Overcoming Challenges in Auditing Spring Data MongoDB
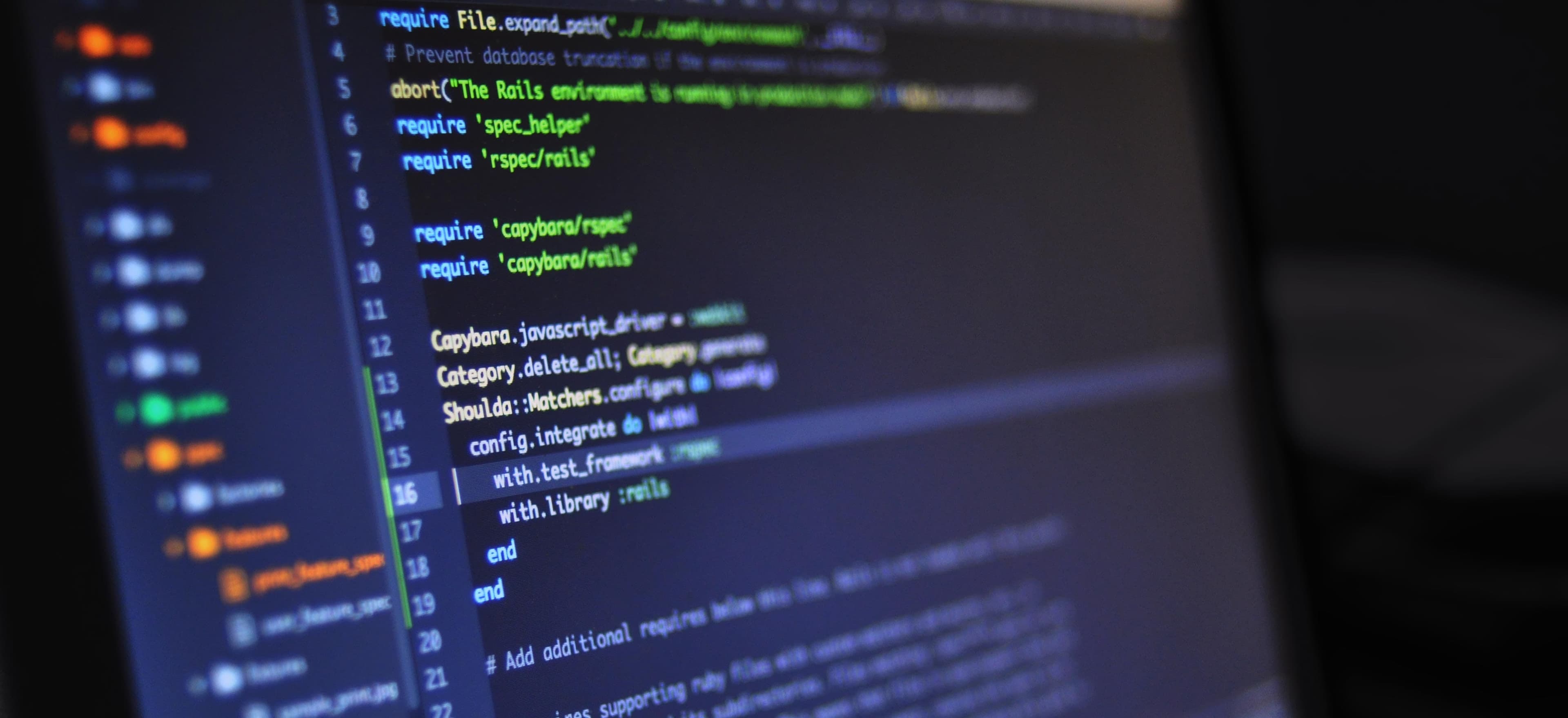
- Published on
Overcoming Challenges in Auditing Spring Data MongoDB
In today’s fast-paced and data-driven world, managing information effectively while ensuring that data integrity is maintained is paramount. Spring Data MongoDB provides a powerful platform for working with MongoDB within a Spring application. However, like any technology, it comes with its own set of challenges. One common area of concern is auditing changes to data in your MongoDB collections. In this post, we will explore the intricacies of auditing in Spring Data MongoDB and how to overcome these challenges effectively.
Understanding Auditing
Auditing involves tracking and storing changes made to your data. This is especially important for applications requiring accountability, compliance with regulations, or need to maintain a historical record of data modifications. In a typical auditing scenario, you may want to track:
- Who made the change?
- What data was changed?
- When the change occurred?
- Why the change was made?
In a Spring Data MongoDB context, leveraging the built-in auditing capabilities can help streamline this process.
