Troubleshooting Spring Remoting: Common Pitfalls to Avoid
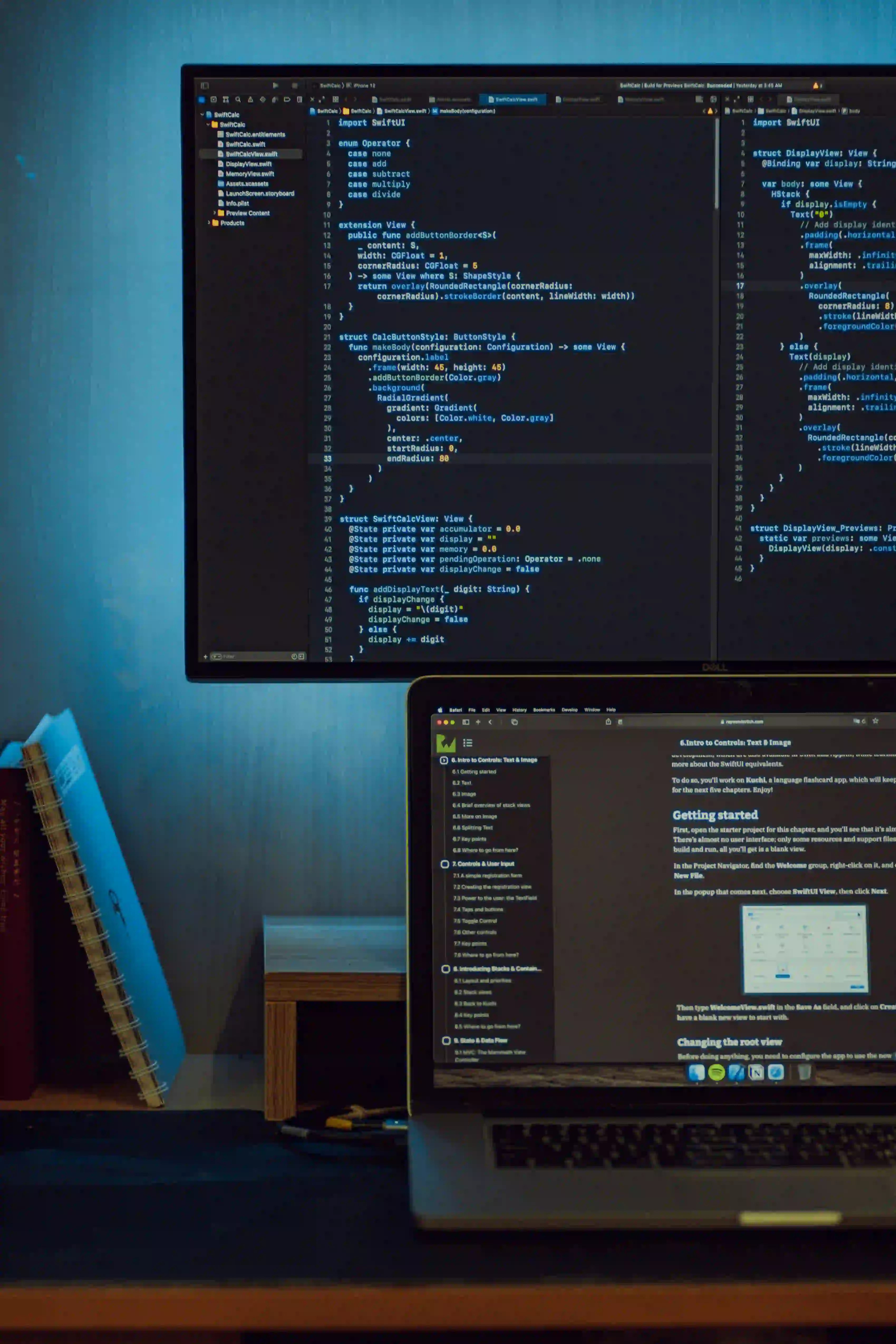
Troubleshooting Spring Remoting: Common Pitfalls to Avoid
Spring Remoting is a powerful feature of the Spring Framework that enables seamless communication between client and server applications. However, developers often encounter pitfalls that can lead to frustrating issues and prolonged debugging sessions. In this blog post, we will explore common pitfalls associated with Spring Remoting, how to troubleshoot them effectively, and best practices to avoid these issues altogether.
