Enhancing Spring Data Solr with Custom Repository Methods
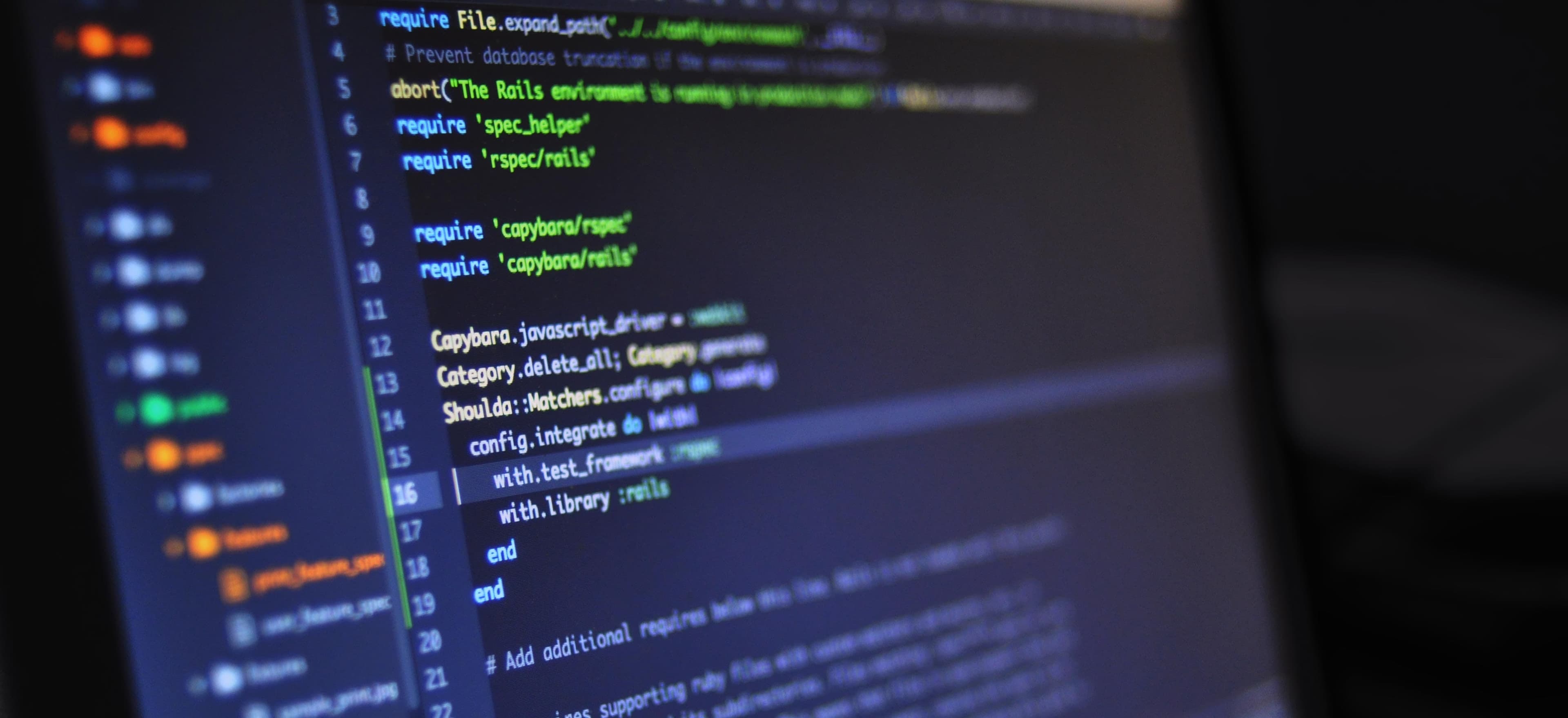
- Published on
Enhancing Spring Data Solr with Custom Repository Methods
Spring Data Solr is a powerful tool designed to streamline the use of Apache Solr in Java applications. It simplifies the process of managing Solr repositories, providing automatic implementations for common tasks. However, developers often encounter unique requirements that necessitate custom methods. This blog post discusses how to enhance Spring Data Solr with custom repository methods, providing practical examples and code snippets along the way.
What is Spring Data Solr?
Before diving into the customization process, let's briefly revisit what Spring Data Solr is. Spring Data Solr offers a Spring-based framework for interacting with Solr. It allows developers to think in terms of repositories rather than manual query construction, promoting cleaner, more maintainable code.
For more information on Spring Data Solr's capabilities, refer to the official documentation.
