Real-Time Tweet Indexing: Overcoming Common Challenges
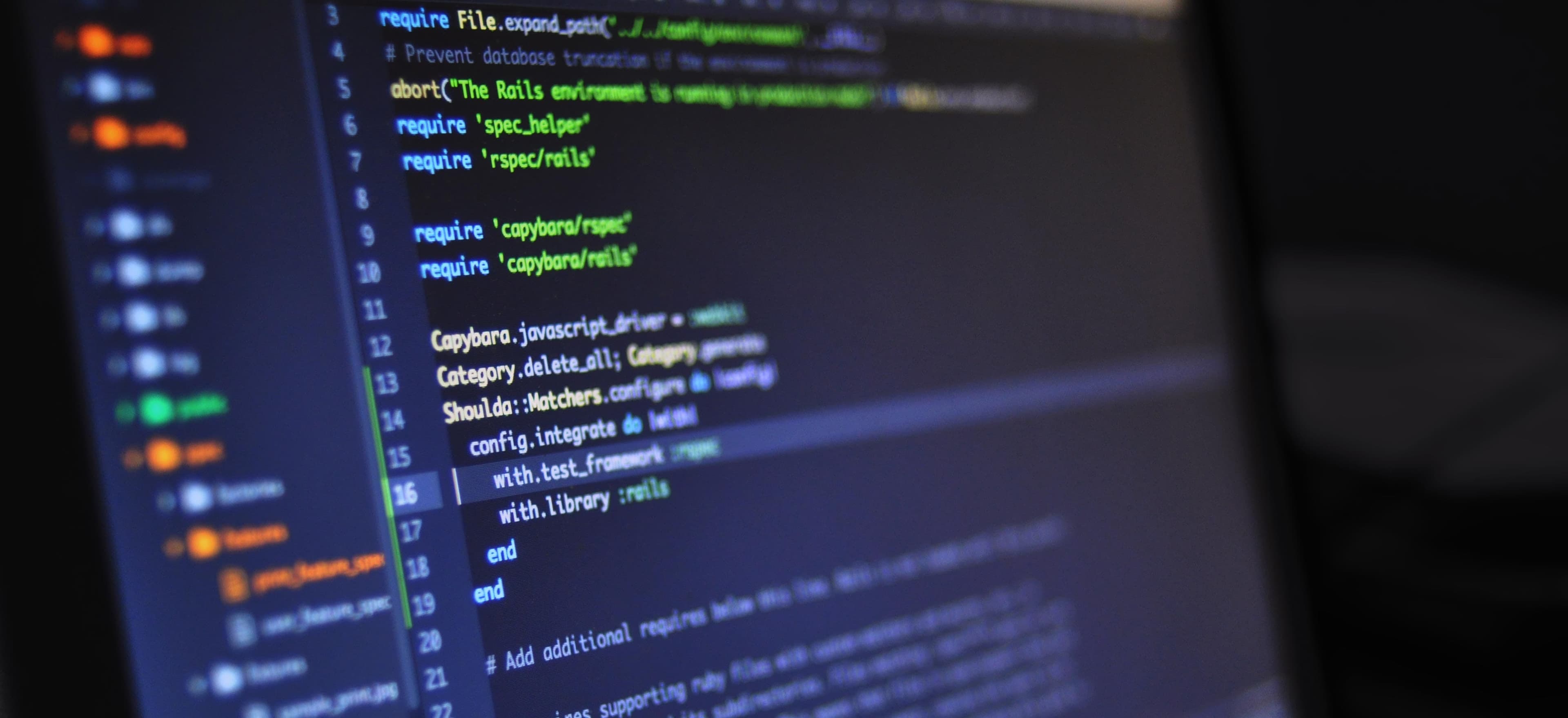
- Published on
Real-Time Tweet Indexing: Overcoming Common Challenges
In today's fast-paced digital landscape, social media platforms like Twitter generate massive volumes of data in real-time. With millions of tweets sent every day, efficiently indexing this information presents unique challenges. This blog will delve into the complexities of real-time tweet indexing and provide practical solutions to overcome common hurdles faced by developers and data engineers.
Understanding Real-Time Tweet Indexing
Tweet indexing refers to the process of organizing and storing tweets in a way that allows for efficient searching and retrieval. In real-time, this means capturing tweets as they are created, processing the data, storing it, and making it readily available for querying. The goal is to ensure that users or applications can access the latest tweets quickly and efficiently.
Challenges in Real-Time Tweet Indexing
1. Volume and Velocity of Data
With Twitter's API, every second brings a deluge of tweets. The sheer volume of data can overwhelm traditional indexing mechanisms. Therefore, scalable solutions are imperative.
Solution: Leverage Stream Processing
Using stream processing frameworks like Apache Kafka and Apache Flink can help you manage large streams of data. These tools allow real-time processing and are designed to scale horizontally, thus accommodating the influx of tweets.
