Common Logging Mistakes That Can Sink Your Application
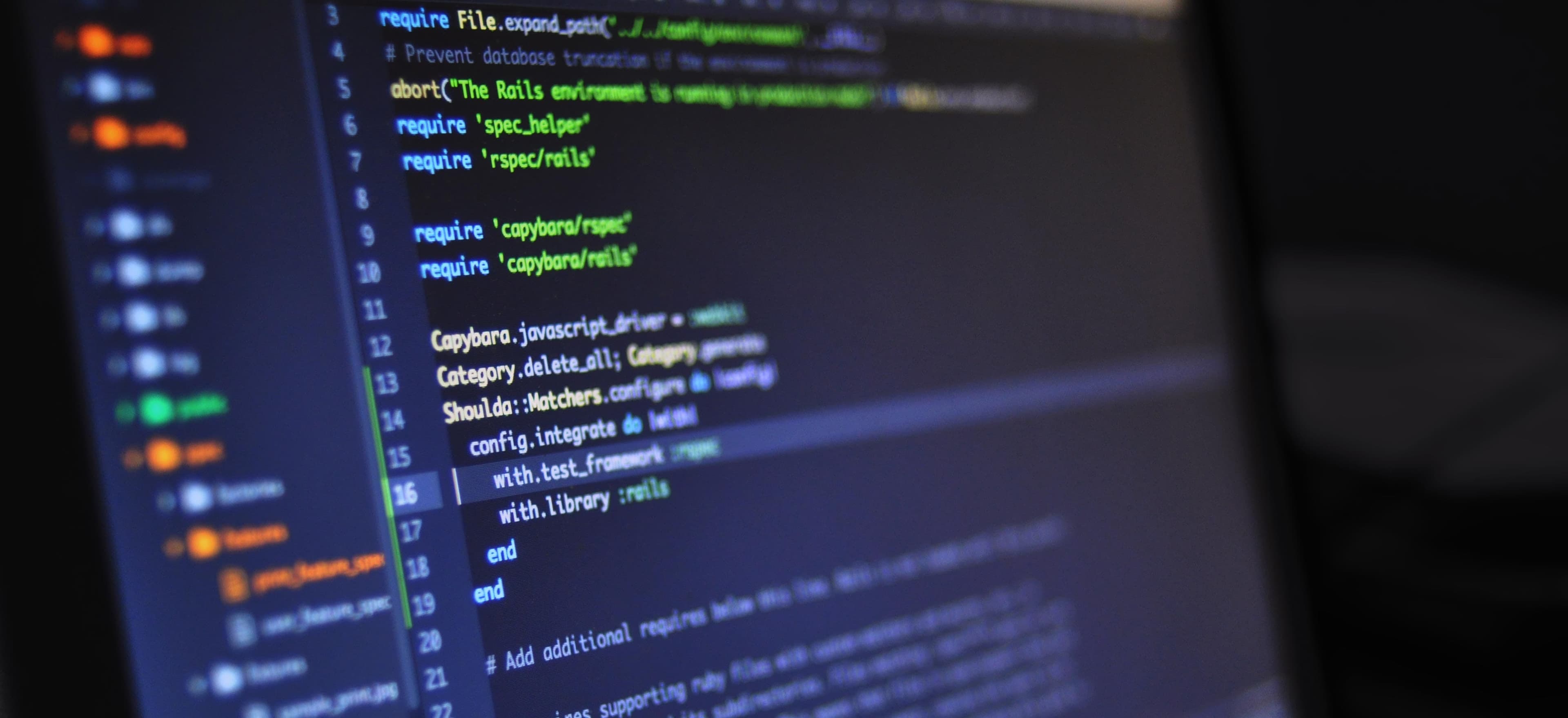
- Published on
Common Logging Mistakes That Can Sink Your Application
Logging is a critical aspect of application development, yet it is often overlooked or poorly implemented. It serves as both a diagnostic tool and an essential component for monitoring application health. However, there are common logging mistakes that can significantly hinder your application's performance and reliability. This article will delve into these pitfalls, providing clear examples and solutions to help you enhance your logging practices.
1. Logging Too Much or Too Little
The Problem:
One of the most significant mistakes developers make is failing to find the right balance between logging verbosity. Excessive logging fills storage space, can slow down your application, and makes it difficult to sift through logs when troubleshooting issues. On the other hand, inadequate logging can leave you in the dark when it’s time to debug.
