Conquering Lost Updates: A Beginner's Guide to Locking
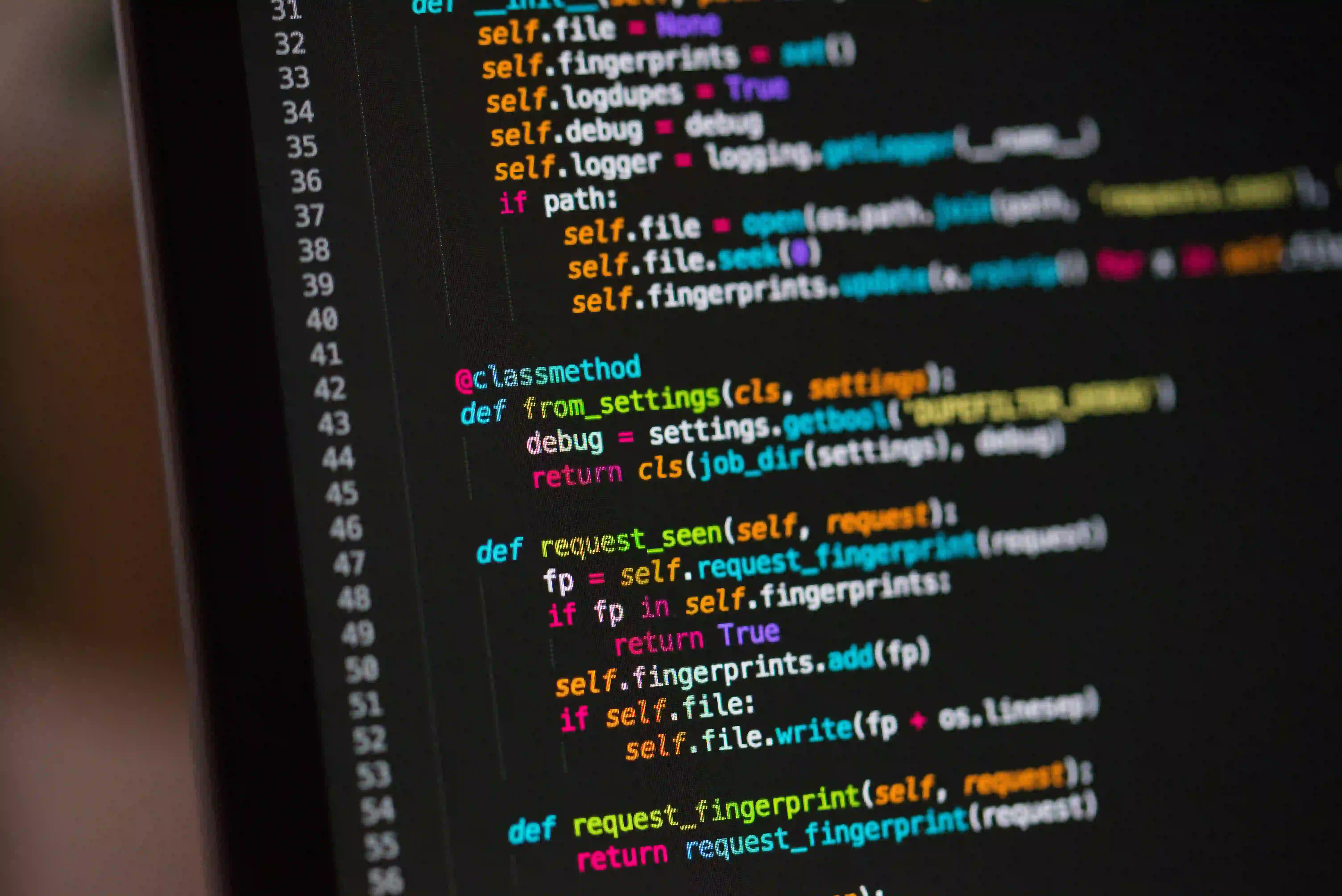
Conquering Lost Updates: A Beginner's Guide to Locking in Java
In the world of concurrent programming, one of the most daunting challenges developers face is the risk of "lost updates." When multiple threads access and modify shared data simultaneously, the likelihood of encountering conflicts increases. This blog aims to shed light on lost updates and how locking mechanisms in Java can help mitigate this issue, ensuring data integrity.
