Overcoming Docker Networking Challenges in CI/CD Pipelines
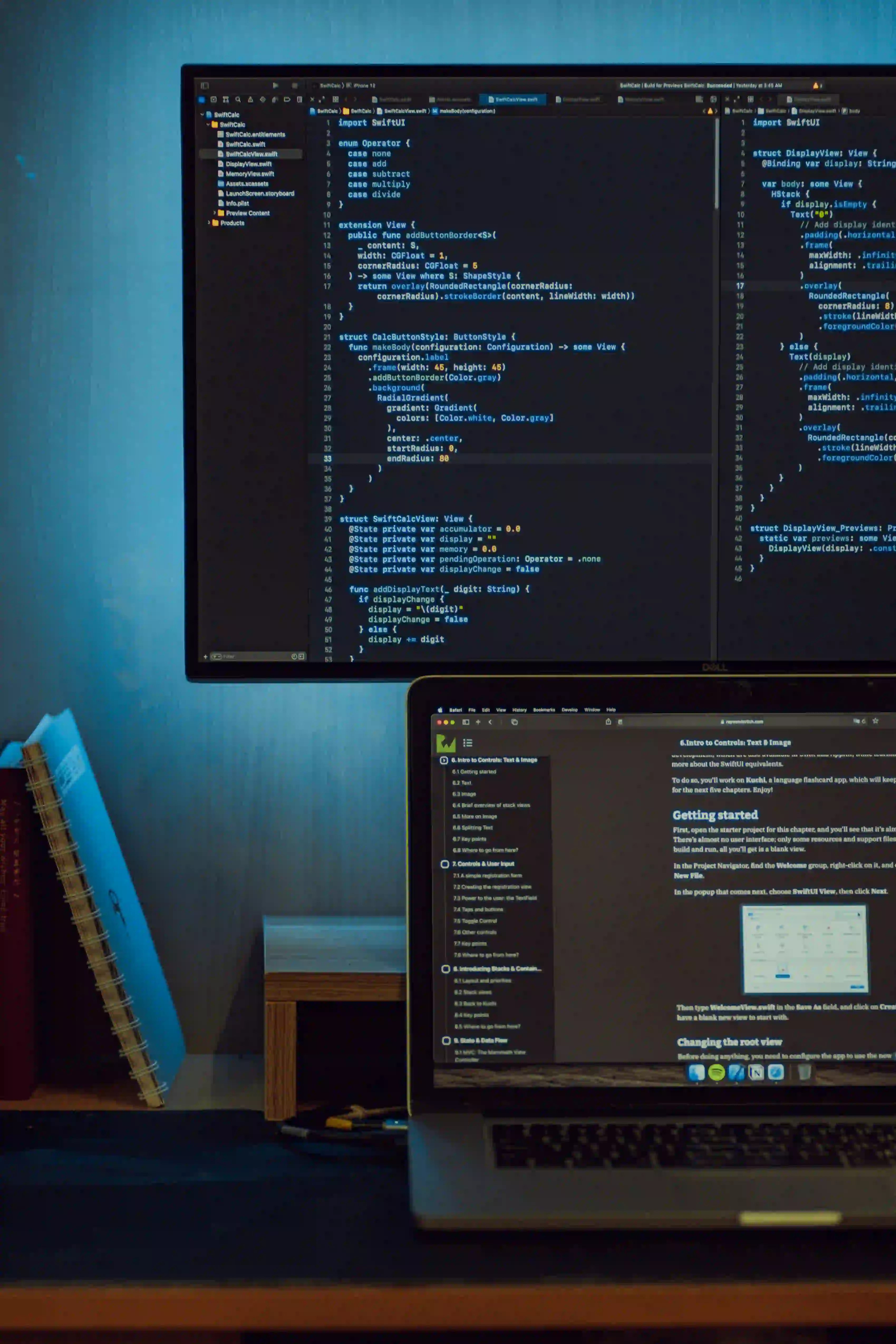
Overcoming Docker Networking Challenges in CI/CD Pipelines
In today's software development landscape, Continuous Integration (CI) and Continuous Deployment (CD) have become indispensable practices. Among the myriad tools that facilitate these methodologies, Docker stands out for its containerization capabilities. While Docker enhances flexibility and scalability, it also introduces networking complexities that can prove challenging in CI/CD pipelines. This blog post delves into these common networking challenges and presents effective strategies to overcome them.
