Common Pitfalls with Lombok Constructor Annotations
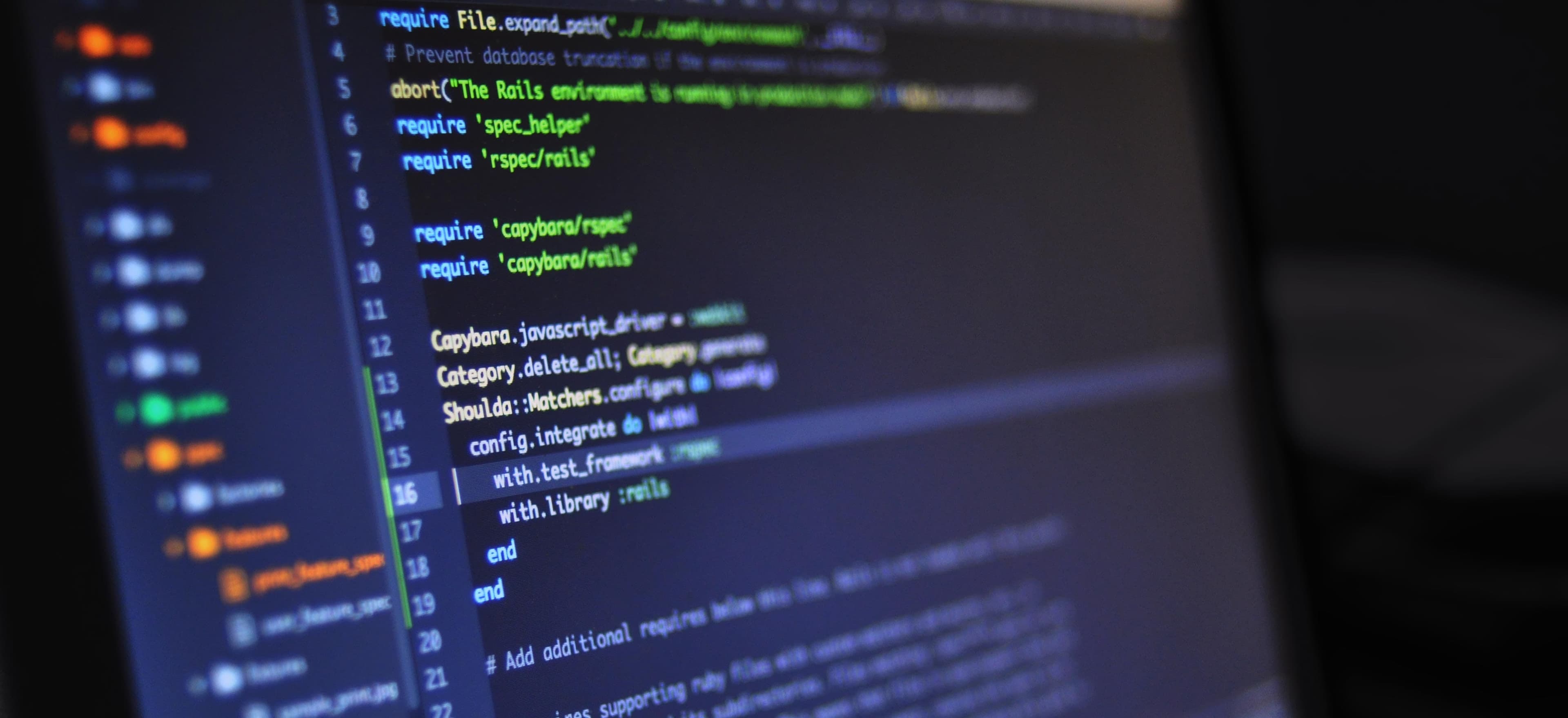
- Published on
Common Pitfalls with Lombok Constructor Annotations
In the realm of Java programming, Lombok has steadily carved out a niche as a powerful tool for reducing boilerplate code. At its heart, Lombok allows developers to leverage annotations to automatically generate methods, reducing the amount of code written and improving readability. One of its prominent features is its constructor annotations: @RequiredArgsConstructor
, @AllArgsConstructor
, and @NoArgsConstructor
. While these annotations simplify object creation, they can also lead to common pitfalls. This post will explore these pitfalls in detail and provide clarity on how to avoid them.
