Mastering Elegant Date-Time Formatting in Code
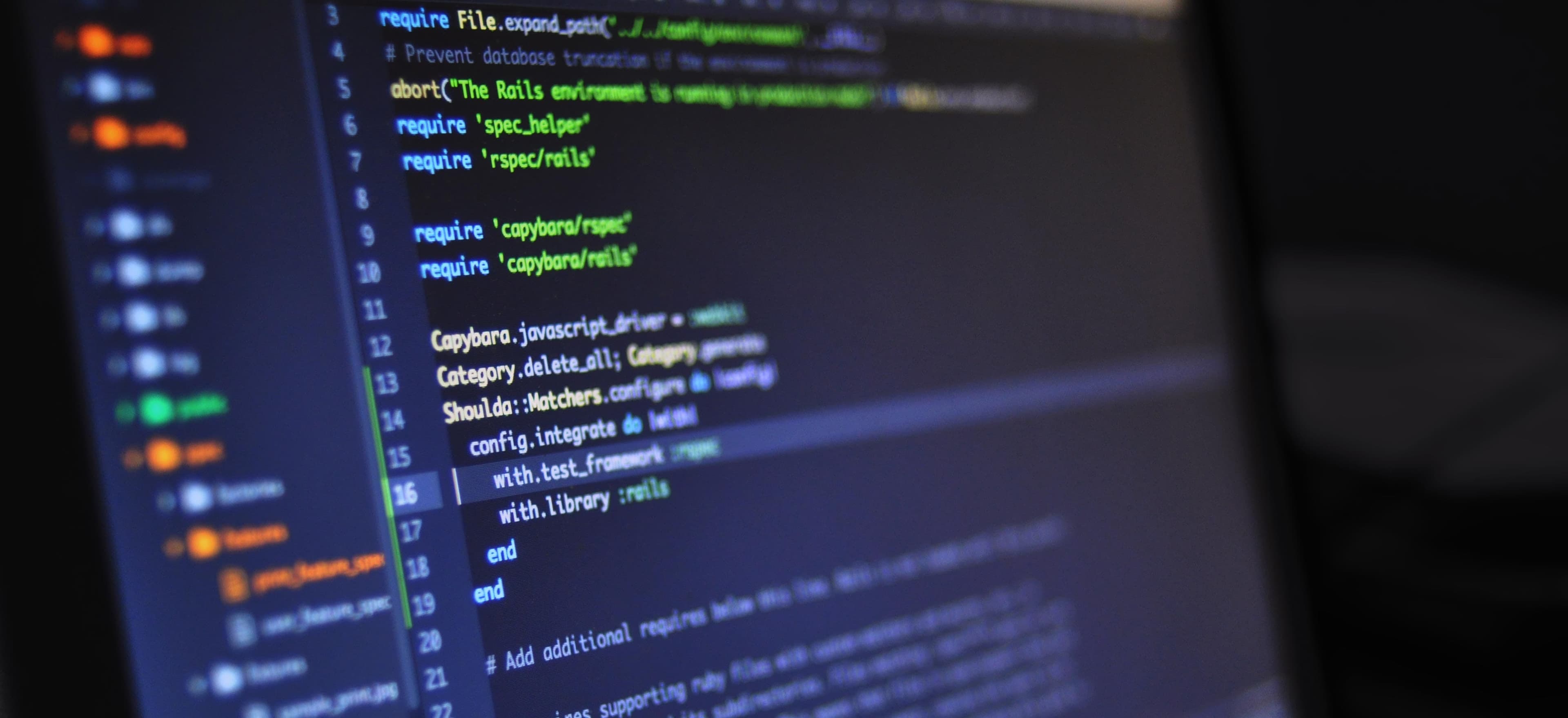
- Published on
Mastering Elegant Date-Time Formatting in Code
When it comes to software development, handling date and time can be one of the trickiest aspects. Timezones, daylight savings, and various formats can make the best of us blink twice. In this article, we'll dive deeply into date-time formatting using Java, helping you master it with elegance and efficiency.
Understanding the Importance of Date-Time Formatting
Before we embark on the coding journey, let's understand why date-time formatting is critical. Well-formatted dates contribute to better user experience, ensure data consistency, and enhance the readability of timestamps across different systems and interfaces.
For example, while 2023-10-05
is pretty standard, a user from France may expect the date as 05-10-2023
. A well-implemented date-time formatting system can therefore bridge cultural or regional habits seamlessly.
