Navigating Java's Modular System: Common Pitfalls Explained
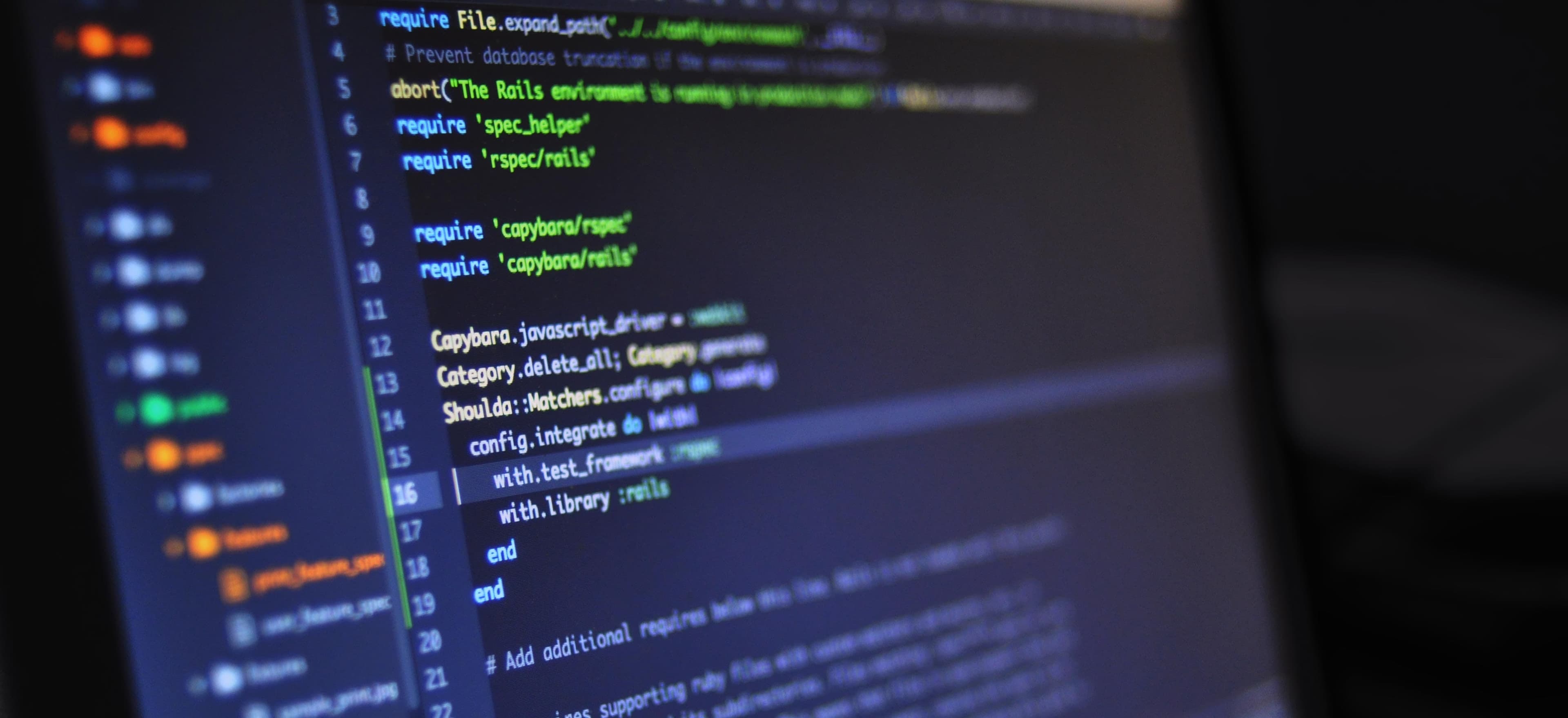
- Published on
Navigating Java's Modular System: Common Pitfalls Explained
In recent years, Java has undergone a significant evolution with the introduction of the modular system in Java 9. This modular system allows developers to package code and dependencies better, increasing maintainability and scalability. However, with this new paradigm comes a set of challenges and common pitfalls that developers must navigate. In this blog post, we will explore these pitfalls and provide effective solutions to manage them while implementing the Java modular system.
Understanding the Java Modular System
Before diving into common pitfalls, it's essential to understand what the modular system is and why it was introduced. The main goal of the Java Platform Module System (JPMS) is to provide a more structured way of organizing and managing code. Modularization allows developers to create smaller, reusable components called modules that can be independently managed.
