Common Mistakes in Validating JWTs with Spring Security
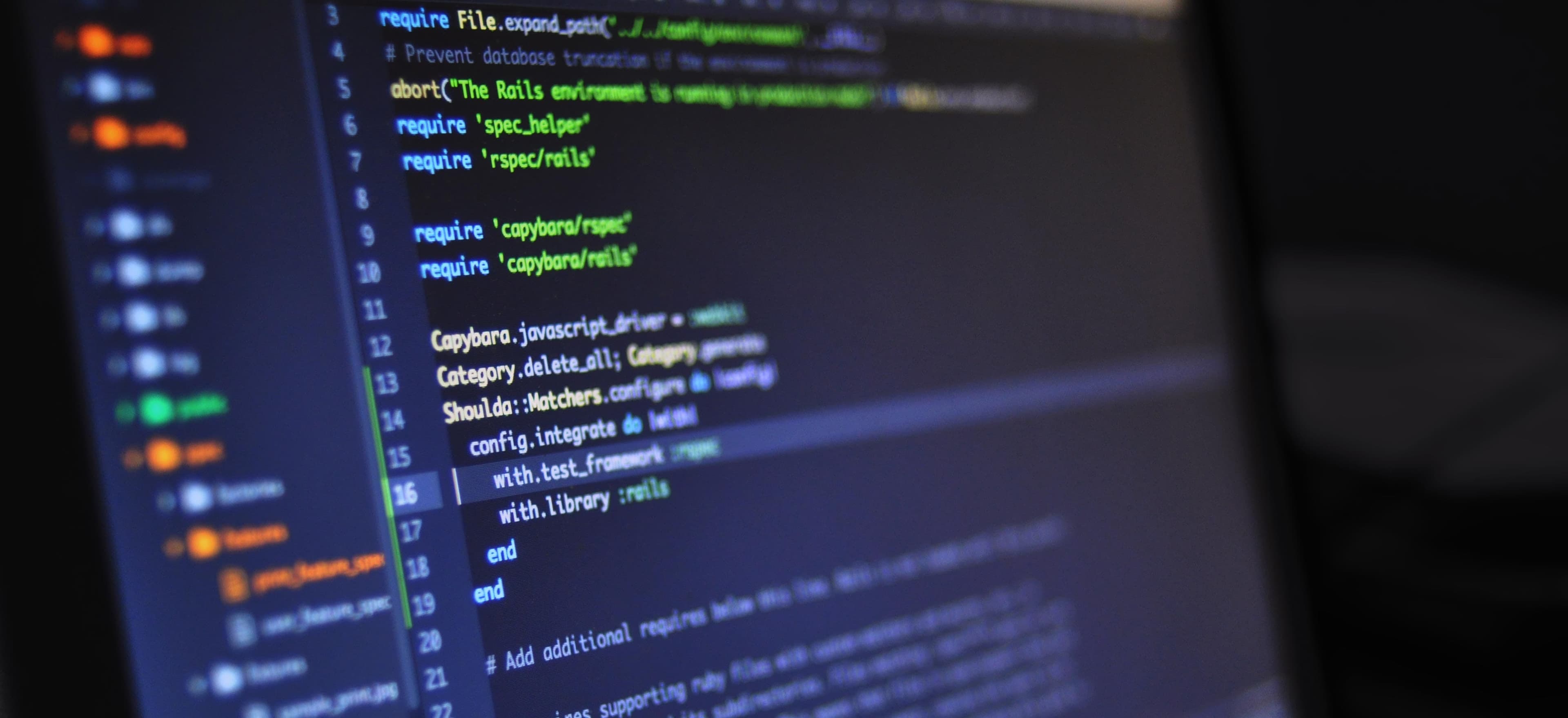
- Published on
Common Mistakes in Validating JWTs with Spring Security
JSON Web Tokens (JWTs) have become a popular choice for authentication and authorization in modern applications. They provide a stateless and compact way to verify users while maintaining scalability. When using JWTs in a Spring Security context, developers sometimes overlook critical aspects that can lead to security vulnerabilities. This blog post highlights common mistakes in validating JWTs with Spring Security, offering best practices to follow for a secure implementation.
Understanding JWTs
Before diving into the common mistakes, let’s briefly recap what a JWT is. A JWT consists of three parts: the header, the payload, and the signature. The header typically contains the type of the token (JWT) and the signing algorithm used. The payload contains the claims, which are the statements about an entity (typically, the user) and additional metadata. Finally, the signature is used to verify that the sender of the JWT is who it says it is and ensure that the message wasn't changed along the way.
For a more detailed overview, you can check out the official RFC 7519.
