How to Handle JUnit Pass Test Cases on Failures?
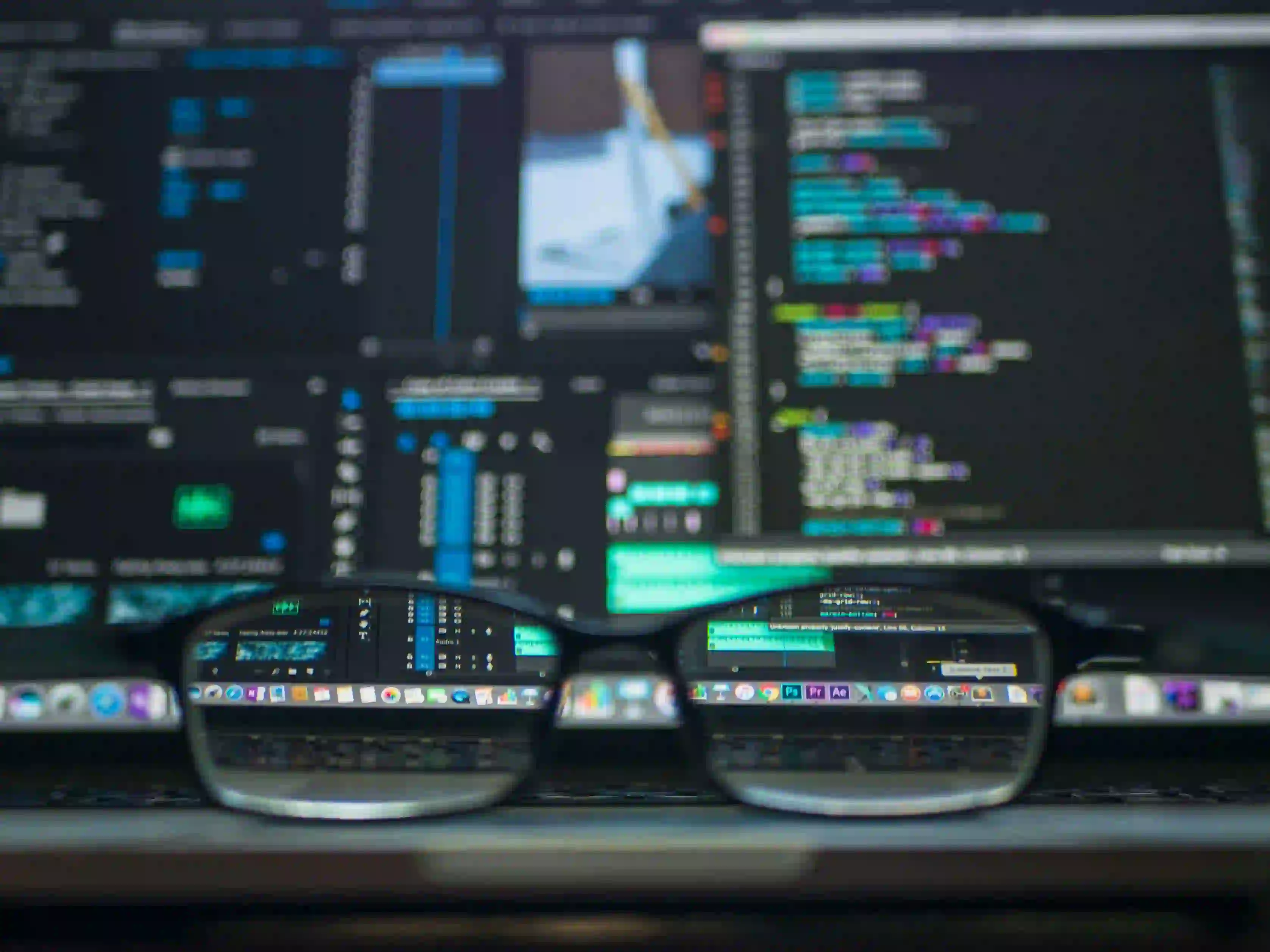
How to Handle JUnit Pass Test Cases on Failures
JUnit is a widely-used testing framework for Java. It helps developers ensure the quality and performance of their applications through unit testing. However, sometimes you might find yourself confronting a scenario where you need to handle pass test cases on failures. This blog post aims to dissect this problem and provide effective solutions. By the end of this article, you will be equipped with knowledge about how to deal with resilient test cases and maintain confidence in your testing suite.
Understanding JUnit Test Cases
What is JUnit?
