Building Spring Integration Apps: Common Pitfalls to Avoid
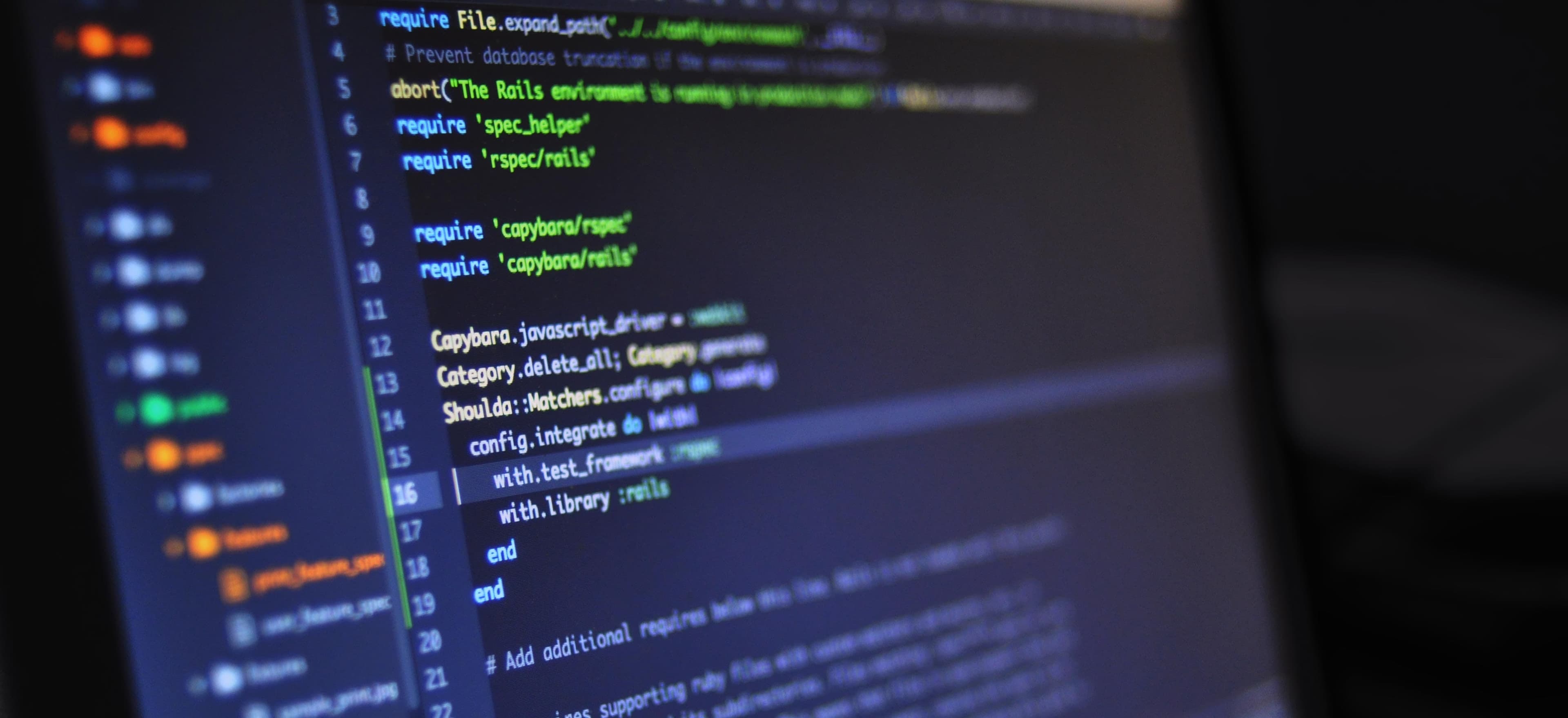
- Published on
Building Spring Integration Apps: Common Pitfalls to Avoid
In the world of software development, Spring Integration stands out as a powerful framework that facilitates the integration of different enterprise systems. The flexibility it offers allows developers to create complex integration flows that connect various applications seamlessly. However, navigating this vast landscape can be challenging. Here, we will delve into common pitfalls when building Spring Integration apps and provide solutions that can lead you to success.
Understanding Spring Integration
Before diving into pitfalls, it's useful to understand what Spring Integration is. It’s an extension of the Spring framework that specializes in messaging and integration patterns. This framework enables applications to communicate with each other efficiently via message channels, enabling both synchronous and asynchronous interactions.
The architecture employs various components such as:
- Channels: Pipes through which the messages travel.
- Message Endpoints: Points where messages are sent or received.
