Choosing between DataNucleus and Hibernate: A Clear Guide
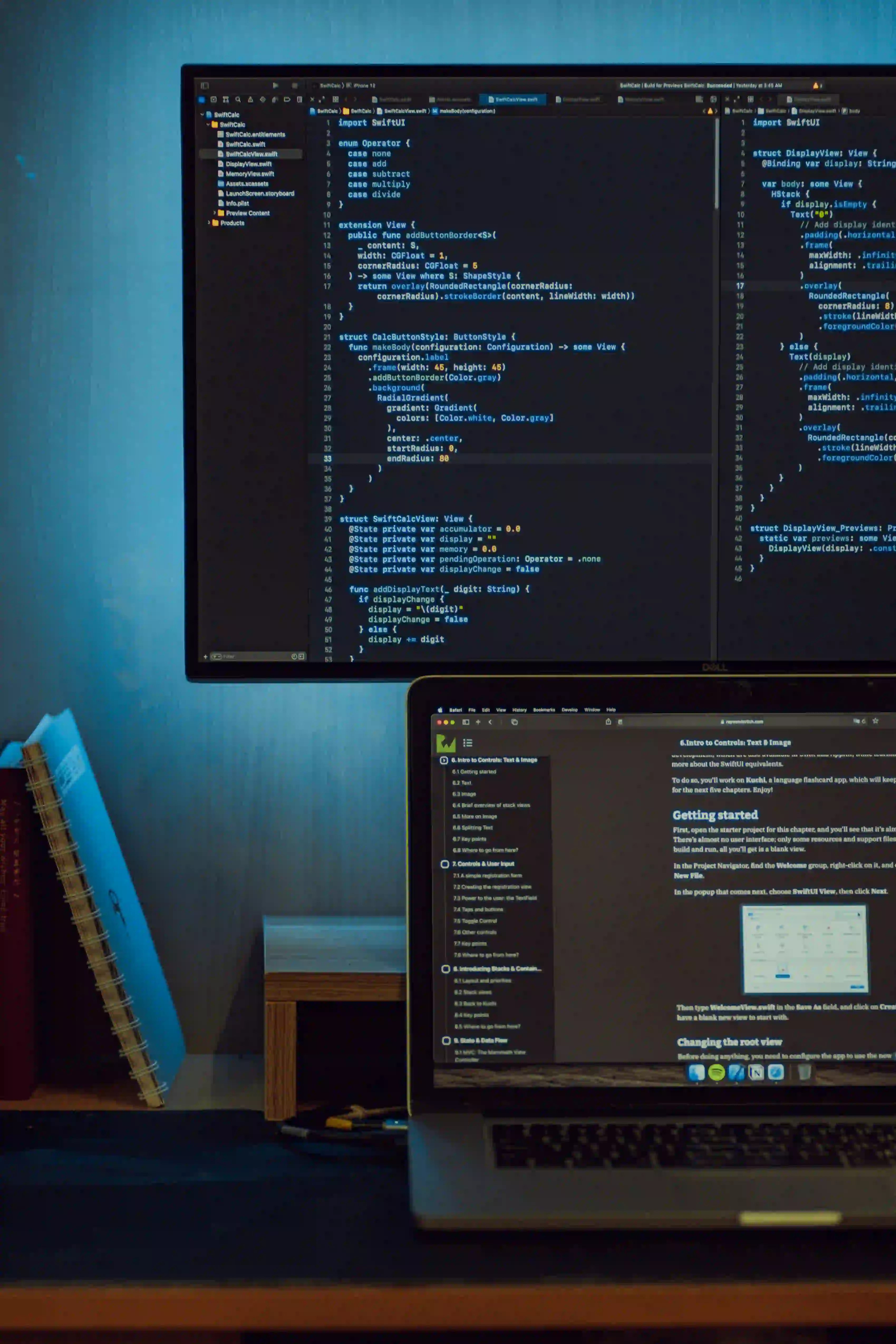
Choosing Between DataNucleus and Hibernate: A Clear Guide
When it comes to Java persistence, developers often find themselves at a crossroads between different Object-Relational Mapping (ORM) frameworks. Two of the most prominent options in this domain are DataNucleus and Hibernate. In this blog post, we'll take a detailed look at both technologies to help you make an informed decision for your project.
What is Object-Relational Mapping (ORM)?
Before diving into the specifics of DataNucleus and Hibernate, it's essential to understand ORM. ORM is a programming technique that facilitates the conversion of data between incompatible type systems in object-oriented programming languages. It allows developers to interact with the database using Java objects rather than SQL queries, promoting clearer and more maintainable code.
Overview of DataNucleus
DataNucleus is a versatile Java data persistence solution that can handle various data stores, including relational databases and NoSQL stores like MongoDB. It supports JPA (Java Persistence API) and JDO (Java Data Objects), making it a great choice for a wide range of applications.
