Boost Your Spring Boot App: Common Performance Pitfalls
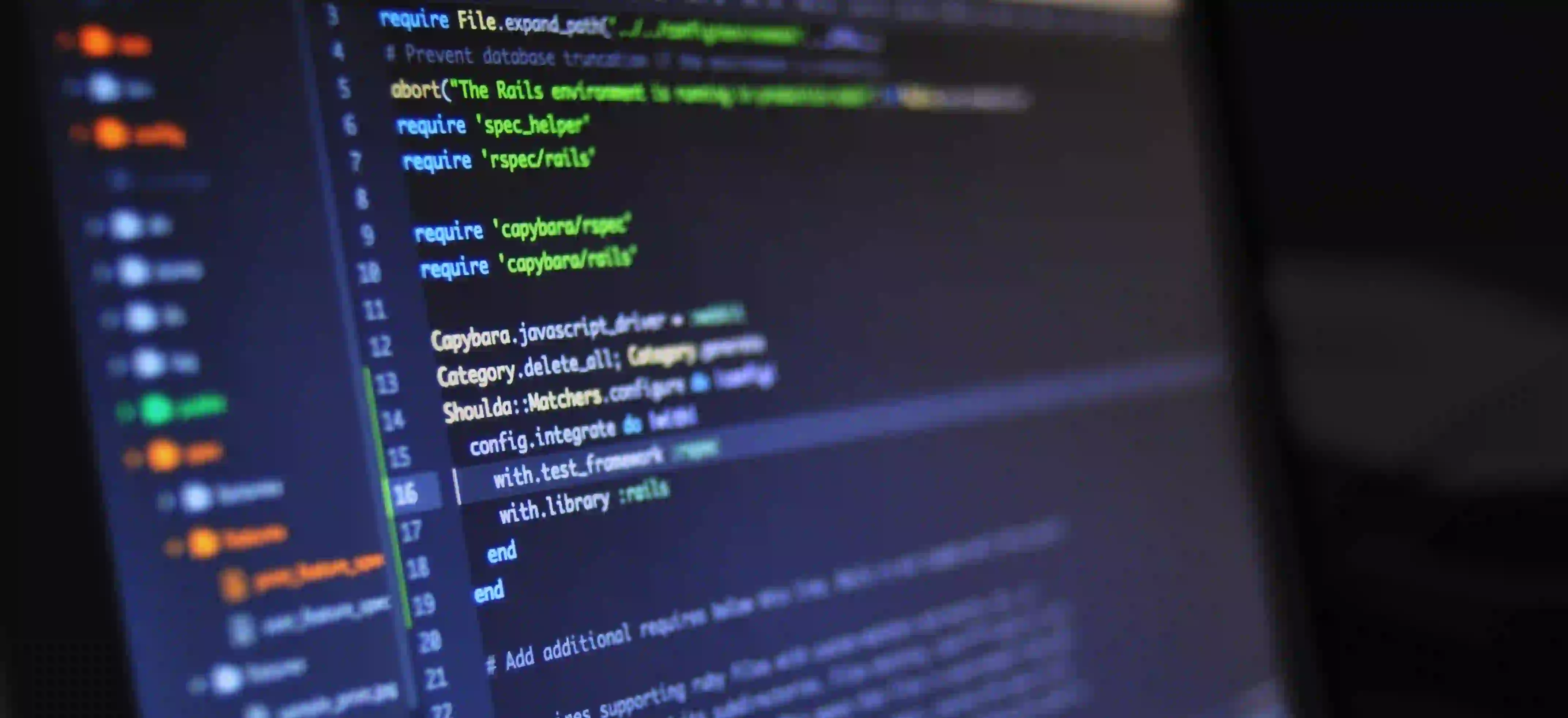
Boost Your Spring Boot App: Common Performance Pitfalls
Spring Boot is an immensely popular framework for building Java applications. Its ease of use, configuration, and rapid development features have made it a favorite in the developer community. However, something that can often be overlooked is performance optimization. In this blog post, we’ll delve into common performance pitfalls when developing Spring Boot applications and how you can avoid them for efficient, high-performing systems.
Understanding Performance Bottlenecks
Before diving into optimization techniques, it’s essential to understand what a performance bottleneck is. Bottlenecks are parts of your application that slow down processing. It could be caused by inefficient code, database interactions, or network latency. Addressing these can lead to significant improvements.
