Mastering Java 17: Navigating New Language Pitfalls
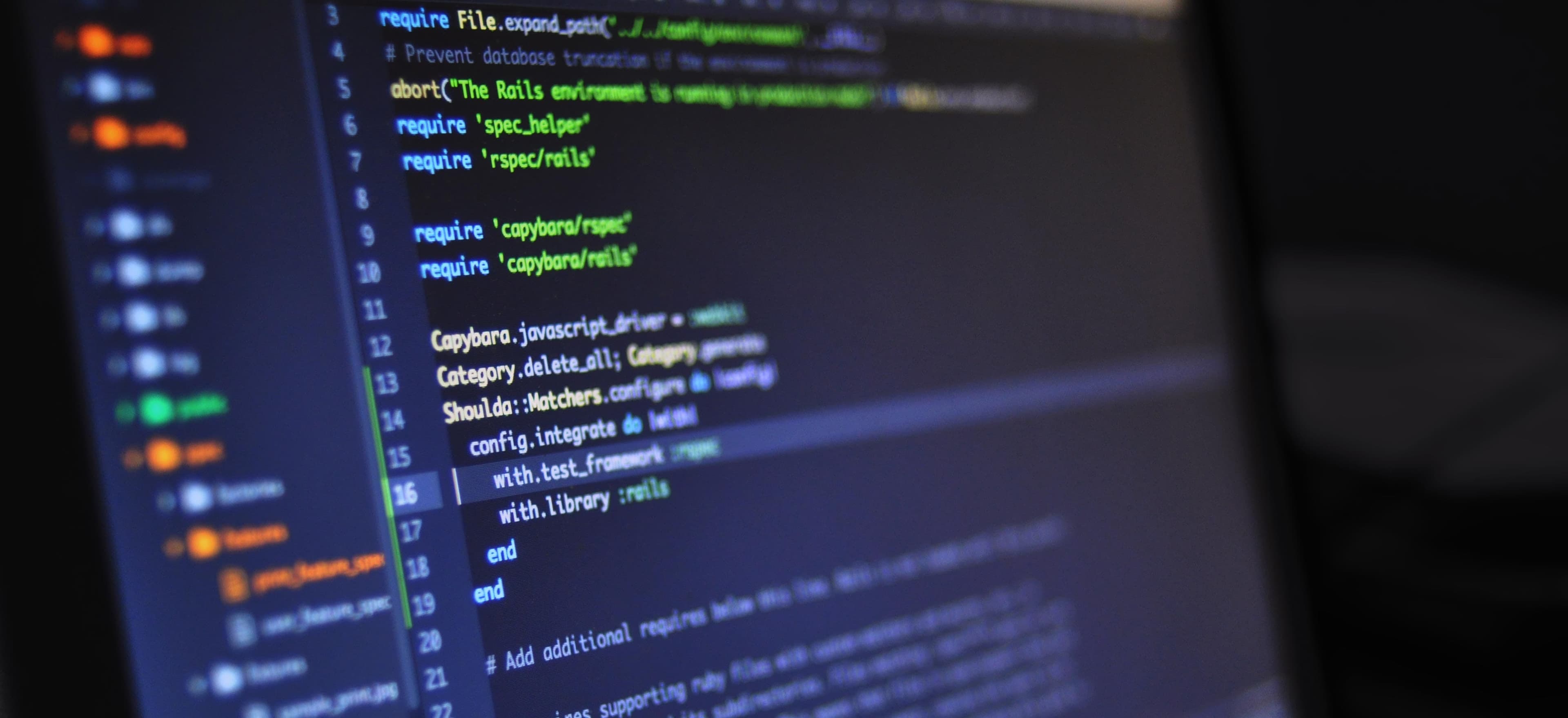
- Published on
Mastering Java 17: Navigating New Language Pitfalls
Java has long been a stalwart in the programming world, known for its portability, robust performance, and vast ecosystem. With the arrival of Java 17, a long-term support version, developers are greeted with a host of features that enhance their productivity and code quality. However, along with exciting enhancements come new potential pitfalls. In this guide, we will navigate the key updates in Java 17 and highlight common pitfalls that developers may encounter.
