Mastering Java's Reflection API: Avoiding Common Pitfalls
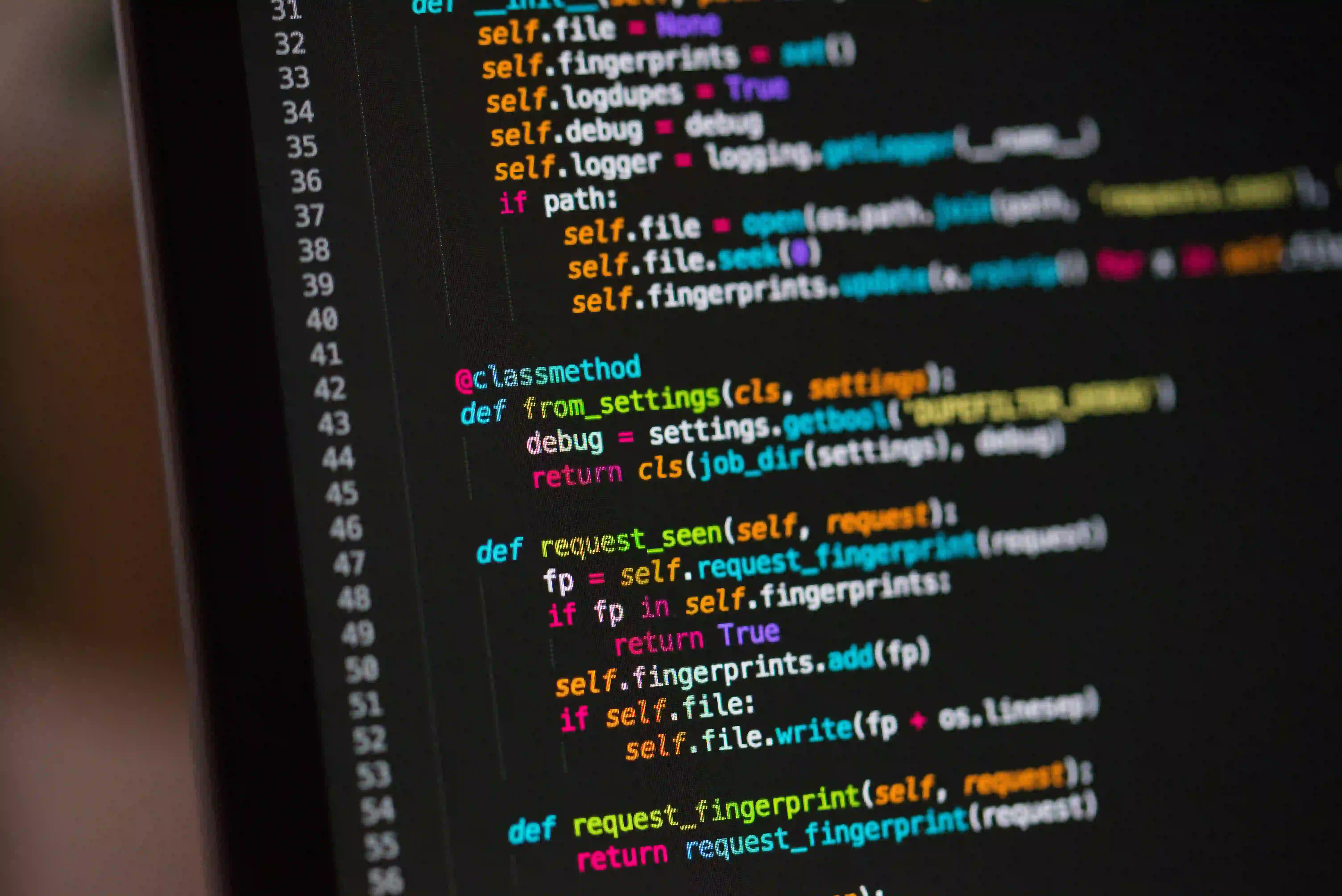
Mastering Java's Reflection API: Avoiding Common Pitfalls
Java's Reflection API is a powerful feature that allows developers to inspect classes, methods, and fields at runtime, enabling dynamic behavior and advanced programming techniques. While this capability opens up exciting possibilities, it also comes with its share of complexities and potential pitfalls. In this post, we will explore the Reflection API, its compelling use cases, and the common mistakes to avoid when utilizing it.
What is the Reflection API?
Reflection in Java is a part of the java.lang.reflect
package. It provides a way to dynamically access classes, methods, interfaces, and fields, allowing developers to manipulate them at runtime. The benefits of Reflection include:
- Introspection: Examine a class's properties and behaviors.
- Dynamic Method Invocation: Call methods without knowing their names at compile time.
- Dynamic Class Loading: Load classes at runtime using their names.
