Troubleshooting Issues with JavaFX Nest Thermostat Control
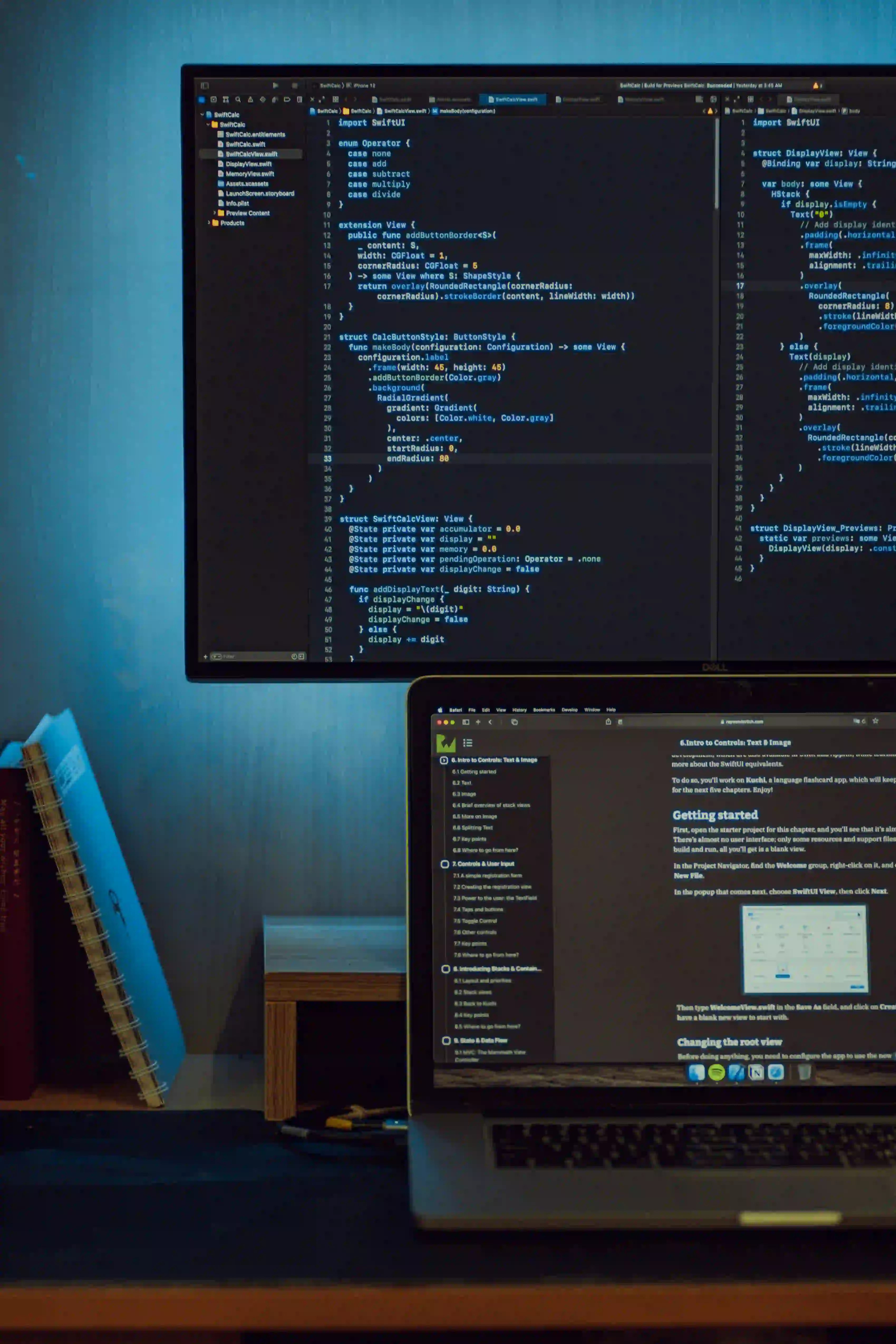
Troubleshooting Issues with JavaFX Nest Thermostat Control
JavaFX is a powerful framework for building rich client applications in Java, and when coupled with IoT devices like the Nest Thermostat, it allows developers to create intuitive and user-friendly interfaces. However, integrating JavaFX with Nest Thermostat can sometimes introduce challenges. In this blog post, we will explore common issues that may arise and how to troubleshoot them effectively.
