Struggling with Custom Audit Logs in Spring & Hibernate?
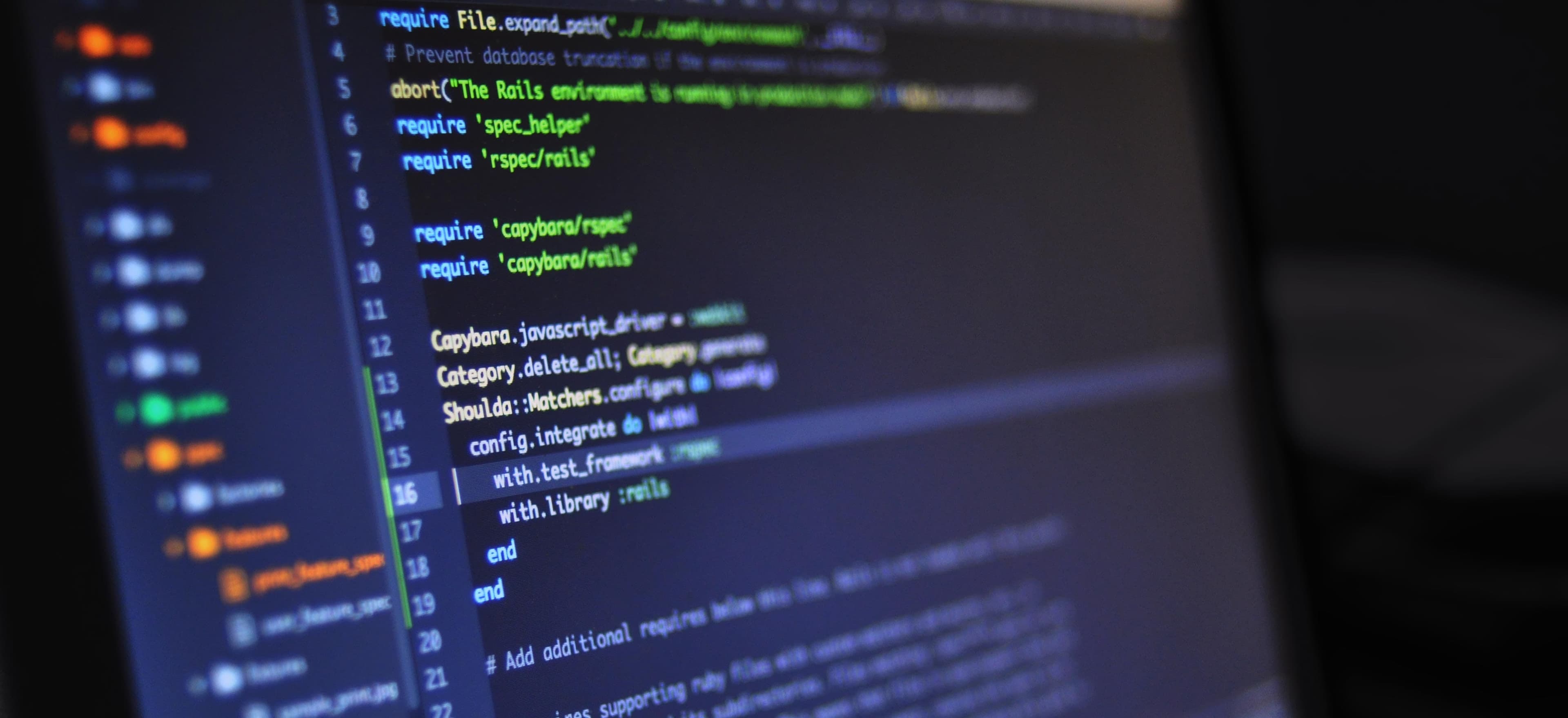
- Published on
Struggling with Custom Audit Logs in Spring & Hibernate?
In the realm of Java development, the use of Spring and Hibernate simplifies database interactions and web layer management significantly. One often overlooked yet vital aspect of modern applications is logging, particularly audit logging. Custom audit logs can help track changes, monitor user actions, and maintain compliance. But setting up custom audit logs can be challenging. If you find yourself grappling with this task, you're not alone. In this blog post, we will take a closer look at how you can implement custom audit logs in your Spring and Hibernate applications effectively.
Understanding the Need for Audit Logs
Before diving into the implementation, let’s establish why audit logs are important:
- Accountability: Who did what in your application? Audit logs help maintain a trail of actions taken by users.
