Mastering Gradle: Displaying Buildscript Dependencies Made Easy
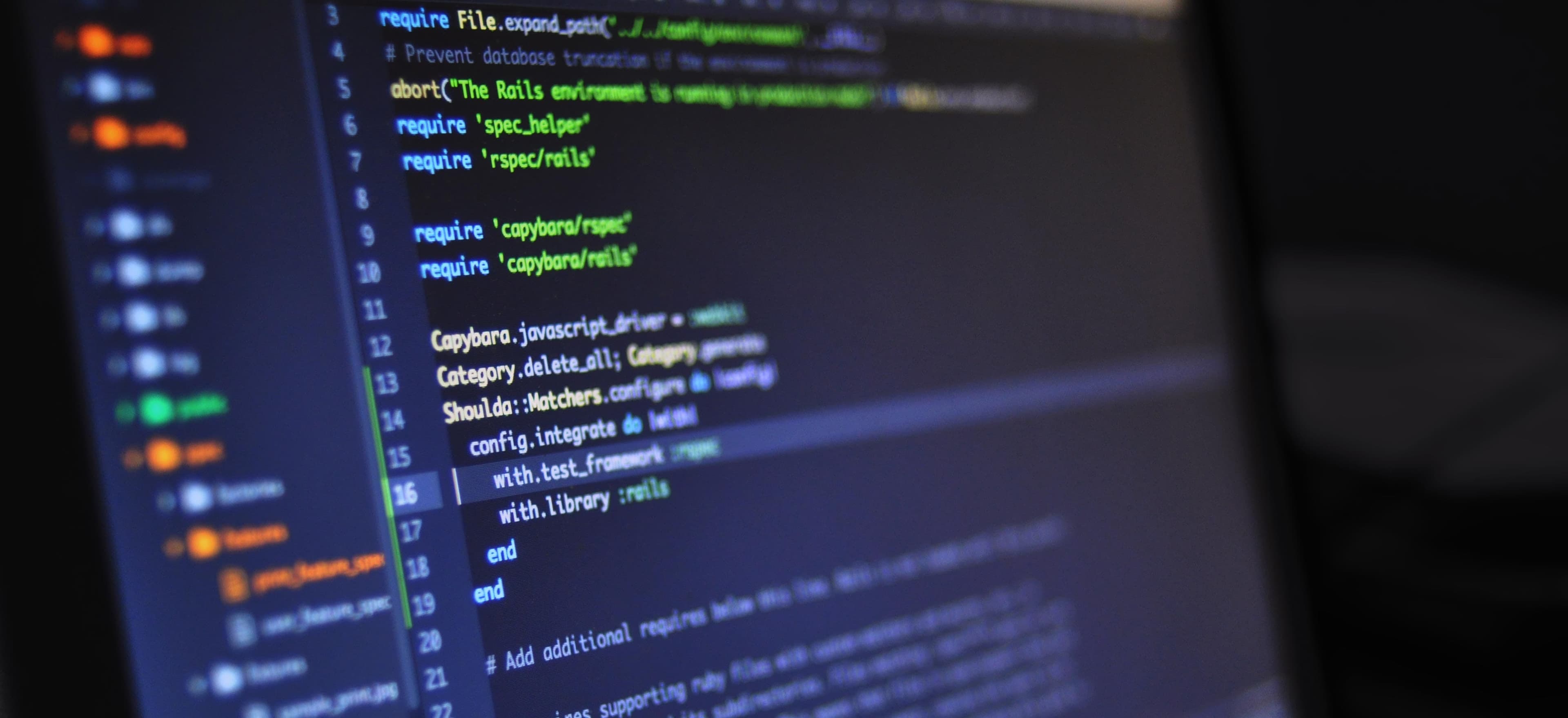
- Published on
Mastering Gradle: Displaying Buildscript Dependencies Made Easy
When dealing with Java projects, build automation plays a crucial role in streamlining workflows. One of the most popular tools for this purpose is Gradle. It offers flexibility, performance, and ease of use. Understanding how to manage and display your buildscript dependencies in Gradle can make your development process significantly smoother.
In this blog post, we will delve into the intricacies of Gradle, focusing specifically on how to display your buildscript dependencies. By the end, you should understand how to utilize Gradle features to your advantage. Let's get started!
What is Gradle?
Gradle is a powerful build automation tool capable of supporting multi-language projects. Written in Groovy (and Kotlin), Gradle allows you to define your build logic in code, which brings flexibility and maintainability to your project. With features like dependency management, task management, and customizable build scripts, Gradle has become the go-to for many developers in the Java ecosystem.
Gradle's core strength lies in its dependency management system, where you can manage libraries your application uses, including those for testing and production. Dependencies can be defined both in your main application and in your buildscript, the latter being the focus of this post.