JUnit is a testing framework that allows developers to write and execute repeatable tests. It plays a critical role in Test-Driven Development (TDD), and it serves as a backbone for many continuous integration/continuous deployment (CI/CD) practices. Let's take a look at a simple JUnit test case to get started:
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class CalculatorTest {
@Test
public void testAddition() {
Calculator calculator = new Calculator();
assertEquals(5, calculator.add(2, 3), "2 + 3 should equal 5");
}
}
In this minimal example, we test the addition functionality of the Calculator
class. If calculator.add(2, 3)
returns 5, the test passes. Otherwise, it fails, and JUnit reports it.
The Problem: False Positives in Testing
What Are False Positives?
A false positive in a testing context occurs when a test case reports success while the functionality it tests is not in the expected state. This can happen due to various reasons, including:
- Misleading test assertions
- Possible environmental issues
- Dependency shifts affecting the outcome
It is crucial to identify these false positives to ensure that your tests are reliable. Because if your tests pass when they should not, you risk deploying faulty software.
Real-World Implications
Imagine you have an application that calculates loan interests. You write a test case to verify its correctness. If this test passes even when the code has changed (and no longer produces valid results), you might ship buggy code to production before catching the issue, leading to financial discrepancies.
Strategies for Handling Failures
Now that we understand the implications of false positives, let's explore strategies for effectively handling failures in JUnit tests:
1. Design Robust Assertions
Ensure that assertions in your test cases are robust and cover a broad range of scenarios.
assertEquals(expectedValue, actualValue, "The values should be equal.");
In the above code, adding a message helps in debugging. When the assertion fails, it tells you exactly what was expected and what was actual.
2. Use @Test with Expected Exceptions
JUnit allows you to create tests that will pass only if a certain exception is thrown. This is particularly useful for testing failure scenarios.
@Test(expected = ArithmeticException.class)
public void testDivisionByZero() {
Calculator calculator = new Calculator();
calculator.divide(1, 0);
}
By setting the expected
parameter, you can verify that the correct exception occurred, thus ensuring that your application doesn't fail unexpectedly.
3. Refactor Your Code Regularly
Consistently refactoring your code will help in maintaining test clarity and reliability. A well-structured codebase is easier to test and less likely to introduce bugs.
4. Incorporate Dependency Injection
Managing dependencies effectively can also help mitigate failure risks associated with tests. Using dependency injection allows you to replace real dependencies with mock objects when testing.
public class PaymentProcessor {
private PaymentGateway paymentGateway;
public PaymentProcessor(PaymentGateway paymentGateway) {
this.paymentGateway = paymentGateway;
}
public boolean processPayment(Payment payment) {
// process payment logic
return paymentGateway.process(payment);
}
}
In the above example, you can inject a mocked PaymentGateway
during tests to control the behavior and assertions effectively.
5. Implement Continuous Integration (CI)
Integrating your JUnit tests into a CI pipeline ensures that they are run regularly and reliably. This automated process can catch failures early, thus enhancing your development workflow. Services like Travis CI and Jenkins offer seamless integration for Java applications.
6. Consider Using @TestInstance
JUnit 5 provides the @TestInstance
annotation, allowing you to use instance methods and fields in your tests. This can help retain state between test methods.
@TestInstance(TestInstance.Lifecycle.PER_CLASS)
public class StatefulTest {
private int state = 0;
@Test
public void testIncrement() {
state++;
assertEquals(1, state);
}
@Test
public void testDoubleIncrement() {
state += 2;
assertEquals(2, state);
}
}
This approach can simplify the handling of states, although you need to maintain awareness of potential side-effects across tests.
7. Use Test Fixtures
Deploy test fixtures to prepare a known state before each test runs. This way, you can guarantee that failures will not arise from unanticipated conditions.
@BeforeEach
public void setUp() {
// Initialize necessary states or mock configurations
}
The @BeforeEach
annotation allows you to setup preconditions before each test.
Final Considerations
Handling pass test cases on failures is pivotal for maintaining the integrity of your Java applications. By employing robust assertions, using exception handling effectively, refactoring regularly, and making use of CI tools, you can significantly enhance the reliability of your testing suite.
As you continue to work with JUnit, consider integrating the above strategies into your workflow. Doing so not only improves your testing accuracy but also instills a culture of quality in your development process.
For further reading, check out the official JUnit 5 documentation or Testing in Java for extensive tutorials and best practices.
Now you are equipped to tackle pass test cases on failures confidently! Happy coding!