How Escape Analysis Impacts JIT Compiler Performance
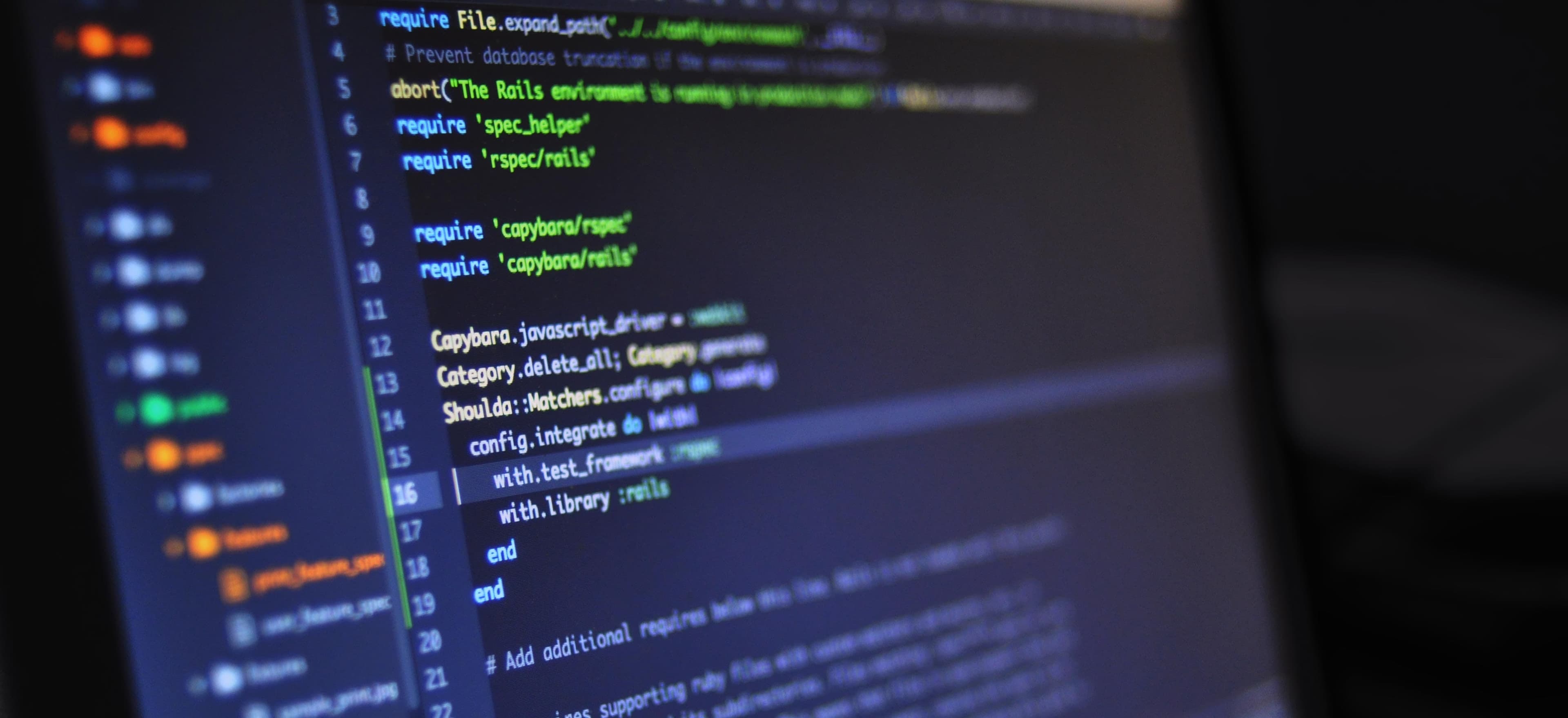
- Published on
How Escape Analysis Impacts JIT Compiler Performance
In the world of Java programming, memory management and performance optimization are critical topics. With Java being a more managed environment, developers often rely on the Just-In-Time (JIT) compiler to harness the power of the Java Virtual Machine (JVM). One of the intriguing aspects of the JIT compiler is its use of Escape Analysis, a technique that can significantly impact performance.
In this article, we will delve into the concept of Escape Analysis, explore its impact on JIT compiler performance, and provide code snippets to illustrate key points clearly and effectively.
What is Escape Analysis?
Escape Analysis is a technique that studies the scope or reach of objects in a program. Specifically, it determines whether an object allocated in a method can be accessed from outside that method. If an object can be confined to a single method or thread, it is said to be non-escaping.
