Overcoming Challenges in JUnit 5 DynamoDB Testing
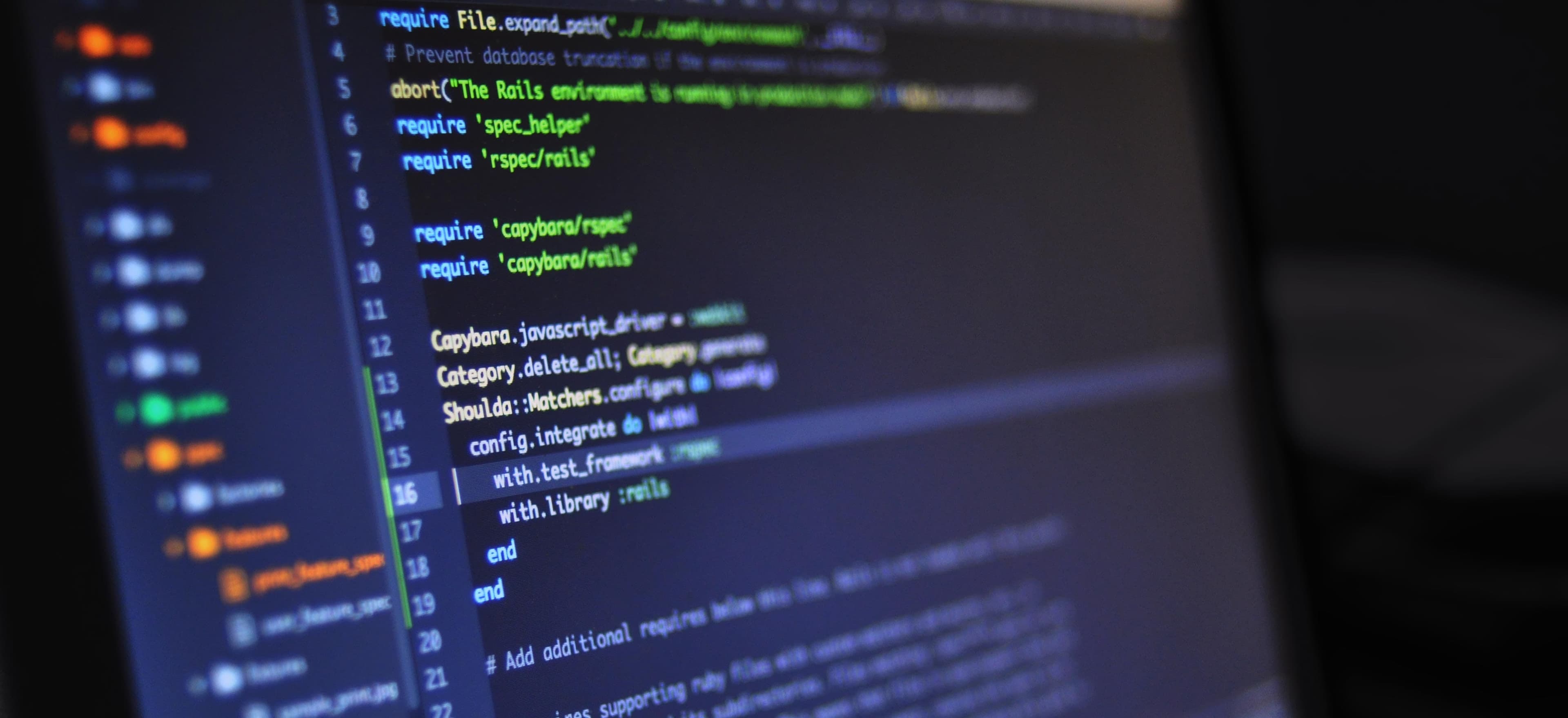
- Published on
Overcoming Challenges in JUnit 5 DynamoDB Testing
Testing is a critical component of software development, ensuring that your application behaves as expected. When it comes to working with AWS DynamoDB, effective testing becomes slightly more complex, particularly when using JUnit 5. In this blog post, we will delve deep into the challenges faced while conducting unit tests with JUnit 5 for DynamoDB, and how to overcome these hurdles with effective strategies and code implementations.
Understanding DynamoDB and Its Challenges
DynamoDB is a fully managed NoSQL database service provided by Amazon Web Services (AWS). It offers scalability, reliability, and low latency, but it does come with challenges, especially when you're trying to test your code.
- Latency and Network Issues: Testing against a live database can introduce variability due to network latency.
- Cost: Running many tests against a live DynamoDB instance can lead to unexpected costs.
- Data Integrity: Ensuring test data is set up and torn down properly can be cumbersome.
- Complex Setup: Mocking the service or using tools for local testing adds complexity.
Understanding these challenges sets the stage for overcoming them. Let's discuss how you can use JUnit 5 with DynamoDB effectively.
Setting Up Your Maven Project
To get started, ensure that you have the right dependencies in your Maven project. You'll need JUnit 5
for testing and AWS SDK
for interacting with DynamoDB.
Here’s an example of how your pom.xml
might look:
<dependencies>
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-api</artifactId>
<version>5.8.1</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>software.amazon.awssdk</groupId>
<artifactId>dynamodb</artifactId>
<version>2.17.112</version>
</dependency>
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-engine</artifactId>
<version>5.8.1</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.mockito</groupId>
<artifactId>mockito-core</artifactId>
<version>3.11.2</version>
<scope>test</scope>
</dependency>
</dependencies>
Modify the versions accordingly to your needs or check for the latest versions on Maven Central.
