Overcoming Memory Overhead in Adaptive Heap Sizing
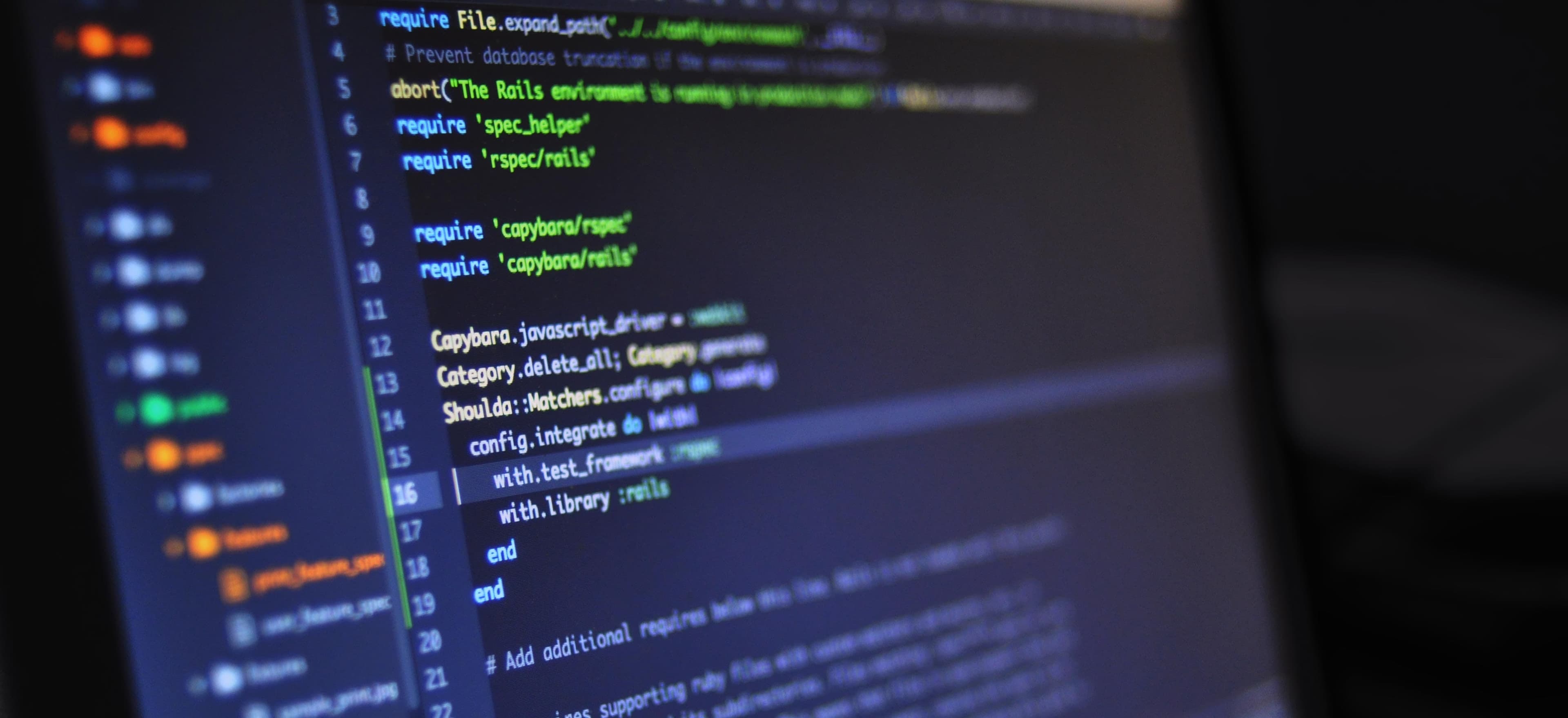
- Published on
Overcoming Memory Overhead in Adaptive Heap Sizing
Memory management is a critical aspect of Java programming. While Java abstracts away many complexities of memory management, understanding how to optimize memory usage is essential for developing efficient applications. One of the innovative techniques in this realm is Adaptive Heap Sizing, which can significantly improve memory efficiency. In this post, we will explore Adaptive Heap Sizing, discuss its benefits, and delve into how to overcome memory overhead often associated with its implementation.
What is Adaptive Heap Sizing?
Adaptive Heap Sizing is a technique used by the Java Virtual Machine (JVM) to dynamically adjust the size of the heap memory during the execution of a program. The JVM monitors memory usage and attempts to optimize the allocation of heap space based on the performance characteristics of the application. This leads to improved efficiency and reduced garbage collection (GC) pauses.
The Importance of Heap Management
Heap management is crucial because it directly impacts application performance. Efficient heap allocation minimizes GC times — the more efficiently the memory is managed, the less frequently the GC runs. Adaptive Heap Sizing ensures that there is just enough memory available for the application’s needs without wasting resources, which is typically the hallmark of static heap sizing approaches.