Key Benefits of Modularization:
- Encapsulation: Modules limit the visibility of packages, exposing only what is necessary.
- Dependency Management: You can manage external dependencies more effectively.
- Improved Performance: The JVM can optimize performance by only loading necessary modules.
For a comprehensive understanding of JPMS, you can visit the official documentation.
Common Pitfalls in Using Java's Modular System
1. Mixing Module and Non-Module Code
One of the most common pitfalls developers face when transitioning to Java's modular system is mixing module and non-module code. This can create confusion, especially with improper accessibility and dependency management.
Solution:
Ensure that you consistently use modules across your project. If a part of your application doesn’t fit into the modular framework, consider wrapping it into a module or refactoring the code. Non-module code can lead to unexpected behaviors and runtime errors.
Example:
module com.example.service {
requires com.example.util;
exports com.example.service;
}
In the above snippet, com.example.service
explicitly requires com.example.util
. If you had non-module code that tried to access it directly, it could lead to runtime exceptions due to the modules’ encapsulation rules.
2. Misunderstanding the Module Path
Another critical area to watch is the module path. When you compile a modular application, using a classpath instead can lead to confusion. This is particularly true when working with dependencies.
Solution:
Always use the module path (--module-path
) rather than the classpath when running your application. This ensures that the JVM understands you're working with modules, allowing it to enforce modular policies correctly.
javac --module-path lib -d out src/com/example/*.java
java --module-path lib:out -m com.example/main
In this example, we explicitly define the module path that contains both the libraries and the compiled module as separate entities.
3. Not Defining the 'module-info.java' File Properly
The module-info.java
file is the centerpiece of the modular system, but many developers either fail to create it or do not configure it correctly. Errors in this file can result in accessibility issues and can confuse the dependency resolution process.
Solution:
Always define your module-info.java
correctly. Specify both the required modules and the packages you want to export clearly.
module com.example.app {
requires com.example.service;
exports com.example.app;
}
In this snippet, the module com.example.app
requires com.example.service
and exposes its packages. If anyone tries to access non-exported packages, they will encounter errors.
4. Circular Dependencies
Circular dependencies occur when two modules require each other, creating an unresolvable loop. This is a severe architectural issue that will make your application fail to compile or run.
Solution:
To solve circular dependencies, analyze the dependencies between modules carefully and refactor them. Sometimes, introducing a third module that both original modules can depend on can help.
module com.example.a {
requires com.example.shared;
}
module com.example.b {
requires com.example.shared;
}
module com.example.shared {
exports com.example.shared;
}
Here, com.example.shared
can serve as a common ground for com.example.a
and com.example.b
, breaking the circular dependency.
5. Improperly Handling Reflection
Java’s modular system introduces restrictions on reflective access for security reasons. Attempting to use reflection to access private members of a class in another module can lead to IllegalAccessException
.
Solution:
If reflection is necessary, consider using opens
within your module-info.java
. Doing so will allow specific packages to be dynamically accessible while maintaining modular encapsulation.
module com.example.app {
opens com.example.internal to com.example.tests;
}
Here, the com.example.internal
package is made accessible to the com.example.tests
module, allowing it access while ensuring proper encapsulation.
6. Dependencies Missing at Runtime
Oftentimes, developers face missing dependencies at runtime even after proper compilation. This is usually due to neglecting to include all required modules in the module path.
Solution:
Double-check all dependencies, ensuring that they are included in the module path when you execute your application. Review the module-info.java
for all required modules, and ensure these are located correctly.
java --module-path out:lib -m com.example.app/com.example.app.Main
Wrapping Up
While Java's modular system offers numerous advantages, a range of pitfalls can undermine these benefits if not properly navigated. By understanding and addressing these common pitfalls, developers can create more maintainable, robust applications.
In summary, keep in mind the following strategies to navigate the modular landscape effectively:
- Emphasize strict modularity.
- Always use the module path for compiling and running applications.
- Properly configure
module-info.java
. - Avoid circular dependencies by carefully analyzing architecture.
- Handle reflection access with the
opens
directive. - Verify all dependencies at runtime.
With diligence and awareness, the modular system can elevate your Java projects' architecture and performance.
For further reading, consider checking out these resources on modular programming in Java:
- Java Platform Module System (JPMS)
- Effective Java by Joshua Bloch – an insightful text on best practices in Java.
Embrace modularity, and watch your Java applications thrive!