Understanding BLOB vs CLOB: Key Differences Explained
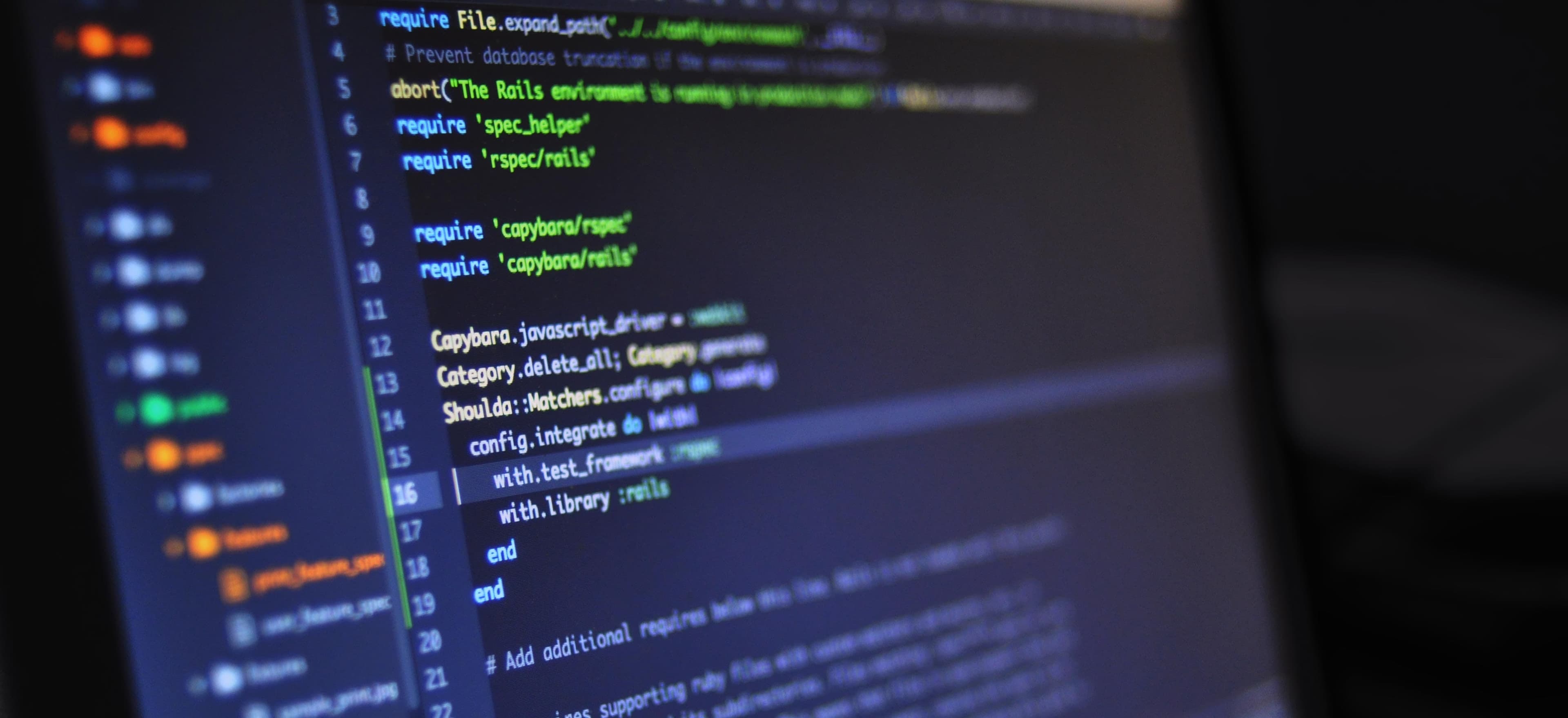
- Published on
Understanding BLOB vs CLOB: Key Differences Explained
In the world of databases, managing different types of data is essential for effective storage and retrieval. When dealing with large amounts of data, particularly text and binary data, we often encounter two key data types: BLOB (Binary Large Object) and CLOB (Character Large Object). This blog post aims to unpack these two types, explore their differences, and when to use each of them.
What is a BLOB?
A BLOB, or Binary Large Object, is a data type that is used to store large amounts of binary data. This could include images, audio files, videos, or other multimedia objects. The BLOB data type is particularly beneficial for applications requiring the storage of files as part of a database.
