Common Pitfalls When Using Jakarta Persistence 3.2
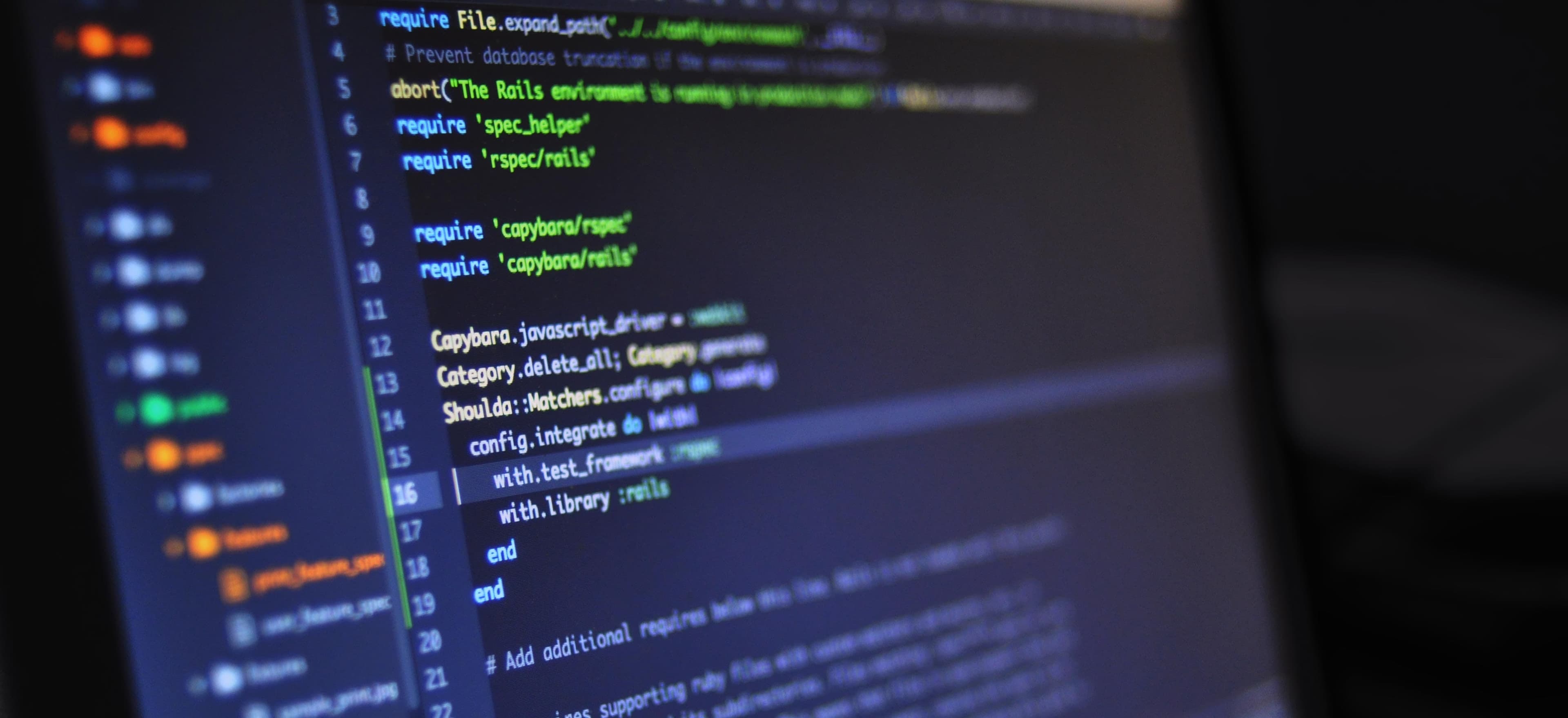
- Published on
Common Pitfalls When Using Jakarta Persistence 3.2
Jakarta Persistence, formerly known as Java Persistence API (JPA), is a key component in managing relational data in Java applications. With the release of Jakarta Persistence 3.2, many developers are excited to adopt its features. However, transitioning to this version, especially if you’re coming from JPA 2.x, can present its own set of challenges. In this post, we will explore some common pitfalls when using Jakarta Persistence 3.2, along with solutions to navigate these challenges effectively.
Understanding Jakarta Persistence
Before delving into the pitfalls, let us briefly clarify the role of Jakarta Persistence. It provides a set of APIs for accessing and managing relational data in the Java programming language. This allows developers to perform CRUD (Create, Read, Update, Delete) operations without needing to write lots of boilerplate code.
Key Features of Jakarta Persistence 3.2
- Support for Streams: Jakarta Persistence 3.2 introduces support for the Java Streams API, allowing developers to perform pipelined operations on collections of entities.
- Immutable Entities: This version allows entities to be immutable by default, enhancing data integrity.
