Navigating the Challenges of JDK 9's Deprecated Annotations
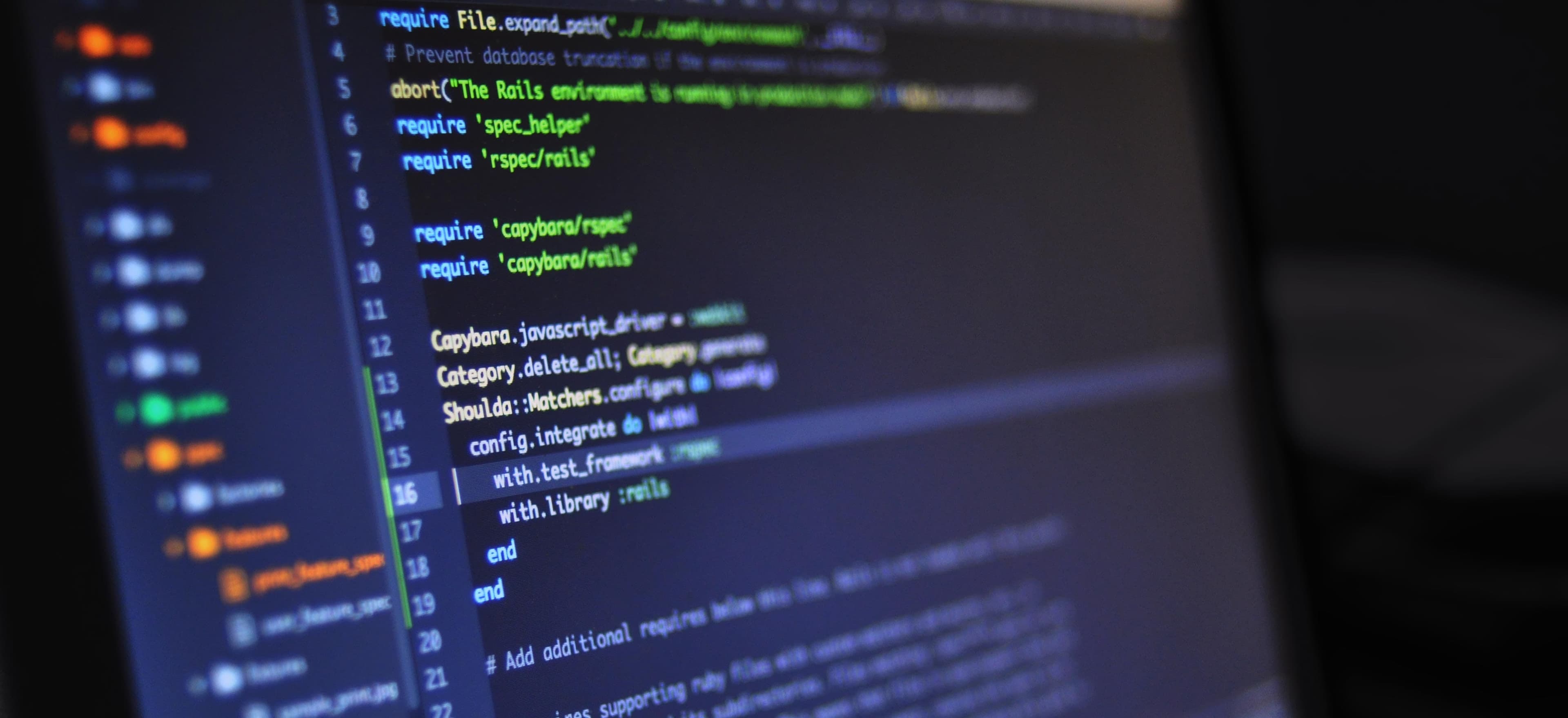
- Published on
Navigating the Challenges of JDK 9's Deprecated Annotations
The introduction of Java Development Kit (JDK) 9 marked a significant step forward in the evolution of the Java programming language. With new features like the Java Platform Module System and several enhancements, JDK 9 has streamlined and modernized numerous aspects of Java. However, with progress comes challenges, particularly in the form of deprecated annotations.
In this blog post, we will explore the implications of deprecated annotations in JDK 9, discuss common use cases, and provide guidance on how to navigate these challenges effectively.
Understanding Deprecated Annotations
Annotations in Java are a form of metadata that provide data about a program but are not part of the program itself. They can be applied to classes, methods, parameters, and fields, and are generally used for various purposes, including providing information to the compiler, generating code, and influencing runtime behavior.