Table of Contents
- Understanding Lost Updates
- The Basics of Locking
- Java's Built-in Locking Mechanisms
- Synchronized Methods
- Synchronized Blocks
- Reentrant Locks
- Best Practices for Locking
- Conclusion
Understanding Lost Updates
A "lost update" occurs when two or more threads read the same data and then write their changes back without being aware of each other's modifications. Imagine a scenario with a shared resource, like an online bank account. If two users are transferring money from the same account simultaneously, without proper synchronization, one transfer may overwrite the other, leading to incorrect balances.
Example Scenario
Consider an online banking application where Alice and Bob attempt to withdraw money from the same account:
- The current balance is $100.
- Alice checks the balance and sees $100.
- Bob also checks the balance and sees $100.
- Alice withdraws $50 and updates the balance to $50.
- Bob withdraws $30 and updates the balance to $70, inadvertently losing Alice's update.
In this case, the account ends up with $70 instead of the correct $50.
The Basics of Locking
Locking is a technique used to control access to shared resources. When a thread locks a resource, all other threads attempting to access that resource must wait until the lock is released. This prevents lost updates, ensuring that one thread's changes do not overwrite another's.
Types of Locks
Locking solutions usually fall into two categories:
- Exclusive Locks: Only one thread can hold the lock at a time.
- Shared Locks: Multiple threads can hold the lock simultaneously for read operations.
Example of Exclusive Locking in Java
Let's look at a simple code example to demonstrate locking in Java using synchronized methods.
public class BankAccount {
private int balance = 100;
public synchronized void deposit(int amount) {
int newBalance = balance + amount;
balance = newBalance;
}
public synchronized void withdraw(int amount) {
int newBalance = balance - amount;
balance = newBalance;
}
public synchronized int getBalance() {
return balance;
}
}
Commentary
By marking the methods with the synchronized
keyword, we ensure that only one thread can execute these methods at a time. This way, the balance cannot be changed by one thread while another is calculating it, effectively preventing lost updates.
Java's Built-in Locking Mechanisms
Java provides multiple built-in mechanisms for handling locks and synchronizations. Here are some of the most common methods for locking:
Synchronized Methods
Synchronized methods lock the entire method, preventing concurrent access. However, this can lead to performance bottlenecks if the method takes a long time to execute.
Synchronized Blocks
Sometimes, you may only need to lock a specific block of code. Synchronized blocks can help minimize the scope of the lock, improving performance.
public void withdraw(int amount) {
synchronized(this) {
int newBalance = balance - amount;
balance = newBalance;
}
}
Commentary
In this code, the synchronized block limits the locked section to just the operations on the balance, allowing other non-critical code to run without waiting for the lock.
Reentrant Locks
Java provides a more flexible locking mechanism through the ReentrantLock
class in the java.util.concurrent.locks
package. Reentrant locks allow for more intricate locking strategies, including the ability to interrupt threads waiting for the lock.
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
public class BankAccount {
private int balance = 100;
private final Lock lock = new ReentrantLock();
public void deposit(int amount) {
lock.lock();
try {
int newBalance = balance + amount;
balance = newBalance;
} finally {
lock.unlock();
}
}
public void withdraw(int amount) {
lock.lock();
try {
int newBalance = balance - amount;
balance = newBalance;
} finally {
lock.unlock();
}
}
public int getBalance() {
return balance;
}
}
Commentary
The lock.lock()
method acquires the lock before performing the operations, while lock.unlock()
in a finally
block ensures that the lock is released even if an exception occurs. This prevents deadlocks where no thread can proceed because the lock isn't released.
Best Practices for Locking
- Minimize Lock Scope: Keep the locked area's code as brief as possible to reduce the risk of contention and deadlocks.
- Use Timeouts with Locks: Utilize lock timeouts to avoid waiting indefinitely if a lock cannot be acquired.
- Avoid Nested Locks: Attempting to lock more than one resource can lead to deadlocks. If nested locks are unavoidable, always acquire them in a specific order.
- Consider Read/Write Locks: Use
ReadWriteLock
if your application has far more read operations than write operations, allowing multiple threads to read while restricting write access.
Wrapping Up
Lost updates pose a significant threat to the integrity of applications involving concurrent programming. However, Java provides powerful synchronous mechanisms that can help you manage concurrency more effectively. By understanding and implementing these techniques, you can safeguard against lost updates while ensuring that your application remains responsive.
For a more profound understanding of concurrent programming in Java, consider exploring the Java Concurrency Tutorial offered by Oracle.
As you venture into Java programming, always remember that concurrency can be a double-edged sword. With great power comes great responsibility; mastering the art of locking is essential in building robust and efficient applications. Happy coding!