Unlocking Database Potential: XML vs. JSON Struggles
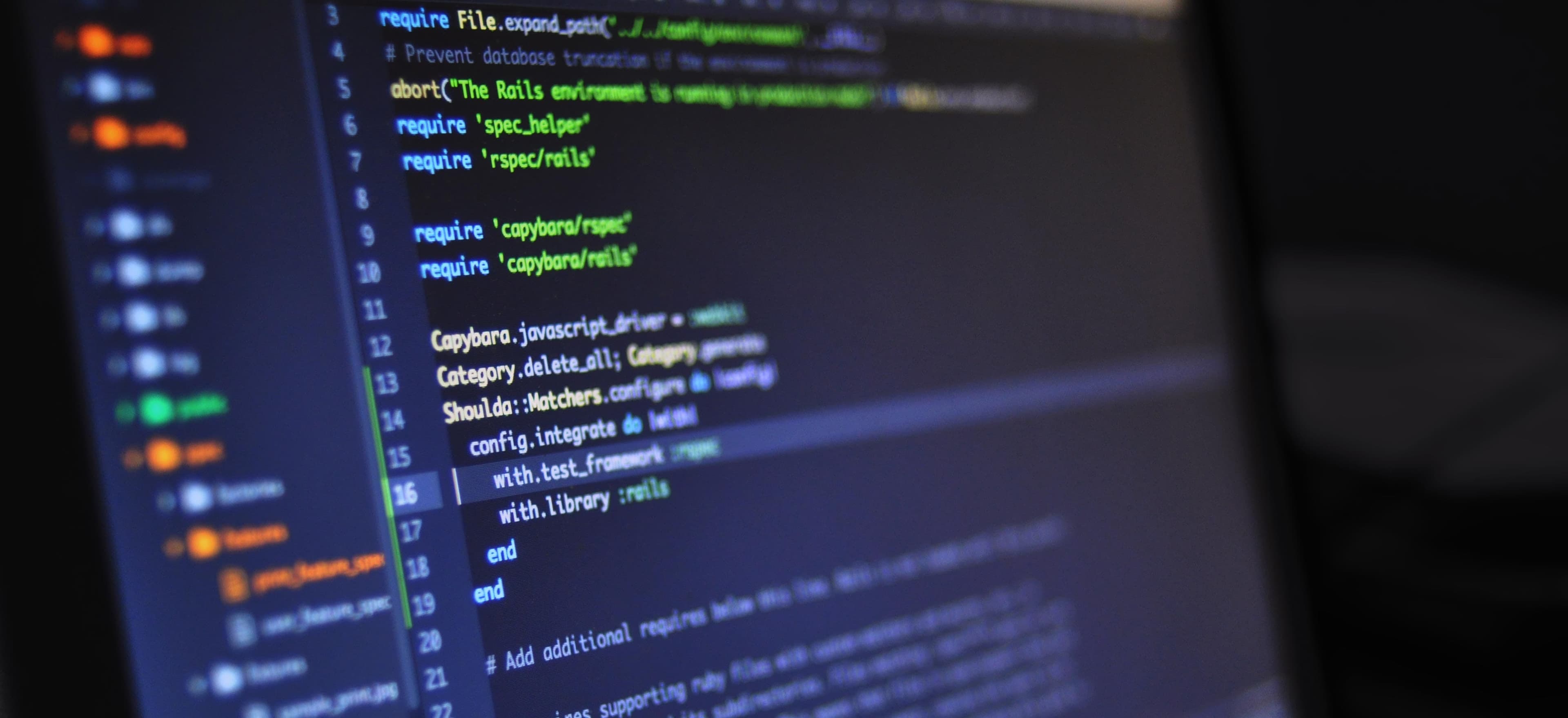
- Published on
Unlocking Database Potential: XML vs. JSON Struggles
When it comes to data interchange formats, XML (eXtensible Markup Language) and JSON (JavaScript Object Notation) are two heavyweights that have stood the test of time. Both serve the purpose of relaying data in a human-readable format, but they take significantly different approaches to structure and syntax. In this blog post, we will dive deep into the XML vs. JSON debate, assessing their strengths and weaknesses while providing insights into their utilization within a Java environment.
Understanding XML and JSON
What is XML?
XML is a markup language that’s designed to store and transport data. It is both human-readable and machine-readable, enabling easy data sharing across diverse systems. Here are some characteristics of XML:
- Hierarchical Structure: XML supports nested elements, making it excellent for representing data with complex relationships.
- Self-Descriptive: With tags indicating data type, XML can describe its data structure effectively.
