Struggling with Test Coverage? Tips for Meaningful Testing!
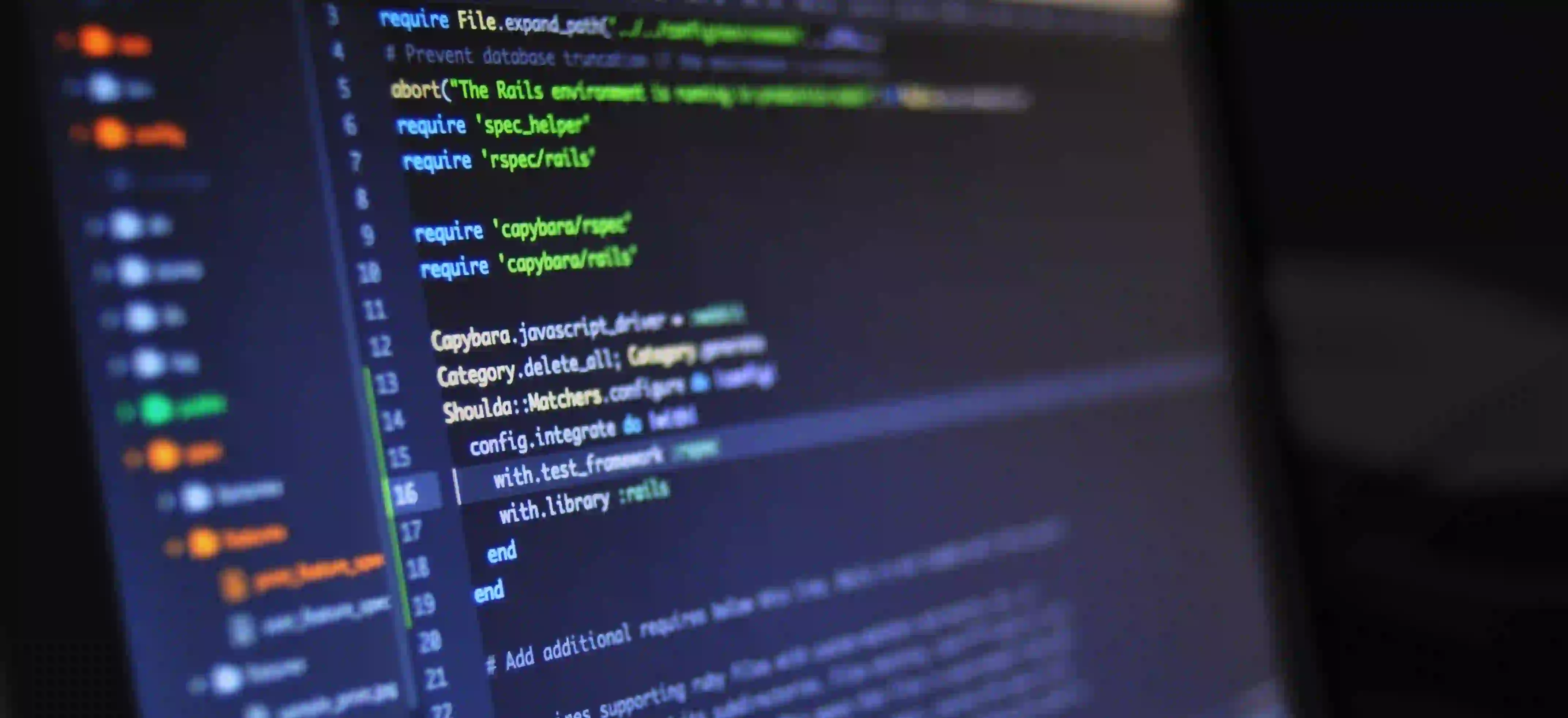
Struggling with Test Coverage? Tips for Meaningful Testing!
When it comes to software development, testing is more than just a necessary step in the process; it's a vital practice that ensures quality, reliability, and performance of the application. Test coverage serves as a key metric that signifies the amount of your codebase tested through automated tests. Unfortunately, many developers struggle with understanding and implementing meaningful test coverage. If this resonates with you, keep reading!
In this blog post, we'll explore concepts related to test coverage, its significance, and practical tips to achieve higher, meaningful coverage in your Java applications.
Understanding Test Coverage
What is Test Coverage?
Test coverage measures how much of your code is tested by automated tests. It can be expressed in several forms:
- Line Coverage: The percentage of executable lines of code executed while running tests.
- Branch Coverage: The percentage of branches in control structures (like
if
statements) tested. - Function Coverage: How many of the declared functions or methods have been called during tests.
