Troubleshooting Common Google Services Auth Errors
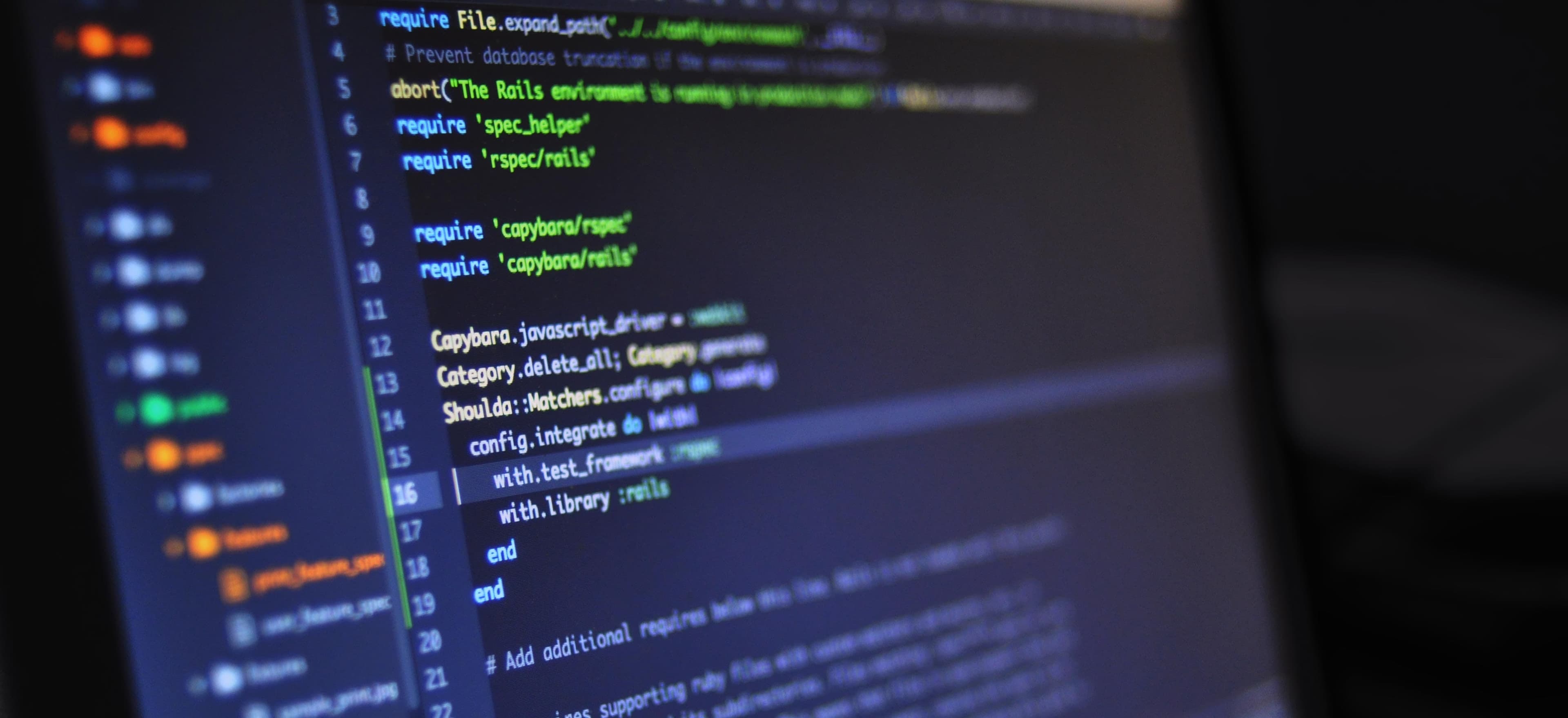
- Published on
Troubleshooting Common Google Services Auth Errors
When integrating Google services into your applications, you might encounter various authentication errors. These errors can halt your development process and cause frustration. In this blog post, we'll guide you through common Google services authentication issues and provide clear solutions.
Table of Contents
- Understanding Google Authentication
- Common Google Services Authentication Errors
- 2.1 Invalid Credentials
- 2.2 OAuth Consent Screen Errors
- 2.3 API Quota Errors
- 2.4 Required Scopes Not Granted
- Step-by-Step Troubleshooting
- Conclusion
Understanding Google Authentication
Google provides a powerful set of APIs through its Google Cloud Platform. To access these APIs, developers must authenticate their applications using OAuth 2.0. This protocol allows applications to obtain limited access to user accounts without exposing passwords. Understanding the basics of Google authentication is crucial for effective troubleshooting.
Key Elements of Google Authentication
- Client ID: A unique identifier for your application.