Characteristics of BLOB
- Storage of Binary Data: BLOBs are specifically designed for handling binary formats.
- No Character Set: BLOBs do not interpret the stored data as character data; hence, they do not require a specific character encoding.
- Size Limitations: While the size can vary between database systems, BLOBs can typically hold a significant amount of data, often up to several gigabytes.
- Manipulation: BLOBs are processed using specialized methods as text manipulation functions won't apply to binary data.
Code Snippet: Storing a BLOB
In this example, we will look at how to store a BLOB in a database using Java and JDBC:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.io.File;
import java.io.FileInputStream;
public class BlobExample {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/mydatabase";
String user = "username";
String password = "password";
String sql = "INSERT INTO mytable (myblob) VALUES (?)";
try (Connection conn = DriverManager.getConnection(url, user, password);
PreparedStatement pstmt = conn.prepareStatement(sql);
FileInputStream fis = new FileInputStream(new File("path/to/image.jpg"))) {
pstmt.setBlob(1, fis);
pstmt.executeUpdate();
System.out.println("BLOB data inserted successfully.");
} catch (Exception e) {
e.printStackTrace();
}
}
}
In this code snippet, we establish a connection to the database and prepare a SQL statement for inserting a BLOB. The file stream of an image is utilized here, showcasing how simple it is to adapt this approach for larger binary files.
What is a CLOB?
A CLOB, or Character Large Object, is aimed at storing large amounts of character data. This data type is ideal for handling substantial text such as articles, documentation, or other large strings.
Characteristics of CLOB
- Storage of Character Data: CLOBs handle textual data specifically, allowing for character encoding, like UTF-8.
- Character Set Required: Data in a CLOB is subject to character set rules, making it suitable for internationalization.
- Size: CLOBs can also store a significant amount of data, often similar to BLOBs, typically reaching several gigabytes.
- Text Manipulation: Since CLOBs are character sets, they can be manipulated with text functions such as concatenation and substring.
Code Snippet: Storing a CLOB
Here’s an example that illustrates how to store a CLOB in a database:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.Clob;
public class ClobExample {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/mydatabase";
String user = "username";
String password = "password";
String sql = "INSERT INTO mytable (myclob) VALUES (?)";
try (Connection conn = DriverManager.getConnection(url, user, password);
PreparedStatement pstmt = conn.prepareStatement(sql)) {
String textData = "This is a large text data that needs to be stored in a CLOB.";
Clob clob = conn.createClob();
clob.setString(1, textData);
pstmt.setClob(1, clob);
pstmt.executeUpdate();
System.out.println("CLOB data inserted successfully.");
} catch (Exception e) {
e.printStackTrace();
}
}
}
In this illustration, we create a CLOB and insert a lengthy string that can be managed as text data, showing how text integration into database systems is seamless.
Key Differences Between BLOB and CLOB
| Feature | BLOB | CLOB | |-----------------------------|-------------------------------|-------------------------------| | Data Type | Binary large objects | Character large objects | | Suitable For | Images, audio, video | Large texts, articles | | Character Encoding | N/A | Required (e.g., UTF-8) | | Text Manipulation | No | Yes | | Storage Size | Often up to several gigabytes | Often up to several gigabytes | | Functionality | Accessed with specialized methods| Accessed with SQL functions |
When to Use BLOB or CLOB
Use BLOB When:
- Your application is dealing with multimedia content.
- You need to store non-text files like images, audio, or videos.
- You require raw binary format access for files.
Use CLOB When:
- Your focus is primarily on large text strings or documents.
- You need to perform text-oriented operations on your data.
- You're working with applications that require text to be internationalized.
Lessons Learned
Understanding the differences between BLOB and CLOB is crucial for effective database design and management. Efficiently using these data types allows your application to handle large amounts of data in ways that enhance performance and scalability.
Whether it's storing multimedia files using BLOB or large text documents with CLOB, choosing the appropriate type can significantly impact your data handling. For deeper insights into BLOB and CLOB, you may refer to Oracle Documentation on LOBs and SQLite’s BLOB data type description.
Invest the time to understand how to utilize these data types effectively within your application, and you will be better prepared for the challenges of handling large volumes of data in your databases.
Keep coding, and happy programming!
Checkout our other articles