Benefits of Adaptive Heap Sizing
- Performance Optimization: Adaptive heap sizing can lead to enhanced performance by reducing GC pauses, thus providing a smoother experience during runtime.
- Resource Utilization: By adjusting to the application's needs, it minimizes wasted memory, leading to better resource utilization.
- Dynamic Adaptation: Unlike static approaches, adaptive sizing can handle varying workloads efficiently, making it ideal for applications with fluctuating memory requirements.
How Does It Work?
The JVM employs strategies to continuously monitor the applications' memory usage patterns. When memory pressure is detected, it either expands or shrinks the heap size. The primary components involved in this process include:
- Young Generation: Where new objects are allocated. This area is usually smaller and is collected more frequently.
- Old Generation: Where long-lived objects reside. Collecting this area is more cumbersome due to its size.
- Survivor Spaces: Allowing the JVM to distinguish between short-lived and long-lived objects.
When an application runs, the JVM collects statistics about memory usage. These statistics inform the Adaptive Heap Sizing decision-making process. The goal is to prevent OutOfMemoryExceptions while avoiding excessive memory allocation that can lead to overhead.
Implementation of Adaptive Heap Sizing
To enable Adaptive Heap Sizing, you typically adjust the following JVM parameters:
java -XX:+UseAdaptiveSizePolicy -Xms512m -Xmx2g -XX:MaxTenuringThreshold=4 -XX:NewRatio=3 -XX:SurvivorRatio=8 -jar YourApplication.jar
-XX:+UseAdaptiveSizePolicy
: Enables the adaptive size policy.-Xms512m
: Sets the initial heap size to 512MB.-Xmx2g
: Sets the maximum heap size to 2GB.-XX:MaxTenuringThreshold=4
: Limits the number of times a young object can survive a GC cycle before being promoted to the old generation.-XX:NewRatio=3
: Controls the allocation ratio between the young and old generations.-XX:SurvivorRatio=8
: Specifies the ratio between the Eden space and the Survivor spaces in the young generation.
Each parameter plays a role in molding the heap structure and controlling memory allocation dynamics, so understanding their implications is crucial.
Overcoming Memory Overhead
While Adaptive Heap Sizing is generally efficient, improper configuration can lead to memory overhead. Here, we discuss some strategies to mitigate this:
1. Monitor Memory Usage
Regularly monitor your application's memory footprint. Tools like VisualVM and JConsole can help visualize heap usage. Here’s a simple way to incorporate memory monitoring into your application:
import java.lang.management.ManagementFactory;
import java.lang.management.MemoryMXBean;
import java.lang.management.MemoryUsage;
public class MemoryMonitor {
public static void monitorMemory() {
MemoryMXBean memoryMXBean = ManagementFactory.getMemoryMXBean();
MemoryUsage heapMemoryUsage = memoryMXBean.getHeapMemoryUsage();
System.out.println("Used Heap Memory: " + heapMemoryUsage.getUsed() / 1024 / 1024 + " MB");
System.out.println("Committed Heap Memory: " + heapMemoryUsage.getCommitted() / 1024 / 1024 + " MB");
}
public static void main(String[] args) {
// Call this method at different points in your application to monitor memory
monitorMemory();
}
}
2. Fine-Tune Parameters
The JVM parameters for Adaptive Heap Sizing are not a one-size-fits-all approach. Test different configurations to find the best fit for your application. Always run performance tests that mimic production loads to evaluate their impact.
3. Leverage Profiling Tools
Utilize profiling tools like YourKit, Eclipse MAT, or JProfiler to analyze memory pressure points and understand how memory is allocated throughout its lifecycle. These tools provide invaluable insights into memory leaks, unnecessary object retention, and other issues that may contribute to overhead.
4. Conduct Load Testing
Load tests simulate the actual performance of your application under varying traffic conditions. Ensure that you do load testing before deploying to production. This will help you identify the optimal heap size and other JVM settings to minimize overhead.
Resources for Further Learning
For more detailed insights into Java memory management and heap tuning, consider exploring these resources:
- Oracle's Java Performance Tuning Guide
- JVM Garbage Collection and Memory Management
A Final Look
Adaptive Heap Sizing is a powerful feature of the JVM that allows for efficient memory management, significantly impacting an application’s performance. By dynamically adjusting the heap size based on the memory needs of the application, Java allows developers to write more efficient code without worrying as much about memory constraints.
However, it also comes with potential overhead. By applying the strategies discussed in this article—monitoring memory usage, fine-tuning parameters, leveraging profiling tools, and conducting load testing—you can minimize the risks associated with Adaptive Heap Sizing. Ultimately, a proactive approach toward memory management can lead to optimized applications, ensuring a smooth user experience.
And remember, the key to effective memory management lies in understanding your application’s unique behavior and needs. Each application is different, and only through careful analysis and adjustments can you achieve the best performance with Adaptive Heap Sizing. Happy coding!