Mastering Your Game Loop: Avoiding Common Pitfalls in Android Games
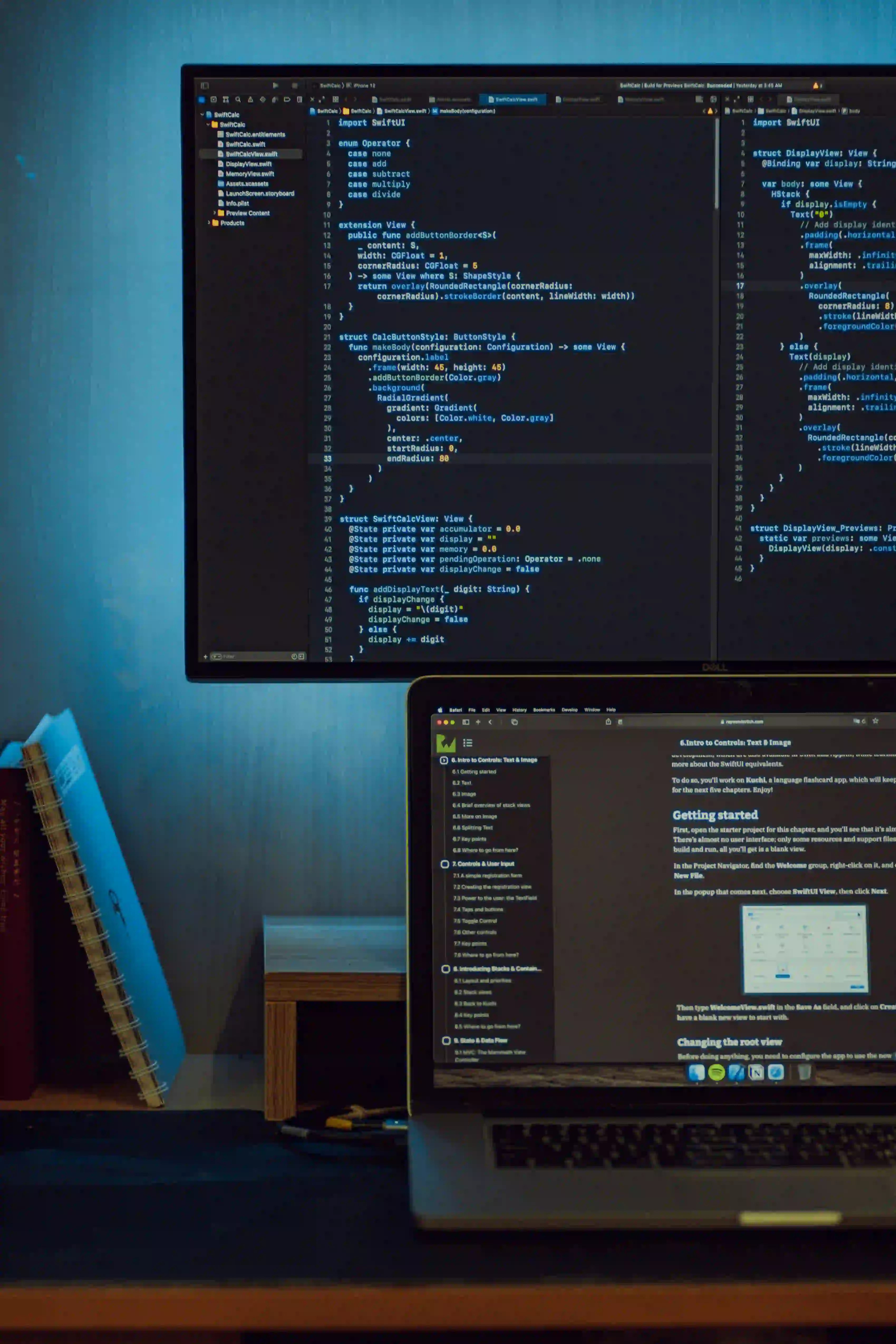
Mastering Your Game Loop: Avoiding Common Pitfalls in Android Games
Creating a game for Android is an exciting journey. However, at the heart of every game is the game loop, a fundamental concept in game development that requires careful attention. It is critical for updating game state, rendering graphics, and handling user inputs efficiently. Yet, many developers, especially beginners, stumble upon common pitfalls while implementing their game loops. In this blog post, we will explore the intricacies of game loops in Android development and how to avoid common mistakes.
What is a Game Loop?