Setting Up Your Project
To start using Spring Data Solr, first ensure your Java Spring application is set up correctly. In your pom.xml
, include the required Spring Data Solr dependencies:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-solr</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
This setup ensures you have everything you need to start developing with Solr and Spring Data.
Creating a Solr Document
Defining a Solr document is your first step in building a repository. A Solr document is basically a representation of your data that you want to index in Solr.
Here’s an example of a simple Solr document representing a Book
:
import org.springframework.data.annotation.Id;
import org.springframework.data.solr.core.mapping.SolrDocument;
@SolrDocument(solrCoreName = "books")
public class Book {
@Id
private String id;
private String title;
private String author;
private double price;
// Constructors, getters, and setters omitted for brevity
public Book(String id, String title, String author, double price) {
this.id = id;
this.title = title;
this.author = author;
this.price = price;
}
}
In this example, the @SolrDocument
annotation denotes that this class can be mapped to a Solr core named "books". The @Id
annotation is essential to specify the unique identifier for each document in Solr.
Setting Up Your Repository
Next, create a repository interface for your Book
document. Spring Data Solr auto-implements basic methods based on the method names you declare. Here is how it looks:
import org.springframework.data.solr.repository.SolrCrudRepository;
public interface BookRepository extends SolrCrudRepository<Book, String> {
// Custom method signatures can go here
}
Why Use SolrCrudRepository?
Using SolrCrudRepository
offers out-of-the-box capabilities like save, delete, and find, making it easier to manage basic CRUD operations. However, to meet your application's specific requirements, you might need custom methods that extend the capabilities of this basic repository.
Adding Custom Methods
To add custom behavior, you'll need to create a custom implementation for your repository. Let's implement a custom method to find books by their author.
First, we declare the method in the BookRepository
interface:
import java.util.List;
public interface BookRepository extends SolrCrudRepository<Book, String> {
List<Book> findByAuthor(String author);
}
Next, create a custom implementation:
import org.apache.solr.client.solrj.SolrClient;
import org.apache.solr.client.solrj.SolrQuery;
import org.apache.solr.client.solrj.response.QueryResponse;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.solr.core.SolrOperations;
import java.util.List;
public class BookRepositoryImpl implements BookRepositoryCustom {
@Autowired
private SolrOperations solrOperations;
@SuppressWarnings("unchecked")
@Override
public List<Book> findByAuthor(String author) {
SolrQuery query = new SolrQuery();
query.setQuery("author:" + author);
// More complex queries can include filters and boosting
QueryResponse response = solrOperations.getSolrClient().query(query);
return (List<Book>) response.getBeans(Book.class);
}
}
Explanation of the Custom Method
-
SolrQuery: You create a new SolrQuery instance to construct your query. The
setQuery
method effectively searches the index for documents matching the criteria. -
QueryResponse: The results are accessed through the
QueryResponse
object. ThegetBeans(Book.class)
method converts the results into a list ofBook
objects.
By separating custom queries into an implementation class, you maintain a clean codebase and follow the Single Responsibility Principle.
Configuring Your Custom Repository
To let Spring know about your custom repository, annotate your main application class with the following:
@EnableSolrRepositories(basePackages = "com.example.repository")
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
With the @EnableSolrRepositories
, you tell Spring to scan for repositories and their implementations.
Testing Your Custom Method
To ensure your custom logic works as expected, consider writing a test case. Here's an example using JUnit:
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import java.util.List;
import static org.assertj.core.api.Assertions.assertThat;
@SpringBootTest
public class BookRepositoryTests {
@Autowired
private BookRepository bookRepository;
@Test
public void testFindByAuthor() {
// Assuming books with author 'John Doe' are indexed
List<Book> books = bookRepository.findByAuthor("John Doe");
assertThat(books).isNotEmpty();
assertThat(books.get(0).getAuthor()).isEqualTo("John Doe");
}
}
Why Testing Matters
Testing your custom methods not only verifies that your implementation works correctly but also enables future maintenance and refactoring with confidence. Catching bugs early in the development lifecycle saves time and reduces technical debt.
The Bottom Line
By extending Spring Data Solr with custom repository methods, you can tailor your application to meet its unique demands, all while leveraging the power of Solr. The combination of automatic CRUD functionality and custom queries offers limitless possibilities for your data access strategy.
For more information on Spring Data Solr, visit the Spring Data Solr Reference Documentation. As you continue to explore, think critically about when to use custom logic versus built-in capabilities, maintaining a balance that best supports your development objectives.
If you found this post helpful, please share it with your colleagues or on social media! Happy coding!
Checkout our other articles