Common JAXB Pitfalls Newbies Face and How to Avoid Them
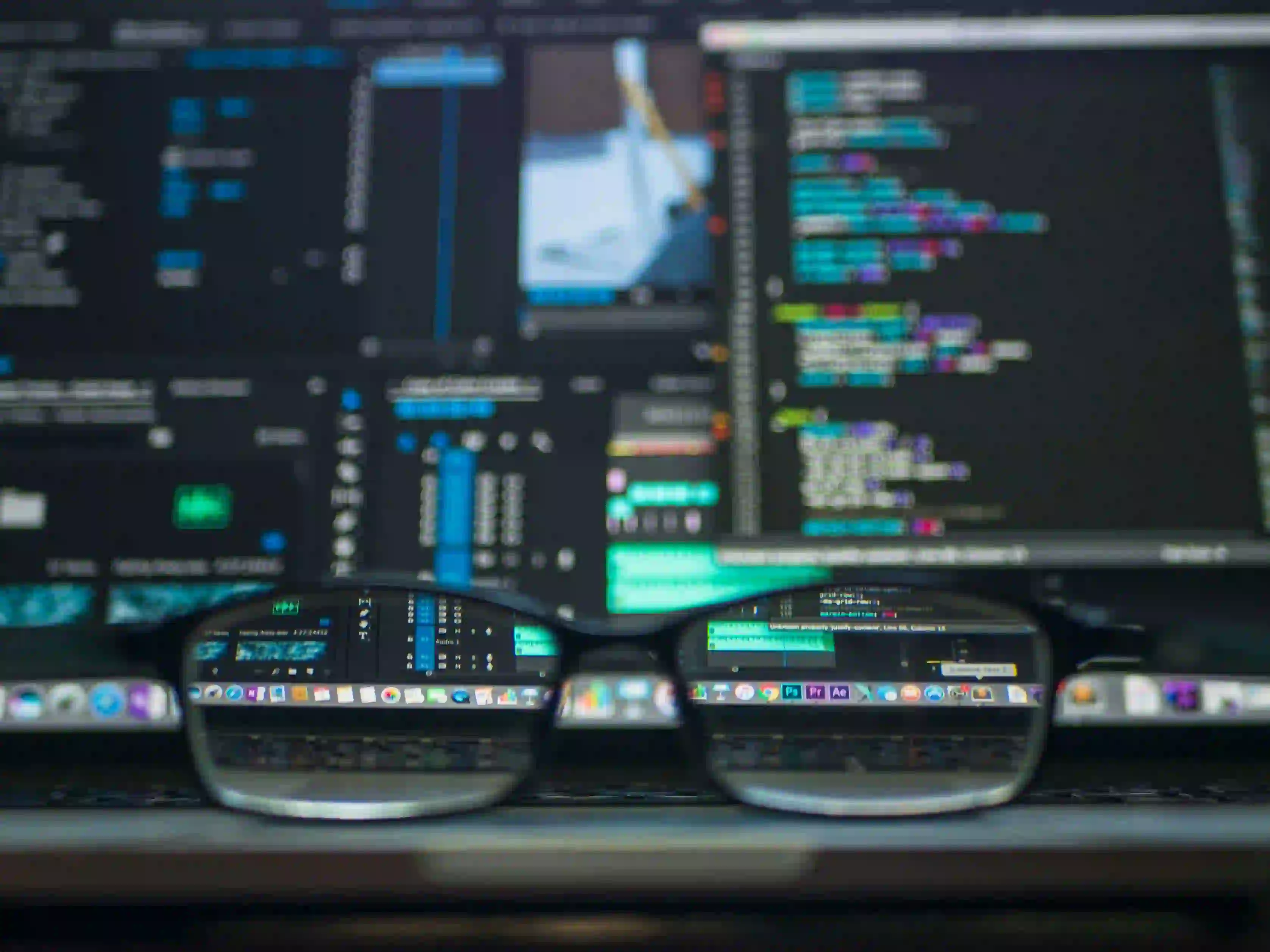
Common JAXB Pitfalls Newbies Face and How to Avoid Them
Java Architecture for XML Binding (JAXB) is a powerful framework that helps developers to convert Java objects to XML and vice versa. Despite its utility, many newcomers encounter a range of challenges while using JAXB. In this blog post, we will explore some common pitfalls faced by newbies and provide practical strategies to overcome them.
What is JAXB?
Before diving into the pitfalls, let's clarify what JAXB is. It allows developers to interact with XML easily, without requiring deep knowledge of the XML language. In general, the process works as follows:
- Create Java Classes: Define classes that correspond to your XML structure.
