Top Java Parsing Libraries: Overcoming Common Challenges
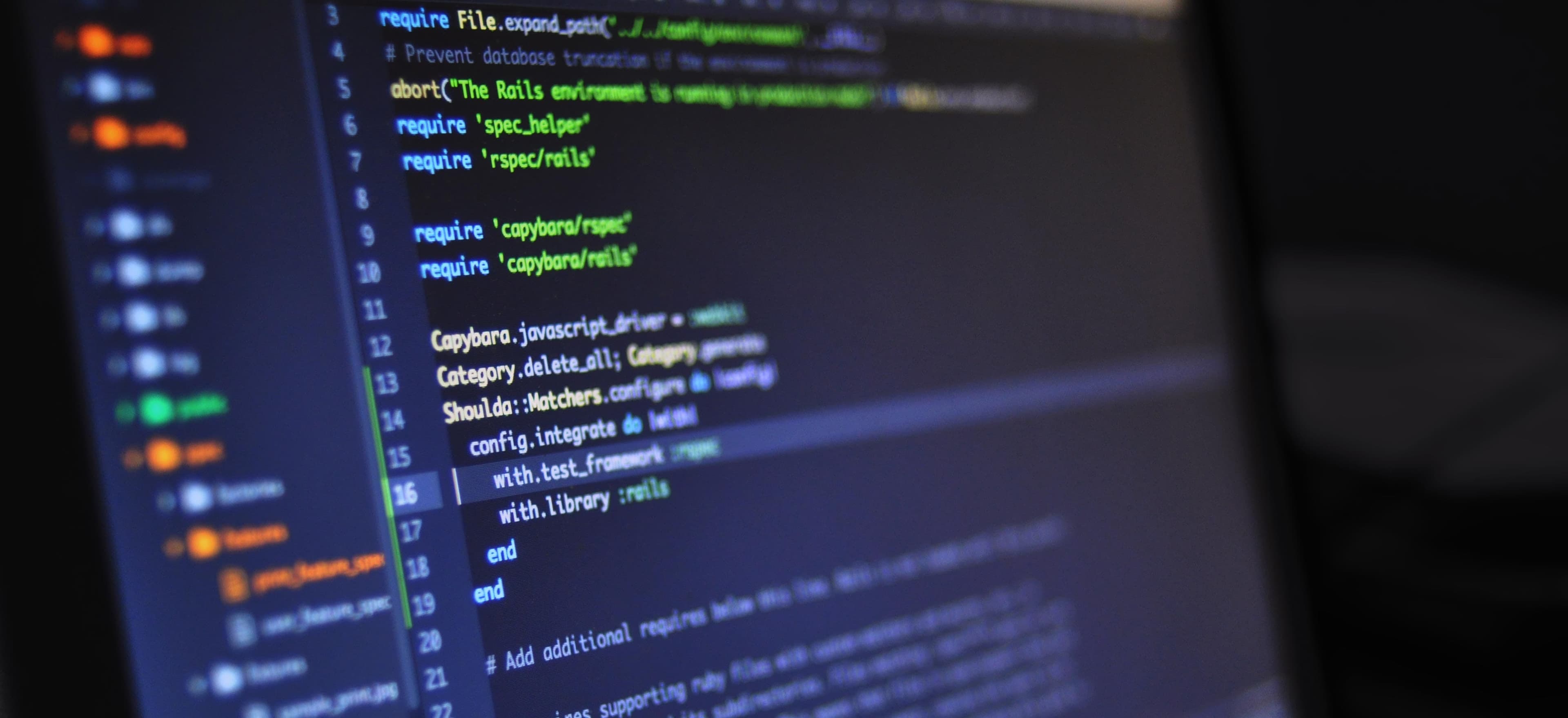
- Published on
Top Java Parsing Libraries: Overcoming Common Challenges
Parsing is a common task in software development. It involves analyzing a sequence of symbols, either in natural languages or in programming languages, and converting them into a more usable format. In Java, various libraries can assist developers in parsing data like XML, JSON, HTML, and even specialized formats like CSV. This blog post highlights some of the best Java parsing libraries, discusses common challenges developers face, and how these libraries help overcome them.
Understanding the Need for Parsing Libraries
Before we delve into specific libraries, let’s examine why using a dedicated parsing library is crucial.
- Time-Saving: Writing custom parsers from scratch can be time-consuming. Libraries reduce the workload significantly.
- Reliability: Well-established libraries are often tested across various scenarios, which means they are generally more reliable than home-grown solutions.
- Performance: Many parsing libraries utilize optimized algorithms that can handle large datasets efficiently.
Common Challenges in Parsing
- Data Format Variability: Different sources can represent the same data in various formats.
- Complex Structures: Nested and hierarchical data structures can complicate parsing.
- Error Handling: Dealing with malformed input is often challenging.
- Performance Issues: Parsing large datasets can lead to performance bottlenecks.
Understanding these challenges will help you appreciate the advantages offered by parsing libraries.
Best Java Parsing Libraries
Below, we will explore noteworthy Java parsing libraries that can help you tackle the common challenges mentioned above.
