Troubleshooting JMS Template Message Conversion Issues
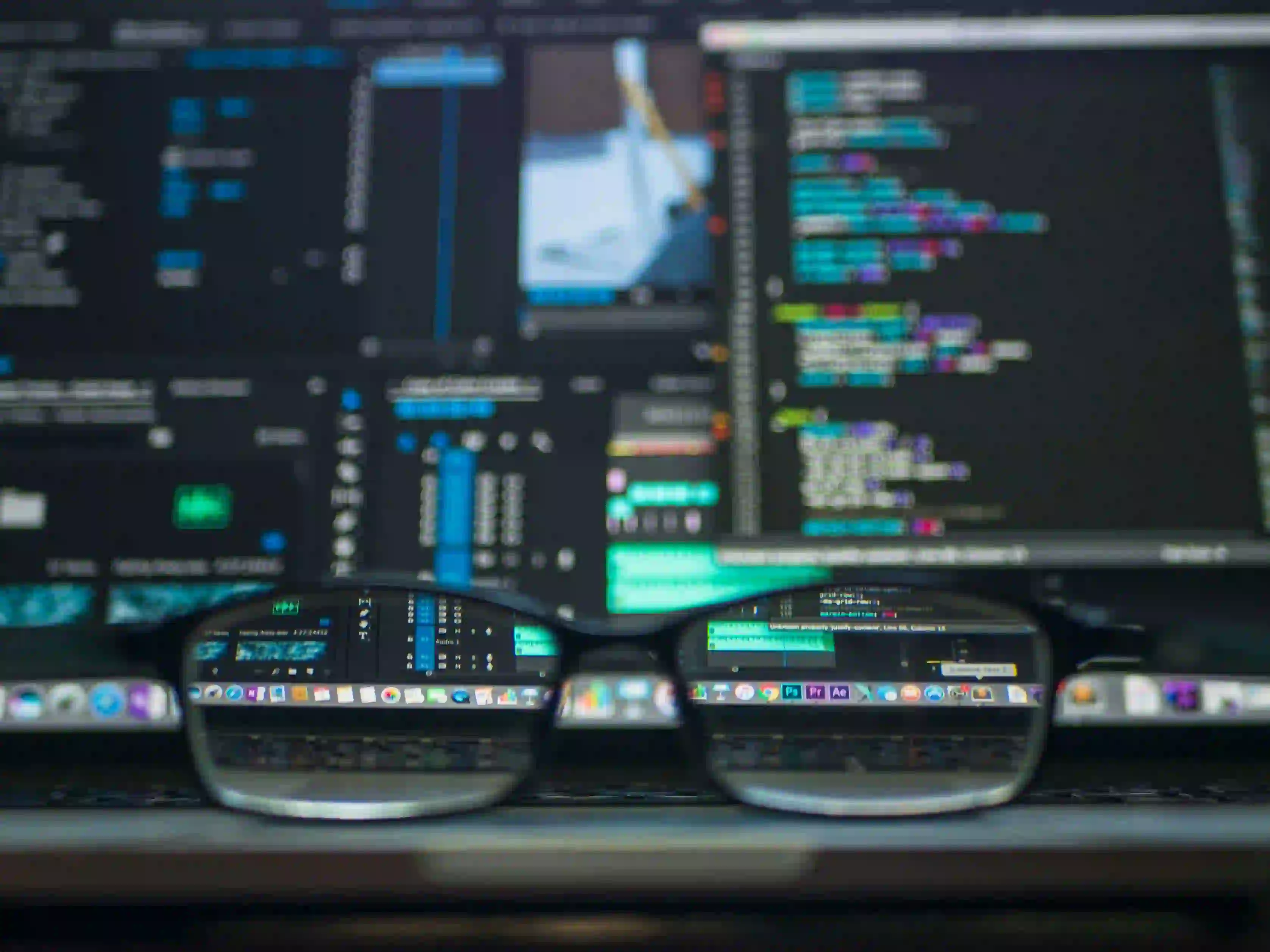
Troubleshooting JMS Template Message Conversion Issues in Spring
Java Message Service (JMS) is a critical component for creating distributed applications in Java. It provides a way for Java applications to create, send, receive, and read messages. When using Spring's JmsTemplate
, you may encounter message conversion issues, which can slow down development and cause frustration. In this blog post, we will explore common JMS Template message conversion issues and how to troubleshoot them effectively.
Understanding the Basics of JMS and JmsTemplate
Before we delve into the troubleshooting process, it's essential to understand what JMS and JmsTemplate
are.
- JMS: A Java API that allows communication between applications over a messaging protocol, facilitating asynchronous communication.