Example: Below is a simple Kafka producer example in Java that sends tweets to a Kafka topic.
import org.apache.kafka.clients.producer.KafkaProducer;
import org.apache.kafka.clients.producer.ProducerRecord;
import java.util.Properties;
public class TweetProducer {
public static void main(String[] args) {
// Configure the producer
Properties properties = new Properties();
properties.put("bootstrap.servers", "localhost:9092");
properties.put("key.serializer", "org.apache.kafka.common.serialization.StringSerializer");
properties.put("value.serializer", "org.apache.kafka.common.serialization.StringSerializer");
KafkaProducer<String, String> producer = new KafkaProducer<>(properties);
// Sample tweet
String tweet = "Hello, Twitter World!";
// Send tweet to specific topic
producer.send(new ProducerRecord<>("tweets", null, tweet));
producer.close();
}
}
Why: Using a Kafka producer allows you to handle large streams of data efficiently. The message is published to a specified topic that can be processed later.
2. Data Structure and Storage
The next challenge is how to represent and store tweet data effectively. Tweets contain various attributes such as user information, timestamps, and content.
Solution: Use NoSQL Databases
NoSQL databases like MongoDB or Elasticsearch can store semi-structured data. They allow for rapid indexing and retrieval, accommodating changes in data structure without significant overhead.
Example: Here is a simple MongoDB insertion logic for a tweet.
import com.mongodb.MongoClient;
import com.mongodb.client.MongoCollection;
import com.mongodb.client.MongoDatabase;
import org.bson.Document;
public class TweetStorage {
public static void main(String[] args) {
MongoClient mongoClient = new MongoClient("localhost", 27017);
MongoDatabase database = mongoClient.getDatabase("twitter_db");
MongoCollection<Document> collection = database.getCollection("tweets");
// Create Document representing a tweet
Document tweet = new Document("user", "user123")
.append("content", "Hello, World!")
.append("timestamp", System.currentTimeMillis());
// Insert tweet into MongoDB
collection.insertOne(tweet);
mongoClient.close();
}
}
Why: MongoDB's flexible schema allows you to adapt to the dynamic nature of tweet data while ensuring quick read and write operations.
3. Latency in Data Processing
Real-time applications require low-latency processing. If tweets take too long to index, users may miss critical updates.
Solution: Employ In-Memory Data Grids
Using technologies like Apache Ignite or Redis, you can implement an in-memory data grid that keeps data in memory for faster access.
Example: Here’s how you might use Redis to maintain a cache of recent tweets.
import redis.clients.jedis.Jedis;
public class TweetCache {
private static final String REDIS_HOST = "localhost";
private static final int REDIS_PORT = 6379;
public static void main(String[] args) {
Jedis jedis = new Jedis(REDIS_HOST, REDIS_PORT);
// Store the latest tweet in Redis
String tweet = "Hello, Real-Time World!";
jedis.lpush("latest_tweets", tweet);
// Retrieve latest tweet
String latestTweet = jedis.lpop("latest_tweets");
System.out.println("Latest Tweet: " + latestTweet);
jedis.close();
}
}
Why: Using Redis ensures low-latency access to frequently accessed data, thereby improving overall performance.
4. Handling Duplicate Tweets
In a real-time environment, duplicates may arise due to user retweets or repeated API calls. This can clutter your index and complicate searches.
Solution: Implement Deduplication Logic
Creating a unique identifier for each tweet or leveraging Twitter's ID can help you identify and filter duplicates before they are processed.
Example: Here is a simple method to check for duplicates using a HashSet.
import java.util.HashSet;
public class TweetDeduplicator {
private HashSet<String> seenTweets = new HashSet<>();
public boolean isDuplicate(String tweetId) {
// Return true if tweet has already been seen
return !seenTweets.add(tweetId);
}
}
Why: This deduplication approach avoids unnecessary storage and improves query performance by ensuring that only unique tweets are indexed.
5. Query Performance
As your indexed data grows, querying it efficiently can become a challenge. Users expect instant responses to their queries.
Solution: Optimize Index Structure
Using inverted indexes or designing specific indexes based on common queries can drastically improve retrieval times.
Example: In Elasticsearch, you can create an index with mappings tailored for efficient searching:
PUT /tweets
{
"mappings": {
"properties": {
"user": { "type": "keyword" },
"content": { "type": "text" },
"timestamp": { "type": "date" }
}
}
}
Why: This structured approach allows Elasticsearch to efficiently execute queries based on expected usage patterns.
The Closing Argument
Real-time tweet indexing is fraught with challenges, but with the right tools and approaches, these obstacles can be effectively managed. By leveraging stream processing frameworks, NoSQL databases, in-memory data grids, deduplication strategies, and optimized indexing structures, you can build a resilient and efficient tweet indexing system.
For further reading, consider exploring these additional resources:
By addressing the challenges of real-time tweet indexing, you position yourself to harness the power of social media data effectively, driving insights and value from ever-changing digital conversations.
Checkout our other articles