How to Efficiently Find the Minimum Element in a Java Array
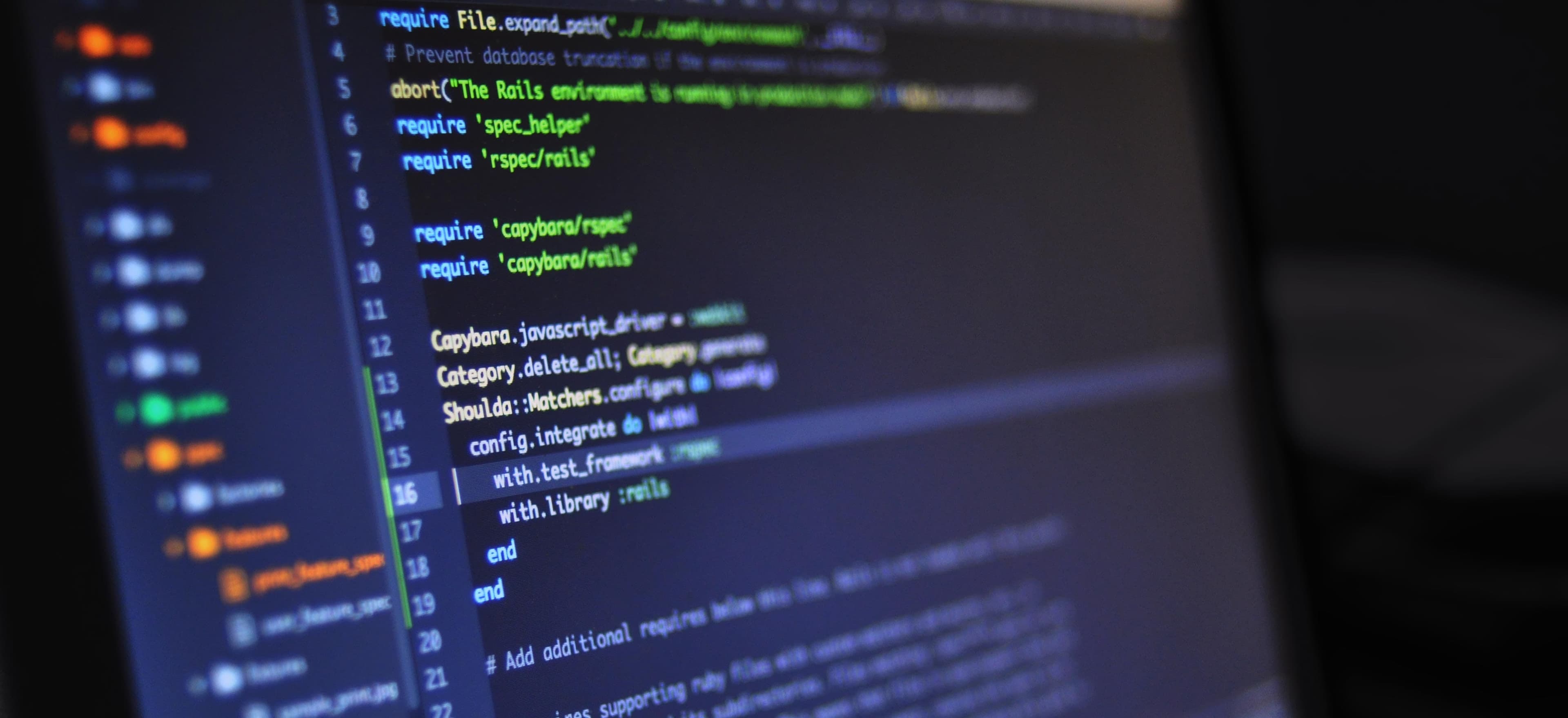
- Published on
How to Efficiently Find the Minimum Element in a Java Array
When working with arrays in Java, one common task is finding the minimum element. This can seem straightforward, but as array sizes grow, the efficiency of your approach becomes a crucial factor. In this blog, we will explore various methods for finding the minimum element in a Java array, detailing their implementations and performance considerations.
Understanding the Problem
Before getting into the code, it’s essential first to understand why efficiently locating a minimum element matters. For large datasets, the algorithm you choose can significantly impact your program’s performance.
