The Paradox of Smart and Lazy: Unlocking Developer Productivity
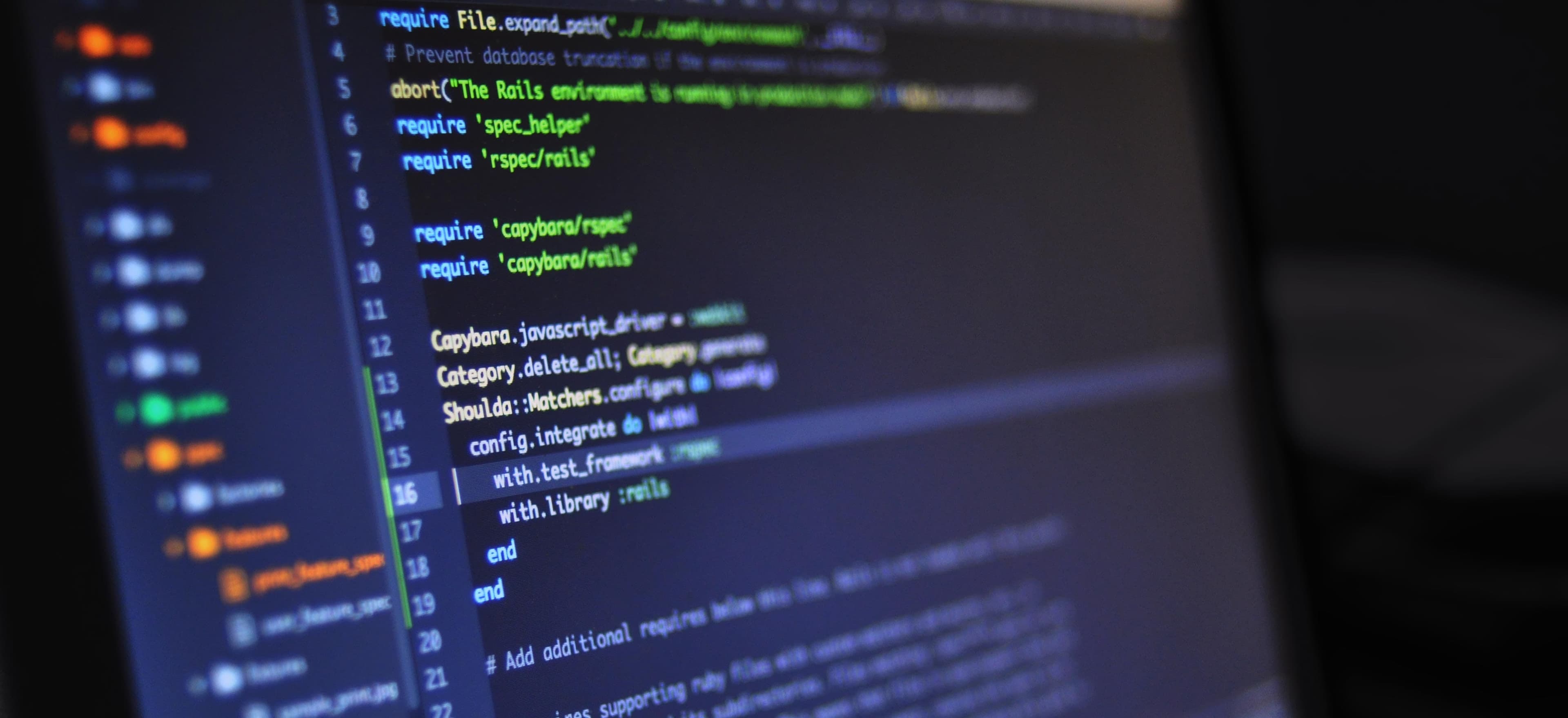
- Published on
The Paradox of Smart and Lazy: Unlocking Developer Productivity
In the fast-paced world of software development, there's an eternal debate about the nature of productivity. Professional software developers often find themselves in a paradox: the "smart" way to work sometimes appears to be downright "lazy." In this blog post, we will explore how adopting a more intelligent approach can lead to heightened productivity, ultimately benefiting both developers and their organizations.
Understanding the Smart and Lazy Paradox
At its core, the paradox of smart and lazy highlights a common misconception about work ethic. Many believe that working harder or longer hours directly correlates with higher productivity. However, the truth often lies in working smarter, not necessarily harder.
The most successful developers focus on:
- Efficiency: Writing cleaner and more maintainable code.
- Automation: Implementing tools that save time and eliminate repetitive tasks.