Understanding Lombok Constructor Annotations
Before diving into the pitfalls, let’s clarify what these annotations do:
- @RequiredArgsConstructor: Generates a constructor for all final fields and fields marked as
@NonNull
. - @AllArgsConstructor: Creates a constructor for all fields.
- @NoArgsConstructor: Produces a default constructor with no arguments.
Example Usage
Here’s a simple example demonstrating how these annotations can be implemented:
import lombok.AllArgsConstructor;
import lombok.NoArgsConstructor;
import lombok.RequiredArgsConstructor;
@AllArgsConstructor
@RequiredArgsConstructor
@NoArgsConstructor
public class User {
private String name;
private int age;
private final String email;
// Getters and setters can be generated using Lombok as well
}
In this example, @RequiredArgsConstructor
generates a constructor that accepts the email
field, while @AllArgsConstructor
generates a constructor for all three fields.
Common Pitfalls
While Lombok can significantly streamline Java code, it is essential to be aware of potential issues.
1. Missing NonNull Fields in RequiredArgsConstructor
A common mistake is marking a field as @NonNull
, but forgetting to add it when using the @RequiredArgsConstructor
annotation. This can lead to runtime NullPointerExceptions
.
Solution: Always double-check which fields are required and ensure they are marked correctly.
Example Pitfall
import lombok.NonNull;
import lombok.RequiredArgsConstructor;
@RequiredArgsConstructor
public class Product {
@NonNull
private final String name;
private double price;
}
// Usage
Product product = new Product(null); // Throws NullPointerException
2. Multiple Constructors Creating Ambiguities
When combining multiple constructor annotations, you might inadvertently create constructor ambiguity. For instance, having both @NoArgsConstructor
and @AllArgsConstructor
can potentially lead to confusion when trying to instantiate an object.
Solution: Limit unnecessary constructor overloads based on the use case.
Example Pitfall
import lombok.AllArgsConstructor;
import lombok.NoArgsConstructor;
@NoArgsConstructor
@AllArgsConstructor
public class Product {
private String name;
private double price;
}
// Constructor ambiguity can arise here
Product p1 = new Product(); // Calls no-args constructor
Product p2 = new Product("Gadget", 99.99); // Calls all-args constructor
3. Misunderstanding Default Constructor Behavior
Using @NoArgsConstructor
does not automatically initialize fields with default values, which may lead to unintentional use of null or zero values when creating objects.
Solution: Always set default values or use optional fields where applicable.
Example Pitfall
import lombok.NoArgsConstructor;
@NoArgsConstructor
public class Order {
private String item;
private int quantity;
// item and quantity are not initialized
}
// Usage
Order order = new Order();
System.out.println(order.getItem()); // Prints null
System.out.println(order.getQuantity()); // Prints 0
Tips to Effectively Use Lombok Constructor Annotations
-
Keep It Simple: Only use the necessary constructor annotations for your use case. Avoid cluttering your classes with multiple constructors unless required.
-
Combine Smartly: If a class has complex field requirements, consider using a custom constructor instead of multiple Lombok annotations.
-
Use with Enlightenment: Familiarize yourself with Lombok's behavior, especially regarding
@NonNull
, to prevent null pointer exceptions. -
Consult Documentation: The Lombok Documentation provides a wealth of knowledge about how to use constructor annotations effectively.
Key Takeaways
Lombok offers significant advantages in terms of reducing boilerplate code, particularly with constructor annotations. However, neglecting to understand these annotations can lead to subtle bugs that are hard to track down. By being mindful of common pitfalls and keeping best practices in mind, Java developers can leverage Lombok to enhance productivity and code maintainability.
As Java continues to evolve, the use of tools like Lombok will likely become even more commonplace. Understanding both its strengths and limitations will empower developers to write clean and effective code while avoiding potential missteps.
For more on object-oriented principles with Java and effective methods to maintain code cleanliness, consider checking out resources such as Effective Java by Joshua Bloch and Clean Code by Robert C. Martin.
Happy coding!
Checkout our other articles