Mastering Cross-Browser Testing: Overcoming Common Hurdles
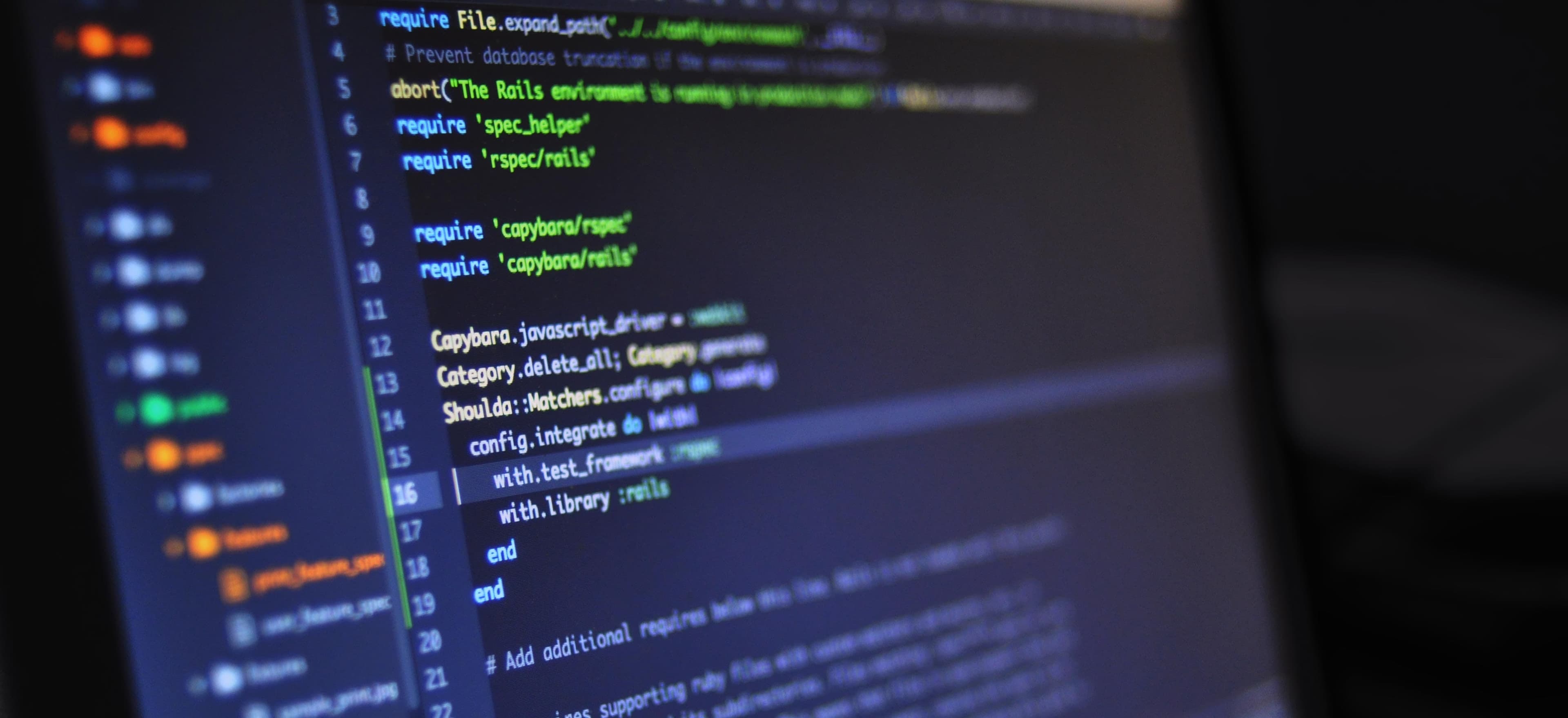
- Published on
Mastering Cross-Browser Testing: Overcoming Common Hurdles
Cross-browser testing is a crucial part of web development that ensures your website or application looks and behaves consistently across different web browsers and devices. In today's diverse web landscape, overlooking this essential step can lead to inconsistent user experiences and missed business opportunities. In this blog post, we will delve into the elements of cross-browser testing, common hurdles developers face, and strategies for overcoming these challenges.
Understanding Cross-Browser Testing
Cross-browser testing involves checking a web application's functionality, compatibility, and performance in different browsers, including Chrome, Firefox, Safari, Edge, and others. Every browser interprets HTML, CSS, and JavaScript somewhat differently, which can lead to variations in design and functionality.
The primary goal of cross-browser testing is to ensure that all users have a seamless and pleasant experience, regardless of their chosen browser.
Common Hurdles in Cross-Browser Testing
-
Inconsistent Renderings
- Different browsers may render the same HTML and CSS differently. This variance can lead to layout discrepancies, misplaced elements, or even functional errors.
