Common Mistakes with @Transactional in Spring Applications
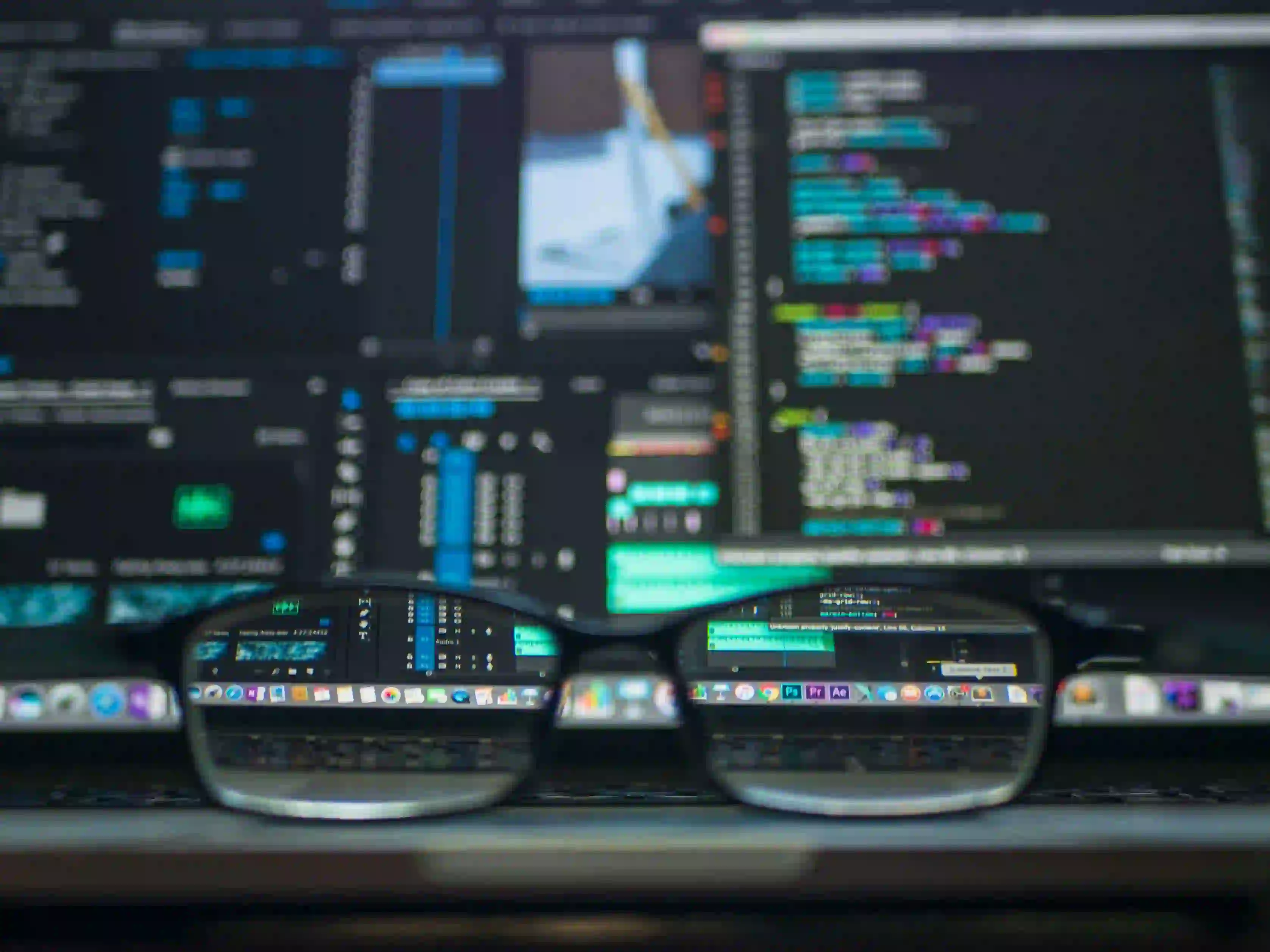
Common Mistakes with @Transactional in Spring Applications
Spring Framework has become the de facto standard for enterprise Java development. Among its many features, the @Transactional
annotation is pivotal for managing transactions effectively. However, improper usage can lead to subtle bugs, performance issues, or data inconsistencies. In this blog post, we will explore some common mistakes developers make while using @Transactional
in Spring applications. We'll provide code examples and clarify the reasons behind best practices.
Understanding @Transactional
Before we dive into the common pitfalls, let's review what @Transactional
does. It provides declarative transaction management, allowing developers to manage transactions on methods or classes without boilerplate code. When a method annotated with @Transactional
is called, a transaction starts. If the method completes successfully, the transaction is committed; otherwise, it is rolled back.
