Mastering Bidirectional Associations: Common Pitfalls Explained
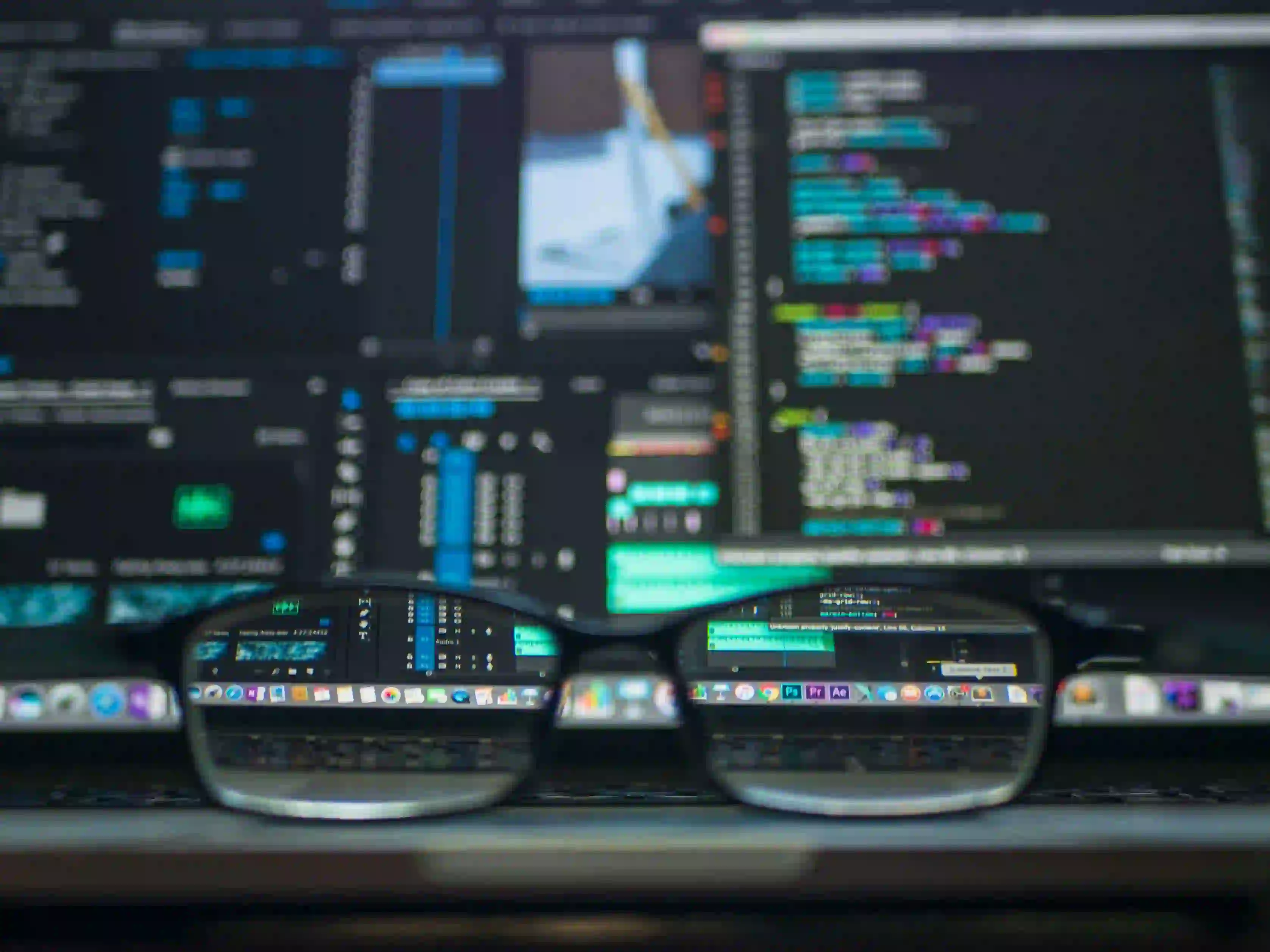
Mastering Bidirectional Associations in Java: Common Pitfalls Explained
In object-oriented programming, particularly in Java, bidirectional associations play a pivotal role in defining relationships between entities. However, they often lead to misunderstandings and pitfalls if not managed correctly. In this blog post, we will dive deep into bidirectional associations, highlight common pitfalls, and offer actionable advice on how to navigate these complexities.