Example of Reflection Usage
Here’s a simple example that demonstrates how to use Java’s Reflection API to inspect a class:
import java.lang.reflect.Method;
public class ReflectionExample {
public static void main(String[] args) {
// Get the class object for String
Class<?> stringClass = String.class;
// Get all declared methods of the String class
Method[] methods = stringClass.getDeclaredMethods();
// Iterate through and print method names
for (Method method : methods) {
System.out.println("Method Name: " + method.getName());
}
}
}
In this example, the String
class is inspected to list out all its methods. This is particularly useful when working with libraries that may not be fully known at compile time.
Common Pitfalls in Using Reflection
While Reflection is a powerful feature, it can also lead to several issues if not used carefully. Here, we will discuss some common pitfalls and how to avoid them.
1. Performance Overhead
Pitfall: Using Reflection is generally slower than direct method calls due to its dynamic nature. The extra overhead comes from the need to inspect classes and access resources at runtime.
Solution: If performance is critical, prefer direct method calls unless you have valid reasons to use Reflection.
Example of Performance Concern
Consider this poor performance example using Reflection:
import java.lang.reflect.Method;
public class PerformanceExample {
public static void main(String[] args) throws Exception {
String string = "Hello, World!";
Method method = String.class.getMethod("charAt", int.class);
for (int i = 0; i < 1_000_000; i++) {
method.invoke(string, 5);
}
}
}
In this snippet, invoking the charAt
method via Reflection is executed a million times. Such usage could significantly degrade performance compared to a direct method call:
public class DirectCallPerformanceExample {
public static void main(String[] args) {
String string = "Hello, World!";
for (int i = 0; i < 1_000_000; i++) {
string.charAt(5);
}
}
}
2. Security Restrictions
Pitfall: Reflection can be restricted by security settings. For example, certain operations may throw IllegalAccessException
if there are security managers in place.
Solution: Understand the security context in which your application will run. If using Reflection in sensitive environments, ensure your code complies with security policies.
Security Example
import java.lang.reflect.Field;
public class SecurityExample {
public static void main(String[] args) {
try {
Class<?> clazz = Class.forName("java.lang.String");
Field field = clazz.getDeclaredField("value");
field.setAccessible(true); // May throw SecurityException
// Additional code...
} catch (Exception e) {
e.printStackTrace();
}
}
}
In this example, attempting to set a private field’s accessibility might lead to security-related exceptions. Proper handling and understanding security policies is crucial in such cases.
3. Unchecked Exceptions
Pitfall: Reflection operations often throw runtime exceptions rather than checked exceptions, making it easier to overlook potential issues leading to NullPointerException
or ClassCastException
.
Solution: Always validate the objects being manipulated and catch the relevant exceptions.
Exception Handling Example
import java.lang.reflect.Method;
public class ExceptionHandlingExample {
public static void main(String[] args) {
try {
Class<?> clazz = Class.forName("NonExistentClass");
Method method = clazz.getMethod("someMethod");
method.invoke(null);
} catch (ClassNotFoundException e) {
System.err.println("Class not found: " + e.getMessage());
} catch (NoSuchMethodException e) {
System.err.println("Method not found: " + e.getMessage());
} catch (Exception e) {
e.printStackTrace();
}
}
}
In this snippet, robust exception handling is employed to catch various exceptions that could arise from Reflection operations.
4. Lack of Type Safety
Pitfall: Reflection bypasses compile-time type checking, leading to potential runtime class cast issues. This can make code more error-prone and harder to debug.
Solution: Ensure you verify types at runtime and use generics where applicable.
Type Safety Example
import java.lang.reflect.Method;
public class TypeSafetyExample {
public static void main(String[] args) {
try {
Class<?> clazz = Class.forName("java.lang.String");
Method method = clazz.getDeclaredMethod("substring", int.class);
String result = (String) method.invoke("Hello, World!", 7); // Safe call
System.out.println(result);
} catch (Exception e) {
e.printStackTrace();
}
}
}
In this example, a substring method is safely invoked through Reflection. Still, ensuring that the casting is legitimate is paramount to avoid ClassCastException
.
When to Use Reflection
Having navigated through common pitfalls, you may wonder when is it appropriate to leverage the Reflection API. Here are a few use cases:
- Testing Frameworks: Tools like JUnit use Reflection to inspect test classes.
- Dependency Injection: Many frameworks (e.g., Spring) utilize Reflection for bean creation and dependency management.
- Serialization Libraries: Libraries like Gson use Reflection to inspect objects at runtime for serialization.
Final Considerations
Mastering Java's Reflection API can significantly enhance your programming capabilities, but it requires a thorough understanding of its implications. Keep in mind the performance overhead, security restrictions, unchecked exceptions, and lack of type safety as you implement this powerful feature. By remaining vigilant and informed, you can harness the full potential of Reflection while avoiding common pitfalls.
For more information on Java Reflection, you can check out the official Java documentation or explore a more advanced approach in projects like Hibernate, which relies on Reflection for ORM capabilities. Always remember, with great power comes great responsibility. Happy coding!