1. Dependency Injection Overhead
Spring's dependency injection is powerful, but it can become a performance overhead, especially when there are many beans to manage.
Why it matters: While DI (Dependency Injection) helps in decoupling our code, using it excessively can have startup time implications and unnecessary complexity.
Solution: Use constructor injection instead of field injection wherever possible. Constructor injection ensures that all required dependencies are satisfied at the time of object creation, which helps improve the performance during application startup.
@Component
public class MyService {
private final MyRepository myRepository;
@Autowired
public MyService(MyRepository myRepository) {
this.myRepository = myRepository;
}
// business logic methods
}
2. Inefficient Database Queries
Unoptimized database queries are a common source of performance problems.
Why it matters: Spring Boot integrates seamlessly with databases, but inefficient queries can slow down application response times significantly.
Solution: Always review your query performance using tools such as Hibernate’s query logs or your database's built-in analysis tools.
@Query("SELECT m FROM MyEntity m WHERE m.status = :status")
List<MyEntity> findByStatus(@Param("status") String status);
This query could be optimized with proper indexing in the database. Consider adding indexes to the fields that are often queried.
3. Caching Strategies
Not using caching is a major pitfall that can affect your app’s performance.
Why it matters: Caching prevents repetitive database calls and can speed up data retrieval times drastically.
Solution: Implement a caching mechanism using Spring Cache. You can easily cache data using annotations.
@Cacheable("users")
public User getUserById(Long id) {
return userRepository.findById(id).orElse(null);
}
By adding the @Cacheable
annotation, the method output will be cached. The next time the same method is invoked, it will check if the result is already available in the cache, significantly speeding up response times.
4. Excessive Logging
While logging is important, logging excessively can slow down your application.
Why it matters: It consumes IO resources and can introduce latency into request processing.
Solution: Use different logging levels wisely. During production, make sure you are only logging ERRORs and WARNs instead of DEBUG or INFO logs.
logging:
level:
root: error
org.springframework: warn
By adjusting the logging level in application.yml
, you can reduce overhead while conserving critical logs.
5. Ineffective Use of Executors
Java's Executor framework can manage thread pools efficiently, but incorrect configurations can lead to performance degradation.
Why it matters: An incorrectly sized thread pool can lead to thread contention, where too many threads compete for limited resources.
Solution: Configure the thread pool size based on expected load and performance benchmarks.
@Bean
public Executor taskExecutor() {
ThreadPoolTaskExecutor executor = new ThreadPoolTaskExecutor();
executor.setCorePoolSize(10);
executor.setMaxPoolSize(20);
executor.setQueueCapacity(100);
executor.initialize();
return executor;
}
This bean configuration will help handle tasks efficiently without overwhelming system resources.
6. Resource Management
Improper resource management, such as not closing connections or streams, can lead to resource leaks and lower application performance.
Why it matters: Resource leaks can crash your application due to a lack of available system resources.
Solution: Always use try-with-resources or ensure in a finally block that resources get closed properly.
try (Connection connection = dataSource.getConnection()) {
// some database operations
} catch (SQLException e) {
// handle exception
}
// No need for a finally block to close the connection
7. Profiling and Monitoring
Skipping application profiling is a frequent mistake made by developers.
Why it matters: Profiling and monitoring enable you to spot performance issues before they escalate to production failures.
Solution: Use tools like Spring Boot Actuator and applications like Prometheus or Grafana for monitoring.
To enable Actuator, add the following dependency and configure your application properties:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
management:
endpoints:
web:
exposure:
include: "*"
This will expose endpoints that provide performance metrics, helping you keep track of resource consumption and performance bottlenecks in your application.
Additional Resources
For those looking to dig deeper into Spring Boot performance, there are various resources available. Spring.io offers excellent documentation on best practices and performance optimizations. Additionally, consider reading “Effective Java” by Joshua Bloch for a broader perspective on Java performance optimizations.
Key Takeaways
Optimizing performance in your Spring Boot application is essential for creating scalable, efficient software. By being mindful of dependency injection practices, database queries, caching strategies, logging levels, and resource management, you can significantly improve your application’s performance. Always remember to monitor your application post-deployment, as this will provide invaluable insights into any areas that could still benefit from further optimization.
Implement these strategies today and watch your Spring Boot application soar to new heights!