- Compliance: Many industries require organizations to retain logs of user actions for regulatory compliance.
- Debugging: When issues arise in your application, logs provide a way to trace backward and identify the root cause.
Given their significance, implementing a custom auditing strategy is not merely a nice-to-have; it’s essential.
Setting Up Custom Audit Logs
Dependencies
To get started, ensure you have the following dependencies in your Maven pom.xml
:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
These dependencies provide support for Spring Data JPA and basic web functionalities.
Creating the Audit Entity
First, you need to create an entity that will represent the audit log. Here’s a simple audit log entity:
import javax.persistence.*;
import java.time.LocalDateTime;
@Entity
@Table(name = "audit_log")
public class AuditLog {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String entityName;
private String action;
private String username;
private LocalDateTime timestamp;
// Constructors, Getters, and Setters
}
Commentary
- Entity Name: This can be the name of the class whose changes you want to track.
- Action: This represents the operation performed (CREATE, UPDATE, DELETE).
- Username: Capture the user performing the action.
- Timestamp: It’s crucial to know when an action occurred.
Repository for Audit Logs
Next, you’ll want a repository to manage auditor log entries:
import org.springframework.data.jpa.repository.JpaRepository;
public interface AuditLogRepository extends JpaRepository<AuditLog, Long> {
}
Commentary
Using JpaRepository
eliminates boilerplate code by providing CRUD operations for the AuditLog
entity.
Aspect-Oriented Programming (AOP)
To capture changes automatically, we can leverage Aspect-Oriented Programming (AOP). Create an aspect class for auditing purposes:
import org.aspectj.lang.JoinPoint;
import org.aspectj.lang.annotation.AfterReturning;
import org.aspectj.lang.annotation.Aspect;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
@Aspect
@Component
public class AuditAspect {
@Autowired
private AuditLogRepository auditLogRepository;
@AfterReturning(pointcut = "execution(* com.example.repository.*.save(..))", returning = "result")
public void logAfterSave(JoinPoint joinPoint, Object result) {
AuditLog auditLog = new AuditLog();
// Assuming we have a way to get the user information
auditLog.setUsername("username"); // Replace with actual user retrieval logic
auditLog.setEntityName(joinPoint.getSignature().getDeclaringTypeName());
auditLog.setAction("CREATE");
auditLog.setTimestamp(LocalDateTime.now());
auditLogRepository.save(auditLog);
}
}
Commentary
In the above aspect:
- Pointcut: This defines the methods we want to monitor, in this case, any save operation in our repositories.
- JoinPoint: This represents the context of the intercepted method. You can access method parameters and other contextual information.
- Returning Value: Make sure to save only after the method has executed successfully.
Handling Other Actions
The above setup only logs CREATE actions. To accommodate UPDATE and DELETE actions, you can add similar pointcut definitions within the same aspect:
@AfterReturning(pointcut = "execution(* com.example.repository.*.delete(..))")
public void logAfterDelete(JoinPoint joinPoint) {
AuditLog auditLog = new AuditLog();
auditLog.setUsername("username"); // Replace with actual user retrieval logic
auditLog.setEntityName(joinPoint.getSignature().getDeclaringTypeName());
auditLog.setAction("DELETE");
auditLog.setTimestamp(LocalDateTime.now());
auditLogRepository.save(auditLog);
}
Displaying Audit Logs
Now that we have an audit logging system in place, it’s time to retrieve and display these logs. You can create a simple REST controller to expose the audit logs:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
import java.util.List;
@RestController
public class AuditLogController {
@Autowired
private AuditLogRepository auditLogRepository;
@GetMapping("/audit-logs")
public List<AuditLog> getAllAuditLogs() {
return auditLogRepository.findAll();
}
}
Wrapping Up
Congratulations! You have successfully set up a custom audit logging system in your Spring and Hibernate application. This simple but effective audit log captures critical changes and maintains accountability and compliance.
In case you want to further enhance or scale your logging mechanism, consider integrating with tools such as Logstash or ELK stack for better log management and visualization.
For additional readings on Spring AOP and its intricacies, check out the Spring AOP documentation.
Remember, a solid auditing framework can significantly elevate your application's integrity, making it easier for you to manage user actions across different components.
Feel free to leave any questions or experiences in the comments section below. Happy coding!