Getting Started with Spring Data MongoDB
Before diving into auditing, ensure you have your Spring Data MongoDB application up and running. Here’s how to set up a basic Spring Boot application using MongoDB.
Step 1: Dependency Management
Add the necessary dependencies in your pom.xml
file:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-mongodb</artifactId>
</dependency>
Step 2: Configuration
Configure your MongoDB connection in the application.properties
file:
spring.data.mongodb.uri=mongodb://localhost:27017/mydatabase
Setting Up Auditing in Spring Data MongoDB
Spring Data offers a straight-forward way to implement auditing. Here’s how you can enable auditing capabilities across your application.
Step 3: Enable Auditing
Enable auditing in your main application class:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.data.jpa.repository.config.EnableJpaAuditing;
import org.springframework.data.mongodb.config.EnableMongoAuditing;
@SpringBootApplication
@EnableMongoAuditing
public class MyApplication {
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
}
}
Step 4: Create Auditable Entity
Define your auditable entity with fields you want to track:
import org.springframework.data.annotation.CreatedBy;
import org.springframework.data.annotation.CreatedDate;
import org.springframework.data.annotation.LastModifiedBy;
import org.springframework.data.annotation.LastModifiedDate;
import org.springframework.data.mongodb.core.mapping.Document;
import java.time.LocalDateTime;
@Document
public class User {
private String id;
@CreatedDate
private LocalDateTime createdDate;
@LastModifiedDate
private LocalDateTime lastModifiedDate;
@CreatedBy
private String createdBy;
@LastModifiedBy
private String lastModifiedBy;
// Getters and Setters
}
Explanation
- @Document: Marks the class as a MongoDB document.
- @CreatedDate / @LastModifiedDate: Automatically filled with the date/time when the entity was created or modified.
- @CreatedBy / @LastModifiedBy: Automatically filled using the security context for user tracking.
Monitoring Challenges in Auditing
Challenge 1: User Context Management
When working in multi-user environments, correctly capturing the user who makes a change can be tricky. If not correctly configured, you may end up auditing the wrong user or no user at all.
Solution
Utilize a custom AuditorAware
implementation. Here's how:
import org.springframework.data.domain.AuditorAware;
import org.springframework.stereotype.Component;
import java.util.Optional;
@Component
public class SpringSecurityAuditorAware implements AuditorAware<String> {
@Override
public Optional<String> getCurrentAuditor() {
// Fetch the username from your security context or session
return Optional.of("system"); // Replace with actual logic
}
}
Challenge 2: Handling Complex Entities
When auditing complex entities with nested objects or arrays, ensuring that all relevant fields are captured can become cumbersome.
Solution
Instead of tracking all fields manually, use a library such as MongoDB Change Streams. Change streams allow your application to receive real-time notifications when data changes occur.
Step 5: Implementing Change Streams
Here's an example of how to implement change streams:
import com.mongodb.client.MongoCollection;
import org.bson.Document;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.mongodb.core.MongoTemplate;
import org.springframework.stereotype.Service;
@Service
public class AuditService {
@Autowired
private MongoTemplate mongoTemplate;
public void monitorChanges() {
MongoCollection<Document> collection = mongoTemplate.getCollection("user");
collection.watch().forEach(change -> {
System.out.println("Detected change: " + change.toString());
});
}
}
Addressing Audit Log Management
Challenge 3: Volume of Audit Logs
As your application grows, the volume of audit logs can rapidly increase, potentially causing performance issues and high storage costs.
Solution
Consider archiving or pruning older logs at regular intervals. This can be managed through scheduled tasks or external tools.
import org.springframework.scheduling.annotation.Scheduled;
import org.springframework.stereotype.Component;
@Component
public class AuditLogScheduler {
@Scheduled(cron = "0 0 0 * * ?") // Every midnight
public void pruneOldLogs() {
// Implement log pruning logic here
}
}
Challenge 4: Performance Impact
Audit logging can introduce latency if not handled efficiently, especially in high-throughput applications.
Solution
Batch your audit log writes. Instead of writing each log immediately, store them in-memory and write them out in batches to reduce overhead.
The Bottom Line
Auditing in Spring Data MongoDB is essential for maintaining the integrity and accountability of your data. Though challenges such as user context management, complex entity handling, log volume, and performance impact exist, they can be effectively managed through proper configuration and best practices.
For more insights on Spring Data MongoDB and auditing, check out the official Spring Data MongoDB documentation. Implement these strategies within your application and ensure a robust auditing mechanism that stands the test of time.
Feel free to share your thoughts and experiences in the comments. Your input can help others facing similar challenges. Remember, every solution starts with understanding the problem at hand! Happy coding!