Key Features of DataNucleus
- Support for Multiple Data Stores: Unlike most ORM frameworks, DataNucleus can connect to various underlying data sources, giving developers flexibility.
- JPA and JDO Compliance: Its adherence to standards allows for greater interoperability.
- Custom Query Language: DataNucleus offers support for its custom query language, JPQL (Java Persistence Query Language) and JDOQL (Java Data Objects Query Language).
Overview of Hibernate
Hibernate is one of the most popular ORM frameworks in Java development. It simplifies database operations by mapping Java objects to database tables, significantly reducing boilerplate code.
Key Features of Hibernate
- HQL (Hibernate Query Language): Hibernate provides a powerful query language that allows you to execute queries using object-oriented syntax.
- Caching: Integrated caching mechanisms enhance performance by reducing database access.
- Wide Community Support: Being an established framework, Hibernate has extensive community and documentation support.
Comparison of DataNucleus and Hibernate
To help you make an informed choice between DataNucleus and Hibernate, let’s compare their capabilities along several dimensions.
1. Performance
-
Hibernate: Known for its efficient caching system, Hibernate can handle complex queries without heavy performance implications. It optimizes SQL generation and minimizes database calls.
-
DataNucleus: While DataNucleus has a considerable performance footprint, it can vary based on the data store used. Its performance is generally acceptable for most use cases but may lag behind Hibernate in complex scenarios.
2. Complexity
-
Hibernate: The learning curve for Hibernate can be steep due to its vast array of features such as caching, lazy loading, and session management. However, once understood, it offers a rich set of functionalities.
-
DataNucleus: DataNucleus is less complex, especially if your project requires multiple data stores. Yet, it may lack certain advanced features that Hibernate provides.
3. Community and Documentation
-
Hibernate: Boasting a vast and active community, Hibernate has extensive documentation, examples, and support resources available.
-
DataNucleus: While it has a dedicated community, the resources and examples are not as abundant as Hibernate’s.
4. Integration
-
Hibernate: It easily integrates with various Spring modules. If you’re using Spring Boot, Hibernate is often a preferred option due to built-in support.
-
DataNucleus: It can be integrated into applications that use other frameworks, but the support and resources may not be as robust as with Hibernate.
5. Use Cases
-
Hibernate: Best suited for traditional relational databases where extensive functionalities (caching, transactions) are needed.
-
DataNucleus: Ideal for applications needing multi-database support, including NoSQL stores.
Code Snippets: DataNucleus vs Hibernate
To further illustrate, let us examine simple examples of how to use DataNucleus and Hibernate.
DataNucleus Example
Assuming we want to persist a simple User
entity with DataNucleus:
import javax.jdo.*;
import javax.jdo.annotations.*;
@PersistenceCapable
public class User {
@PrimaryKey
@Persistent(valueStrategy = IdGeneratorStrategy.IDENTITY)
private Long id;
@Persistent
private String name;
public User(String name) {
this.name = name;
}
// Getters and Setters
}
// Persistence code to save the user
PersistenceManagerFactory pmf = JDOHelper.getPersistenceManagerFactory("YourDataNucleusConfig");
PersistenceManager pm = pmf.getPersistenceManager();
try {
pm.makePersistent(new User("John Doe"));
} finally {
pm.close();
}
Commentary
In this snippet:
- The
@PersistenceCapable
annotation marks the class as a persistent entity. - A persistence manager is created to handle the transaction.
- The code effectively saves a new
User
instance, demonstrating DataNucleus's friendly syntax.
Hibernate Example
Now let’s perform the same operation using Hibernate:
import javax.persistence.*;
@Entity
@Table(name = "users")
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
public User(String name) {
this.name = name;
}
// Getters and Setters
}
// Persistence code to save the user
SessionFactory factory = new Configuration().configure("hibernate.cfg.xml").buildSessionFactory();
Session session = factory.openSession();
try {
session.beginTransaction();
session.save(new User("John Doe"));
session.getTransaction().commit();
} finally {
session.close();
}
Commentary
In this Hibernate example:
- An
@Entity
annotation marks the class as a database entity. - The session is used to manage transactions, making the code straightforward and efficient.
- It showcases how Hibernate abstracts away SQL-related boilerplate.
Final Thoughts
When deciding between DataNucleus and Hibernate, your choice should be guided by your project requirements. If you need support for multiple data stores or a simpler ORM, DataNucleus may be your best bet. On the other hand, if you expect a robust performance in a relational context and require a wealth of community support, Hibernate is the clear leader.
Additional Resources
By weighing the strengths and weaknesses discussed, you’ll be better prepared to choose the right ORM for your needs. Remember that whatever option you choose, understanding its nuances will significantly benefit the overall architecture and maintainability of your application.