- Client Secret: A confidential string used to authenticate your application.
- Redirect URI: The endpoint to which Google redirects users after they authenticate.
Resources
Common Google Services Authentication Errors
2.1 Invalid Credentials
This error occurs when the Client ID
or Client Secret
is incorrect.
Solution
- Verify that your credentials are copied accurately from the Google Cloud Console.
- Ensure that your app is using the correct set of credentials for both development and production environments.
Example Code Snippet:
import com.google.api.client.googleapis.auth.oauth2.GoogleClientSecrets;
import java.io.FileReader;
import java.io.IOException;
public class AuthService {
private GoogleClientSecrets loadClientSecrets() throws IOException {
return GoogleClientSecrets.load(
JacksonFactory.getDefaultInstance(),
new FileReader("client_secret.json")
);
}
}
Why: This code snippet loads your client secrets from a JSON file. Ensure the path is correct and the contents are valid.
2.2 OAuth Consent Screen Errors
You may encounter errors related to the OAuth consent screen, especially if it’s not set up correctly.
Solution
- Ensure you have configured the OAuth consent screen in the Google Cloud Console.
- Add necessary scopes based on your application's requirements.
- Test the consent screen for validity and ensure it doesn't display misleading or incomplete information.
Example Configuration:
- Visit the OAuth consent screen configuration page in Google Cloud Console.
- Fill in all required fields.
Why: An improperly configured consent screen can lead to denied permissions, which will interrupt the user flow.
2.3 API Quota Errors
APIs have usage limits. If these limits are exceeded, you will likely see quota errors.
Solution
- Monitor your API usage in the Google Cloud Console.
- Apply for quota increases if necessary.
- Optimize your API calls by caching responses or reducing unnecessary calls.
Example Code Snippet:
import com.google.api.services.sheets.v4.Sheets;
import com.google.api.services.sheets.v4.model.*;
public void fetchData(Sheets sheetsService) {
try {
ValueRange response = sheetsService.spreadsheets().values()
.get("spreadsheetId", "A1:B2")
.execute();
List<List<Object>> values = response.getValues();
if (values == null || values.isEmpty()) {
System.out.println("No data found.");
} else {
for (List row : values) {
System.out.printf("%s, %s\n", row.get(0), row.get(1));
}
}
} catch (Exception e) {
System.out.println("Error fetching data: " + e.getMessage());
}
}
Why: Catching exceptions ensures you can handle errors gracefully, especially when API limits are reached.
2.4 Required Scopes Not Granted
If your application does not request the required scopes, authentication can fail.
Solution
- Review the scopes defined in your request.
- Ensure that users are aware of and consent to the necessary permissions your application requires.
Example Code Snippet:
import com.google.api.client.googleapis.auth.oauth2.GoogleAuthorizationCodeFlow;
import com.google.api.client.util.Strings;
public class OAuthService {
public void authorize() {
GoogleAuthorizationCodeFlow flow = new GoogleAuthorizationCodeFlow.Builder(
HTTP_TRANSPORT, JSON_FACTORY, clientSecrets, Arrays.asList("https://www.googleapis.com/auth/drive.file"))
.setAccessType("offline").build();
String redirectUri = flow.newAuthorizationUrl().setRedirectUri("YOUR_REDIRECT_URI").build();
// Redirect user to their browser for consent
System.out.println("Go to the following URL: " + redirectUri);
}
}
Why: Specifying the correct scopes is crucial for ensuring the application can access the necessary resources.
Step-by-Step Troubleshooting
In this section, we will outline a systematic method for troubleshooting authentication issues:
-
Check Google Cloud Project Settings
- Log into the Google Cloud Console.
- Verify that the project is active and that APIs are enabled.
-
Validate Authentication Credentials
- Ensure that your
Client ID
andClient Secret
are correct. - Check if the credentials fit the environment.
- Ensure that your
-
Inspect OAuth Consent Screen
- Navigate to the OAuth consent screen configuration and review displayed information.
-
Analyze API Quotas
- View quota usage in the Google Cloud Console and request increases as necessary.
-
Review Error Logs
- Examine logs for any specific error messages and corresponding context.
-
Re-test Authentication Flow
- After making adjustments, always retest the authentication flow to verify that issues are resolved.
To Wrap Things Up
Troubleshooting authentication errors in Google services can seem daunting, but by systematically checking your configurations and reviewing common pitfalls, you can streamline your development process.
If you're still encountering issues after following this guide, be sure to check the Google API documentation for more detailed information or to engage with community forums for additional support.
Remain persistent, and you'll soon harness the full potential of Google's robust services without interruption! Happy coding!
Checkout our other articles