Java's Date-Time API
Since Java 8, the introduction of the Java Time API was a game-changer, built around the java.time
package. This API provides a comprehensive handling system for dates and times.
Key Classes in the Java Time API
- LocalDate: Represents a date without a time-zone.
- LocalTime: Represents a time without a time-zone.
- LocalDateTime: Combines local date and time without a time-zone.
- ZonedDateTime: A date-time with a time-zone.
- Duration and Period: Duration represents a time-based amount of time, while Period represents a date-based amount.
Example of LocalDate and LocalDateTime
import java.time.LocalDate;
import java.time.LocalDateTime;
public class DateExample {
public static void main(String[] args) {
// Getting Current Date
LocalDate currentDate = LocalDate.now();
System.out.println("Current Date: " + currentDate);
// Getting Current DateTime
LocalDateTime currentDateTime = LocalDateTime.now();
System.out.println("Current DateTime: " + currentDateTime);
}
}
Here, we utilize LocalDate.now()
and LocalDateTime.now()
to fetch the current date and date-time seamlessly.
Formatting Date and Time
Effective formatting can transform raw date and time values into user-friendly strings. This is where java.time.format.DateTimeFormatter
comes into play.
Example: Formatting a LocalDate
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
public class DateFormattingExample {
public static void main(String[] args) {
LocalDate today = LocalDate.now();
// Format date to DD/MM/YYYY
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("dd/MM/yyyy");
String formattedDate = today.format(formatter);
System.out.println("Formatted Date: " + formattedDate);
}
}
In the code above, we define a format of dd/MM/yyyy
, resulting in a string that may appear as 05/10/2023
. The DateTimeFormatter
is powerful because it allows you to create patterns that match the user's preferences seamlessly.
Common Date-Time Patterns
To facilitate ease of formatting, here are some commonly used patterns:
yyyy-MM-dd
for ISO date formatdd/MM/yyyy
for European formatMM-dd-yyyy
for US formatyyyy-MM-dd'T'HH:mm:ss.SSS'Z'
for ISO 8601
For detailed patterns, refer to the Java documentation.
Parsing Dates from Strings
Parsing is equally crucial; it allows you to convert formatted dates back into LocalDate
or LocalDateTime
objects.
Example: Parsing a Date String
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
public class DateParsingExample {
public static void main(String[] args) {
String dateString = "05/10/2023";
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("dd/MM/yyyy");
LocalDate parsedDate = LocalDate.parse(dateString, formatter);
System.out.println("Parsed Date: " + parsedDate);
}
}
In this example, a string 05/10/2023
is parsed into a LocalDate
using the same formatter. Note how the formatter used for parsing must match the format of the date string precisely.
Handling Time Zones with ZonedDateTime
When dealing with applications that span multiple regions, ZonedDateTime
proves critical. Time offsets and daylight savings can create complex scenarios.
Example: Working with ZonedDateTime
import java.time.ZonedDateTime;
import java.time.format.DateTimeFormatter;
public class ZonedDateExample {
public static void main(String[] args) {
ZonedDateTime zonedDateTime = ZonedDateTime.now();
// Format with timezone
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss z");
String formattedZonedDateTime = zonedDateTime.format(formatter);
System.out.println("Formatted Zoned DateTime: " + formattedZonedDateTime);
}
}
Here, we utilize ZonedDateTime.now()
to get the current date-time with zone information. Formatting including the zone (z
in the pattern) offers clarity about the timing context.
Best Practices for Date-Time Formatting
- Use the Java Time API: Avoid
java.util.Date
andjava.util.Calendar
as they are less efficient and more error-prone. - Be Consistent: Utilize the same patterns throughout your application for consistency.
- Consider User Preferences: If users are from different regions, consider dynamically formatting dates based on user location or settings.
- Fallback Options: Always provide default formats or error-handling routines to manage bad input gracefully.
The Last Word
Mastering date-time formatting in Java not only enhances the user experience but also promotes code quality and maintainability. Leveraging the powerful Java Time API allows developers to work with dates and times effectively, reducing the likelihood of bugs and inconsistencies.
As you venture further into date-time handling, consider exploring libraries like Joda-Time for specialized needs. For comprehensive understanding, dive deeper into the Java Time documentation.
Feel free to share your date-time formatting experiences and best practices in the comments below. Happy coding!
Checkout our other articles