- Extensible: New tags can be created as needed, offering a flexible solution for evolving data requirements.
Example XML Document:
<employees>
<employee>
<id>101</id>
<name>John Doe</name>
<department>Engineering</department>
</employee>
<employee>
<id>102</id>
<name>Jane Smith</name>
<department>Marketing</department>
</employee>
</employees>
What is JSON?
JSON, on the other hand, is a lightweight data interchange format primarily used for transmitting data between a server and web applications. Its main features include:
- Compact Structure: JSON uses less verbose syntax compared to XML, making it generally quicker to parse and traverse.
- Data Representation: JSON supports arrays and associative arrays, allowing for straightforward representation of complex data types.
- Native JavaScript Integration: JSON easily integrates with JavaScript, making it the go-to format for web applications.
Example JSON Document:
{
"employees": [
{
"id": "101",
"name": "John Doe",
"department": "Engineering"
},
{
"id": "102",
"name": "Jane Smith",
"department": "Marketing"
}
]
}
Performance Comparison
When considering performance, JSON usually has the upper hand. JSON data formats are leaner, translating to faster parsing and serialization processes. For tasks requiring quick data manipulation, JSON can substantially reduce load times.
Why Use JSON in Java?
Java developers often prefer JSON for its simplicity and ease of use with libraries like Jackson and GSON. Here's a brief demonstration of how to work with JSON in Java:
Parsing JSON in Java using Jackson
First, ensure you include the Jackson dependency in your pom.xml
file:
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>2.13.1</version>
</dependency>
Here’s a simple example:
import com.fasterxml.jackson.databind.ObjectMapper;
public class JsonExample {
public static void main(String[] args) {
ObjectMapper mapper = new ObjectMapper();
// JSON string
String jsonString = "{ \"id\": \"101\", \"name\": \"John Doe\", \"department\": \"Engineering\" }";
try {
// Deserialize JSON to Java object
Employee employee = mapper.readValue(jsonString, Employee.class);
System.out.println(employee);
} catch (Exception e) {
e.printStackTrace();
}
}
// Employee class representing JSON structure
static class Employee {
public String id;
public String name;
public String department;
@Override
public String toString() {
return "Employee{id='" + id + "', name='" + name + "', department='" + department + "'}";
}
}
}
Why Not Use XML in Java?
While XML has its uses, it often feels cumbersome in Java. An XML parsing library like JAXP can be more complex, requiring additional setup and knowledge of XML structure.
Parsing XML in Java using JAXB
For instance, here’s how you might parse the earlier provided XML using JAXB:
<dependency>
<groupId>javax.xml.bind</groupId>
<artifactId>jaxb-api</artifactId>
<version>2.3.1</version>
</dependency>
Then, create the classes to represent your XML structure:
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
@XmlRootElement(name = "employee")
class Employee {
private String id;
private String name;
private String department;
@XmlElement
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
@XmlElement
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
@XmlElement
public String getDepartment() {
return department;
}
public void setDepartment(String department) {
this.department = department;
}
@Override
public String toString() {
return "Employee{id='" + id + "', name='" + name + "', department='" + department + "'}";
}
}
This complexity can deter many developers from using XML in favor of JSON.
Use Cases: XML vs. JSON
When to Use XML
- Configuration Files: XML is widely utilized in configuration files, such as Maven's
pom.xml
. - Complex Data Structures: If your data is inherently hierarchical, XML may be the better choice, allowing for easier representation.
When to Use JSON
- Web APIs: Most modern web APIs prefer JSON for data exchange due to its efficient parsing.
- Mobile Applications: Given its compact size and speed, JSON is ideal for mobile applications needing to retrieve quick data.
Compatibility and Community Support
Both XML and JSON enjoy a broad level of community support and compatibility across programming languages. However, JSON has risen to prominence in the recent decade as a result of its lighter syntax and compatibility with JavaScript, making it a go-to for developers working on web technologies.
For a more detailed comparison and examples, check out W3School's JSON vs. XML page.
In Conclusion, Here is What Matters
Deciding between XML and JSON is not solely about technical superiority; it’s about selecting the right tool for your specific use case. JSON provides a quicker and simpler solution when working with web applications, mobile apps, and RESTful services, while XML might be the preferred option for applications requiring more intricate data relationships.
Ultimately, understanding your project requirements and the skill level of your team will help you unlock the full potential of these data formats, paving the way for effective data management and interchange.
References
- Jackson Library
- JAXB Reference Documentation
By weighing the pros and cons, you can make an informed choice that enhances your application's performance and maintainability. Whether you choose XML or JSON, the road to efficient data management starts with you.
Checkout our other articles