Solving Image Download Issues in Android Volley
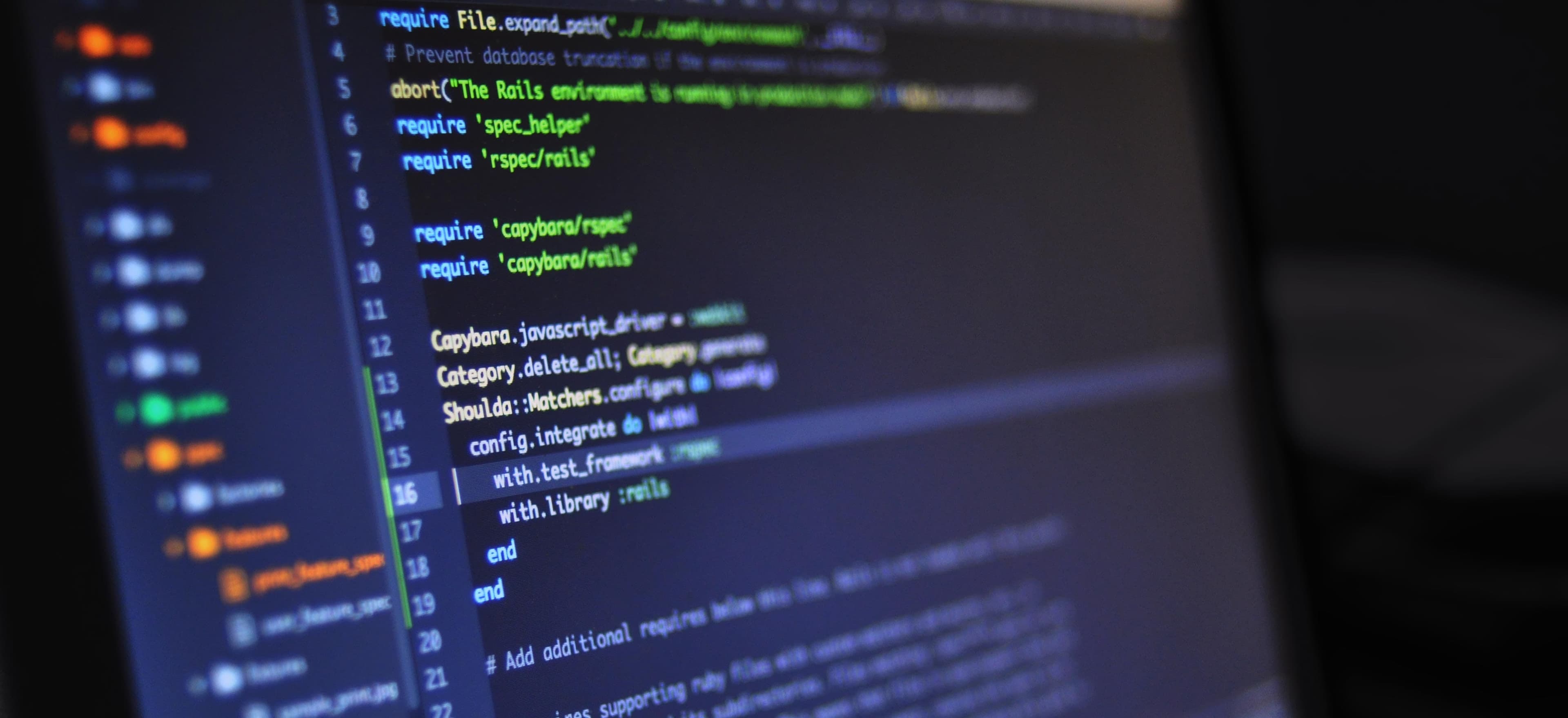
- Published on
Solving Image Download Issues in Android Volley
When developing Android applications, handling images efficiently is paramount for a seamless user experience. Android Volley, a library developed by Google, simplifies networking tasks, including image downloading. However, developers often face challenges when dealing with image downloading issues. This blog post will delve into common image download issues in Android Volley and how to fix them effectively.
What is Android Volley?
Android Volley is a powerful HTTP library that makes networking for Android apps faster and easier. By providing an easy-to-use API and features like efficient request handling, caching, and image loading, Volley enhances the development experience. Specifically, it is designed to handle network requests on the main application thread and overcome some of the basic challenges present in network calls.
Key Features of Android Volley:
- Asynchronous Requests: Volley provides a straightforward way to send network requests off the main thread to keep the UI responsive.
- Caching: It provides built-in caches for responses, reducing loading times and improving app performance.
- Image Loading: Volley allows efficient image downloading and caching to minimize network overhead.