1. Jackson for JSON Parsing
Jackson is one of the most popular libraries for parsing JSON in Java. Its speed and ease of use make it a top choice.
Key Features:
- Data Binding: Automatically binds JSON to Java objects.
- Streaming API: Efficiently parses large JSON files.
Example Code Snippet:
import com.fasterxml.jackson.databind.ObjectMapper;
public class JsonParserExample {
public static void main(String[] args) {
ObjectMapper objectMapper = new ObjectMapper();
String json = "{\"name\":\"John\", \"age\":30}";
try {
// Deserialize JSON to object
Person person = objectMapper.readValue(json, Person.class);
System.out.println(person);
} catch (Exception e) {
e.printStackTrace();
}
}
}
class Person {
public String name;
public int age;
@Override
public String toString() {
return "Person [name=" + name + ", age=" + age + "]";
}
}
Commentary:
In this code snippet, Jackson's ObjectMapper
is used to deserialize JSON into a Java object. The simplicity of this process showcases Jackson's strengths—efficiently turning JSON data into usable Java objects with minimal code.
For more details on Jackson's features, you can visit the Jackson official documentation.
2. Jsoup for HTML Parsing
When it comes to parsing HTML, Jsoup is an outstanding library. It provides a clean API for extracting and manipulating data, using DOM, CSS, and JQuery-like methods.
Key Features:
- Querying: Allows easy querying of HTML elements.
- Robust Output: Handles malformed HTML gracefully.
Example Code Snippet:
import org.jsoup.Jsoup;
import org.jsoup.nodes.Document;
import org.jsoup.nodes.Element;
public class HtmlParserExample {
public static void main(String[] args) {
String html = "<html><body><p>Hello, World!</p></body></html>";
// Parse HTML and extract elements
Document doc = Jsoup.parse(html);
Element paragraph = doc.select("p").first();
System.out.println(paragraph.text());
}
}
Commentary:
In this example, the Jsoup
library is used to parse a simple HTML string. The ability to directly select elements and extract text showcases Jsoup's utility for web scraping or any task involving HTML manipulation.
For a comprehensive overview, visit the Jsoup documentation.
3. Apache Commons CSV for CSV Parsing
When dealing with CSV files, Apache Commons CSV is a mature and versatile library. It provides convenient and flexible ways to read and write CSV data.
Key Features:
- Custom Delimiters: Supports multiple delimiters.
- Header Support: Easily manages headers.
Example Code Snippet:
import org.apache.commons.csv.CSVFormat;
import org.apache.commons.csv.CSVParser;
import org.apache.commons.csv.CSVRecord;
import java.io.FileReader;
import java.io.Reader;
public class CsvParserExample {
public static void main(String[] args) throws Exception {
Reader in = new FileReader("example.csv");
CSVParser csvParser = new CSVParser(in, CSVFormat.DEFAULT.withHeader());
for (CSVRecord record : csvParser) {
String name = record.get("Name");
String age = record.get("Age");
System.out.println(name + ": " + age);
}
csvParser.close();
}
}
Commentary:
This snippet demonstrates how to read a CSV file and access each record's fields effortlessly. The withHeader
method allows for easy mapping of header names to values, showcasing the library’s flexibility in handling varied CSV structures.
4. ANTLR for Custom Language Parsing
If you're looking to parse domain-specific languages (DSLs) or build compilers, ANTLR (Another Tool for Language Recognition) is a powerful parser generator.
Key Features:
- Flexibility: Can parse any type of structured text.
- Code Generation: Offers options to generate code in multiple languages.
Example Code Snippet:
import org.antlr.v4.Tool;
public class AntlrExample {
public static void main(String[] args) {
// Simulating command-line input to ANTLR
String grammarFile = "MyGrammar.g4";
String inputFile = "input.txt";
Tool antlrTool = new Tool(new String[]{grammarFile, "-o", "outputDir", inputFile});
antlrTool.process();
}
}
Commentary:
In this example, we simulate invoking ANTLR to process a custom grammar. While specific grammar definitions are outside this snippet, ANTLR’s ability to generate parsers from these definitions makes it an exceptional tool for language processing tasks.
Bringing It All Together
Java offers a rich ecosystem of parsing libraries that can help developers tackle the myriad challenges associated with parsing data. Each of the libraries highlighted in this post provides unique capabilities designed to simplify your parsing tasks.
- Jackson for JSON: Excellent for data binding.
- Jsoup for HTML: Ideal for web scraping and HTML manipulation.
- Apache Commons CSV for CSV: Simplifies handling CSV files.
- ANTLR for custom languages: Great for defining and parsing new languages.
By leveraging these specialized tools, you can save time, avoid common pitfalls, and create more maintainable and efficient Java applications. Whether you are parsing JSON from web APIs, extracting data from HTML, reading CSV files, or building a custom language, these libraries will help you conquer the parsing challenges you face.
If you’ve had experiences with parsing libraries or want to share additional useful tools, feel free to leave your comments below! Happy coding!
Checkout our other articles