Avoiding Data Loss: Properly Shutting Down Java Containers
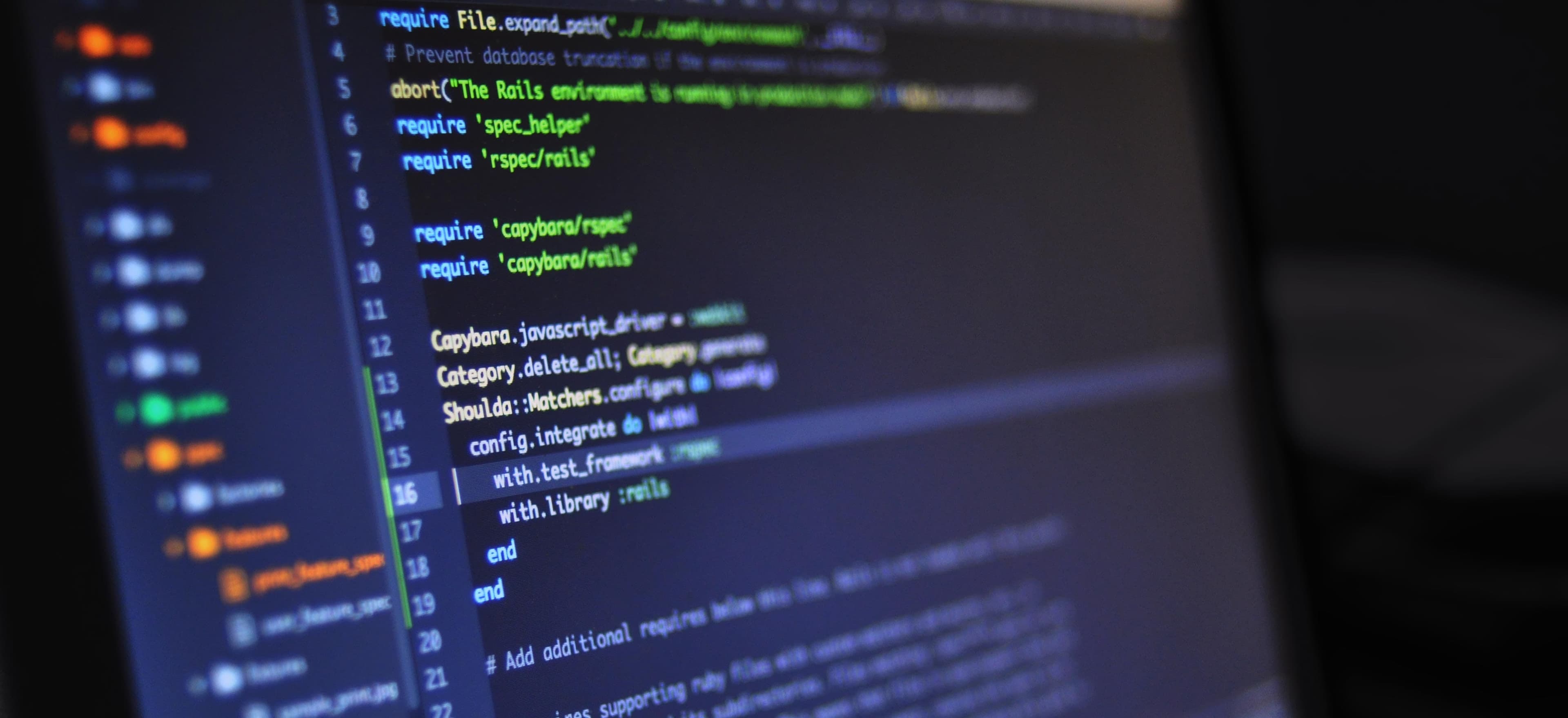
- Published on
Avoiding Data Loss: Properly Shutting Down Java Containers
Modern applications are increasingly constructed as microservices and deployed in containers, predominantly using platforms like Docker. While these technologies promise easy scalability and simplified deployments, improperly shutting down Java containers can lead to significant data loss. In this blog post, we will explore the best practices for effectively shutting down Java containers, ensuring your application’s integrity and preventing unwanted data loss.
Understanding Container Shutdown
When a container is shut down, multiple processes and services inside the container must be terminated. This can include:
- Database connections
- In-memory caches
- Background tasks
If these services are not stopped gracefully, it can result in data not being persisted correctly or transactions being lost.