Basic Example
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
@Service
public class UserService {
@Transactional
public void createUser(User user) {
userRepository.save(user);
// Additional operations, like sending a welcome email, can be done here.
}
}
In this example, the createUser
method saves a user. If anything goes wrong during the execution of this method, the transaction will roll back, ensuring that no partial user data is committed.
Common Mistakes
1. Not Understanding the Propagation Behavior
One of the most common mistakes is misunderstanding the propagation level of transactions. The default propagation is REQUIRED
, which means that if there's already a transaction in progress, the current method will join that transaction.
Example of Misuse
@Transactional(propagation = Propagation.REQUIRES_NEW)
public void method1() {
method2();
}
@Transactional
public void method2() {
// Some logic
}
In the above code, if method1
starts a new transaction, method2
will not be part of it if REQUIRES_NEW
is used. This can lead to unexpected results, especially if method2
fails.
Recommendation
Always analyze your transaction requirements. Use REQUIRES_NEW
sparingly and only when you specifically want a new transaction to be created, independent of any active transaction.
2. Using @Transactional on Private Methods
In Spring, AOP (Aspect-Oriented Programming) is used to apply the @Transactional
annotation. However, AOP proxies only wrap public methods. Therefore, if you annotate a private method with @Transactional
, it will not have any transactional behavior.
Example of Misuse
@Service
public class UserService {
@Transactional
public void createUser(User user) {
saveUser(user);
}
private void saveUser(User user) {
userRepository.save(user);
}
}
In this example, when createUser
is called, the transaction will not encompass the call to saveUser
. The underlying save
operation will execute outside the transaction context.
Recommendation
Always apply the @Transactional
annotation to public methods. If you have shared logic, extract it to a public method.
3. Not Handling Exceptions Properly
By default, @Transactional
will only roll back on unchecked exceptions (like RuntimeException
) and errors. It won't roll back for checked exceptions unless specified.
Example of Misuse
@Transactional
public void processUser(User user) throws CustomException {
userRepository.save(user);
// Some other operations that might throw CustomException
}
In this case, if CustomException
is thrown, the transaction will not roll back, leading to potential data inconsistencies.
Recommendation
Clearly specify which exceptions should trigger a rollback using rollbackFor
:
@Transactional(rollbackFor = CustomException.class)
public void processUser(User user) throws CustomException {
// Logic here
}
4. Ignoring Transaction Timing
Another common error is not understanding the timing of transactions. Transaction boundaries can affect performance significantly.
Example of Misuse
@Transactional
public void batchProcess() {
for (User user : getUsers()) {
userRepository.save(user);
// Possibly costly operations inside the loop
}
}
This would start a transaction for each save operation, leading to performance bottlenecks.
Recommendation
Batch process operations strategically to minimize the transactional overhead:
@Transactional
public void batchProcess() {
List<User> users = getUsers();
userRepository.saveAll(users); // One transaction for all saves
}
5. Forgetting to Use Isolation Levels
Different transactions might interfere with each other. Understanding isolation levels is crucial to avoid phenomena such as dirty reads, non-repeatable reads, or phantom reads.
Example of Misuse
@Transactional
public void transferFunds(Account source, Account target, double amount) {
source.debit(amount);
target.credit(amount);
}
If multiple threads execute this method concurrently, it might lead to inconsistent states in your accounts.
Recommendation
Specify an appropriate isolation level based on your application's concurrency requirements when the default level is insufficient:
@Transactional(isolation = Isolation.SERIALIZABLE)
public void transferFunds(Account source, Account target, double amount) {
// transaction logic
}
6. Mixing Transaction Management Approaches
By mixing programmatic and declarative transaction management, you might unintentionally create unpredicted behavior.
Example of Misuse
@Transactional
public void addUser(User user) {
userRepository.save(user);
transactionManager.commit(); // Mixing approaches
}
In this scenario, you're manually controlling transaction management while also using @Transactional
, which can create confusion and lead to issues.
Recommendation
Stick to one transaction management approach: either use @Transactional
or manage transactions programmatically, but not both in the same method.
Bringing It All Together
Using the @Transactional
annotation correctly is crucial for the integrity and performance of your Spring applications. By avoiding the common pitfalls we've discussed, you can ensure that you manage transactions effectively, maintain data consistency, and prevent potential issues down the line.
Further Reading
For more details on transaction management in Spring, refer to the official Spring documentation: Spring Transaction Management. Understanding these principles can significantly improve development efficiency and application stability.
This post intended to simplify some of the complexities around @Transactional
. We invite feedback or questions in the comments below. Happy coding!