Understanding Buildscript Dependencies
In a Gradle project, there are generally two types of dependencies: project dependencies and buildscript dependencies.
- Project dependencies are the libraries that your application needs to compile and run.
- Buildscript dependencies are the libraries that the build system itself requires to execute the build process.
By specifying buildscript dependencies, you can extend Gradle's functionality. This is particularly useful when you need to use plugins or custom tasks defined outside of your base Gradle project.
How to Specify Buildscript Dependencies
Buildscript dependencies can be defined within the buildscript
closure in your build.gradle
file. Here’s a simple example:
buildscript {
repositories {
mavenCentral() // Enables you to pull from Maven Central
}
dependencies {
classpath 'com.android.tools.build:gradle:4.1.0' // Example of a buildscript dependency
}
}
Breakdown of the Code
buildscript { ... }
: This block specifies that the dependencies inside should be loaded by the build script itself.repositories { ... }
: Here, we declare where Gradle should search for the dependencies.dependencies { ... }
: In this section, you can add dependencies necessary for your build process.
Adding the required dependencies in this manner ensures that your build environment has everything it needs to execute tasks correctly.
Displaying Buildscript Dependencies
Displaying buildscript dependencies can be incredibly useful for debugging or understanding what libraries your build environment relies on. Gradle provides a straightforward way to visualize this. By using the command line, you can list all your dependencies easily.
Using Gradle Command Line
To display your buildscript dependencies, you can use the following command:
./gradlew buildEnvironment
Understanding the Output
The output will provide you with a report similar to this:
> Task :buildEnvironment
------------------------------------------------------------
Root project
------------------------------------------------------------
buildscript classpath
+--- com.android.tools.build:gradle:4.1.0
+--- org.jetbrains.kotlin:kotlin-gradle-plugin:1.3.72
This output shows the dependencies required by your buildscript. Notably, you can see the libraries included, which gives you insight into the plugins and tools you're using for your project.
Alternative: Using Gradle Build Scan
For a more detailed view of your project dependencies, consider using Gradle Build Scans. This feature provides an extensive overview and is particularly useful for larger projects. To enable build scans, you can add the following to your project:
plugins {
id 'com.gradle.build-scan' version '3.0'
}
buildScan {
publishOnFailure()
}
After running a build with the command ./gradlew build
, you will receive a link to the build scan where you can explore your dependencies in an interactive graph.
Viewing Dependencies via IDE
Many modern IDEs, like IntelliJ IDEA and Eclipse, offer built-in tools to visualize Gradle dependencies. In IntelliJ, you can view dependencies through the "Gradle" tab located on the right side of the window:
- Open the Gradle tab.
- Expand your project.
- Navigate to
Tasks -> help
and findbuildEnvironment
.
Here you can see a tree of your write dependency structure.
Why Optimize Your Buildscript Dependencies?
Optimizing and understanding buildscript dependencies can lead to improved build performance and reduced complexity. When your development environment is clean and efficient, you:
- Reduce build time: Avoid unnecessary dependencies that could slow down your build process.
- Minimize issues: Prevent conflicts and potential runtime issues by clarifying which libraries are loaded.
- Promote better collaboration: Ensure that other developers on your team can replicate your environment easily.
Best Practices for Managing Buildscript Dependencies
- Keep It Clean: Regularly review and remove unused dependencies. This keeps your build script lean and efficient.
- Use Latest Versions: Make sure to use updated libraries to promote security and performance.
- Document Your Dependencies: Comment on what each dependency is used for within your project to aid future developers.
Example of a Well-Organized build.gradle
Here’s an example of a well-structured build.gradle
file:
buildscript {
repositories {
mavenCentral()
}
dependencies {
classpath 'org.springframework.boot:spring-boot-gradle-plugin:2.5.4'
classpath 'com.github.johnrengelman.shadow:shadow-gradle-plugin:7.0.0' // Allows creating fat JAR
}
}
apply plugin: 'org.springframework.boot'
apply plugin: 'io.spring.dependency-management'
repositories {
mavenCentral()
}
dependencies {
implementation 'org.springframework.boot:spring-boot-starter-web'
implementation 'org.springframework.boot:spring-boot-starter-data-jpa'
testImplementation 'org.springframework.boot:spring-boot-starter-test'
}
Key Takeaways
- By structuring your
build.gradle
file clearly and maintaining a concise list of dependencies, you can greatly improve the readability and performance of your project. - Gradle makes it simple to manage and visualize dependencies, which ultimately leads to a more efficient development process.
The Last Word
Mastering Gradle is pivotal for any modern Java developer. Understanding how to accurately define and display buildscript dependencies helps create a more manageable development environment. With the command line tools, IDE support, and best practices outlined in this post, you can ensure that your project remains clean, efficient, and easy to collaborate on.
For more information on Gradle best practices, the Gradle User Manual is an excellent resource to continue your learning. Happy building!