Key Features of Java 17
Before diving into pitfalls, let's first understand what Java 17 brings to the table:
- Sealed Classes and Interfaces: This concept allows developers to control which classes can extend or implement a class or interface. This is crucial for maintaining a controlled hierarchy.
- Pattern Matching for
instanceof
: This simplifies the code for type checks, reducing boilerplate code significantly. - New Language Features: Features like
switch
expressions, text blocks, and enhancedenum
capabilities. - Backwards compatibility: Ensures that existing Java applications run smoothly without needing substantial refactoring.
Let’s delve deeper into each of these and discuss pitfalls.
1. Sealed Classes and Interfaces
Sealed classes and interfaces promote better design by allowing class authors to define a closed set of subclasses.
public sealed class Shape
permits Circle, Square {
}
public final class Circle extends Shape {
private final double radius;
public Circle(double radius) {
this.radius = radius;
}
}
public final class Square extends Shape {
private final double side;
public Square(double side) {
this.side = side;
}
}
Why Use Sealed Classes? Sealed classes restrict inheritance, which helps improve the security and clarity of your code base. However, a common pitfall is overusing them. Remember: they can introduce complexity. Use them judiciously.
2. Pattern Matching for instanceof
Pattern matching simplifies the casting process. Instead of repeated type checks, you can streamline your code.
if (obj instanceof String s) {
System.out.println(s.length());
}
Elimination of Boilerplate Code This concise syntax reduces clutter and improves readability. Yet, developers may forget that pattern matching is only available in specific contexts.
3. Enhanced switch
Expressions
Java 17’s switch
expressions are a game changer. They offer a more powerful way to handle branching logic.
String dayType = switch (day) {
case MONDAY, FRIDAY, SATURDAY, SUNDAY -> "Weekend";
case TUESDAY, WEDNESDAY, THURSDAY -> "Weekday";
default -> throw new IllegalArgumentException("Invalid day: " + day);
};
Why the Change?
This approach allows for cleaner code and eliminates the need for break
statements. However, new users may misuse the ->
syntax by failing to include a default
case, leading to runtime exceptions.
4. Text Blocks
Text blocks provide a simpler way to handle multi-line strings, which is particularly useful for JSON or SQL queries.
String json = """
{
"name": "Java",
"version": "17"
}
""";
System.out.println(json);
Automatic Formatting Text blocks automatically manage line breaks, improving readability. Yet, a common mistake is forgetting to use the proper indentation, leading to awkward formatting in output.
Common Pitfalls in Java 17
Even with these advancements, developers should remain vigilant against certain pitfalls:
1. Overusing Sealed Classes
As previously mentioned, sealed classes serve a purpose, but unnecessarily restricting class inheritance can lead to limited extensibility. Use them primarily when you are certain that a fixed set of subtypes aligns with your design requirements.
2. Forgetting the New Syntax Nuances
With new features comes new syntax, which can easily confuse those used to Java’s previous iterations. Invest time in understanding these changes, particularly with pattern matching and switch expressions, to avoid common mistakes in production code.
3. Not Leveraging var
Java 17 allows for local variable type inference. Using var
can make your code less verbose.
var numbers = new ArrayList<String>();
Be Cautious: Overusing var
can hinder code readability, particularly for complex expressions. When types are not explicit, it may become difficult to ascertain the variable's type at a glance.
4. Ignoring Performance Improvements
Java 17 includes various performance enhancements, but developers may not fully utilize them. For instance, features such as the new garbage collector options and optimized method handles provide benefits but require close attention and understanding.
5. Not Reading Release Notes
With every new version, release notes contain critical information about deprecated features, migrations, and best practices. Familiarizing yourself with these details can save time and effort later on. The official Java 17 release notes are an invaluable resource.
Embracing the Change
Java 17 is undeniably powerful, but like any new technology, it comes with its challenges. By understanding both its features and pitfalls, developers can better navigate this landscape and exploit Java 17’s capabilities fully.
The Bottom Line
As we have explored, the latest iteration of Java is not only about new features but also about refining how we write and maintain code. By being mindful of the new language pitfalls, developers can enhance the robustness of their applications. Stay up to date with industry best practices and continue learning as the Java landscape evolves.
In embracing these changes, you position yourself as a forward-thinking developer ready to tackle modern software challenges.
For more insights, check out Oracle's official Java tutorials. Happy coding!