Using LocalStack for Local DynamoDB Testing
One of the best practices for testing against DynamoDB without incurring costs or dealing with a live environment is using LocalStack. LocalStack provides a fully functional local AWS cloud stack, allowing you to spin up a local version of DynamoDB.
Installation of LocalStack
First, you need to install LocalStack. You can run it via Docker:
docker run -p 4566:4566 -p 4510-4559:4510-4559 localstack/localstack
This command runs LocalStack, which exposes DynamoDB on port 4566.
Example of Setting Up a Local DynamoDB Client
Here is how to set up a local DynamoDB client for testing:
import software.amazon.awssdk.services.dynamodb.DynamoDbClient;
import software.amazon.awssdk.regions.Region;
public class DynamoDBClient {
public static DynamoDbClient createClient() {
return DynamoDbClient.builder()
.endpointOverride(URI.create("http://localhost:4566"))
.region(Region.US_EAST_1)
.build();
}
}
Explanation
In this example, we are creating a local DynamoDB client. The .endpointOverride
method points to our LocalStack, allowing us to interact with the local instance of DynamoDB, thereby eliminating the need for network calls that lead to latency issues.
Writing Your First JUnit 5 Test
Let’s write a simple test case for a DynamoDB table operation.
Creating a Table and Inserting Data
First, we will need a helper method to create a table:
import software.amazon.awssdk.services.dynamodb.model.*;
public class DynamoDBTestHelper {
public static void createTable(DynamoDbClient dynamoDB) {
CreateTableRequest request = CreateTableRequest.builder()
.tableName("TestTable")
.keySchema(KeySchemaElement.builder().attributeName("Id").keyType(KeyType.HASH).build())
.attributeDefinitions(AttributeDefinition.builder().attributeName("Id").attributeType(ScalarAttributeType.N).build())
.provisionedThroughput(ProvisionedThroughput.builder().readCapacityUnits(1L).writeCapacityUnits(1L).build())
.build();
dynamoDB.createTable(request);
}
}
Explanation of Code
- Key Schema: Here we define the key schema for our table. In DynamoDB, the key schema determines how data is organized.
- Provisioned Throughput: For local testing, we set low throughput values for cost efficiency.
Example Test Class with JUnit 5
import org.junit.jupiter.api.BeforeEach;
import org.junit.jupiter.api.Test;
import software.amazon.awssdk.services.dynamodb.DynamoDbClient;
import software.amazon.awssdk.services.dynamodb.model.*;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class DynamoDBIntegrationTest {
private DynamoDbClient dynamoDB;
@BeforeEach
void setUp() {
dynamoDB = DynamoDBClient.createClient();
DynamoDBTestHelper.createTable(dynamoDB);
}
@Test
void testPutItem() {
PutItemRequest putItemRequest = PutItemRequest.builder()
.tableName("TestTable")
.item(Map.of("Id", AttributeValue.builder().n("1").build()))
.build();
dynamoDB.putItem(putItemRequest);
// Fetching the item back
GetItemRequest getItemRequest = GetItemRequest.builder()
.tableName("TestTable")
.key(Map.of("Id", AttributeValue.builder().n("1").build()))
.build();
Map<String, AttributeValue> returnedItem = dynamoDB.getItem(getItemRequest).item();
assertEquals("1", returnedItem.get("Id").n());
}
}
Explanation of the Test Class
@BeforeEach
: This annotation ensures that we create a new table before each test, keeping our tests isolated.- Putting and Getting Item: We demonstrate how to insert an item and then retrieve it to verify it was correctly stored.
Challenges of Data Management
When performing tests, data management is crucial. You need to ensure that the database state is reset after each test to avoid unwanted side effects. Consider using transaction features or cleanup operations to clear out the table post-testing.
Cleanup Implementation
You might want to add a cleanup method to delete the table after tests:
@AfterEach
void tearDown() {
dynamoDB.deleteTable(DeleteTableRequest.builder().tableName("TestTable").build());
}
Mocking DynamoDB for Unit Testing
When you want to run unit tests that do not necessarily require a real database, you should consider using mocking. Libraries like Mockito can help with this.
Here’s an example:
import static org.mockito.Mockito.*;
public class MongoDBUnitTest {
private DynamoDbClient dynamoDBMock;
@BeforeEach
void setUp() {
dynamoDBMock = mock(DynamoDbClient.class);
}
@Test
void testMockPutItem() {
PutItemRequest putItemRequest = PutItemRequest.builder()
.tableName("TestTable")
.item(Map.of("Id", AttributeValue.builder().n("1").build()))
.build();
doNothing().when(dynamoDBMock).putItem(putItemRequest);
// Your unit test logic here, no network call is made to DynamoDB
}
}
Why Mock?
Mocking allows you to test your business logic without relying on actual database interactions. It speeds up tests and makes them more reliable.
Final Thoughts
Testing with JUnit 5 in a DynamoDB context presents specific challenges, but with the right tools and strategies, these challenges can be effectively managed. By using LocalStack, carefully structuring your tests, and considering mocks where appropriate, you can develop a robust testing framework for your applications.
For more insights on AWS DynamoDB testing strategies, check out AWS Testing Best Practices and JUnit 5 User Guide.
By following these guidelines and strategies, you'll find that your JUnit 5 testing with DynamoDB becomes not just manageable, but enjoyable as well. Happy coding!
Checkout our other articles