Understanding Bidirectional Associations
In Java, a bidirectional association means that two classes refer to each other. This can often be seen in parent-child relationships where a parent class contains a reference to its child class and vice versa.
For instance, consider two classes: Author
and Book
. An Author
can write multiple Books
, while a Book
has exactly one Author
. This can be represented as:
class Author {
private List<Book> books = new ArrayList<>();
public void addBook(Book book) {
books.add(book);
book.setAuthor(this);
}
// Getters and other methods...
}
class Book {
private Author author;
public void setAuthor(Author author) {
this.author = author;
}
// Getters and other methods...
}
Why Bidirectional?
Understanding the "why" behind using bidirectional associations is crucial. They enforce the integrity of relationships in your models. In the example above, when you add a Book
to an Author
, it automatically sets the Author
on the Book
, ensuring both sides of the relationship are consistent.
Common Pitfalls
Let's discuss some common pitfalls developers face when managing bidirectional associations and how to avoid them.
1. Circular References
One of the first pitfalls is the creation of circular references. In the above model, it can be easy for one class to keep a reference to the other indefinitely.
Solution: Implement proper lifecycle methods, such as destructors or removal functions, to unhook objects when they are no longer needed.
Example of Correct Lifecycle Management:
public void removeBook(Book book) {
books.remove(book);
book.setAuthor(null); // Removing the reference
}
2. Inconsistent State
When maintaining bidirectional associations, it's crucial to update both entities simultaneously. Failing to do this leads to inconsistencies in the data.
Solution: Always ensure that whenever you're assigning a relationship from one side, you appropriately adjust the other side as shown in our addBook
method.
Avoiding Inconsistency:
author1.addBook(book1);
author1.addBook(book2); // Ensures book1 and book2 both have the correct author reference.
3. Memory Leaks
Improper handling of references can lead to memory leaks. If an object retains a reference to another object no longer in use, the garbage collector cannot reclaim that memory.
Solution: Use weak references when appropriate, or ensure that objects clean up references properly.
Best Practices for Managing Bidirectional Associations
To effectively manage bidirectional associations in Java, here are some best practices to consider:
1. Use Collections Wisely
When implementing bidirectional relationships, it may seem tempting to use arrays, but collections like List
, Set
, or Map
provide more flexibility.
Example of Using Collections:
class Author {
private Set<Book> books = new HashSet<>();
public void addBook(Book book) {
books.add(book);
book.setAuthor(this);
}
// Iteration and other methods...
}
A Set
will prevent duplicate books from being added, ensuring data integrity.
2. Ensure Bi-directional Synchronicity
To uphold a consistent state, make sure your methods maintain the synchronization of both sides of the relationship.
This can be achieved by implementing clear methods for adding or removing relationships.
Example of Methods:
class Author {
public void removeBook(Book book) {
if (books.remove(book)) {
book.setAuthor(null); // Clean up reference
}
}
}
3. Consider Using ORMs
When working with databases, consider using an Object-Relational Mapping (ORM) framework like Hibernate or JPA. ORMs inherently deal with bidirectional relationships and provide features to automate link management.
For more insights on ORMs, check out Hibernate's official documentation.
Example Scenario
Let’s put our knowledge into perspective with a practical example. Imagine building a simple library system consisting of Member
and Book
classes.
Models:
class Member {
private List<Book> borrowedBooks = new ArrayList<>();
public void borrowBook(Book book) {
borrowedBooks.add(book);
book.setBorrower(this); // Link both sides
}
public void returnBook(Book book) {
borrowedBooks.remove(book);
book.setBorrower(null); // Clean up reference
}
}
class Book {
private Member borrower;
public void setBorrower(Member member) {
this.borrower = member;
}
}
Why This Works:
In the above models, a Member
can borrow and return Books
. By utilizing the clear borrowBook
and returnBook
methods, it ensures that both classes always maintain a proper reference to one another.
Wrapping Up
Mastering bidirectional associations involves understanding their importance and the potential pitfalls. By implementing best practices such as lifecycle management, using collections effectively, and ensuring consistency, you can effectively work with bidirectional relationships in your Java applications.
Remember, maintaining clean and efficient code should always be a priority. For deeper insights into Java associations, you might want to explore Java's official documentation.
By following these guidelines, you can avoid common pitfalls and create robust applications that fully leverage the capabilities of Java's object-oriented programming paradigm. Keep experimenting, keep coding, and enjoy the journey of mastering Java!