A game loop is a continuous cycle that drives the game process. It continually updates the game state, renders graphics, and processes input. Typically, a standard game loop consists of three core functions:
- Update: Update game state, including positions of characters and other elements within the game.
- Render: Draw all game elements on the screen.
- Input Handling: Respond to user inputs for a responsive gameplay experience.
In this post, we will dive deep into the mechanics of the game loop and discuss how the improper implementation can lead to performance issues.
The Structure of a Game Loop
Below is a simple example of a game loop implemented in an Android game context.
public class GameThread extends Thread {
private boolean running = false;
private GameView gameView;
public GameThread(GameView gv) {
this.gameView = gv;
}
public void setRunning(boolean running) {
this.running = running;
}
@Override
public void run() {
while (running) {
long startTime = System.nanoTime();
// Update game state
gameView.update();
// Render game elements
gameView.render();
long elapsedTime = System.nanoTime() - startTime;
long sleepTime = (1000 / 60) - (elapsedTime / 1000000);
if (sleepTime > 0) {
try {
Thread.sleep(sleepTime);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
}
Code Breakdown
- Thread Management: Here, we create a
GameThread
that extendsThread
. This enables the game loop to run independently of the main UI thread. - Control Flow: The
run()
method contains a while loop that continues to execute while the game is running. - Timing: Utilizing
System.nanoTime()
allows us to maintain a frame rate. In our case, we aim for 60 frames per second by calculating the desired time to sleep after each loop iteration.
Common Pitfalls and How to Avoid Them
1. Blocking the Main Thread
One of the most common mistakes is doing heavy computations or long-running tasks in the main thread. Since the game loop runs in the main UI thread, this can lead to lag or a lack of responsiveness.
Solution:
Always execute long-running processes or blocking calls in a separate thread or utilize AsyncTask
or Executors
.
new AsyncTask<Void, Void, Void>() {
@Override
protected Void doInBackground(Void... voids) {
// Perform background operation
return null;
}
@Override
protected void onPostExecute(Void aVoid) {
// Update UI on the main thread
}
}.execute();
2. Inefficient Rendering
Poorly designed rendering routines can significantly affect game performance. Drawing too many objects or using high-resolution images without optimizations can lead to frame drops.
Solution:
Use efficient rendering practices such as:
- Batching Draw Calls: Consolidate multiple draw calls into a single call to reduce overhead.
public void render() {
canvas.drawBitmap(spritesheet, sourceRect, destRect, null);
}
- Texture Atlases: Combine several images into a single texture atlas to minimize state changes.
3. Not Using Delta Time
Delta time refers to the time elapsed since the last frame update. Failing to account for delta time can lead to inconsistent gameplay speeds across different devices.
Solution:
Accumulate the elapsed time and adjust movement updates accordingly.
public void update() {
long currentTime = System.nanoTime();
float deltaTime = (currentTime - lastFrameTime) / 1_000_000_000.0f;
lastFrameTime = currentTime;
player.move(deltaTime * playerSpeed);
}
4. Ignoring Frame Rate Variations
Different devices will have varying performance levels, which means your game might run slower on lower-end devices. If you rigidly set updates to occur at fixed intervals without accounting for performance, the gameplay experience may suffer.
Solution:
Implement dynamic adjustments to gameplay based on device performance. Frame rate can be capped, but allowing for frame variations leads to better user experience.
5. Failing to Manage Resources
Improper resource management can lead to memory leaks and performance degradation. Failing to release resources can cause crashes, especially in long-running applications.
Solution:
Use lifecycle methods wisely. For instance, use onPause()
to stop the game thread or release resources and onResume()
to restart them.
@Override
protected void onPause() {
super.onPause();
gameThread.setRunning(false);
}
@Override
protected void onResume() {
super.onResume();
gameThread.setRunning(true);
gameThread.start();
}
Resource Optimization Tips
- Use Sprites: For 2D games, sprites are a must. They save memory and rendering time by pre-rendering graphics.
- Compress Textures: Make sure to use compressed textures in your images to save memory.
Key Takeaways
Mastering your game loop is critical in developing efficient and responsive Android games. By avoiding the common pitfalls discussed in this blog, you can significantly enhance game performance and user experience.
Creating a responsive game is an iterative process. Always profile your game, test on various devices, and be ready to make adjustments. For further reading on game development, the following resources may help:
- Android Game Development Fundamentals
- Introduction to Game Programming
With these tools and knowledge in hand, you're well on your way to mastering game loops and creating incredible Android games. Happy coding!