Types of Escape
-
Stack Escape: The object can be allocated on the stack rather than the heap. This is beneficial because allocation and deallocation on the stack are faster due to the absence of garbage collection overhead.
-
Thread Escape: The object can be escaped to other threads, which means it will require heap allocation, leading to further overhead and complexity in memory management.
This leads to an intriguing question: how does the JIT compiler leverage Escape Analysis to enhance performance?
Benefits of Escape Analysis
1. Optimized Memory Management
When an object does not escape a method, the JIT compiler can allocate it on the stack instead of the heap. The speed of stack allocation is one key strength of Escape Analysis.
public void process() {
MyObject obj = new MyObject();
// The compiler can optimize memory allocation here
// since obj does not escape this method.
// Perform operations with obj
}
In the example above, if MyObject
does not escape the process
method, during JIT compilation, it can be allocated on the stack.
2. Reduced Garbage Collection Pressure
Heap allocations increase garbage collection (GC) frequency. By confining objects to stack allocation, Escape Analysis reduces unnecessary GC, improving overall application performance.
3. Enabling Inlining
Methods that involve objects that do not escape can be inlined. Inlining enhances performance by eliminating the overhead of method calls, leading to more efficient execution paths.
public class Example {
// This method can be inlined if 'localObject' does not escape
public void inlineExample() {
LocalObject localObject = new LocalObject();
localObject.performAction();
}
}
The Role of the JIT Compiler
Compilation Phases
The JIT compiler operates in several phases, notably:
-
Profiling: It observes the program's execution to identify hot paths, i.e., methods or branches that are frequently executed.
-
Optimization: It applies various optimizations at runtime based on profiling information.
When Escape Analysis is performed, the JIT compiler can decide the most efficient way to allocate memory for objects.
Adaptive Optimization
The JIT compiler employs adaptive optimization, meaning it can change the optimizations as the program runs. If variables that were once determined to escape become non-escaping later in execution, the JIT compiler can adjust its strategy accordingly.
Link between Escape Analysis and Other Optimization Techniques
Escape Analysis doesn't operate in isolation. It interacts with other JIT optimizations (e.g., loop unrolling, method inlining) to enhance performance comprehensively.
For an in-depth exploration of JIT compiler optimization techniques, Java Performance Tuning provides a great resource.
Example: Impact of Escape Analysis
Let's consider a scenario where Escape Analysis can significantly change performance outcomes.
Without Escape Analysis
public class DataProcessor {
public List<Data> processData() {
List<Data> dataList = new ArrayList<>();
for (int i = 0; i < 1000; i++) {
Data data = new Data(i);
dataList.add(data);
}
return dataList; // data objects escape
}
}
In this example, the Data
objects created within processData
escape to the caller, causing them to reside on the heap, which may lead to frequent garbage collection for 1000 instances.
With Escape Analysis
Now consider if we have a modified method in a scenario where the processing does not require escaping:
public class DataProcessor {
public void process() {
for (int i = 0; i < 1000; i++) {
TempData tempData = new TempData(i); // Assume TempData does not escape
tempData.performProcessing(); // Perform some operations
}
}
}
Here, if TempData
are non-escaping objects, the JIT compiler might decide to allocate them on the stack, resulting in reduced overhead and better performance.
Considering Multithreading
Multithreading introduces a level of complexity to Escape Analysis. Objects that will be shared across threads are inherently escaping; the JIT compiler must act accordingly.
public class SharedResource {
private int count;
public synchronized void increment() {
count++;
}
}
In this case, because the SharedResource
class is meant to be accessed from multiple threads, it introduces thread escape, and the compiler can’t optimize this through Escape Analysis.
Wrapping Up
Escape Analysis is a powerful technique that enhances the performance of Java applications by optimizing memory allocation and reducing garbage collection. The JIT compiler utilizes Escape Analysis during its lifecycle phases, allowing adaptive optimization based on object behavior at runtime.
By understanding Escape Analysis, Java developers can write more efficient code and utilize the JVM's potential fully. The benefits of reduced GC pressure, stack allocation, and effective method inlining can lead to noticeable improvements in application performance.
Further Reading
For those looking to delve deeper into JIT compilation and the performance of Java applications, consider checking out the following resources:
- Java Concurrency in Practice to understand how Java handles concurrency and optimization.
- Effective Java for practical advice on Java programming best practices, including performance considerations.
If you have any experiences relating to Escape Analysis or want to share performance optimization tips, feel free to join the discussion in the comments below!
Checkout our other articles