- Generate XML: Utilize JAXB's marshalling capabilities to convert Java objects into XML.
- Parse XML: Use unmarshalling to convert XML back into Java objects.
For instance, JAXB simplifies data transformation through automatic binding between XML and Java objects.
Pitfall 1: Incorrect Annotations
Understanding JAXB Annotations
JAXB uses several key annotations. These include @XmlRootElement
, @XmlElement
, and @XmlAttribute
, among others. Misunderstanding or misplacing these annotations often leads to unintended results.
Solution
Ensure you understand the purpose of each annotation. For instance:
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
@XmlRootElement
public class Person {
private String name;
@XmlElement
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
In this example, the @XmlRootElement
annotation marks the class as root for XML representation, while @XmlElement
annotates a getter method to specify it as an XML element.
Tips
- Always read the JAXB documentation for specific annotations to understand their implications.
- Use the correct combination of annotations to capture your XML structure accurately.
Pitfall 2: Ignoring Default Values
Default Values in Binding
By default, JAXB does not serialize fields that are set to their default values (like null for objects or 0 for numbers). This can lead to XML output that seems incomplete or incorrect.
Solution
If you need to include default values, employ the @XmlAccessorType
annotation:
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
@XmlRootElement
@XmlAccessorType(XmlAccessType.FIELD)
public class Person {
@XmlElement(required = true)
private String name;
@XmlElement
private int age = 0; // Default value included in serialization
// Getters and Setters
}
Tips
- Use
@XmlAccessorType(XmlAccessType.FIELD)
to automatically include all fields, even those with default values, as long as they are marked accordingly. - Always test XML output for completeness before going live.
Pitfall 3: Mismanagement of Namespaces
Context of Namespaces
XML namespaces are crucial in avoiding element name conflicts, especially when integrating differing XML sources. Newbies often overlook this aspect, leading to potential parsing errors.
Solution
Explicitly define namespaces using @XmlSchema
or @XmlType
annotations:
import javax.xml.bind.annotation.XmlType;
@XmlType(name="Person", namespace="http://example.com/people")
public class Person {
// Class definition
}
Tips
- Always declare your namespaces according to your XML schema.
- Utilize an online tool or XML editor to validate your XML structure against the schema.
Pitfall 4: Forgetting to Handle Exceptions
Importance of Exception Handling
When working with XML, exception handling is critical. New developers may ignore JAXBException
, which can lead to unhandled runtime errors.
Solution
Wrap marshalling and unmarshalling operations in try-catch blocks:
import javax.xml.bind.JAXBContext;
import javax.xml.bind.JAXBException;
import javax.xml.bind.Marshaller;
public class MarshallExample {
public static void main(String[] args) {
Person person = new Person();
person.setName("John Doe");
try {
JAXBContext context = JAXBContext.newInstance(Person.class);
Marshaller marshaller = context.createMarshaller();
marshaller.setProperty(Marshaller.JAXB_FORMATTED_OUTPUT, true);
marshaller.marshal(person, System.out);
} catch (JAXBException e) {
e.printStackTrace();
}
}
}
Tips
- Always log exceptions meaningfully.
- Understand the different exception types and handle them accordingly.
Pitfall 5: Serialization Issues with Collections
Collections in JAXB
Working with lists or collections can lead to serialization problems if not handled carefully. JAXB requires collections to be properly annotated.
Solution
Use @XmlElementWrapper
and @XmlElement
annotations:
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlElementWrapper;
import javax.xml.bind.annotation.XmlRootElement;
import java.util.ArrayList;
import java.util.List;
@XmlRootElement
public class Group {
@XmlElementWrapper(name = "members")
@XmlElement(name = "member")
private List<String> members = new ArrayList<>();
// Getters and Setters
}
Tips
- Always wrap collection fields to clarify their serialization format.
- Test with sample XML documents to ensure proper output.
A Final Look
JAXB is an excellent tool for XML binding, but like any powerful framework, it comes with its own set of challenges. By being aware of these common pitfalls, especially regarding annotations, default values, namespaces, exception handling, and collections, you can navigate the complexities of JAXB more effectively.
Mastering JAXB requires practice and the willingness to learn from mistakes. Utilize resources like the Official JAXB Documentation and access community forums such as Stack Overflow for ongoing support.
With the right knowledge and understanding, you can leverage JAXB efficiently in your Java applications and make XML manipulation a breeze.
With these guidelines and insights, beginners can establish a strong foundation in JAXB, paving the way to becoming proficient in XML data handling within Java applications. Keep practicing, stay curious, and happy coding!