Common Mistakes in Validating JWTs
1. Ignoring Token Expiration
One of the most common mistakes developers make when working with JWTs is ignoring token expiration. JWTs can include an 'exp' (expiration) claim that indicates when the token is no longer valid. If you do not validate this claim, users can continue to use stale tokens indefinitely, potentially leading to unauthorized access.
import io.jsonwebtoken.Claims;
import io.jsonwebtoken.Jwts;
public Claims extractClaims(String token) {
return Jwts.parser()
.setSigningKey(SECRET)
.parseClaimsJws(token)
.getBody();
}
public boolean isTokenExpired(Claims claims) {
return claims.getExpiration().before(new Date());
}
In this example, the isTokenExpired
method checks whether the token is expired. Always check for expiration to maintain secure access.
2. Not Validating the Signature
Another frequent oversight is failing to validate the token's signature. The signature confirms that the sender of the token is who it says it is and ensures that the token has not been altered. By neglecting signature validation, you open the door to attackers who may send forged tokens.
To validate the signature, use the appropriate method in your JWT library:
public boolean validateToken(String token, UserDetails userDetails) {
final String username = extractUsername(token);
return (username.equals(userDetails.getUsername()) && !isTokenExpired(extractClaims(token)));
}
Here, we ensure the token is not only created by a valid issuer but also verify that it corresponds to an actual user.
3. Hardcoding Secrets
Hardcoding your signing key or secret is another issue. If an attacker gains access to your application source code, they can extract this information and forge valid JWTs.
Instead of hardcoding, use environment variables or configuration files to manage secrets securely. For instance, in your application.properties
:
jwt.secret=${JWT_SECRET}
And in your Java code, you can retrieve it like this:
@Value("${jwt.secret}")
private String secretKey;
4. Using Weak Algorithms
Using a weak signing algorithm can compromise your JWT's security. Algorithms such as HS256 are commonly used, but they must be implemented correctly. Ensure that your application is set to use a secure algorithm and keep your libraries updated.
String jwtToken = Jwts.builder()
.setSubject(userDetails.getUsername())
.setIssuedAt(new Date())
.setExpiration(new Date(System.currentTimeMillis() + EXPIRATION_TIME))
.signWith(SignatureAlgorithm.HS512, secretKey)
.compact();
In this snippet, we employ the HS512
algorithm, which provides a higher level of security than weaker alternatives.
5. Overlooking Revocation of Tokens
JWTs can be stateless, which makes the concept of revocation tricky. If you do not store a blacklist of revoked tokens, attackers can continue to use expired or compromised tokens. Consider employing a revocation strategy by maintaining a list of invalid tokens.
private Set<String> blackList = new HashSet<>();
public void invalidateToken(String token) {
blackList.add(token);
}
public boolean isTokenRevoked(String token) {
return blackList.contains(token);
}
In this example, we maintain a simple blacklist to keep track of invalidated tokens.
6. Inadequate Logging and Monitoring
Failing to implement adequate logging and monitoring can result in missing signs of security breaches related to token misuse. Always log suspicious activities, such as repeated invalid attempts to authenticate or access resources with invalid tokens.
logger.warn("Invalid token attempt for user: {}", username);
Setting up proper alerts when such activities are detected will allow you to take immediate action.
7. Not Validating Additional Claims
While the core claims like 'sub' (subject) and 'iat' (issued at) are primary, other claims like 'aud' (audience) and 'iss' (issuer) are vital for the security and appropriate functioning of your JWTs. Failing to validate these claims can expose your application to risk.
public boolean validateIssuer(Claims claims) {
return claims.getIssuer().equals(EXPECTED_ISSUER);
}
public boolean validateAudience(Claims claims) {
return claims.getAudience().equals(EXPECTED_AUDIENCE);
}
Incorporate comprehensive validation of claims to keep your authentication secure and precise.
Best Practices for JWT Validation
- Always Validate Expiration: Ensure that the token is checked for its expiration.
- Validate the Signature: Use a secure library to verify the token's signature accurately.
- Use Environment Variables: Store your signing secrets in environment variables.
- Employ Strong Signing Algorithms: Always use strong algorithms and stay updated on security practices.
- Implement Token Revocation: Maintain a blacklist of invalid tokens.
- Log Suspicious Activities: Apply logging and monitoring practices to detect unusual behavior.
- Validate All Claims: Make sure to validate all relevant claims in your JWT.
To Wrap Things Up
JWT validation is a critical aspect of securing your applications. By avoiding common mistakes and adhering to best practices, you can ensure your application maintains a robust level of security. As you maintain your application, remember that security is an ongoing process. Regularly review your implementation and stay informed about emerging security threats to mitigate risks efficiently.
For further reading on JWT authentication and authorization approaches using Spring Security, consider the official Spring Security documentation and the JJWT library documentation.
By addressing the discussed common mistakes, developers can successfully leverage JWTs while ensuring a higher degree of security in their applications.
Checkout our other articles