Common Use Cases
- Data Analysis: When processing large datasets, quickly finding minimum values can help in statistical calculations.
- Sorting Algorithms: Many sorting algorithms rely on finding minimum values repeatedly.
- Dynamic Applications: In games or real-time applications, minimum values might be needed continuously and efficiently.
A Simple Approach: Iteration
The most straightforward method of finding the minimum element is to iterate through the array. This O(n) algorithm involves checking each element and tracking the smallest value found.
Code Example
public class MinElementFinder {
public static int findMin(int[] arr) {
// Assume the first element is the minimum
int min = arr[0];
// Iterate over the array starting from the second element
for (int i = 1; i < arr.length; i++) {
if (arr[i] < min) {
min = arr[i]; // Update min if a smaller value is found
}
}
return min;
}
public static void main(String[] args) {
int[] numbers = {3, 5, 2, 9, 1};
int minimum = findMin(numbers);
System.out.println("The minimum element is: " + minimum); // Output: 1
}
}
Why This Works
This approach is effective because every element in the array is checked exactly once, ensuring a linear time complexity of O(n). It is simple and works well unless you have specific constraints on performance.
Enhanced Approach: Using Streams (Java 8 and Above)
With Java 8, the introduction of Streams allowed for a more functional programming approach to handle operations on collections, including arrays. Using streams, we can find the minimum element in a more concise manner.
Code Example
import java.util.Arrays;
public class MinElementFinder {
public static void main(String[] args) {
int[] numbers = {3, 5, 2, 9, 1};
// Convert array to stream and find minimum
int minimum = Arrays.stream(numbers)
.min()
.orElseThrow(() -> new IllegalArgumentException("Array is empty"));
System.out.println("The minimum element is: " + minimum); // Output: 1
}
}
Why Use Streams
Using streams is both expressive and concise. It reads well and can integrate seamlessly with other operations, thanks to Java's functional programming capabilities. However, under the hood, the stream also traverses the array, maintaining an average time complexity of O(n).
Recursive Approach
While recursion may not be the most efficient for this specific problem, it can be an interesting alternative worth considering for educational purposes. Recursion could be utilized to divide the problem into smaller subproblems.
Code Example
public class MinElementFinder {
public static int findMinRecursively(int[] arr, int index) {
// Base case: last element
if (index == arr.length - 1) {
return arr[index];
}
// Recursive case: find the minimum of the rest of the array
int minInRest = findMinRecursively(arr, index + 1);
// Return the minimum between current and rest
return Math.min(arr[index], minInRest);
}
public static void main(String[] args) {
int[] numbers = {3, 5, 2, 9, 1};
int minimum = findMinRecursively(numbers, 0);
System.out.println("The minimum element is: " + minimum); // Output: 1
}
}
Why Recursion Here?
While this recursive approach offers an elegant solution, note that it incurs additional overhead due to stack frame usage. This adds O(n) space complexity due to the depth of function calls, rendering it less efficient than iterative methods for large arrays.
When to Optimize Further
If you are dealing with large arrays where performance is critical, you may need to optimize beyond just finding the minimum. Consider the following strategies:
-
Parallel Processing: If the array is extremely large and your hardware supports multi-threading, you could divide the array into chunks and use multiple threads to find partial minimums before converging on the final minimum.
-
Data Structures: Depending on your use case, maintaining a data structure that supports dynamic insertion and deletion while keeping track of the minimum might be more effective than repeatedly scanning an array.
Final Thoughts
In summary, finding the minimum element in a Java array can be accomplished through various methods, each with its trade-offs. Here’s a quick recap of what we discussed:
- Iterative Approach: Simple and effective for most scenarios with O(n) time complexity.
- Streams: A modern, concise solution, still O(n) but more readable.
- Recursive Approach: An interesting method, albeit less efficient due to stack overhead.
For more information on efficient algorithms in Java, you can check the GeeksforGeeks article on sorting algorithms or the Java Documentation for a comprehensive guide on array processing.
By understanding the strengths and weaknesses of each approach, you can better decide which method to use for finding minimum elements based on your application's requirements. Happy coding!
Checkout our other articles