Overview of JavaFX and Nest Thermostat Integration
Before we dive into troubleshooting, let’s briefly discuss how JavaFX integrates with the Nest Thermostat. The Nest Thermostat is an Internet of Things (IoT) device that allows users to control their home heating and cooling systems remotely. Through the Nest API, developers can manage settings, retrieve data, and receive updates in real-time.
JavaFX can serve as a frontend interface, allowing you to create an application that interacts with the Nest API. This application can read the thermostat’s current temperature, set new temperature preferences, and much more.
Common Issues and Solutions
1. Authentication Failures
One of the most common issues developers face when trying to connect to the Nest API is authentication failure. Proper authentication is vital for accessing the API.
Solution
Ensure that you have correctly set up the developer account and generated the appropriate OAuth tokens. Double-check the client ID and client secret. Here’s a simple code snippet that shows how to authenticate using OAuth2.
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.Response;
public class NestAPI {
private static final String ACCESS_TOKEN = "YOUR_ACCESS_TOKEN";
public Response getThermostatData() throws Exception {
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder()
.url("https://developer-api.nest.com/thermostats")
.addHeader("Authorization", "Bearer " + ACCESS_TOKEN)
.build();
return client.newCall(request).execute();
}
}
In the snippet above, replace YOUR_ACCESS_TOKEN
with your actual access token. The code uses the OkHttp library to handle HTTP requests and responses. This method helps you check if you are successfully authenticated.
2. Connection Issues
Sometimes, your application may be unable to reach the Nest API due to connectivity issues. This can be due to network problems, incorrect API URLs, or firewall restrictions.
Solution
Perform a simple check to ensure that you can reach the API endpoint from your Java application. Here’s a straightforward way to verify connectivity.
public boolean isApiReachable(String url) {
try {
new URL(url).openConnection().connect();
return true;
} catch (IOException e) {
e.printStackTrace();
return false;
}
}
// Usage
if (!isApiReachable("https://developer-api.nest.com")) {
System.out.println("API is not reachable. Please check your connection.");
}
3. JSON Parsing Errors
When interacting with the Nest API, you receive JSON responses. If your code fails to parse JSON, it typically results in runtime exceptions. This can happen if the JSON structure changes or if there are any unexpected values.
Solution
Use a robust library for parsing JSON, like Jackson or Gson, which can handle a variety of error scenarios. Below is an example of how to use Jackson to parse the JSON response:
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
public class JsonParser {
public void parseThermostatData(String jsonData) {
ObjectMapper objectMapper = new ObjectMapper();
try {
JsonNode rootNode = objectMapper.readTree(jsonData);
JsonNode thermostats = rootNode.path("thermostats");
// Process thermostats data
} catch (Exception e) {
e.printStackTrace(); // Log the error for troubleshooting
}
}
}
In the example above, make sure to handle exceptions correctly, as they will provide insights if the JSON structure does not match expectations.
4. UI Responsiveness
JavaFX applications must maintain UI responsiveness, especially when making network calls. A common pitfall is blocking the JavaFX application thread with synchronous calls.
Solution
Utilize JavaFX’s Task
or Service
to offload heavy processing to a separate thread. Here’s a sample implementation using Task
:
import javafx.concurrent.Task;
public void loadThermostatData() {
Task<Void> task = new Task<Void>() {
@Override
protected Void call() throws Exception {
// Fetch data from Nest API
Response response = getThermostatData();
if (response.isSuccessful()) {
parseThermostatData(response.body().string());
}
return null;
}
};
task.setOnSucceeded(e -> {
// Update UI with thermostat data
});
task.setOnFailed(e -> {
// Handle failure
Throwable error = task.getException();
error.printStackTrace(); // Log the error
});
new Thread(task).start();
}
5. UI Elements Not Updating
After fetching data, you might find that your UI does not update to reflect new thermostat settings. This usually occurs due to thread safety issues in JavaFX.
Solution
Always update UI components on the JavaFX Application Thread using Platform.runLater()
. Below is an example:
Platform.runLater(() -> {
thermostatLabel.setText("Current Temperature: " + currentTemperature);
});
My Closing Thoughts on the Matter
Integrating JavaFX with the Nest Thermostat can bring a lot of power and functionality to your applications, but it doesn't come without challenges. By understanding and troubleshooting common issues like authentication failures, connection problems, JSON parsing errors, and ensuring UI responsiveness, you can create a robust and user-friendly application.
For additional information and resources, consider visiting the following links:
Happy coding, and may your JavaFX applications thrive!