When an annotation is marked as deprecated, it indicates that the annotation should no longer be used, and there is typically a recommended alternative. In JDK 9, several annotations were deprecated, paving the way for cleaner, more efficient code practices.
Why Annotations are Deprecated
There are several reasons why a Java annotation may be deprecated:
- Redundant Functionality: Newer annotations may provide similar or enhanced functionality.
- Unresolved Issues: Specific annotations might lead to confusion or bugs in code.
- Framework Evolution: Frequently, annotations depend on frameworks that have evolved or undergone major changes, rendering the older annotations obsolete.
To comprehend these points fully, let’s take a look at some of the deprecated annotations from JDK 9.
Key Deprecated Annotations in JDK 9
1. @FunctionalInterface
Originally introduced in Java 8, @FunctionalInterface
ensures that an interface is a functional interface with exactly one abstract method. While this annotation is not deprecated per se, its use is discouraged in the context of certain situations where being explicit is unnecessary. The JDK team elected to provide guidance on its usage rather than fully deprecate it.
Common Mistake: Developers often assume that all interfaces meant for Lambda expressions should have this annotation. This is not always necessary.
Code Example:
@FunctionalInterface
public interface MyFunction {
void apply(String input);
}
Why This Matters: The @FunctionalInterface
annotation serves as documentation and enforces design contracts, but relying on it when not needed could clutter your codebase.
2. @Deprecated
with no Replacement
In many cases, you might find annotations that have been deprecated with no direct replacement. This situation can be tricky as it does not point you toward a clear alternative.
Common Scenario: Using Deprecated Annotations
Suppose you encounter the @Deprecated
annotation on @Override
, which originally existed to clarify intentions in overriding methods. While removing @Deprecated
from your code might seem like an easy fix, you could inadvertently lose clarity.
Code Example:
@Deprecated
@Override
public void finalMethod() {
// This method may still execute, but it's advised not to use it
}
Why This Matters: Code maintainability is crucial. Even though a method is deprecated, it’s vital to respect its purpose in your application, especially if it’s involved in system-level operations or user-facing functionalities.
3. @SuppressWarnings
The @SuppressWarnings
annotation allows programmers to instruct the compiler to ignore specific warnings. In recent versions, such warnings have been re-evaluated.
Code Example:
@SuppressWarnings("unchecked")
public void convertList(List rawList) {
List<String> stringList = (List<String>) rawList; // Cast may throw ClassCastException
}
Why This Matters: Suppressing warnings can lead to hidden bugs. It is often more effective to address the underlying warning rather than silencing it.
Navigating Deprecated Annotations
Now that we understand which annotations are deprecated, how do we navigate these changes effectively? Here are some best practices:
1. Stay Informed
Regularly update your knowledge about Java releases. Resources such as the official Java documentation provide valuable insights into deprecated features.
2. Refactor Your Codebase
Consider refactoring parts of your code that utilize deprecated annotations. This can include removing unused annotations or adapting your code to no longer rely on specific deprecated behaviors.
3. Utilize IDE Features
Most modern Integrated Development Environments (IDEs) provide tools for detecting deprecated annotations. Take advantage of these tools to identify affected parts of your code.
4. Test Thoroughly
When altering or removing deprecated annotations, ensure that you run comprehensive tests on your codebase. This will help catch any potential issues before deployment.
Example Case Study
Imagine a project that originally utilized the @Deprecated
annotation for an older configuration handling system. To illustrate, consider the transition to a more modular architecture with the new features in JDK 9.
Old Code Implementation:
@Deprecated
public class ConfigurationLoader {
public void load() {
// Loads configurations from legacy config files.
}
}
Refactored Code Implementation:
Replace the deprecated class with a new, modular approach using Java's ServiceLoader
.
public class ModularConfigurationLoader {
public void load() {
// Load configurations using Java's ServiceLoader for modularity.
}
}
Final Note
While the transition to eliminate deprecated annotations can be challenging, it also presents an excellent opportunity to improve your code’s overall architecture and maintainability. Keeping your codebase updated not only enhances performance but also makes the application easier to maintain and evolve in the long run.
For more on Java annotations and their applications, check out Baeldung on Java Annotations.
By being proactive and informed about deprecated annotations in JDK 9, you will position your projects for better performance and sustainability. Remember to implement a continuous learning culture and keep abreast of Java developments as you navigate these changes. Happy coding!
Checkout our other articles