Mastering JVM GC: Common Performance Tuning Pitfalls
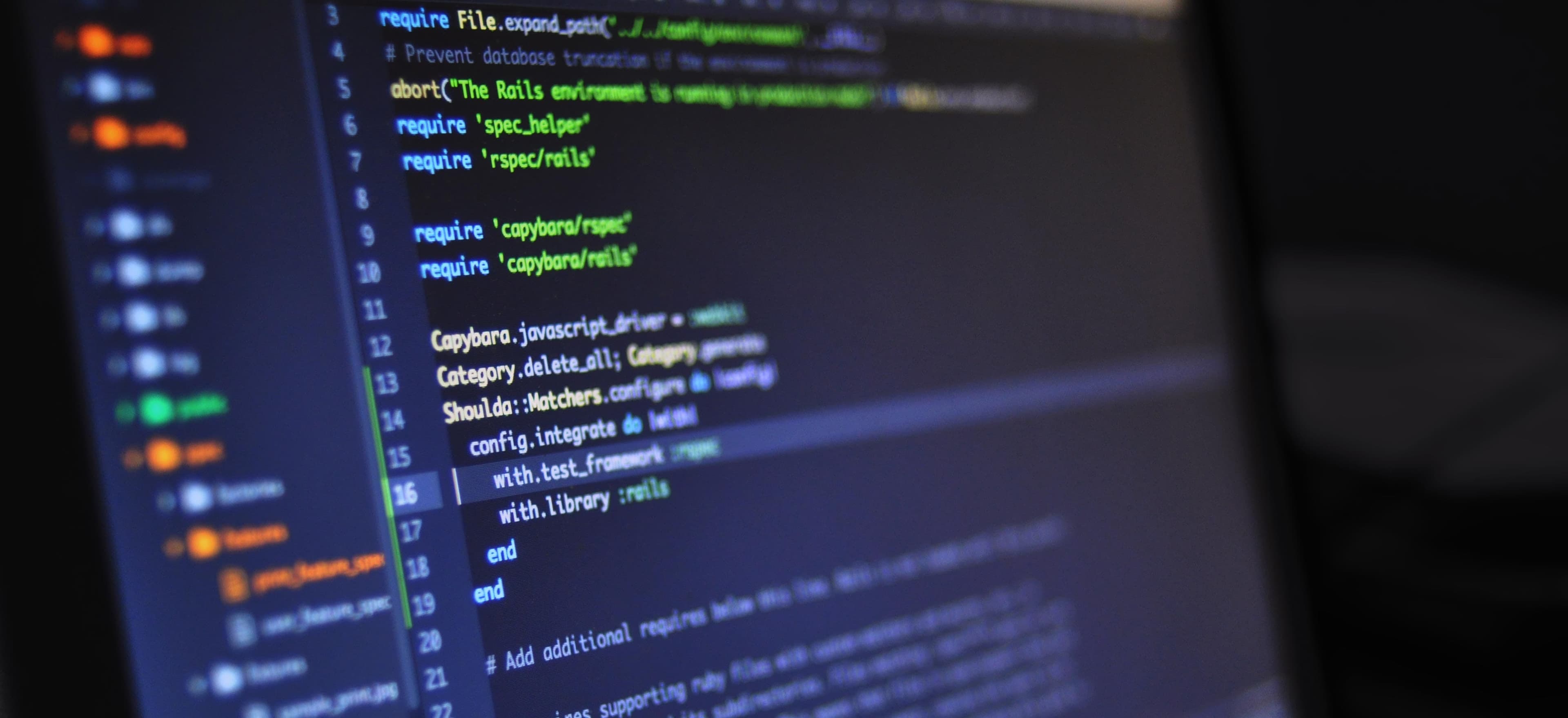
- Published on
Mastering JVM GC: Common Performance Tuning Pitfalls
Java Virtual Machine (JVM) Garbage Collection (GC) is a crucial aspect of Java development. It's designed to automate memory management, thus alleviating developers from the burden of manual memory allocation and deallocation. However, if not mastered, it can lead to performance bottlenecks in applications. In this blog post, we will delve into common pitfalls in JVM GC performance tuning, understand why they occur, and how to avoid them.
Understanding Garbage Collection
Garbage Collection is the process by which the JVM automatically identifies and removes objects that are no longer in use, freeing up memory resources. The JVM employs various algorithms for garbage collection, each with its strengths and weaknesses. For example, the G1 collector is designed for applications with large heaps and aims to minimize pause times, while the CMS (Concurrent Mark-Sweep) collector focuses on minimizing the time an application is paused during GC.