Understanding Spring Remoting
Before diving into troubleshooting, let's take a moment to understand what Spring Remoting is. Spring Remoting provides mechanisms for invoking services over remote interfaces. It supports various protocols like RMI (Remote Method Invocation), Hessian, and Burlap, making it flexible for different architectures.
Why Use Spring Remoting?
- Decoupling: It allows for a clear separation between client and server components.
- Interoperability: Supports multiple protocols, making it easy to interact with different applications.
- Ease of Use: Integrates smoothly within the Spring ecosystem.
Common Pitfalls in Spring Remoting
1. Misconfigured Service Beans
One of the first hurdles is misconfiguration of the service beans. This is often due to the service not being properly declared in the application context.
Example of Incorrect Configuration
<!-- Incorrect service bean configuration -->
<bean id="myService" class="com.example.MyService" />
Correction
Ensure that the bean is correctly defined and the service port is specified, like this:
<bean id="myService" class="com.example.MyService">
<property name="myDependency" ref="myDependencyBean"/>
</bean>
Why It Matters
If your service isn't correctly defined, Spring won't be able to locate it, leading to a BeanNotFoundException
.
2. Network Issues
Network issues can be a major roadblock when working with Spring Remoting. This includes firewall restrictions, incorrect hostnames, or port settings that block communication.
Troubleshooting Tips
- Ping the server: Check network connectivity.
- Verify Ports: Ensure the correct ports are open in firewall settings.
- DNS Resolution: Confirm that the hostname resolves correctly.
3. Serialization Pitfalls
Serialization is crucial for remote communication. If a class is not serializable properly, you'll receive a java.io.NotSerializableException
.
Example of a Non-Serializable Class
public class NonSerializableClass {
private final String data = "Example Data";
// Transient fields won't be serialized
private transient String transientData;
}
Correction for Serialization
Make sure your classes implement Serializable
and handle transient fields properly.
public class SerializableClass implements Serializable {
private static final long serialVersionUID = 1L;
private String data;
// Make sure to define transient fields if not needed in serialization
private transient String transientData;
}
4. Classpath Issues
Another common issue is classpath mismatches. If your client and server applications are using different versions of libraries, this can lead to runtime errors.
Resolution Steps
- Dependency Version Alignment: Use a build tool like Maven or Gradle to manage versions consistently across projects.
- Check the ClassLoader: Ensure that the expected classes are being loaded by the correct ClassLoader.
5. No Service Interface
Spring Remoting requires an interface that defines the remote service. If you are invoking a service method without an interface, you will encounter runtime exceptions.
Correct Implementation
Define a service interface:
public interface MyService {
String processData(String data);
}
Then implement it:
public class MyServiceImpl implements MyService {
public String processData(String data) {
return "Processed: " + data;
}
}
Using an interface ensures type safety and allows the Spring framework to handle remote method calls effectively.
6. Version Compatibility Issues
Whenever you're upgrading Spring or associated dependencies, version compatibility can become a major concern. A minor version change can introduce breaking changes.
Actionable Advice
- Check Release Notes: Always read the release notes for changes in compatibility.
- Testing on Staging: Before deploying your updated application, test it extensively in a staging environment to catch any issues early.
7. Error Handling
In Spring Remoting, error handling can often be overlooked. If a remote call fails, you want to ensure that the client gets an understandable error message.
Implementation of Custom Exceptions
Create custom exceptions for better clarity:
public class MyCustomException extends RuntimeException {
public MyCustomException(String message) {
super(message);
}
}
In your service implementation, throw this exception:
public String processData(String data) {
if (data == null) {
throw new MyCustomException("Data cannot be null");
}
return "Processed: " + data;
}
8. Using the Wrong Protocol
Spring supports various remoting protocols, and using the wrong one could lead to errors or performance issues.
Choose Wisely
- RMI: Good for Java-to-Java communication but makes your services JVM-dependent.
- Hessian or Burlap: More suitable for cross-language communication.
Key Takeaways
Troubleshooting Spring Remoting can seem daunting, but by understanding these common pitfalls and their solutions, you can streamline your development process. Stay aware of service configuration, networking issues, serialization requirements, and compatibility for a smoother experience.
For further reading on Spring Remoting, you might want to check out the official Spring Documentation.
Make sure to keep best practices at the forefront, and always test your applications before deploying. Happy coding!