The Role of Docker in CI/CD
Docker simplifies the deployment process by encapsulating applications along with their dependencies into isolated containers. This ensures that the application behaves consistently across different environments. However, in the context of CI/CD, especially when connecting multiple containerized services, networking becomes a pivotal issue.
Common Docker Networking Challenges
-
Service Discovery: In a microservices architecture, containers must communicate with each other. How do they find each other amid a constantly changing environment?
-
Port Conflicts: Multiple containers might attempt to bind to the same host port, leading to conflicts that terminate your pipeline prematurely.
-
Data Persistence: Networking setups can lead to ephemeral data loss if not handled correctly, resulting in unreliable application behavior during testing.
-
Debugging Network Issues: When something goes wrong with container communication, it can be challenging to pinpoint the root cause.
-
Environment Configuration: Different environments (development, staging, production) require precise configurations to work appropriately.
Strategies for Overcoming Networking Challenges
Implementing a Service Mesh
A service mesh provides a dedicated infrastructure layer for managing service-to-service communication. Tools like Istio or Linkerd can create a more consistent networking experience by handling service discovery, load balancing, and reliability.
# Example of Istio sidecar injection using kubectl
kubectl label namespace default istio-injection=enabled
Why use a service mesh? With automatic service discovery, you can easily manage container communications without manually configuring each container, enhancing reliability and efficiency in your CI/CD pipeline.
Using Docker Compose for Local Development
Docker Compose simplifies multi-container Docker applications. By defining your services in a docker-compose.yml
file, you can specify how they should interact over a network.
version: '3'
services:
web:
image: nginx
ports:
- "8080:80"
db:
image: postgres
With Docker Compose, service discovery is automatic. Each service gets a hostname that matches its name, allowing for seamless communication without hardcoding IP addresses.
Implementing Health Checks
Health checks are essential for ensuring that your services are up and running before other services attempt to communicate with them. Docker allows you to define health checks in your Dockerfile.
FROM nginx:latest
HEALTHCHECK CMD curl --fail http://localhost/ || exit 1
Why are health checks important? They ensure that your CI/CD process does not proceed to subsequent steps until your services are ready, preventing failure in integration tests.
Optimizing Network Mode
Docker provides various network modes that can be beneficial depending on your requirements:
- Bridge: This is the default mode and works well for most cases where you only need local communication.
- Host: This mode shares the host's network stack. It can be useful for performance but introduces complexity in security.
- Overlay: This is used for multi-host networking, ideal for microservices deployed across a cluster.
# Create an overlay network for a Docker swarm
docker network create --driver overlay my_overlay_network
Choosing the right network mode can significantly impact how services interact and can aid in seamless communication in complex CI/CD workflows.
Handling Port Conflicts in CI/CD
When running multiple tests concurrently, ensuring unique port allocations is crucial. You can specify dynamic ports or allow Docker to auto-allocate them, avoiding hardcoded values.
# Example in docker-compose
web:
image: nginx
ports:
- "80" # Just use a random port
Why address port conflicts? This simple step minimizes downtime in your CI/CD process, making simultaneous test runs more feasible.
Data Persistence through Volumes
Docker containers are ephemeral; therefore, using volumes ensures that data persists beyond the container lifecycle. This is particularly important in testing environments.
# Create a named volume
docker volume create my_db_data
docker run -d -v my_db_data:/var/lib/postgresql/data postgres
Volumes handle data persistence by keeping your data intact even when containers are recreated, allowing for reliable testing and deployments.
Debugging Network Issues
When debugging network issues, consider these techniques:
- Log Network Traffic: Monitor incoming and outgoing traffic to spot anomalies.
- Container Networking Tools: Use tools like
curl
,ping
, andnetstat
within your containers to troubleshoot connectivity. - Docker's Built-in Networking Commands: Utilize commands such as
docker network inspect
to view detailed information about networks and connected containers.
Why prioritize debugging? Proactively tackling networking issues can save valuable time and resources, ensuring your pipeline runs smoothly.
Final Thoughts
Docker has revolutionized the way we approach CI/CD pipelines. Yet, the complexities of networking can slow down progress if not handled correctly. By implementing a service mesh, using Docker Compose, incorporating health checks, optimizing network modes, handling port conflicts, ensuring data persistence, and employing effective debugging techniques, you can streamline your CI/CD process.
To learn more about advanced Docker networking options and best practices, check out the official Docker documentation and explore Istio's guide to service mesh.
By overcoming these challenges, you set the stage for a robust and resilient CI/CD pipeline that accelerates development and delivers quality software consistently. Happy Dockerizing!