While high coverage doesn't guarantee absence of bugs, it does act as a useful indicator of testing thoroughness.
Why is Test Coverage Important?
- Bug Detection: Higher coverage often correlates with identifying potential bugs early, saving time and costs later.
- Refactoring Confidence: When your code is well-tested, you can refactor or add new features without the fear of breaking existing functionality.
- Documentation: Tests serve as a form of documentation, illustrating how to use the code and showing its expected behavior.
Common Challenges in Achieving Test Coverage
Developers often face various obstacles when attempting to achieve meaningful test coverage:
- Overemphasis on Coverage Percentage: Sometimes teams fixate on achieving a specific percentage instead of focusing on testing functionalities that matter.
- Complex Code Structure: Code that is tightly coupled or poorly organized can be tougher to test.
- Integration Testing Issues: It can be difficult to derive unit tests when services depend on other services or components.
With these challenges in mind, let’s dive into some practical tips for improving test coverage in your Java applications.
Practical Tips for Meaningful Testing
1. Write Tests First (TDD)
Test-Driven Development (TDD) is an approach where tests are written before the actual code. This can be a game-changer for test coverage.
public class Calculator {
public int add(int a, int b) {
return a + b;
}
}
// Test Class
import static org.junit.jupiter.api.Assertions.*;
import org.junit.jupiter.api.Test;
public class CalculatorTest {
@Test
public void testAddition() {
Calculator calculator = new Calculator();
assertEquals(5, calculator.add(2, 3));
}
}
Why is this effective? TDD encourages you to think about test cases and edge cases from the start, resulting in better coverage and a more robust application.
2. Focus on Critical Paths
Not all code deserves the same level of testing. Identify and focus on critical paths—those parts of the application that, if broken, will severely impact performance or functionality.
For further reading on identifying critical paths, you can refer to Martin Fowler's Refactoring article.
3. Use Code Coverage Tools
Leverage tools like JaCoCo or Cobertura to analyze your Java codebase.
- JaCoCo: Offers detailed reports on test coverage including line, branch, and method coverage.
To integrate it into your Maven project, add the following plugin to your pom.xml
:
<plugin>
<groupId>org.jacoco</groupId>
<artifactId>jacoco-maven-plugin</artifactId>
<version>0.8.7</version>
<executions>
<execution>
<goals>
<goal>prepare-agent</goal>
</goals>
</execution>
<execution>
<id>report</id>
<goals>
<goal>report</goal>
</goals>
</execution>
</executions>
</plugin>
Why? This will help you visualize which parts of your code are being tested and allow you to focus your efforts where they can have the biggest impact.
4. Mock External Dependencies
When unit testing, avoid directly using external systems (like databases or APIs). Instead, use mocking frameworks such as Mockito or JMock. These allow you to create test doubles to isolate the code being tested.
import static org.mockito.Mockito.*;
public class UserService {
private UserRepository userRepository;
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
public User getUserById(int id) {
return userRepository.findById(id);
}
}
// Unit Test
import org.junit.jupiter.api.Test;
public class UserServiceTest {
@Test
public void testGetUserById() {
UserRepository mockRepo = mock(UserRepository.class);
UserService userService = new UserService(mockRepo);
User user = new User("John Doe");
when(mockRepo.findById(1)).thenReturn(user);
assertEquals(user, userService.getUserById(1));
}
}
Why mock? Mocking avoids many side effects that can arise from testing with actual external services, thus keeping your tests isolated and focused.
5. Review Coverage Reports Regularly
After running your tests and generating coverage reports, review these reports regularly. Identify untested areas and create tests for them.
- Focus on edge cases and exceptional scenarios that might not be covered.
6. Continuous Integration (CI)
Integrate testing into your CI pipeline. Tools like Jenkins, Travis CI, or GitHub Actions can automate running your tests and generate coverage reports on each commit.
Why CI? This will ensure that every change is validated against your tests, helping you catch issues early in the development cycle.
To Wrap Things Up
Achieving meaningful test coverage in Java doesn’t happen overnight. It requires strategic planning, a well-defined approach, and consistent practices.
By employing TDD, focusing on critical paths, utilizing the right tools, and regularly reviewing your coverage, you can significantly improve your ability to deliver reliable software while also enhancing your team's confidence during development.
There’s no one-size-fits-all when it comes to testing, but by applying the tips mentioned above, you can foster a culture of quality and improve code coverage in a meaningful way.
Take charge of your testing strategy today. The benefits are worth it!
Happy coding!
Feel free to leave comments below with your thoughts or experiences regarding taking charge of test coverage in your projects!