- JmsTemplate: A Spring framework class that simplifies sending and receiving messages. It abstracts the complexities of JMS APIs, making it easier to perform operations.
import org.springframework.jms.core.JmsTemplate;
import org.springframework.beans.factory.annotation.Autowired;
public class MyMessageSender {
@Autowired
private JmsTemplate jmsTemplate;
public void sendMessage(String destination, String message) {
jmsTemplate.convertAndSend(destination, message);
}
}
In the above code, we use JmsTemplate
to send a message to a specified destination. The convertAndSend
method takes care of converting the message appropriately.
Common Message Conversion Issues
When working with JmsTemplate
, you may face several message conversion issues:
- Unsupported Message Types
- Serialization Errors
- Incorrect Message Content-Type
- Message Listener Configuration
Let’s explore each issue in detail.
1. Unsupported Message Types
Spring’s JmsTemplate
supports several message types but may fail when it processes an unsupported type.
Example Code:
public void sendMessage(String destination, Object message) {
jmsTemplate.convertAndSend(destination, message);
}
Troubleshooting:
- Ensure the object you are trying to send is serializable. Non-serializable objects will throw a
NotSerializableException
. - Implement the
Serializable
interface in your message class.
public class MyMessage implements Serializable {
private String content;
// Getters and Setters
}
2. Serialization Errors
Serialization issues commonly arise when the receiving end cannot reconstruct the sent object.
Common Causes:
- Missing class definition on the consumer side.
- Version mismatches between the producer and consumer.
Troubleshooting:
- Ensure that the classes being sent and received implement
Serializable
. - If using custom serialization, ensure both classes use the same serialization strategies.
3. Incorrect Message Content-Type
In some scenarios, you may need your messages to have a specific content type (for example, JSON, XML). If this content type is misconfigured, it can lead to conversion issues.
Example Code:
import org.springframework.jms.core.MessageCreator;
import javax.jms.Message;
import javax.jms.Session;
public void sendJsonMessage(String destination, String jsonMessage) {
jmsTemplate.send(destination, new MessageCreator() {
@Override
public Message createMessage(Session session) throws JMSException {
Message message = session.createTextMessage(jsonMessage);
message.setJMSType("application/json");
return message;
}
});
}
Troubleshooting:
- Make sure the content type aligns with what your consumer is expecting. If you are sending JSON, verify that you are setting the correct content type.
4. Message Listener Configuration
An improperly configured message listener can also lead to conversion issues. If your listener does not match the expected message type, it will likely throw exceptions when processing incoming messages.
Example Code:
import org.springframework.jms.annotation.JmsListener;
import org.springframework.stereotype.Component;
@Component
public class MyMessageListener {
@JmsListener(destination = "myQueue")
public void onMessage(String message) {
System.out.println("Received message: " + message);
}
}
Troubleshooting:
- Ensure that the message listener expects the correct type. This means that a
String
listener cannot receive an object unless it has a corresponding converter. - Use converters if necessary to map your messages appropriately.
Enabling Logging for Troubleshooting
To better diagnose issues, enable detailed logging for your JMS components. You can add the following properties to your application.properties file:
logging.level.org.springframework.jms=DEBUG
logging.level.org.springframework.jms.listener=TRACE
This will provide insights into the message flow and possible conversion errors.
Testing with Unit and Integration Tests
Testing your message conversion logic can help identify issues before deployment. Use Spring's testing framework to write unit tests covering your JMS interactions.
import org.junit.jupiter.api.Test;
import org.mockito.InjectMocks;
import org.mockito.Mock;
import org.mockito.MockitoAnnotations;
import static org.mockito.Mockito.*;
public class MyMessageSenderTest {
@Mock
private JmsTemplate jmsTemplate;
@InjectMocks
private MyMessageSender myMessageSender;
@Test
public void testSendMessage() {
String destination = "myQueue";
String message = "Test Message";
myMessageSender.sendMessage(destination, message);
verify(jmsTemplate, times(1)).convertAndSend(destination, message);
}
}
This will ensure that your sendMessage
logic works as expected.
Summary
Troubleshooting JMS Template message conversion issues can seem daunting, but by understanding the common pitfalls and employing effective testing methods, you can shoot down these problems before they affect production.
To summarize:
- Ensure that message types are supported and serializable.
- Correctly manage content types to meet consumer expectations.
- Properly configure message listeners for expected types.
- Implement logging and testing as part of your development process.
For further details on JMS and Spring handling, you may want to explore the Spring JMS Documentation and the Java EE JMS Overview.
By following these guidelines, you can streamline your usage of JMS Template, avoid common issues, and ultimately create a more robust messaging system in your applications. Happy coding!