Troubleshooting External Calls in Jersey Client Testing
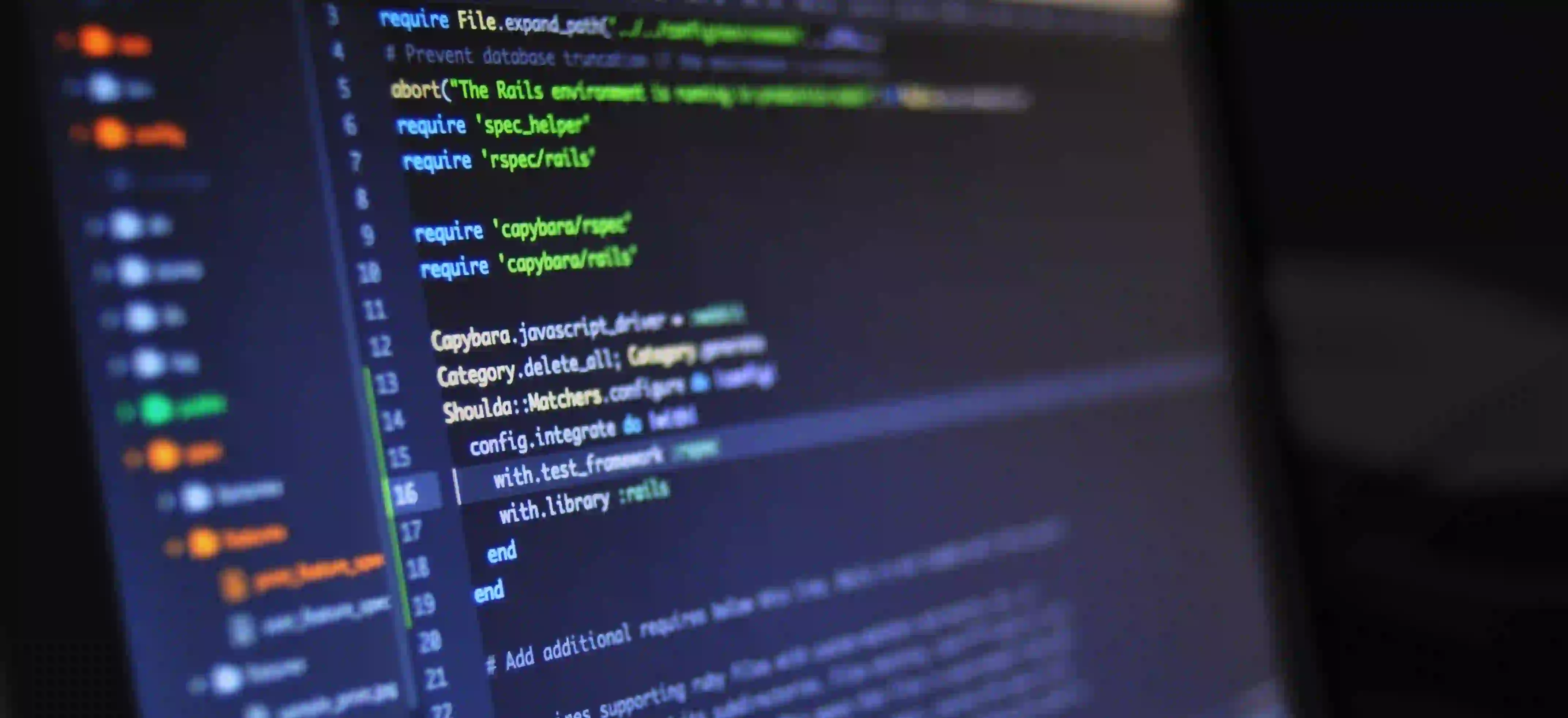
Troubleshooting External Calls in Jersey Client Testing
The Jersey client is a powerful library that simplifies the process of making RESTful calls from Java applications. However, working with external services can sometimes lead to complications due to network issues, server responses, or even API changes. In this blog post, we will explore effective strategies to troubleshoot external calls using the Jersey Client.
Understanding the Jersey Client
Jersey is an open-source framework that provides RESTful web services in Java. It simplifies the process of sending HTTP requests and processing responses. If you are integrating with various services, understanding how to effectively utilize the Jersey client can make your integration more robust.