- Improved Batch Processing: Optimized for batch processing, resulting in performance improvements when working with large volumes of data.
Common Pitfalls in Jakarta Persistence 3.2
1. Misunderstanding the Entity Lifecycle
One of the most common pitfalls is misunderstanding the lifecycle of entities. In Jakarta Persistence, entities can be in different states: transient, managed, detached, or removed. Each state behaves differently in relation to the persistence context.
Solution
Ensure you are familiar with the lifecycle:
- Transient: Entities not associated with any persistence context.
- Managed: Currently being tracked and synchronized with the database.
- Detached: Previously managed but now not being tracked.
- Removed: Marked for deletion.
Here’s how you might unintentionally leave an entity in a detached state:
EntityManager em = entityManagerFactory.createEntityManager();
em.getTransaction().begin();
MyEntity entity = new MyEntity();
em.persist(entity); // Transient to Managed
em.getTransaction().commit();
em.clear(); // Disconnects the entity
// Now the entity is in a Detached state.
Understanding these states will help you avoid unexpected behaviors, such as changes not being persisted due to the entity being detached.
2. Poorly Configured Fetch Strategies
Fetch strategies determine how and when data is loaded from the database. A common misconception is that using EAGER
fetching will always yield better performance. In fact, overusing EAGER
can result in the N+1 select problem, where one query results in several additional queries.
Solution
Utilize LAZY
fetching where appropriate and manage transactions properly:
@Entity
public class User {
@OneToMany(mappedBy = "user", fetch = FetchType.LAZY)
private List<Orders> orders;
}
By using LAZY
fetching, you can optimize data retrieval by loading data only when it’s needed, reducing the number of queries to the database.
3. Not Leveraging Streams
With the update in Jakarta Persistence 3.2, one can utilize the Java Streams API for more flexible and functional handling of collections. Many developers are still not taking advantage of this, opting for traditional loops instead.
Solution
Here’s an example of using streams:
List<User> users = entityManager.createQuery("SELECT u FROM User u", User.class).getResultList();
users.stream()
.filter(user -> user.getAge() > 18)
.forEach(System.out::println);
This allows for more readable code and can lead to potential performance gains by processing data in a more functional manner.
4. Ignoring Unit Tests
Testing persistence code is critical, but many developers overlook it. Unit tests provide assurance that your database interactions work as expected and can catch issues early in development.
Solution
Utilize tools such as JUnit and H2 Database for in-memory testing:
@Test
void testUserPersist() {
EntityManager em = entityManagerFactory.createEntityManager();
em.getTransaction().begin();
User user = new User("John Doe", 30);
em.persist(user);
em.getTransaction().commit();
User foundUser = em.find(User.class, user.getId());
assertEquals("John Doe", foundUser.getName());
em.close();
}
By validating your persistence logic, you will reduce the risk of introducing bugs into your application.
5. Inefficient Bulk Updates
Bulk operations can result in better performance but are often done incorrectly. For example, using the EntityManager
for bulk updates may lead to unexpected results, as the persistence context might not be in sync with the database.
Solution
Use JPQL for bulk updates:
entityManager.getTransaction().begin();
entityManager.createQuery("UPDATE User u SET u.status = :status WHERE u.age < :age")
.setParameter("status", "inactive")
.setParameter("age", 18)
.executeUpdate();
entityManager.getTransaction().commit();
This approach updates the database directly without going through the persistence context, ensuring that performance remains high.
6. Mapping Issues with Immuntable Entities
Jakarta Persistence 3.2 introduced support for immutable entities, but there can be pitfalls in the mapping. Developers may try to mix immutable and mutable entities in a single transaction erroneously.
Solution
Make clear distinctions between your entity classes. Use the following pattern for a proper immutable entity:
@Entity
public class ImmutableUser {
@Id
private final Long id;
private final String name;
// Constructor and getters
public ImmutableUser(Long id, String name) {
this.id = id;
this.name = name;
}
public Long getId() { return id; }
public String getName() { return name; }
}
By using final
fields and an explicit constructor, you will enforce immutability and ensure that changes cannot occur post-creation.
Bringing It All Together
Jakarta Persistence 3.2 offers a wealth of features that can greatly enhance your Java data management experience. However, there are numerous pitfalls that developers face when transitioning to this new version. By understanding the entity lifecycle, leveraging streams effectively, configuring fetch strategies properly, employing unit tests, executing bulk updates efficiently, and accurately mapping immutable entities, you can avoid these common mistakes.
Additional Resources
For further reading, consider these insightful resources:
- Jakarta Persistence Documentation
- Effective Java by Joshua Bloch
- Building a RESTful API with Spring & JPA
By keeping the above considerations in mind, you will be well-equipped to implement Jakarta Persistence in a way that maximizes performance, maintainability, and efficiency. Let’s continue to explore this fantastic technology and push our Java applications forward. Happy coding!