Why is GC Tuning Necessary?
While the JVM does a fantastic job of managing memory, applications with specific performance requirements may need fine-tuning to optimize GC operations. Without tuning, applications can suffer from long GC pause times, excessive memory consumption, and unpredictable performance.
Common Pitfalls in GC Tuning
- Overcomplicating the GC Parameters
One of the most common mistakes developers make is trying to tune multiple GC parameters simultaneously without a clear understanding of their implications. Every JVM flags can have diverse effects on your application.
Example: Setting JVM Options
-XX:+UseG1GC -Xms512m -Xmx4g -XX:MaxGCPauseMillis=200
Here’s what these options mean:
-XX:+UseG1GC
: Enables the G1 garbage collector.-Xms512m
: Sets the initial heap size to 512 MB.-Xmx4g
: Sets the maximum heap size to 4 GB.-XX:MaxGCPauseMillis=200
: Tries to limit pause times to 200 milliseconds.
Why: Each option interacts with others, and tuning them blindly can lead to unforeseen performance issues. Solutions should be approached methodically. Begin by changing one parameter at a time and monitor performance impacts.
- Neglecting the Impact of Object Lifetimes
Developers often overlook the significance of object lifetimes in GC tuning. Objects that are created and discarded frequently can cause more frequent GC cycles, leading to pauses.
Example: Understanding Memory Allocation
public class UserSession {
public UserSession() {
// Initialize session
}
}
If you frequently create and destroy UserSession
objects, the GC will have to work harder. Instead, consider reusing the objects if possible.
Why: Implementing object pooling can significantly reduce GC overhead by minimizing the number of objects created.
- Ignoring the Application's Workload Patterns
Many developers tune GC based on synthetic benchmarks rather than real application workloads. Performance under test conditions can vary significantly from actual usage.
Example: Using Profiling Tools
Utilize profiling tools, such as JVisualVM or YourKit, to observe the application's memory usage in a production-like environment.
Why: By understanding the workload patterns, you can make informed decisions about heap sizes and GC configurations that align with actual usage scenarios.
- Setting Heap Size Incorrectly
The size of the heap can significantly impact the frequency and efficiency of garbage collections. Setting the heap too small can lead to frequent collections, while setting it too large may result in longer GC pause times.
Example: Configuring Heap Sizes
java -Xms2g -Xmx4g -XX:+UseG1GC MyApp
Why: Find the optimal balance based on profiling data as mentioned earlier. It’s a good practice to start with the default settings and incrementally modify them based on observed performance metrics.
- Ignoring Generational Garbage Collection
The JVM GC is designed with a generational approach, segregating objects based on their lifetimes: young, old, and permanent generations. Many developers fail to leverage this feature effectively.
Example: Young Generation Tuning
-XX:NewSize=256m -XX:MaxNewSize=512m
The young generation handles short-lived objects and can be tuned separately.
Why: By optimizing young generation sizes and tuning minor GC collection frequencies, you can significantly speed up the reclaiming of memory for many short-lived objects.
- Not Monitoring GC Activity
Failing to monitor GC activity can make it challenging to identify issues as they arise. If GC logs aren’t examined, you’ll miss vital information that could lead to identifying memory leaks or performance bottlenecks.
Example: Enabling GC Logging
-XX:+PrintGCDetails -XX:+PrintGCDateStamps -Xloggc:gc.log
By using the above, you can get detailed GC logs.
Why: Continuous monitoring helps you understand how GC impacts your application's performance over time and allows you to make informed tuning decisions.
- Believing One Size Fits All
It’s a common misconception that tuning settings which worked for one application will work for another. Every application has unique challenges and performance requirements.
Example: Application-Specific Tuning
Take into account the specifics of your application's behavior and usage patterns when designing your GC tuning strategy.
Why: Customize GC parameters based on the unique workload of each application for optimal performance.
Final Considerations
Mastering JVM garbage collection is pivotal for creating high-performance Java applications. By understanding the common pitfalls in GC tuning—ranging from over-complication, misunderstanding object lifetimes, and neglecting workload patterns—you can significantly improve the performance and reliability of your applications.
Starting with a streamlined approach, intensive monitoring, and a willingness to adapt tuning strategies based on the specific application context are essential. Embrace profiling and continuous testing to refine GC performance and ensure your application runs smoothly.
For more in-depth understanding, check out these fantastic resources:
- Understanding Java Garbage Collection
- Java Garbage Collection Basics
With these insights, you're now well-equipped to tackle the complexities of JVM GC performance tuning effectively. Happy coding!
Checkout our other articles