provide navigation controls like "Back" and "Next." This lets users roam freely, which is essential for a good user experience.
public class NavigationController {
private final NavigationWizard wizard;
public NavigationController(NavigationWizard wizard) {
this.wizard = wizard;
}
public void back() {
if (!wizard.isFirstStep()) {
wizard.previousStep();
}
}
public void next() {
if (!wizard.isLastStep()) {
wizard.nextStep();
}
}
}
Adding a navigation controller that checks first and last steps can improve user comfort and control over their progress.
4. Ineffective Error Handling
The Issue:
Error messages can quickly become a headache, especially if they are cryptic or overloaded with technical jargon.
Solution:
Design clear, user-friendly error messages that offer actionable steps for resolution.
public class ErrorHandler {
public void displayError(String errorCode) {
switch(errorCode) {
case "EMPTY_FIELD":
System.out.println("Please fill out all required fields.");
break;
case "INVALID_EMAIL":
System.out.println("Please enter a valid email address.");
break;
default:
System.out.println("An unexpected error occurred. Please try again.");
}
}
}
Clear and actionable messages prevent user frustration. By guiding them to resolve issues, you enhance usability.
5. Over-complicating the Wizard
The Issue:
In an effort to be comprehensive, developers may include too many steps or information, leading to a confusing process.
Solution:
Streamline all steps. Include only necessary information and tasks that need to be completed.
public class SimpleWizard {
public void displayStep(Step step) {
switch(step) {
case USERINFO:
System.out.println("Please enter your user information.");
break;
case EMAILVERIFICATION:
System.out.println("Check your email for a verification link.");
break;
case PAYMENT:
System.out.println("Enter payment details.");
break;
}
}
}
By focusing on essential steps, you uphold clarity and user engagement.
Final Considerations
The Wizard Design Pattern, when implemented correctly, can greatly enhance user experience and engagement in complex applications. However, as outlined, there are common pitfalls that can detract from its effectiveness.
By adhering to clear step design, providing meaningful feedback, ensuring effective navigation, delivering user-friendly error messages, and streamlining the wizard interface, developers can create effective applications that guide users seamlessly through intricate tasks.
For further reading on design patterns, check out the Gang of Four Design Patterns for broader context and examples.
In what ways have you encountered or addressed pitfalls while implementing the Wizard Design Pattern? Join the discussion below!