Setting Up Android Volley
Before diving into resolving image download issues, let’s ensure you have Volley correctly integrated into your Android project. Here’s how to set it up:
- Add Volley Dependency:
In your app-level build.gradle
file, add the following dependency:
dependencies {
implementation 'com.android.volley:volley:1.2.1'
}
- RequestQueue: You need a
RequestQueue
to handle the requests.
import com.android.volley.RequestQueue;
import com.android.volley.toolbox.Volley;
// Within your Activity
RequestQueue requestQueue = Volley.newRequestQueue(this);
Now with the RequestQueue
in place, let’s explore some common image downloading issues and their solutions.
Common Image Downloading Issues
- Image Not Displaying
- Slow Image Loading
- Memory Leaks
- Not Cached Properly
Issue 1: Image Not Displaying
One of the most frustrating issues is when an image URL doesn't display an image in the UI. Here’s a common scenario and how to address this.
Solution: Validate Image URL
Ensure your image URLs are correct and accessible. An incorrect URL or a broken link will result in a failure to fetch the image.
String imageUrl = "https://example.com/image.jpg"; // Double-check your URL
ImageRequest imageRequest = new ImageRequest(imageUrl,
new Response.Listener<Bitmap>() {
@Override
public void onResponse(Bitmap response) {
// Set the image to an ImageView
imageView.setImageBitmap(response);
}
}, 0, 0, ImageView.ScaleType.CENTER_CROP, Bitmap.Config.ARGB_8888,
new Response.ErrorListener() {
@Override
public void onErrorResponse(VolleyError error) {
// Handle error
Log.e("Image Request", "Error loading image");
}
});
requestQueue.add(imageRequest);
This ImageRequest
handles the image downloading process and sets it to an ImageView
. The onErrorResponse
callback helps manage failed loads, guiding you to potential causes.
Issue 2: Slow Image Loading
Solution: Caching Strategy
One method to improve the speed of image loading is to implement caching effectively. Volley provides built-in response caching, which can reduce your load times. Make sure to configure your cache when initializing the RequestQueue
.
int cacheSize = 20 * 1024 * 1024; // 20MB
DiskCache cache = new DiskBasedCache(getCacheDir(), cacheSize);
ImageLoader imageLoader = new ImageLoader(requestQueue, new LruBitmapCache(cacheSize));
// Load the image
imageLoader.get(imageUrl, new ImageLoader.ImageListener() {
@Override
public void onResponse(ImageLoader.ImageContainer response, boolean isImmediate) {
imageView.setImageBitmap(response.getBitmap());
}
@Override
public void onErrorResponse(VolleyError error) {
Log.e("ImageLoader", "Error loading image");
}
});
With this implementation, images are cached on disk, minimizing redundant downloads and enhancing loading speed.
Issue 3: Memory Leaks
Another critical issue to watch out for is memory leaks, which can occur if large objects are not disposed of correctly.
Solution: Use Weak References
To mitigate memory leaks when dealing with contexts, it's wise to use WeakReference
. Here’s how you can do this:
public class ImageLoader {
private WeakReference<Context> contextWeakReference;
public ImageLoader(Context context) {
contextWeakReference = new WeakReference<>(context);
}
public void loadImage(String url) {
Context context = contextWeakReference.get();
if (context != null) {
// Proceed with loading the image
}
}
}
Using WeakReference
prevents unnecessary holding of a strong reference to the context, thereby allowing the system to reclaim memory when necessary.
Issue 4: Not Cached Properly
Sometimes images may not behave as expected due to cache settings.
Solution: Inspect Cache Control Headers
Verify that your server sets proper cache headers. If not configured correctly, clients can mismanage cached images.
For example, you can force Volley to cache images properly and avoid unnecessary requests:
imageRequest.setRetryPolicy(new DefaultRetryPolicy(
DefaultRetryPolicy.DEFAULT_TIMEOUT_MS,
DefaultRetryPolicy.DEFAULT_MAX_RETRIES,
DefaultRetryPolicy.DEFAULT_BACKOFF_MULT));
By adjusting cache headers and retry policies, you can ensure that images are efficiently cached, speeding up load times.
Closing the Chapter
Image downloading issues in Android Volley can hamper user experience. By addressing common issues—such as images not displaying, slow loading times, memory leaks, and caching problems—you can significantly enhance your application’s performance and usability.
Don’t forget to validate your image URLs and implement proper caching strategies to optimize your app further. Now is a great time to dive deeper into Android Volley! For a more in-depth look at caching strategies, consider checking out Android Developers: Networking. Also, refer to Understanding Cache in Volley for further reading on implementing effective caching techniques.
By following the solutions outlined in this post, you should be well-equipped to handle image downloading issues in Android Volley like a pro!
Checkout our other articles