- Collaboration: Leveraging collective knowledge and skill sets.
To illustrate this concept in a clearer manner, let's consider an analogy: a lumberjack who spends all day physically chopping down trees versus a lumberjack who uses a chainsaw. The latter isn't being lazy; he’s being smart about his work.
Key Strategies for Enhancing Developer Productivity
1. Embrace Automation
Automation plays a crucial role in freeing developers from repetitive tasks. Imagine a time-consuming manual testing process that could take hours; automating that process allows for quicker feedback and minimizes human error.
Code Snippet: Automated Testing with JUnit
Let’s implement a simple unit test using JUnit to demonstrate the power of automation in testing:
import static org.junit.jupiter.api.Assertions.assertEquals;
import org.junit.jupiter.api.Test;
class MathUtilsTest {
@Test
void testAdd() {
MathUtils mathUtils = new MathUtils();
assertEquals(5, mathUtils.add(2, 3)); // Expect the output to be 5
}
}
Why this matters: Automated testing saves time. Instead of manually checking outcomes, developers can run tests with a single command. This also ensures that new features do not break existing functionality.
2. Utilize Version Control Systems
Version control systems, such as Git, allow developers to manage changes to code. This practice encourages collaborative work and keeps track of every modification made. Understanding version control is not laziness; it’s an essential skill.
Code Snippet: Basic Git Commands
Below are some basic Git commands that developers should know:
# Initialize a new Git repository
git init
# Add changes to the staging area
git add .
# Commit changes with a message
git commit -m "Initial commit"
# Push changes to a remote repository
git push origin main
Why this matters: Version control systems provide a safety net. If something goes wrong, developers can revert to a previous version of the code, minimizing downtime and productivity loss.
3. Optimize Workflows with Integrated Development Environments (IDEs)
Utilizing powerful IDEs can significantly boost productivity. IDEs offer autocomplete suggestions, debugging tools, and even integration with build systems—all in one place.
Code Snippet: Using IntelliJ IDEA for Code Refactoring
In an IDE like IntelliJ IDEA, you can easily refactor your code. For example, suppose you have the following function that calculates square roots:
public double calculateSquareRoot(double number) {
return Math.sqrt(number);
}
If you want to change its name for clarity, simply use the refactoring feature:
- Right-click on the function name.
- Select Rename.
- Type the new name and hit Enter.
Why this matters: Refactoring keeps code clean and maintainable. IDEs automate a lot of the under-the-hood changes, allowing developers to focus more on logic instead of syntax.
4. Code Reviews and Pair Programming
Engaging in code reviews and pair programming fosters a collaborative environment. It benefits developers through knowledge sharing and immediate feedback.
Example Workflow
-
Code Review Process:
- Developer A writes code.
- Developer B reviews and provides feedback.
- Corrections are made, enhancing the codebase’s quality.
-
Pair Programming:
- Two developers work together at one workstation.
- One writes code (the Driver) while the other reviews each line (the Navigator).
Why this matters: Code reviews ensure that best practices are maintained, while pair programming leads to higher quality code. Both foster a learning culture that champions collaboration.
5. Focus on Continuous Learning
Lastly, the best developers recognize the importance of continuous learning. With the tech industry evolving rapidly, it’s vital to stay up-to-date on new frameworks, tools, and practices.
Online Resources for Continuous Learning
- Coursera: Online courses on various programming languages and frameworks.
- edX: Offers free online courses from top universities.
- LeetCode: A platform for practicing coding problems to enhance problem-solving skills.
Why this matters: Continuous learning keeps developers sharp and relevant in a competitive job market. It fosters adaptability, a crucial attribute in tech.
Lessons Learned: Finding the Balance
The paradox of smart and lazy reveals an essential truth about productivity in the developer realm. By prioritizing intelligent solutions over simply putting in hours, developers can maximize their effectiveness.
Embrace automation. Utilize version control. Opt for IDEs designed to streamline your workflow. Engage in collaborative practices like code reviews and pair programming. And finally, never stop learning.
Remember, working smarter doesn’t mean being lazy; it means being resourceful and efficient. Whether you're a seasoned developer or just starting, understanding and leveraging this paradox can unlock new levels of productivity and job satisfaction.
Further Reading
- The Myth of the Overworked Developer
- The Importance of Automated Testing
- Developer Productivity: A Comprehensive Guide
By implementing these strategies and recognizing the smart and lazy paradox, developers can ultimately transform their productivity, paving the way for a more fulfilling career in tech.
Checkout our other articles