The Solution:
Adopt a logging framework that allows for adjustable log levels (e.g., DEBUG, INFO, WARN, ERROR). Adjust your log levels based on the environment (development vs. production).
Example:
Using SLF4J with Logback in a Java application, here is how you can define logging levels in your logback.xml
file:
<configuration>
<appender name="STDOUT" class="ch.qos.logback.core.ConsoleAppender">
<encoder>
<pattern>%d{yyyy-MM-dd HH:mm:ss} - %msg%n</pattern>
</encoder>
</appender>
<logger name="com.myapp" level="INFO"/>
<logger name="com.myapp.debug" level="DEBUG"/>
<root level="ERROR">
<appender-ref ref="STDOUT"/>
</root>
</configuration>
Why This Matters:
By setting appropriate log levels, you can control the amount of data logged in different environments. It allows for critical tracking in production without overwhelming logs or impacting performance.
2. Not Including Context in Log Messages
The Problem:
Context is vital when it comes to debugging. Logs that lack context can confuse developers and make it difficult to understand the circumstances surrounding issues. For example, logging "User failed to log in" offers minimal insight without specific details.
The Solution:
Always include relevant context in your log messages. This may include user IDs, session information, and input parameters.
Example:
Instead of:
logger.error("User failed to log in");
Use:
logger.error("User [{}] failed to log in at [{}]", userId, LocalDateTime.now());
Why This Matters:
Adding context to logs enhances their usefulness during debugging. It allows developers to pinpoint where things went wrong and why, making resolution quicker and more accurate.
3. Ignoring Exception Logging
The Problem:
Java applications often throw exceptions, and failing to log these exceptions properly can lead to an incomplete understanding of application behavior. Ignored exceptions can cause silent failures, leading to a degraded user experience.
The Solution:
Log exceptions with stack traces. This will provide insight into where the error occurred in the code and help diagnose issues effectively.
Example:
Instead of logging only the message:
logger.error("Something went wrong");
Use:
catch (Exception e) {
logger.error("Error processing request: {}", e.getMessage(), e);
}
Why This Matters:
Capturing the full stack trace helps in understanding the context of an error. It saves critical time during the debugging process.
4. Hardcoding Log Messages
The Problem:
Hardcoding log messages can be tempting, but it diminishes the flexibility of your logging approach. If you need to change or translate messages, you may face significant challenges or overlook necessary updates.
The Solution:
Make use of constants or a dedicated logging message file. This approach allows for easier management and localization of log messages.
Example:
Instead of this:
logger.info("Processing user input failed");
Create a messages file or use constants:
public static final String USER_INPUT_FAILED = "Processing user input failed";
logger.info(USER_INPUT_FAILED);
Why This Matters:
By centralizing log messages, you gain better control over the logging messages used throughout your application, facilitating potential updates and translations.
5. Unoptimized Log Storage
The Problem:
Storing logs inefficiently can take up large amounts of disk space and complicate access. Many developers underestimate the importance of log management.
The Solution:
Implement log rotation and archiving strategies. This helps manage log volume and provides a timeline for the logging history.
Example:
Using logback for log rotation in your logback.xml
:
<appender name="FILE" class="ch.qos.logback.core.rolling.RollingFileAppender">
<file>myapp.log</file>
<rollingPolicy class="ch.qos.logback.core.rolling.TimeBasedRollingPolicy">
<fileNamePattern>myapp.%d{yyyy-MM-dd}.log</fileNamePattern>
<maxHistory>30</maxHistory>
</rollingPolicy>
<encoder>
<pattern>%d{yyyy-MM-dd HH:mm:ss} - %msg%n</pattern>
</encoder>
</appender>
Why This Matters:
Setting up log rotation prevents logs from consuming excessive space and allows for more manageable data retention.
A Final Look
Effective logging is crucial for diagnosing issues and monitoring the health of your application. By avoiding these common mistakes and implementing best practices, you can enhance your application’s performance and reliability.
Motivated by best practices, you can ensure your application is not just functional but maintainable. Remember to strike a balance in logging, include essential context, and expertly manage your logs. For more insights into logging frameworks and strategies, check out the official SLF4J documentation and Logback documentation.
By following these guidelines, you’ll leverage logging not just as a tool but as a treasure trove of insights into your application’s operation. Happy logging!
Checkout our other articles