- Message Transformers: Functions that convert messages from one format to another.
- Service Activators: Components that implement business logic.
By defining these components properly, developers can create powerful integration applications.
Common Pitfalls and How to Avoid Them
Now that we have an introduction, let’s dive into the most common pitfalls when building Spring Integration apps and how to effectively avoid them.
1. Overcomplicating the Integration Flow
Pitfall: Developers often try to create complex integration flows, thinking it would enhance capability. However, convoluted flows lead to maintenance nightmares and can introduce bugs.
Solution: Keep it simple. Break down your integration flow into manageable parts. Use the principles of microservices by focusing on individual services that can communicate through APIs or message channels.
// Simple integration flow using Spring Integration
@Bean
public IntegrationFlow simpleFlow() {
return IntegrationFlows.from("inputChannel")
.handle("myService", "process")
.get();
}
In the above code snippet, we define a straightforward integration flow. The focus is on what the service does (process) instead of overwhelming it with functionality.
2. Ignoring Message Transformation
Pitfall: Failing to consider message formats can lead to incompatibility issues between systems, making it difficult to communicate effectively.
Solution: Understand the data formats your systems require and incorporate message transformers to convert between formats as needed.
// Using a Transformer to convert String messages to JSON
@Bean
public IntegrationFlow messageTransformFlow() {
return IntegrationFlows.from("inputChannel")
.transform(String.class, message -> {
// Convert String to JSON
return convertToJson(message);
})
.channel("outputChannel")
.get();
}
private String convertToJson(String message) {
// Example conversion logic
return "{ \"message\": \"" + message + "\" }";
}
This transformation ensures that the systems consuming messages can operate without problems due to format discrepancies.
3. Neglecting Error Handling
Pitfall: Errors in integration flows can cause significant issues if not properly handled. Neglecting error handling can lead to data loss or system crashes.
Solution: Implement robust error handling strategies, such as retries and dead-letter channels. This way, you ensure that messages that fail will not disrupt your processes or lead to data corruption.
@Bean
public IntegrationFlow errorHandlingFlow() {
return IntegrationFlows.from("inputChannel")
.handle("myService", "process")
.route("payload -> (payload.error ? 'errorChannel' : 'outputChannel')",
mapping -> mapping
.subFlowMapping("errorChannel", sf -> sf.handle("errorService", "handleError")))
.get();
}
This flow includes a routing mechanism that sends errors to a dedicated error handling service, effectively segregating issues without affecting the main data flow.
4. Misconfiguring Message Channels
Pitfall: Message channels are critical in any integration workflow. Misconfiguring them can lead to performance bottlenecks or even message loss.
Solution: Dedicate time to understand and configure channels properly. For high-throughput scenarios, consider using direct channels or queues rather than simple channels.
@Bean
public Executor taskExecutor() {
ThreadPoolTaskExecutor executor = new ThreadPoolTaskExecutor();
executor.setCorePoolSize(5);
executor.setMaxPoolSize(10);
executor.setQueueCapacity(100);
executor.initialize();
return executor;
}
@Bean
public IntegrationFlow asyncFlow() {
return IntegrationFlows.from("inputChannel")
.handle("myService", "process", e -> e.asyncExecutor(taskExecutor()))
.channel("outputChannel")
.get();
}
In this example, we define an asynchronous flow, allowing for better throughput and responsiveness by efficiently managing threads.
5. Not Using Spring Boot Features
Pitfall: Many developers overlook the advantages of Spring Boot when building Spring Integration applications, which can simplify configuration and reduce boilerplate code.
Solution: Take advantage of Spring Boot's auto-configuration and Spring Starter dependencies to streamline your setup.
You can set up a minimal configuration for your Spring Integration app with the following Maven dependency:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-integration</artifactId>
</dependency>
With Spring Boot, you can seamlessly manage components and configuration.
6. Skipping Documentation
Pitfall: It’s common for developers to underestimate the importance of documentation. This leads to confusion, especially in teams, about how the integration flows operate.
Solution: Always document the flow of your integrations. Include details on inputs, outputs, exceptions, and processing logic.
Utilizing tools such as Spring Cloud Data Flow can help you visually document and manage your data pipelines.
The Closing Argument
Building Spring Integration applications can be a rewarding experience, but it's essential to avoid common pitfalls that can hinder your efforts. By keeping the integration flows simple, paying attention to message transformation, implementing robust error handling, and leveraging Spring Boot features, you can create applications that are both effective and maintainable.
Additional Resources
By integrating these practices into your development workflow, you will drive more successful outcomes in your Spring Integration projects. Happy coding!