Here is a basic example of how to use the Jersey client to make a GET request:
import javax.ws.rs.client.Client;
import javax.ws.rs.client.ClientBuilder;
import javax.ws.rs.core.Response;
public class JerseyClientExample {
public static void main(String[] args) {
Client client = ClientBuilder.newClient();
Response response = client.target("https://api.example.com/data")
.request()
.get();
String responseData = response.readEntity(String.class);
System.out.println("Response Data: " + responseData);
response.close(); // Always close the response
client.close(); // And the client
}
}
This example initiates a client, sends a GET request, retrieves a response, and finally prints the data. However, while making external calls, issues can arise.
Common Issues with External Calls
1. Network Issues
Transient network issues can occur, impacting connectivity with the external service. Ensure that the server is reachable from your environment. You can use tools like ping
or curl
to test connectivity.
Code Snippet for Handling Network Issues
import javax.ws.rs.core.Response;
import java.net.SocketTimeoutException;
try {
Response response = client.target("https://api.example.com/data")
.request()
.get();
} catch (SocketTimeoutException e) {
System.out.println("Network issue: Timeout occurred while trying to reach the service.");
}
This snippet catches timeout exceptions and provides a message for troubleshooting.
2. Server Response Codes
Understanding HTTP status codes is crucial. Responses can indicate different outcomes based on the server’s status. For instance:
- 200 OK: Successful requests
- 404 Not Found: Resource does not exist
- 500 Internal Server Error: Server encountered an unexpected condition
By logging and analyzing the response codes, you can gain insights into what might be wrong.
Code Snippet for Checking Response Codes
Response response = client.target("https://api.example.com/data")
.request()
.get();
if (response.getStatus() != 200) {
System.out.println("Error: " + response.getStatus());
}
This simple check can guide you to take appropriate actions based on the actual responses from the server.
3. Request Payload and Headers
Sometimes, the issue may arise from improperly formatted requests or missing headers. Always ensure you are sending the correct data and headers. For example, Content-Type should match what the API expects.
Code Snippet for Custom Headers
Response response = client.target("https://api.example.com/data")
.request()
.header("Authorization", "Bearer your_token")
.post(Entity.entity(data, MediaType.APPLICATION_JSON));
if (response.getStatus() != 200) {
System.out.println("Failed to post data: " + response.getStatus());
}
This example illustrates including an Authorization header in your POST request. Authenticate correctly to prevent 401 Unauthorized errors.
Logging Requests and Responses
Implementing logging in your Jersey Client can greatly aid in troubleshooting. The log can capture outgoing requests and incoming responses, allowing you to track down issues.
Code Snippet for Enabling Logging
import org.glassfish.jersey.logging.LoggingFeature;
Client client = ClientBuilder.newBuilder()
.register(LoggingFeature.class)
.build();
Using LoggingFeature
, you can easily monitor request and response details, making it easier to identify where things are going wrong.
Why Logging Matters
Logging helps in two significant ways:
- Traceability: It provides a clear path of requests and responses.
- Error Diagnosis: You can check the exact payloads being sent and received, making it easier to identify format issues.
Asynchronous Calls with Jersey Client
When working with potentially long-running external calls, utilizing asynchronous requests can prevent blocking. This enhances user experience and application responsiveness.
Code Snippet for Asynchronous Requests
import javax.ws.rs.client.InvocationCallback;
client.target("https://api.example.com/data")
.request()
.async()
.get(new InvocationCallback<Response>() {
@Override
public void completed(Response response) {
// Handle successful response
String responseData = response.readEntity(String.class);
System.out.println("Async Response: " + responseData);
}
@Override
public void failed(Throwable throwable) {
// Handle failure
System.out.println("Async request failed: " + throwable.getMessage());
}
});
With asynchronous calls, you can react to success or failure without blocking your main thread. This method is particularly useful for user interfaces.
Utilizing External Services for Better Insight
Sometimes it's beneficial to leverage external tools that can assist in monitoring and debugging. Services like Postman can simulate API calls and provide quick feedback. You can replicate your calls in Postman to see how they behave outside of your application.
My Closing Thoughts on the Matter
In this blog post, we have covered various aspects of troubleshooting external calls in Jersey Client testing. By understanding common issues, implementing robust logging, and utilizing asynchronous processing, you can create a much more reliable integration with external services.
For further reading, you can refer to the official Jersey Documentation and Spring REST Client for best practices on making RESTful calls using different frameworks.
By applying these strategies, you can mitigate challenges and ensure smoother interactions with external APIs. Happy coding!