The shutdown process typically involves:
- (1) Signaling to the application that it needs to shut down
- (2) Allowing the application to complete any pending requests
- (3) Finally, shutting down all services and terminating the container
Let’s delve into each of these steps.
Signaling the Application
In Java containers (like those using Spring Boot), you can design your application to respond to shutdown signals. The standard way to signal Java applications in a containerized environment is through the SIGTERM signal.
import org.springframework.boot.CommandLineRunner;
import org.springframework.stereotype.Component;
@Component
public class ShutdownHook implements CommandLineRunner {
@Override
public void run(String... args) throws Exception {
Runtime.getRuntime().addShutdownHook(new Thread(() -> {
// Custom cleanup logic
System.out.println("Shutting down gracefully...");
// Example: Close database connections
closeDatabaseConnections();
}));
}
private void closeDatabaseConnections() {
// Close all your database connections here
System.out.println("Closing database connections...");
}
}
Why is this important?
By adding a shutdown hook as shown above, you're ensuring that your application has a chance to execute any necessary cleanup operations before it completely terminates. This allows you to handle resource management and avoid abrupt disconnections to external services, keeping data integrity intact.
Allowing Time for Current Operations
Once your application acknowledges the shutdown signal, it’s crucial not to immediately terminate; rather, allow ongoing operations to complete. This might involve waiting for in-flight requests to finish.
In a Spring Boot application, this can be managed automatically, but for more advanced use cases, you may want something like:
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class YourController {
private volatile boolean shuttingDown = false;
@PostMapping("/process")
public String processRequest() {
if (shuttingDown) {
return "Server is shutting down, please try again later.";
}
// Process your request here
return "Processing your request.";
}
public void initiateShutdown() {
shuttingDown = true;
// Your other shutdown logic here
}
}
Why this approach?
The volatile variable shuttingDown
ensures thread-safety and informs your application about the ongoing shutdown process. This way, new incoming requests won't be processed while the application is in the shutdown phase, effectively preventing data loss.
Close Resources and Services
When it comes to Java applications, resource management is paramount. Resources such as database connections, threads, and file handles can be lost if not properly managed during a shutdown.
public void closeServices() {
// Ensure each service is closed properly
try {
databaseService.close();
cacheService.clear();
// Other necessary cleanup
} catch (Exception e) {
e.printStackTrace();
}
}
Importance of Cleanup
Closing the database connection and other services ensures that:
- Data transactions are properly committed or rolled back
- Resource leaks are avoided
- Future operations starting with a clean state
Configuring Docker for Graceful Shutdowns
To take full advantage of your Java application's shutdown capabilities, configure your Docker container for graceful termination. Here's how you can set the timeout for Docker:
docker run --name your_container \
--stop-timeout 30 \
your_image
Why configure Docker?
The --stop-timeout
option allows Docker to wait for the specified duration (in seconds) for the container to exit gracefully before forcefully terminating it. This gives your Java application enough time to complete its shutdown hooks and cleanup tasks.
Utilize Readiness and Liveness Probes
To further enhance reliability, utilize Kubernetes readiness and liveness probes. These ensure that your application can seamlessly handle traffic during shutdown and avoid serving requests from a container that is shutting down.
Example readiness probe configuration for Kubernetes might look like this:
apiVersion: apps/v1
kind: Deployment
metadata:
name: your-app
spec:
template:
spec:
containers:
- name: your-app
image: your-image
readinessProbe:
httpGet:
path: /actuator/health
port: 8080
initialDelaySeconds: 5
periodSeconds: 10
Why implement these probes?
Readiness probes prevent traffic from being directed to instances that are in the process of shutting down. Thus, requests are only served by healthy instances, which protects data integrity and improves user experience.
Bringing It All Together
Preventing data loss during Java container shutdown involves a combination of techniques, including signaling the application, allowing existing operations to finish, closing resources properly, configuring your Docker container, and utilizing Kubernetes probes.
As application infrastructures evolve, understanding and implementing proper shutdown strategies ensures that your application is robust, reliable, and ready to handle failovers gracefully.
For more information, consider exploring these resources:
By following the best practices highlighted in this blog post, you not only strengthen your application’s resilience but also provide a better experience for your users. Happy coding!