-
JavaScript Behavior
- JavaScript features may behave differently on various browsers. Certain functions or methods may not be supported or may require polyfills for broader compatibility.
-
CSS Support
- CSS features often come with varying levels of compatibility. Some styles may work perfectly on one browser but completely break on another.
-
Mobile vs. Desktop Browsers
- The capabilities and rendering of browsers differ significantly between mobile and desktop. Responsive design can therefore compound testing efforts.
-
Legacy Browser Support
- Some users may still use older browser versions that do not support modern web standards, making thorough testing essential.
Strategies to Overcome Challenges
1. Leverage Cross-Browser Testing Tools
Using specialized tools can significantly streamline the testing process. Some popular cross-browser testing tools include:
These platforms allow developers to run tests across multiple browsers and devices simultaneously. They provide real-time testing, eliminating the need for managing numerous installations and setups.
2. Use Feature Detection
Instead of relying solely on user-agent strings to detect browsers, consider using feature detection libraries like Modernizr. Feature detection allows you to test for specific capabilities, which can help to gracefully handle browser inconsistencies.
// Example: Using Modernizr to check for Flexbox support
if (Modernizr.flexbox) {
// Flexbox is supported
// Apply flexbox styles
} else {
// Fallback for browsers without flexbox support
// Apply float or other styles
}
This approach is advantageous because it focuses on whether a feature is available rather than which browser is being used.
3. Embrace Responsive Design Principles
Building a responsive website ensures it works well on various devices. Employ frameworks like Bootstrap or CSS Grid for grid systems that adapt across devices.
/* Example: Using CSS Grid for responsive layouts */
.container {
display: grid;
grid-template-columns: repeat(auto-fill, minmax(250px, 1fr));
gap: 20px; /* Set spacing between grid items */
}
This strategy minimizes the need for device-specific testing and adapts the layout accordingly.
4. Use CSS Resets
Browsers apply default styles, which can lead to inconsistencies. CSS resets or normalize stylesheets can help to mitigate these differences by providing a consistent baseline.
/* Example: CSS Reset */
* {
margin: 0;
padding: 0;
box-sizing: border-box; /* Ensures padding and borders do not affect width */
}
A reset allows your design to be predictable across various browsers and minimizes cross-browser styling issues.
5. Continuous Integration/Continuous Deployment (CI/CD)
Integrating cross-browser testing into your CI/CD pipeline ensures testing is a regular part of your development process. Tools like Jenkins, CircleCI, or Travis CI can automate browser testing, catching issues before they reach the end-user.
6. Emphasize User Testing
In addition to automated testing, involving real users in the testing process can reveal issues that automated tools might miss. You can use platforms such as UserTesting to gain insights on real-world browser usage and behavior.
Example Testing Scenario
Consider you're developing a web application that includes a tab interface using JavaScript. Here's a simplified example:
<div class="tab">
<button class="tablinks" onclick="openTab(event, 'Home')">Home</button>
<button class="tablinks" onclick="openTab(event, 'News')">News</button>
</div>
<div id="Home" class="tabcontent">
<h3>Home</h3>
<p>Some content here.</p>
</div>
<div id="News" class="tabcontent" style="display:none;">
<h3>News</h3>
<p>Some content here.</p>
</div>
<script>
function openTab(evt, tabName) {
// Hide all tab contents
let tabcontent = document.getElementsByClassName("tabcontent");
for (let i = 0; i < tabcontent.length; i++) {
tabcontent[i].style.display = "none";
}
// Remove active class from all buttons
let tablinks = document.getElementsByClassName("tablinks");
for (let i = 0; i < tablinks.length; i++) {
tablinks[i].className = tablinks[i].className.replace(" active", "");
}
// Show the current tab and add an "active" class to the button
document.getElementById(tabName).style.display = "block";
evt.currentTarget.className += " active";
}
</script>
Why This Code Matters
In this example, we create a tab interface where each tab displays different content. This simple interaction requires testing across various browsers to ensure that events are handled properly and the display changes accordingly.
Testing interactions like these across browsers can highlight discrepancies in JavaScript execution timing or event propagation, leading to layout or data display issues.
In Conclusion, Here is What Matters
Cross-browser testing is no longer optional in the era of web development—it is indispensable. By understanding the unique hurdles it presents and utilizing strategic tools and methods, developers can ensure a consistent, high-quality user experience.
Remember, employing comprehensive testing and continuous integration will not only save time but also enhance the reliability of your web applications. Make cross-browser testing a key part of your development workflow to overcome potential pitfalls and deliver a seamless experience to all your users.
For more insights on testing methodologies, check out MDN Web Docs on Testing and learn how to stay ahead in your testing practices.
Happy testing!
Checkout our other articles